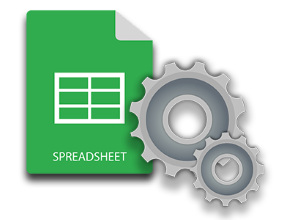
In this article, you will learn how to implement Excel automation features from within your Android applications. After reading this article, you will be able to create Excel XLSX or XLS file from scratch in your Android app programmatically. In addition, this article will cover how to update an existing Excel file, generate charts, apply formulas, and add pivot tables in Excel worksheets.
- Android API to Create Excel File
- Create Excel XLSX or XLS Files
- Edit Existing Excel Files
- Create Charts in Excel Files
- Create a Pivot Table in an XLSX
- Add Formulas for Cells in XLSX
Android API to Create Excel File - Free Download
Aspose.Cells for Android via Java is a powerful spreadsheet manipulation API that lets you create or modify Excel files without MS Office. The API supports adding charts, graphs, formulas, and perform other spreadsheet manipulation operations programmatically. You can either download the API or install it using the following configurations in build.gradle.
maven {
url "https://repository.aspose.com/repo/" }
compile (
group: 'com.aspose',
name: 'aspose-cells',
version: '21.3',
classifier: 'android.via.java')
Create Excel XLSX or XLS in Android
Each Excel workbook is composed of one or more worksheets that further contain the rows and columns to keep the data in the form of cells. The following are the steps to create an Excel XLSX file from scratch.
- Create an instance of Workbook class.
- Access the desired worksheet using Workbook.getWorksheets.get() method.
- Put the value in the desired cell in the worksheet using the cell’s identifier, such as A1, B3, etc.
- Save the workbook as an Excel file using the Workbook.save() method.
The following code sample shows how to create an Excel XLSX file in Android.
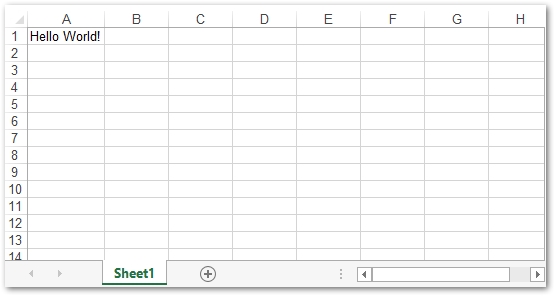
Edit an Excel XLSX File in Android
Lets now have a look at how to modify or insert data into an existing MS Excel file. For this, you can simply load the file, access the desired worksheet and save the updated file. The following are the steps to modify an existing Excel file.
- Open Excel file using Workbook class.
- Access the worksheets and cells using the Worksheet and Cell classes respectively.
- Save the updated workbook as an Excel .xlsx file.
The following code sample shows how to edit an existing MS Excel file in Android.
Create Charts or Graphs in Excel in Android
Charts in spreadsheets are used to visually represent the data stored in the worksheets. They make it easier to analyze a large amount of data quite easily. Aspose.Cells for Android via Java provides a wide range of charts that you can create within the Excel files programmatically. The following are the steps to create a chart in an Excel XLSX file.
- Create a new Excel file or load an existing one using Workbook class.
- Add data to the worksheet (optional).
- Get the chart collection of the worksheet using the Worksheet.getCharts() method.
- Add a new chart using Worksheet.getCharts().add() method.
- Get the newly created chart from the collection.
- Specify the cells’ range to set NSeries for the chart.
- Save the workbook as an Excel .xlsx file.
The following code sample shows how to create a chart in Excel XLSX in Android.
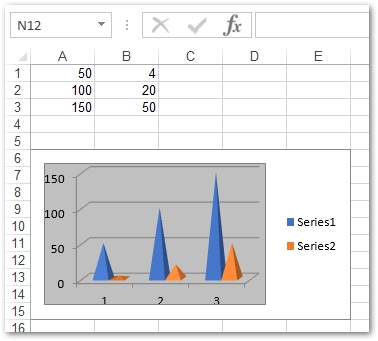
Create a Pivot Table in Excel XLSX in Android
Pivot tables in Excel worksheets have various purposes such as adding filters to the data, computing totals, summarizing data, and etc. Pivot tables can be created using the range of the cells in the worksheet. The following are the steps to create a pivot table in an Excel worksheet.
- Create a new Workbook or load an existing file.
- Insert data into the worksheet (optional).
- Access the pivot table collection using Worksheet.getPivotTables() method.
- Add a new pivot table in the worksheet using Worksheet.getPivotTables().add() method.
- Provide data to the pivot table.
- Save the workbook.
The following code sample shows how to create a pivot table in Excel.
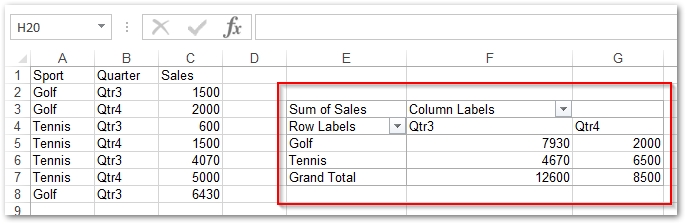
Add Formulas for Cells in Excel File
Aspose.Cells for Android via Java also allows you to work with formulas in the Excel worksheets. You can apply the built-in as well as add-in functions to the cells.
Apply Built-in Functions in Excel
For using the built-in functions, you can simply access the desired cell in the worksheet and add formula using the Cell.setFormula(String) method. The following code sample shows how to set a built-in formula.
Add Add-in Functions in Excel
There might be a case when you have to use a user-defined function. For this, you will have to register the add-in function using a .xlam (Excel macro-enabled add-in) file and then use it for the desired cells. For registering the add-in functions, Aspose.Cells for Android via Java provides registerAddInFunction(int, String) and registerAddInFunction(String, String, boolean) methods. The following code sample shows how to register and use an add-in function.
Conclusion
In this article, you have seen how to create MS Excel files from scratch in Android without MS Office. You have also learned how to update workbooks, create charts, add tables, and apply formulas to cell values within MS Excel worksheets. You can learn more about Android Excel API using documentation. In case you would have any queries, feel free to let us know via our forum.