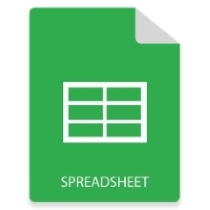
While generating and manipulating Excel files, you may need to insert and delete rows in the worksheets programmatically. Therefore, this article covers how to dynamically manipulate rows in Excel worksheets. Particularly, you will learn how to insert or delete rows in an Excel worksheet in Java.
- Java API to Insert or Delete Rows in Excel Files
- Insert Rows in an Excel File in Java
- Delete Rows from an Excel Worksheet in Java
Java API to Insert or Delete Excel Rows - Free Download
To insert or delete rows in Excel XLS/XLSX files, we’ll use Aspose.Cells for Java. It is a powerful and feature-rich spreadsheet manipulation API for Excel automation in Java applications. You can either download the API or install it using the following Maven configurations.
Repository:
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
Dependency:
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-cells</artifactId>
<version>22.8</version>
</dependency>
Insert Rows in an Excel File using Java
The following are the steps to insert rows in an Excel worksheet in Java.
- First, load the Excel file using the Workbook class.
- Then, access the desired worksheet by index using Workbook.getWorksheets().get(index) method.
- Use Worksheet.getCells().insertRows(rowIndex, totalRows) method to insert the rows in which the first parameter is the row index and the second parameter is the number of rows you want to insert.
- Finally, use Workbook.save(String) method to save the updated file.
The following code sample shows how to insert rows in an Excel file in Java.
Delete Rows in an Excel XLS in Java
The following are the steps to delete rows from an Excel worksheet in Java.
- First, load the Excel file using the Workbook class.
- Then, access the desired worksheet by index using Workbook.getWorksheets().get(index) method.
- Delete rows using Worksheet.getCells().deleteRows(rowIndex, totalRows) method in which the first parameter is the row index and the second parameter is the number of rows you want to delete.
- Finally, save the updated file using Workbook.save(String) method.
The following code sample shows how to delete rows from an Excel file in Java.
Java API to Insert/Delete Rows in Excel - Get a Free License
You can get a free temporary license to manipulate rows in Excel files without evaluation limitations.
Java Excel API - Read More
You can explore more about the Java Excel API using the documentation. Also, you may have a look at the API references to learn more about the API. Furthermore, you can share your queries with us via our forum.
Conclusion
In this article, you have learned how to work with rows in Excel files programmatically. Particularly, you have seen how to insert or delete rows in Excel worksheets using Java. You can use this feature when dynamically generating or manipulating Excel files.