In this article, we’ll show you how to generate barcodes programmatically using Java. Furthermore, you will also learn how to scan and read different types of barcodes using Java.

Barcode technology is a popular and widely used way to visually represent data about objects in the form of different patterns. The barcode is the encoded and machine-readable form of the data that can be decoded or read using the barcode scanners. These days, almost every product contains a barcode that can be scanned to retrieve information about that product. Furthermore, the increasing number of online businesses has also influenced the usage of different types of barcodes in the purchase process. Keeping an eye on today’s trends, this article aims to show you how to generate and scan various types of barcodes within your Java applications using Aspose’s barcode generator and scanner library.
In this article, you will learn how to:
- Barcode Generator and Reader API in Java
- Generate a barcode using Java
- Java QR Code Generator
- Create barcodes with a customized appearance using Java
- Create barcodes with a caption using Java
- Scan and read barcodes in Java
Barcode Generator and Reader API in Java
In order to generate barcodes in Java-based applications, i.e., console applications and Java Swing applications, Aspose.BarCode for Java has proved to be a flexible and feature-rich Java barcode generator and scanner library. You can download the JAR of the API or install it in your Maven-based application using the following configurations:
Repository:
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
Dependency:
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-barcode</artifactId>
<version>20.3</version>
<classifier>jdk17</classifier>
</dependency>
Generate a Barcode using Java
The following are the simple steps to generate a barcode as an image.
- Create an object of the BarcodeGenerator class.
- Initialize the BarcodeGenerator object with the encoding type and the text to encode.
- Set the resolution of the resultant image (optional).
- Generate a barcode using the BarcodeGenerator.save(string) method.
The following code sample shows how to generate a barcode using Java.
Generated Barcode

Java QR Code Generator
You can generate a variety of barcode types using Aspose.BarCode for Java. The supported barcode symbologies of the API include, but are not limited to:
- Code128
- Code11
- Code39
- QR
- Datamatrix
- EAN13
- EAN8
- ITF14
- PDF417
- and more.
You can generate any of the above-mentioned barcode types by specifying the respective encoding type using the EncodeTypes parameter while initializing the BarcodeGenerator’s object. For the demonstration, we’ll generate a QR barcode.
The following code sample shows how to generate a QR barcode in Java:
Generated QR Code

Generate Customized Barcode using Java
By default, the barcodes are generated in a black and white color combination. However, in certain cases, you may want or need to customize its appearance. Aspose.BarCode for Java lets you customize the barcode’s forecolor, back color, text’s color, font, and etc. The following is the recipe to generate a customized barcode.
- Create an object of the BarcodeGenerator class.
- Initialize BarcodeGenerator with the encoding type and the text to encode.
- Access and set the parameters related to the appearance of the barcode using the BarcodeGenerator.getParameters().
- Generate barcode using the BarcodeGenerator.save(string) method.
The following code sample shows how to generate an AZTEC barcode with a customized appearance using Java.
Barcode with Customized Appearance

Generate Barcodes with Captions in Java
The barcode images may also contain their captions. You can add the caption below the barcode, above the barcode, or at both of the locations at the same time. The following steps are used to set captions for a barcode:
- Create an object of the BarcodeGenerator class and initialize it with the encoding type and the code text.
- Access and set the captions using the BarcodeGenerator.getParameters().getCaptionAbove().setText() or getCaptionBelow().setText() methods.
- Set the visibility of the captions using the setVisible() method.
- Generate barcode using the BarcodeGenerator.save(string) method.
The following code sample shows how to generate a barcode with a caption in Java.
Barcode with Captions

Read or Scan Barcode in Java
Along with generating the barcodes, you can also scan the barcode images to decode and read the information and data they contain. Since an image may contain more than one barcode, you may access and read all of them at the same time. The following are the steps to scan and read a barcode image:
- Create an object of the BarCodeReader class and initialize it with the path of the barcode image file.
- You can also pass the DecodeType as the second parameter to BarCodeReader’s constructor to read the barcodes with a particular symbology only.
- Read the barcodes using the BarCodeReader.readBarCodes() method.
The following code sample shows how to scan and read barcode images in Java.
Output
CodeText: Aspose.BarCode
Symbology type: Code128
Get a Free License
You can get a free temporary license to test all of the features of the API without any evaluation limitations.
Barcode Generator Online
You may also use this free online barcode generator web app to generate various types of barcodes.
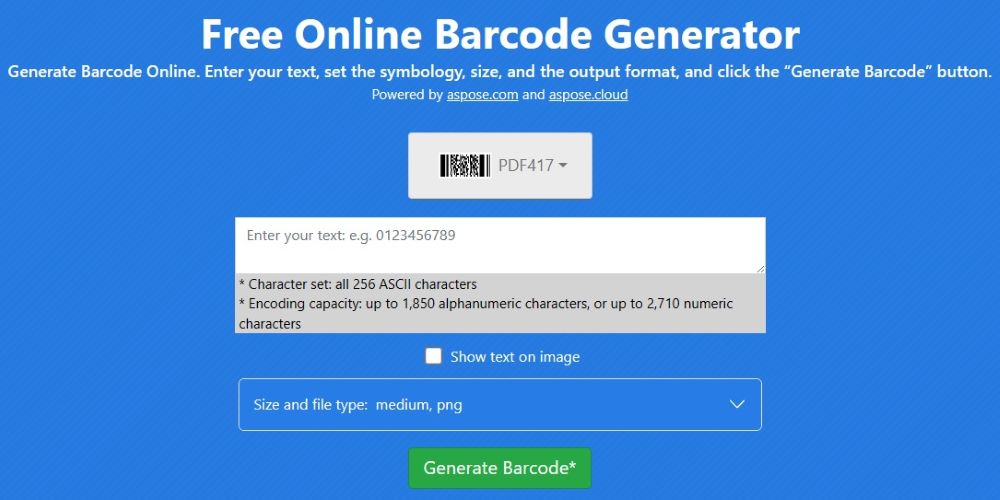
Barcode Generator – Free Learning Resources
You can learn more about generating and reading barcodes and explore other features of the API using the resources below:
Conclusion
In this article, we have seen how to generate different types of barcodes using Java. The step by step guide and code samples demonstrated how to customize the barcode’s appearance and scan the barcodes within Java applications. In case of any ambiguity, please feel free to contact us on our free support forum.