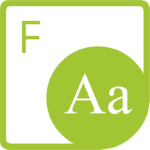
في [الطباعة] الرقمية 1 ، تحدد الخطوط أنماطًا معينة لاستخدامها في مظهر الأحرف. في أغلب الأحيان ، يتم استخدام الخطوط في المستندات وصفحات الويب لتصميم النص. يتم وصف كل خط في ملف يحتوي على معلومات حول حجم الأحرف ووزنها وأنماطها بالإضافة إلى ترميزها. نظرًا لأن الخطوط جزء مهم من تنسيقات الملفات المتنوعة ، فإن Aspose يقدم واجهة برمجة تطبيقات مخصصة للتعامل مع معالجة وعرض أنواع الخطوط الشائعة بما في ذلك TrueType و CFF و OpenType ، و النوع 1. في هذه المقالة ، ستتعلم كيفية تحميل وحفظ واستخراج المعلومات من الخطوط باستخدام C# مع Aspose.Font for .NET.
- NET Font Manipulation and Management API
- تحميل وحفظ خطوط CFF و TrueType و Type1 باستخدام C#
- استخراج معلومات مقاييس الخط باستخدام C#
- كشف الرموز اللاتينية في الخطوط باستخدام C#
NET Font Manipulation API - تنزيل مجاني
Aspose.Font for .NET هي واجهة برمجة تطبيقات محلية تتيح لك تنفيذ ميزات إدارة الخطوط باستخدام C# داخل تطبيقات .NET. إلى جانب التحميل والحفظ واستخراج المعلومات من الخط ، تتيح لك واجهة برمجة التطبيقات أيضًا عرض الحرف الرسومي أو النص إذا لزم الأمر. يمكنك إما تنزيل API أو تثبيته داخل تطبيقات .NET الخاصة بك باستخدام NuGet.
PM> Install-Package Aspose.Font
تحميل أو حفظ الخطوط من ملف باستخدام C#
يمكنك تحميل خط من الملف المخزن على وحدة التخزين الرقمية الخاصة بك لاسترداد معلومات الخط. يعرض Aspose.Font لـ .NET فئات منفصلة للتعامل مع أنواع معينة من الخطوط مثل CFF و TrueType وما إلى ذلك. فيما يلي خطوات تحميل الخط وحفظه.
- قم بتحميل الخط من الملف (باستخدام مسار الملف أو صفيف البايت) باستخدام فئة FontDefinition.
- الوصول إلى معلومات الخط باستخدام CffFont أو TtfFont أو Type1Font class.
- احفظ الملف (إذا لزم الأمر).
تحميل وحفظ خط CFF
يوضح نموذج التعليمات البرمجية التالي كيفية تحميل وحفظ خطوط CFF باستخدام C#.
// للحصول على أمثلة وملفات بيانات كاملة ، يرجى الانتقال إلى https://github.com/aspose-font/Aspose.Font-for-.NET
//مجموعة بايت لتحميل الخط من
string dataDir = RunExamples.GetDataDir_Data();
byte[] fontMemoryData = File.ReadAllBytes(dataDir + "OpenSans-Regular.cff");
FontDefinition fd = new FontDefinition(FontType.CFF, new FontFileDefinition("cff", new ByteContentStreamSource(fontMemoryData)));
CffFont cffFont = Aspose.Font.Font.Open(fd) as CffFont;
//العمل مع البيانات من كائن CffFont الذي تم تحميله للتو
//احفظ CffFont على القرص
//اسم ملف خط الإخراج بالمسار الكامل
string outputFile = RunExamples.GetDataDir_Data() + "OpenSans-Regular_out.cff";
cffFont.Save(outputFile);
تحميل وحفظ خط تروتايب
يوضح نموذج التعليمات البرمجية التالي كيفية تحميل وحفظ خطوط تروتايب باستخدام C#.
// للحصول على أمثلة وملفات بيانات كاملة ، يرجى الانتقال إلى https://github.com/aspose-font/Aspose.Font-for-.NET
//مجموعة بايت لتحميل الخط من
string dataDir = RunExamples.GetDataDir_Data();
byte[] fontMemoryData = File.ReadAllBytes(dataDir + "Montserrat-Regular.ttf");
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new ByteContentStreamSource(fontMemoryData)));
TtfFont ttfFont = Aspose.Font.Font.Open(fd) as TtfFont;
//العمل مع البيانات من كائن TtfFont الذي تم تحميله للتو
//احفظ TtfFont على القرص
//اسم ملف خط الإخراج بالمسار الكامل
string outputFile = RunExamples.GetDataDir_Data() + "Montserrat-Regular_out.ttf";
ttfFont.Save(outputFile);
تحميل وحفظ الخط Type1
يوضح نموذج التعليمات البرمجية التالي كيفية تحميل وحفظ خطوط Type1 باستخدام C#.
// للحصول على أمثلة وملفات بيانات كاملة ، يرجى الانتقال إلى https://github.com/aspose-font/Aspose.Font-for-.NET
string fileName = dataDir + "courier.pfb"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.Type1, new FontFileDefinition("pfb", new FileSystemStreamSource(fileName)));
Type1Font font = Aspose.Font.Font.Open(fd) as Type1Font;
استخراج مقاييس الخط باستخدام C#
يتيح لك Aspose.Font لـ .NET أيضًا استرداد معلومات مقاييس الخط مثل Ascender و Descender و TypoAscender و TypoDescender و UnitsPerEm. فيما يلي خطوات للقيام بذلك.
- استخدم فئة FontDefinition لتحميل خط TrueType أو Type1 من ملف.
- افتح الخط باستخدام فئة نوع الخط المعنية مثل Type1Font و TtfFont وما إلى ذلك.
- الوصول إلى معلومات مقاييس الخط.
توضح نماذج التعليمات البرمجية التالية كيفية استخراج مقاييس الخط من خطوط TrueType و Type1 باستخدام C#.
استخراج المقاييس من خط تروتايب
// للحصول على أمثلة وملفات بيانات كاملة ، يرجى الانتقال إلى https://github.com/aspose-font/Aspose.Font-for-.NET
string fileName = dataDir + "Montserrat-Regular.ttf"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new FileSystemStreamSource(fileName)));
TtfFont font = Aspose.Font.Font.Open(fd) as TtfFont;
string name = font.FontName;
Console.WriteLine("Font name: " + name);
Console.WriteLine("Glyph count: " + font.NumGlyphs);
string metrics = string.Format(
"Font metrics: ascender - {0}, descender - {1}, typo ascender = {2}, typo descender = {3}, UnitsPerEm = {4}",
font.Metrics.Ascender, font.Metrics.Descender,
font.Metrics.TypoAscender, font.Metrics.TypoDescender, font.Metrics.UnitsPerEM);
Console.WriteLine(metrics);
//احصل على جدول ترميز cmap unicode من الخط ككائن TtfCMapFormatBaseTable للوصول إلى معلومات حول الحرف الرسومي للخط للرمز 'A'.
//تحقق أيضًا من أن الخط يحتوي على كائن TtfGlyfTable (جدول 'glyf') للوصول إلى الصورة الرمزية.
Aspose.Font.TtfCMapFormats.TtfCMapFormatBaseTable cmapTable = null;
if (font.TtfTables.CMapTable != null)
{
cmapTable = font.TtfTables.CMapTable.FindUnicodeTable();
}
if (cmapTable != null && font.TtfTables.GlyfTable != null)
{
Console.WriteLine("Font cmap unicode table: PlatformID = " + cmapTable.PlatformId + ", PlatformSpecificID = " + cmapTable.PlatformSpecificId);
//رمز للرمز "أ"
char unicode = (char)65;
//فهرس الحرف الرسومي لـ "A"
uint glIndex = cmapTable.GetGlyphIndex(unicode);
if (glIndex != 0)
{
//Glyph لـ "A"
Glyph glyph = font.GetGlyphById(glIndex);
if (glyph != null)
{
//مقاييس طباعة الصورة الرمزية
Console.WriteLine("Glyph metrics for 'A' symbol:");
string bbox = string.Format(
"Glyph BBox: Xmin = {0}, Xmax = {1}" + ", Ymin = {2}, Ymax = {3}",
glyph.GlyphBBox.XMin, glyph.GlyphBBox.XMax,
glyph.GlyphBBox.YMin, glyph.GlyphBBox.YMax);
Console.WriteLine(bbox);
Console.WriteLine("Width:" + font.Metrics.GetGlyphWidth(new GlyphUInt32Id(glIndex)));
}
}
}
استخراج القياسات من الخط Type1
// للحصول على أمثلة وملفات بيانات كاملة ، يرجى الانتقال إلى https://github.com/aspose-font/Aspose.Font-for-.NET
string fileName = dataDir + "courier.pfb"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.Type1, new FontFileDefinition("pfb", new FileSystemStreamSource(fileName)));
Type1Font font = Aspose.Font.Font.Open(fd) as Type1Font;
string name = font.FontName;
Console.WriteLine("Font name: " + name);
Console.WriteLine("Glyph count: " + font.NumGlyphs);
string metrics = string.Format(
"Font metrics: ascender - {0}, descender - {1}, typo ascender = {2}, typo descender = {3}, UnitsPerEm = {4}",
font.Metrics.Ascender, font.Metrics.Descender,
font.Metrics.TypoAscender, font.Metrics.TypoDescender, font.Metrics.UnitsPerEM);
Console.WriteLine(metrics);
كشف الرموز اللاتينية في الخطوط باستخدام C#
يمكنك أيضًا اكتشاف النص اللاتيني في الخطوط عن طريق فك رموز الرموز الرسومية باستخدام Aspose.Font لـ .NET. فيما يلي خطوات إجراء هذه العملية.
- قم بتحميل الخط من الملف باستخدام فئة FontDefinition.
- فك شفرة GlyphId باستخدام طريقة DecodeToGid() لفئة الخط المعنية.
توضح نماذج التعليمات البرمجية التالية كيفية فك تشفير الرموز اللاتينية في خطوط TrueType و Type1 باستخدام C#.
كشف الرموز اللاتينية في خط تروتايب
// للحصول على أمثلة وملفات بيانات كاملة ، يرجى الانتقال إلى https://github.com/aspose-font/Aspose.Font-for-.NET
string fileName = dataDir + "Montserrat-Regular.ttf"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new FileSystemStreamSource(fileName)));
TtfFont ttfFont = Aspose.Font.Font.Open(fd) as TtfFont;
bool latinText = true;
for (uint code = 65; code < 123; code++)
{
GlyphId gid = ttfFont.Encoding.DecodeToGid(code);
if (gid == null || gid == GlyphUInt32Id.NotDefId)
{
latinText = false;
}
}
if (latinText)
{
Console.WriteLine(string.Format("Font {0} supports latin symbols.", ttfFont.FontName));
}
else
{
Console.WriteLine(string.Format("Latin symbols are not supported by font {0}.", ttfFont.FontName));
}
كشف الرموز اللاتينية في خط Type1
// للحصول على أمثلة وملفات بيانات كاملة ، يرجى الانتقال إلى https://github.com/aspose-font/Aspose.Font-for-.NET
string fileName = dataDir + "courier.pfb"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.Type1, new FontFileDefinition("pfb", new FileSystemStreamSource(fileName)));
Type1Font font = Aspose.Font.Font.Open(fd) as Type1Font;
bool latinText = true;
for (uint code = 65; code < 123; code++)
{
GlyphId gid = font.Encoding.DecodeToGid(code);
if (gid == null || gid == GlyphUInt32Id.NotDefId)
{
latinText = false;
}
}
if (latinText)
{
Console.WriteLine(string.Format("Font {0} supports latin symbols.", font.FontName));
}
else
{
Console.WriteLine(string.Format("Latin symbols are not supported by font {0}.", font.FontName));
}
استنتاج
في هذه المقالة ، رأيت كيفية تحميل وحفظ خطوط CFF و TrueType و Type1 برمجيًا باستخدام C#. علاوة على ذلك ، تعلمت كيفية استخراج معلومات مقاييس الخط من خطوط TrueType و Type1 داخل تطبيقات .NET. يمكنك استكشاف المزيد من الميزات الشيقة التي تقدمها Aspose.Font لـ .NET في التوثيق. لمزيد من التحديثات ، استمر في زيارة Aspose.Font مدونة.