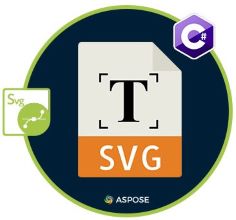
يُستخدم عنصر النص SVG لتعريف نص في SVG. SVG (Scalable Vector Graphics) هو تنسيق ملف متجه صديق للويب. يتم استخدامه لعرض المعلومات المرئية على صفحة ويب. يمكننا بسهولة كتابة أي نص في SVG باستخدام عنصر نص SVG. في هذه المقالة ، سوف نتعلم كيفية تحويل النص إلى SVG في #C.
سيتم تناول الموضوعات التالية في هذه المقالة:
- C# API لتحويل النص إلى SVG
- ما هو نص SVG
- تحويل النص إلى SVG
- تحويل النص باستخدام TSpan إلى SVG
- نص SVG مع TextPath
- تطبيق أنماط نص SVG
C# API لتحويل النص إلى SVG
لتحويل النص إلى SVG ، سنستخدم Aspose.SVG for .NET API. يسمح بتحميل ملفات SVG وتحليلها وعرضها وإنشائها وتحويلها إلى تنسيقات شائعة بدون أي تبعيات للبرامج.
تمثل فئة SVGDocument الخاصة بواجهة برمجة التطبيقات جذر تسلسل SVG الهرمي وتحمل المحتوى بأكمله. لدينا طريقة ()Save في هذه الفئة تسمح بحفظ SVG إلى مسار الملف المحدد. تمثل الفئة SVGTextElement عنصر “النص”. تتوافق الواجهة SVGTSpanElement مع عنصر “tspan”. توفر واجهة برمجة التطبيقات SVGTextPathElement فئة تمثل عنصر “مسار النص” و SVGPathElement لعنصر “المسار”. يمكننا تعيين خصائص / سمات مختلفة لعناصر SVG باستخدام طريقة (سلسلة ، سلسلة)SetAttribute. تضيف طريقة (Node)AppendChild لواجهة برمجة التطبيقات الطفل الجديد للعقدة إلى نهاية قائمة العناصر الفرعية لهذه العقدة.
يرجى إما تنزيل DLL من API أو تثبيته باستخدام NuGet.
PM> Install-Package Aspose.SVG
ما هو نص SVG؟
في SVG ، يتم تقديم النص باستخدام عنصر . بشكل افتراضي ، يتم تقديم النص بتعبئة سوداء وبدون مخطط تفصيلي. يمكننا تحديد السمات التالية:
- x: يحدد موضع النقطة أفقيًا.
- y: يحدد موضع النقطة عموديًا.
- ملء: يحدد لون النص المعروض.
- التحويل: التدوير ، والترجمة ، والانحراف ، والقياس.
تحويل النص إلى SVG باستخدام #C
يمكننا إضافة أي نص إلى SVG باتباع الخطوات الواردة أدناه:
- أولاً ، قم بإنشاء مثيل لفئة SVGDocument.
- بعد ذلك ، احصل على عنصر جذر المستند.
- ثم قم بإنشاء كائن فئة SVGTextElement.
- بعد ذلك ، قم بتعيين سمات مختلفة باستخدام طريقة ()SetAttribute.
- بعد ذلك ، قم باستدعاء طريقة ()AppendChild لإلحاقها بالعنصر الجذر.
- أخيرًا ، احفظ صورة SVG الناتجة باستخدام طريقة ()Save.
يوضح نموذج التعليمات البرمجية التالي كيفية تحويل النص إلى SVG في #C.
// يوضح مثال الكود هذا كيفية إضافة نص إلى SVG.
var document = new SVGDocument();
// الحصول على عنصر svg الجذر للمستند
var svgElement = document.RootElement;
const string @namespace = "http://www.w3.org/2000/svg";
// حدد عنصر نص SVG
var text = (SVGTextElement)document.CreateElementNS(@namespace, "text");
// تحديد النص لإظهاره
text.TextContent = "The is a simple SVG text!";
// تعيين سمات مختلفة
text.SetAttribute("fill", "blue");
text.SetAttribute("x", "10");
text.SetAttribute("y", "30");
// إلحاق نص بالجذر
svgElement.AppendChild(text);
// حفظ باسم SVG
document.Save(@"C:\Files\simple-text.svg");
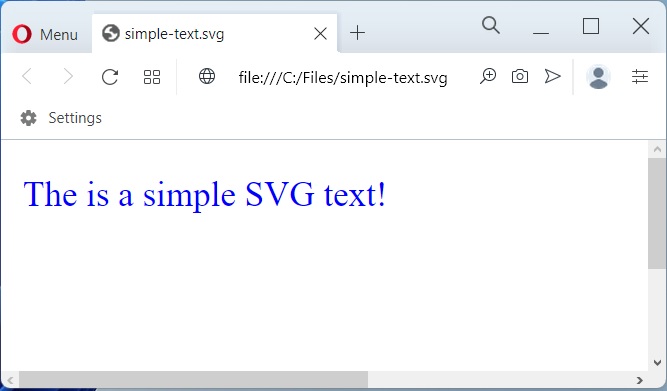
تحويل النص إلى SVG باستخدام C#.
تجدون أدناه محتوى صورة simple-text.svg.
<svg xmlns="http://www.w3.org/2000/svg">
<text fill="blue" x="10" y="30">The is a simple SVG text!</text>
</svg>
تحويل النص باستخدام TSpan إلى SVG في #C
يحدد عنصر SVG نصًا فرعيًا داخل عنصر . يمكننا إضافة أي نص يحتوي على عنصر tspan إلى SVG باتباع الخطوات الواردة أدناه:
- أولاً ، قم بإنشاء مثيل لفئة SVGDocument.
- بعد ذلك ، احصل على عنصر جذر المستند.
- ثم قم بإنشاء كائن فئة SVGTextElement.
- اختياريًا ، قم بتعيين سمات متنوعة باستخدام طريقة ()SetAttribute.
- بعد ذلك ، حدد كائن SVGTSpanElement.
- بعد ذلك ، قم بتعيين سماته باستخدام طريقة ()SetAttribute.
- الآن ، قم باستدعاء طريقة ()AppendChild لإلحاقها بعنصر SVGTextElement.
- كرر الخطوات المذكورة أعلاه لإضافة المزيد من كائنات SVGTSpanElement.
- بعد ذلك ، قم باستدعاء طريقة ()AppendChild لإلحاق SVGTextElement بالعنصر الجذر.
- أخيرًا ، احفظ صورة SVG الناتجة باستخدام طريقة ()Save.
يوضح نموذج التعليمات البرمجية التالي كيفية تحويل النص باستخدام tspan إلى SVG في #C.
// يوضح مثال الكود هذا كيفية إضافة نص باستخدام tspan إلى SVG.
var document = new SVGDocument();
// الحصول على عنصر svg الجذر للمستند
var svgElement = document.RootElement;
const string @namespace = "http://www.w3.org/2000/svg";
// عنصر نص SVG
var text = (SVGTextElement)document.CreateElementNS(@namespace, "text");
text.SetAttribute("style", "font-family:arial");
text.SetAttribute("x", "20");
text.SetAttribute("y", "60");
// عنصر SVG TSpan
var tspan1 = (SVGTSpanElement)document.CreateElementNS(@namespace, "tspan");
tspan1.TextContent = "ASPOSE";
tspan1.SetAttribute("style", "font-weight:bold; font-size:55px");
tspan1.SetAttribute("x", "20");
tspan1.SetAttribute("y", "60");
// ألحق بنص SVG
text.AppendChild(tspan1);
// عنصر TSpan آخر
var tspan2 = (SVGTSpanElement)document.CreateElementNS(@namespace, "tspan");
tspan2.TextContent = "Your File Format APIs";
tspan2.SetAttribute("style", "font-size:20px; fill:grey");
tspan2.SetAttribute("x", "37");
tspan2.SetAttribute("y", "90");
// إلحاق بنص SVG
text.AppendChild(tspan2);
// قم بإلحاق نص SVG بالجذر
svgElement.AppendChild(text);
// احفظ ملف SVG
document.Save(@"C:\Files\svg-tSpan.svg");
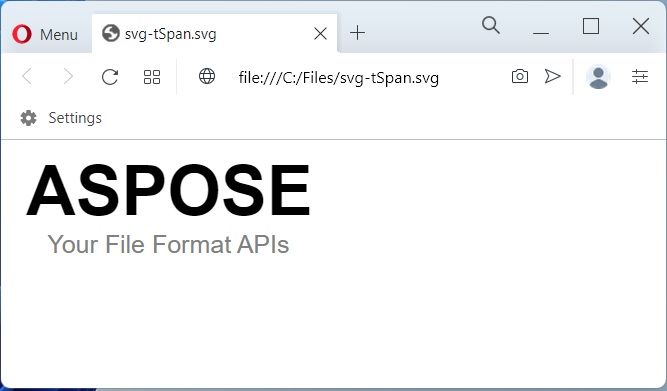
تحويل النص مع tspan إلى SVG في C#.
تجدون أدناه محتوى الصورة svg-tspan.svg.
<svg xmlns="http://www.w3.org/2000/svg">
<text style="font-family: arial;" x="20" y="60">
<tspan style="font-weight: bold; font-size: 55px;" x="20" y="60">ASPOSE</tspan>
<tspan style="font-size: 20px; fill: grey;" x="37" y="90">Your File Format APIs</tspan>
</text>
</svg>
نص SVG مع TextPath في #C
يمكننا أيضًا عرض النص على طول شكل عنصر ، وإحاطة النص في عنصر . يسمح بتعيين مرجع لعنصر باستخدام سمة href. يمكننا تحويل النص بمسارات النص باتباع الخطوات الواردة أدناه:
- أولاً ، قم بإنشاء مثيل لفئة SVGDocument.
- بعد ذلك ، احصل على عنصر جذر المستند.
- بعد ذلك ، قم بإنشاء كائن فئة SVGPathElement وقم بتعيين سمات متنوعة باستخدام طريقة ()SetAttribute.
- بعد ذلك ، قم باستدعاء طريقة ()AppendChild لإلحاقها بالعنصر الجذر.
- بعد ذلك ، قم بإنشاء كائن فئة SVGTextElement.
- بعد ذلك ، قم بتهيئة كائن SVGTextPathElement وقم بتعيين محتوى النص.
- بعد ذلك ، قم بتعيين SVGPathElement إلى سمة href الخاصة به باستخدام طريقة ()SetAttribute.
- ثم ، قم باستدعاء الأسلوب ()AppendChild لإلحاق SVGTextPathElement بعنصر SVGTextElement.
- بعد ذلك ، قم بإلحاق SVGTextElement بالعنصر الجذر باستخدام طريقة AppendChild ().
- أخيرًا ، احفظ صورة SVG الناتجة باستخدام طريقة ()Save.
يوضح نموذج التعليمات البرمجية التالي كيفية تحويل النص باستخدام textPath إلى SVG في #C.
// يوضح مثال الكود هذا كيفية إضافة نص باستخدام textPath إلى SVG.
var document = new SVGDocument();
// الحصول على عنصر svg الجذر للمستند
var svgElement = document.RootElement;
const string @namespace = "http://www.w3.org/2000/svg";
// عنصر مسار SVG
var path1 = (SVGPathElement)document.CreateElementNS(@namespace, "path");
path1.SetAttribute("id", "path_1");
path1.SetAttribute("d", "M 50 100 Q 25 10 180 100 T 350 100 T 520 100 T 690 100");
path1.SetAttribute("fill", "transparent");
// قم بإلحاق مسار SVG بالعنصر الجذر
svgElement.AppendChild(path1);
// Another عنصر مسار SVG
var path2 = (SVGPathElement)document.CreateElementNS(@namespace, "path");
path2.SetAttribute("id", "path_2");
path2.SetAttribute("d", "M 50 100 Q 25 10 180 100 T 350 100");
path2.SetAttribute("transform", "translate(0,75)");
path2.SetAttribute("fill", "transparent");
// قم بإلحاق مسار SVG بالعنصر الجذر
svgElement.AppendChild(path2);
// عنصر نص SVG
var text = (SVGTextElement)document.CreateElementNS(@namespace, "text");
// قم بإنشاء عنصر مسار نص SVG
var textPath1 = (SVGTextPathElement)document.CreateElementNS(@namespace, "textPath");
textPath1.TextContent = "Aspose.SVG for .NET is flexible library for SVG files processing and fully compatible with its specifications.";
textPath1.SetAttribute("href", "#path_1");
// قم بإلحاق مسار نص SVG بنص SVG
text.AppendChild(textPath1);
// عنصر مسار نص SVG آخر
var textPath2 = (SVGTextPathElement)document.CreateElementNS(@namespace, "textPath");
textPath2.TextContent = "Aspose.SVG for .NET is flexible library for SVG files processing and fully compatible with its specifications.";
textPath2.SetAttribute("href", "#path_2");
// قم بإلحاق مسار نص SVG بنص SVG
text.AppendChild(textPath2);
// قم بإلحاق نص SVG بالجذر
svgElement.AppendChild(text);
// احفظ ملف SVG
document.Save(@"C:\Files\svg-textPath.svg");
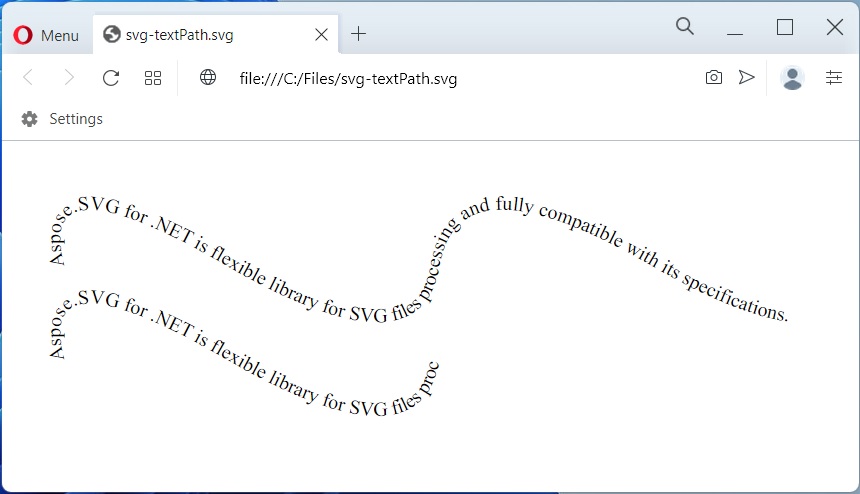
تحويل النص باستخدام textPath إلى SVG في C#.
تجدون أدناه محتوى صورة svg-textPath.svg.
<svg xmlns="http://www.w3.org/2000/svg">
<path id="path_1" d="M 50 100 Q 25 10 180 100 T 350 100 T 520 100 T 690 100" fill="transparent"/>
<path id="path_2" d="M 50 100 Q 25 10 180 100 T 350 100" transform="translate(0,75)" fill="transparent"/>
<text>
<textPath href="#path_1">Aspose.SVG for .NET is flexible library for SVG files processing and fully compatible with its specifications.</textPath>
<textPath href="#path_2">Aspose.SVG for .NET is flexible library for SVG files processing and fully compatible with its specifications.</textPath>
</text>
</svg>
تطبيق نمط نص SVG في #C
يمكننا إضافة أي نص إلى SVG باتباع الخطوات الواردة أدناه:
- أولاً ، قم بإنشاء مثيل لفئة SVGDocument.
- بعد ذلك ، احصل على عنصر جذر المستند.
- ثم قم بإنشاء كائن فئة SVGTextElement.
- بعد ذلك ، قم بتعيين سمة النمط باستخدام طريقة ()SetAttribute.
- بعد ذلك ، قم باستدعاء طريقة ()AppendChild لإلحاقها بالعنصر الجذر.
- أخيرًا ، احفظ صورة SVG الناتجة باستخدام طريقة ()Save.
يوضح نموذج التعليمات البرمجية التالي كيفية تطبيق أنماط CSS على نص SVG في #C.
// يوضح مثال الكود هذا كيفية إضافة نص إلى SVG وتطبيق سمات نمط CSS.
var document = new SVGDocument();
// الحصول على عنصر svg الجذر للمستند
var svgElement = document.RootElement;
const string @namespace = "http://www.w3.org/2000/svg";
// حدد عنصر نص SVG
var text = (SVGTextElement)document.CreateElementNS(@namespace, "text");
// تحديد النص لإظهاره
text.TextContent = "The is a simple SVG text!";
// تعيين سمات مختلفة
text.SetAttribute("fill", "blue");
text.SetAttribute("x", "10");
text.SetAttribute("y", "30");
// تطبيق النمط
text.SetAttribute("style", "font-weight:bold; font-style: italic; text-decoration: line-through; text-transform: capitalize;");
// إلحاق نص بالجذر
svgElement.AppendChild(text);
// حفظ باسم SVG
document.Save(@"C:\Files\text-style.svg");
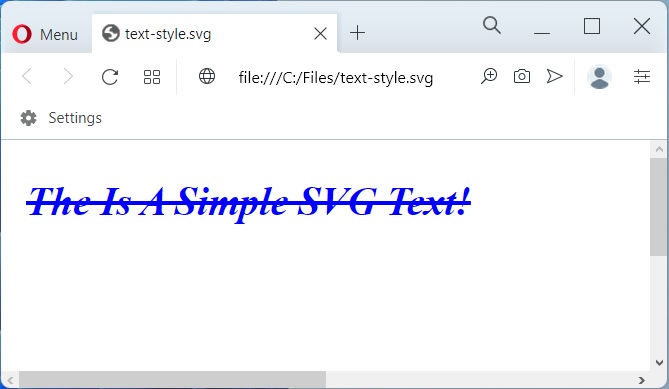
قم بتطبيق نمط نص SVG في C#.
تجدون أدناه محتوى صورة text-style.svg.
<svg xmlns="http://www.w3.org/2000/svg">
<text fill="blue" x="10" y="30" style="font-weight: bold; font-style: italic; text-decoration-line: line-through; text-transform: capitalize;">The is a simple SVG text!</text>
</svg>
احصل على رخصة مؤقتة مجانية
يمكنك الحصول على ترخيص مؤقت مجاني لتجربة Aspose.SVG لـ .NET بدون قيود تقييم.
استنتاج
في هذه المقالة ، تعلمنا كيفية:
- إنشاء صورة SVG جديدة ؛
- استخدام عناصر نص SVG ؛
- تقديم نص SVG إلى المسار ؛
- تعيين موضع وملء سمات نص SVG ؛
- تعيين سمات النمط لنص SVG في #C.
إلى جانب تحويل النص إلى SVG في #C ، يمكنك معرفة المزيد حول Aspose.SVG for .NET في التوثيق واستكشاف الميزات المختلفة التي تدعمها واجهة برمجة التطبيقات. في حالة وجود أي غموض ، لا تتردد في الاتصال بنا على منتدى الدعم المجاني.