
Microsoft Excel is a powerful and widely-used spreadsheet application, and many businesses rely on it for data storage, analysis, and reporting. When working with Java applications, you might find the need to create, modify, or manipulate Excel files programmatically. Aspose.Cells for Java is a robust Java library that provides a comprehensive set of APIs for working with Excel files. In this blog post, we will explore how to use Aspose.Cells for Java to create Excel files in Java and populate them with data.
- Java Library to Create Excel Files - Free Download
- Create Excel XLSX or XLS Files using Java
- Edit Existing Excel Files using Java
- Create Charts in Excel Files using Java
- Create a Pivot Table in an XLSX using Java
- Add Formulas for Cells in XLSX using Java
- Create Excel Files Online for Free
Java Excel Library
Aspose.Cells for Java is a powerful spreadsheet manipulation library that lets you create or modify Excel files without MS Office. The library supports adding charts, graphs, formulas, and perform other spreadsheet manipulation operations programmatically. You can download the library for free or install it within your Maven-based applications.
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-cells</artifactId>
<version>23.4</version>
</dependency>
Create an Excel XLS in Java
Now that you’ve set up your development environment, let’s move on to creating Excel files. The MS Excel files are referred to as workbooks and each workbook is composed of one or more worksheets. The worksheets further contain the rows and columns to keep the data in the form of cells. So let’s start by creating a simple workbook. The following are the steps to create an Excel XLSX file from scratch.
- Create an instance of Workbook class.
- Access the desired worksheet using Workbook.getWorksheets.get() method.
- Put the value in the desired cell in the worksheet using the cell’s identifier, such as A1, B3, etc.
- Save the workbook as an Excel file using the Workbook.save() method.
The following code sample shows how to create an Excel XLSX file in Java.

Edit an Excel XLS in Java
Lets now have a look at how to modify or insert data into an existing MS Excel file. For this, you can simply load the file, access the desired worksheet and save the updated file. The following are the steps to modify an existing Excel file.
- Open Excel file using Workbook class.
- Access the worksheets and cells using the Worksheet and Cell classes respectively.
- Save the updated workbook as an Excel .xlsx file.
The following code sample shows how to edit an Excel file in Java.
Add Charts or Graphs to Excel Files
Charts in spreadsheets are used to visually represent the data stored in the worksheets. They make it easier to analyze a large amount of data quite easily. Aspose.Cells for Java provides a wide range of charts that you can create within the Excel files programmatically. The following are the steps to create an Excel file having chart in Java.
- Create a new Excel file or load an existing one using Workbook class.
- Add data to the worksheet (optional).
- Get the chart collection of the worksheet using the Worksheet.getCharts() method.
- Add a new chart using Worksheet.getCharts().add() method.
- Get the newly created chart from the collection.
- Specify the cells’ range to set NSeries for the chart.
- Save the workbook as an Excel .xlsx file.
The following code sample shows how to create Excel XLSX with a chart in Java.
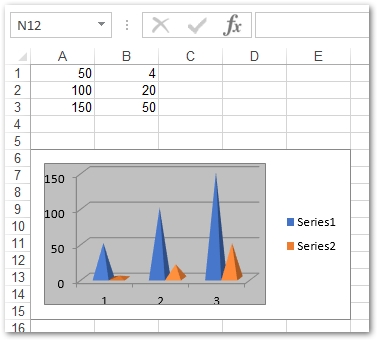
Create an Excel Pivot Table in Java
Pivot tables in Excel worksheets have various purposes such as adding filters to the data, computing totals, summarizing data, and etc. Pivot tables can be created using the range of the cells in the worksheet. The following are the steps to create a pivot table in an Excel XLS file in Java.
- Create a new Workbook or load an existing file.
- Insert data into the worksheet (optional).
- Access the pivot table collection using Worksheet.getPivotTables() method.
- Add a new pivot table in the worksheet using Worksheet.getPivotTables().add() method.
- Provide data to the pivot table.
- Save the workbook.
The following code sample shows how to create pivot table in Excel XLS in Java.
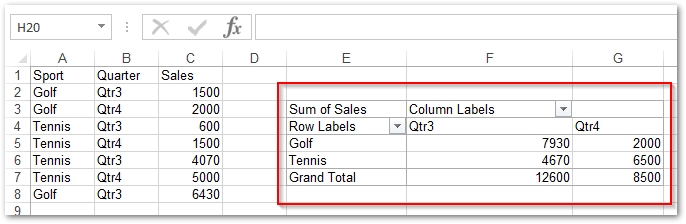
Add Formulas for Cells in Excel Sheets
Aspose.Cells for Java also allows you to work with formulas in the Excel worksheets. You can apply the built-in as well as add-in functions to the cells.
Apply Built-in Functions in Excel
For using the built-in functions, you can simply access the desired cell in the worksheet and add formula using the Cell.setFormula(String) method.
The following code sample shows how to set a built-in formula using Java.
Add Add-in Functions in Excel
There might be a case when you have to use a user-defined function. For this, you will have to register the add-in function using a .xlam (Excel macro-enabled add-in) file and then use it for the desired cells. For registering the add-in functions, Aspose.Cells for Java provides registerAddInFunction(int, String) and registerAddInFunction(String, String, boolean) methods.
The following code sample shows how to register and use an add-in function using Java.
Free Online Excel Editor
We also provide an online Excel editor for you to create and edit Excel files. You can use this powerful spreadsheet editor without creating an account.
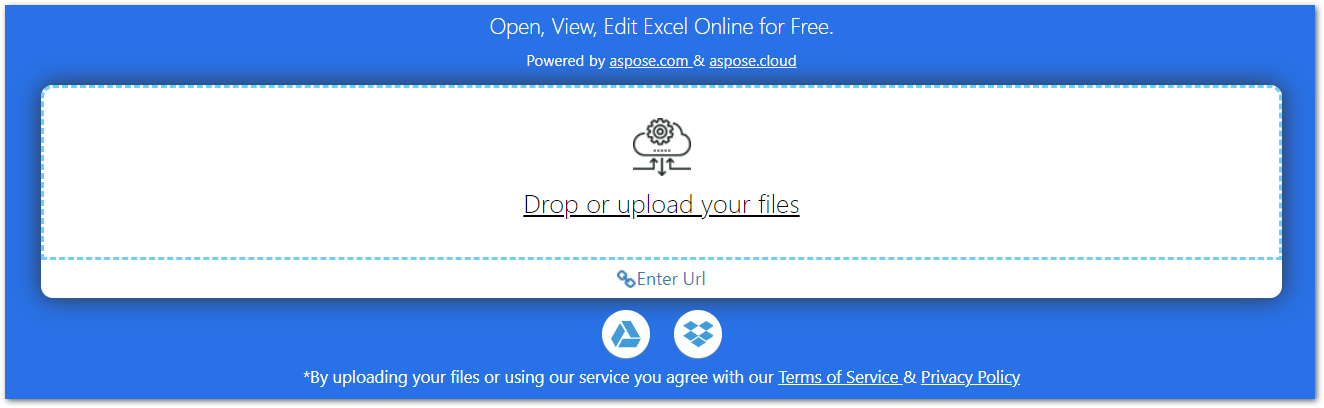
Get Free Java Excel Library
You can use the Java Excel library without evaluation limitations by getting a free temporary license.
Conclusion
Aspose.Cells for Java is a powerful and versatile library that simplifies the process of working with Excel files in Java applications. Whether you need to create basic spreadsheets or perform advanced data analysis and reporting, Aspose.Cells provides the tools and features to make your tasks easier. By following the steps outlined in this blog post, you can get started with creating Excel files in Java using Aspose.Cells for Java and unlock the full potential of Excel manipulation within your Java applications.
You can learn more about Aspose’s Java Excel library using documentation.