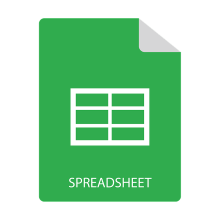
While generating Excel files, often we have to adjust the size of the rows and columns according to the length of the text. MS Excel allows you to enable auto-fitting of rows and columns for such cases. In this article, we will demonstrate how to auto-fit rows and columns in Excel files programmatically in C#. The steps and code samples will make you implement this feature in your .NET applications seamlessly. So let’s begin.
- C# API for Auto-Fitting Rows and Columns in Excel
- Steps to Auto-fit Excel Rows and Columns in C#
- Auto-Fit Rows in Excel in C#
- Auto-Fit Columns in Excel in C#
C# API to Auto-Fit Rows and Columns in Excel - Free Download
To auto-fit Excel rows and columns, we will use Aspose.Cells for .NET. The API provides a rich set of features to generate and process spreadsheets. You can either download the API’s DLL or install it from NuGet.
PM> Install-Package Aspose.Cells
Steps to Auto-fit Excel Rows and Columns in C#
To auto-fit rows or columns, you first need to access the Excel sheet and then use the row and column index to enable auto-fitting. The following are the steps to auto-fit a row or column in Excel using Aspose.Cells for .NET.
- Load the Excel file from the disk.
- Get the reference of the desired worksheet.
- Enable auto-fitting for a row/column.
- Save the updated Excel file to the desired location.
In the following sections, you will learn how to implement these steps and perform auto-fitting of rows and columns in C#.
Auto-Fit Rows in Excel in C#
The following are the steps to auto-fit Excel rows in C#.
- First, load the Excel file using Workbook class.
- Then, get the reference of the worksheet using Workbook.Worksheets[index] collection.
- Call Worksheet.AutoFitRow(rowIndex) method to auto-fit a row using its index.
- Finally, save the updated Excel file using Workbook.Save(fileName) method.
The following code sample shows how to auto-fit an Excel row in C#.
Auto-Fit Columns in Excel in C#
The following are the steps to auto-fit a column in Excel using C#.
- First, load the Excel file using Workbook class.
- Then, get the reference of the worksheet using Workbook.Worksheets[index] collection.
- Call Worksheet.AutoFitColumn(columnIndex) method to auto-fit a column using its index.
- Finally, save the updated Excel file using Workbook.Save(fileName) method.
The following code sample shows how to auto-fit a column in Excel in C#.
C# API to Auto-Fit Excel Rows and Columns - Get a Free License
You can get a free temporary license to auto-fit rows and columns in your Excel files without evaluation limitations.
Conclusion
In this article, you have learned how to auto-fit rows and columns in Excel files in C#. The code samples have demonstrated how to enable auto-fitting for a specific row or column using its index programmatically.
Explore Aspose’ Spreadsheet API for C#
You can visit the documentation to explore other features of Aspose.Cells for .NET. Also, you can share your questions or queries with us via our forum.