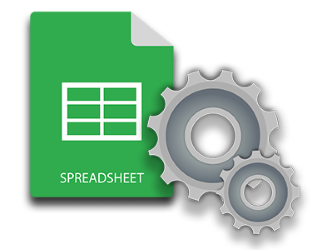
Microsoft Excel is a versatile tool used by millions of people worldwide for data analysis, reporting, and visualization. Excel files, commonly known as spreadsheets, are crucial for businesses and professionals. Creating Excel files programmatically has allowed the developers to automate data management operations using several programming languages. If you are a C# developer looking to create and manipulate Excel files programmatically, Aspose.Cells for .NET is a powerful library that can make your job easier. In this blog post, we’ll explore how to create Excel files in C# using Aspose.Cells for .NET.
- C# API to Create Excel Files - Free Download
- Create Excel XLS or XLSX files in C#
- Write data to the existing Excel file in C#
- Create charts or graphs in an Excel file in C#
- Create a table in an Excel file in C#
- Create Excel Files Online for Free
C# API to Create Excel Files
Aspose.Cells for .NET is a robust and feature-rich API that allows developers to work with Excel files without requiring Microsoft Excel to be installed on the system. This library provides a wide range of capabilities, including creating, modifying, and formatting Excel files, as well as performing complex data operations. Aspose.Cells for .NET is compatible with both .NET Framework and .NET Core, making it a versatile choice for C# developers.
You can either download or install the API using NuGet.
PM> Install-Package Aspose.Cells
Create an Excel XLS in C#
Now that we have the library set up, let’s create a simple Excel file. An Excel file is also known as a Workbook which is composed of single or multiple worksheets containing the rows and columns to hold the data. Thus, a workbook acts as the container of the worksheets in an Excel file. So to create an Excel file, you will first create a workbook and then the worksheets within that workbook.
To create a new Excel workbook in C#, follow these steps:
- Create an instance of Workbook class.
- Access the first worksheet (created by default) of the workbook.
- Access the desired cell(s) of the worksheet and put the value in the cell(s).
- Save the workbook as an XLS or XLSX file.
The following code sample shows how to create an Excel XLSX file using C#.
Output
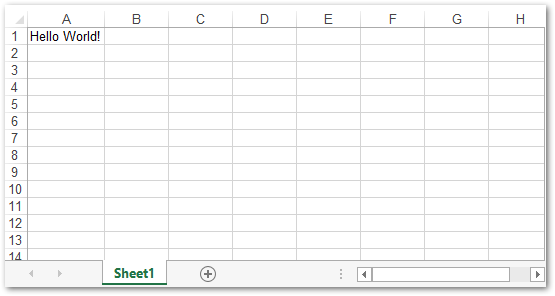
Write Data to an Excel File in C#
In case you want to edit and write data to an existing Excel file, you can also do it similarly. Simply load the source Excel spreadsheet document using the Workbook object and access the desired worksheets and cells. The following are the steps to edit an existing Excel file.
- Open Excel file in a FileStream object.
- Create an instance of Workbook and initialize it with the FileStream object.
- Access the worksheets and cells using the Worksheet and Cell classes respectively.
- Save the workbook as an Excel .xlsx file.
The following code sample shows how to edit and write data to an existing Excel XLSX file in C#.
Insert Charts or Graphs in Excel Sheet in C#
Excel spreadsheets provide a fine way of analyzing or presenting the data visually using graphs and charts. Aspose.Cells for .NET provides a complete set of classes to create and manipulate a variety of charts in Excel spreadsheets where each class is used to perform some specific tasks.
To create the charts in an Excel file, you’ll have to follow the following steps:
- Add some data (to be used as a data source) to the worksheet by accessing its cells.
- Add a new chart object to the worksheet using Worksheet.Charts collection by passing the type of chart using the ChartType enumeration.
- Get the newly created chart from the collection in a Chart object.
- Specify the cells’ range to provide the data source to the chart object.
- Save the workbook as an Excel .xlsx file.
The following code sample shows how to create an Excel XLSX file and add charts in C#.
Output

Learn more about creating charts in Excel worksheets using C#.
Create a Table in an Excel File in C#
You can also create a table from the range of cells in an Excel worksheet and add a row for the total (sum, count, etc.) in the table. The following are the steps to create an Excel (XLSX) file and add a table in C#:
- Load an Excel workbook or create a new one using Workbook class.
- Add data to the cells of the worksheet.
- Add a new ListObject to the worksheet.
- Set ListObject.ShowTotals property to true.
- Calculate the total and save the workbook as an Excel .xlsx file.
The following code sample shows how to create a table in an Excel file in C#.
Output

Learn more about working with tables in Excel worksheets using C#.
Create Excel Files Online
We also provide a free online Excel editor for you to create and edit Excel files. You can use this powerful spreadsheet editor without creating an account.
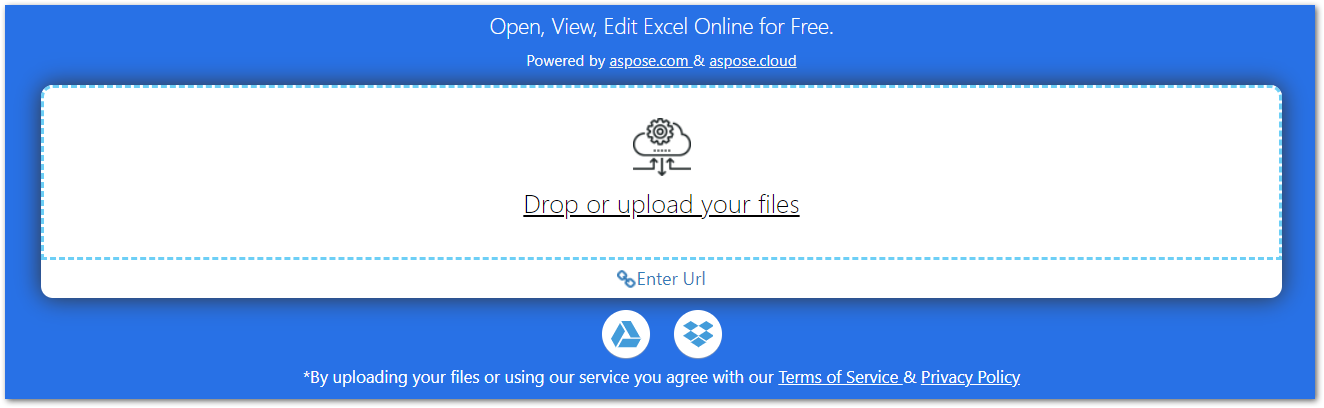
Get Free C# Excel API
You can use Aspose.Cells for .NET without evaluation limitations by getting a free temporary license.
Conclusion
Aspose.Cells for .NET is a versatile and powerful library for working with Excel files in C#. Whether you need to create simple spreadsheets or perform complex data operations, this library provides the tools and capabilities you need. With Aspose.Cells for .NET, you can automate Excel-related tasks and enhance your productivity as a C# developer.
In this article, you have learned how to create Excel files from scratch using C#. Furthermore, you have seen how to insert data in sheets, generate charts, and insert tables in Excel files. We have also provided you a free online Excel editor that you can use to create and edit Excel files within your browser.
You may have a look at the documentation of Aspose.Cells for .NET to learn the advanced features for the manipulation of Excel files in C#.