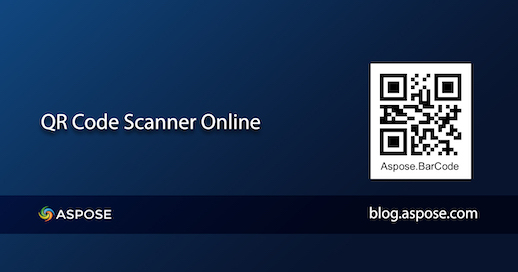
This article explains how to scan a QR code online from an image in any format like JPG, PNG, etc. You do not need any installation or configuration of any tool or application to use the QR Code scanner. Moreover, you will also learn the Developer’s Guide for creating a QR Code reader application in your C#, Java, or Python environment as per your requirements.
You can find all the information about QR Scanner covered under the following sections:
- QR Code Scanner Online
- How to Scan QR Code Online
- Read QR Code in C#
- Recognize QR Code in Java
- Scan QR Code in Python
- Get a Free License for QR Code Scanner
- QR Code Barcode Reader - Learning Resources
QR Code Scanner Online
QR Codes are popularly used to automate different tasks. For instance, many products can contain QR Codes for better inventory management or different counter operations. Similarly, contact numbers or cash payments can be processed using QR Codes. Therefore, you may need a QR code scanner to recognize barcodes online. You can use this free QR Code Scanner online to process QR Codes without any sign-in or registration. It can be used to read any number of QR codes within a few seconds.
Concerned about the data security of your files? Your data is secure as it is deleted from our servers after 24 hours.
How to Scan QR Code Online
You may scan a QR code online with the following steps:
- First, you need to choose the input medium from an image or use the camera of your computer.
- Drag and drop the file or upload the input image.
- Scan the image where you can also select ‘Fast’, ‘Normal’, or ‘Excellent’ recognition.
Read QR Code in C#
You can read a QR Code by following the steps below:
- Create an instance of the BarCodeReader class.
- Initialize an object of BarCodeResult class.
- Read the QR Code.
The code snippet below demonstrates how to read QR code in C#:
using (BarCodeReader reader = new BarCodeReader("QR.png", DecodeType.QR))
{
foreach (BarCodeResult result in reader.ReadBarCodes())
Console.WriteLine($"{result.CodeTypeName}:{result.CodeText}");
}
Recognize QR Code in Java
You can recognize a QR code with the following steps:
- Initialize an object of the BarCodeReader class.
- Instantiate an instance of BarCodeResult class.
- Recognize QR Code and get the output text.
The following code snippet shows how to recognize QR code in Java:
// Create an instance of BarCodeReader class
BarCodeReader reader = new BarCodeReader("input.png", DecodeType.QR);
for (BarCodeResult result : reader.readBarCodes()) {
System.out.println("BarCode CodeText: " + result.getCodeText());
System.out.println("BarCode CodeType: " + result.getCodeTypeName());
}
Scan QR Code in Python
Please follow the steps below to scan a QR code in Python:
- Create an object of the BarCodeReader class.
- Get recognition results with the read_bar_codes() method.
- Finally, get the recognized QR code text.
The code snippet below demonstrates how to scan QR code in Python:
import aspose.barcode as barcode
# Load QR code image
reader = barcode.barcoderecognition.BarCodeReader("C:\\Files\\Sample_qr.jpg")
# Read QR codes
recognized_results = reader.read_bar_codes()
# Show results
for x in recognized_results:
print("Code Text: " + x.code_text)
print("Type: " + x.code_type_name)
Get a Free License for QR Code Scanner
You may request a free temporary license to evaluate the API without any limitations or a watermark.
QR Code Barcode Reader - Learning Resources
You can explore working with QR codes like generating or scanning QR codes by going through the following resources:
Conclusion
In conclusion, this article explains QR Code Scanner Free Online for recognizing QR codes from an image or the camera. Similarly, it covers the developer’s guide for creating a QR Code scanner or reader in C#, Java, or Python as per your preferences. However, in case of any queries, please feel free to reach out to us at the free support forum.