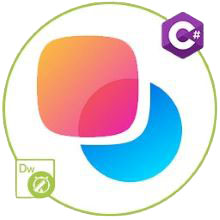
Computer graphics, especially in games, require a great deal of attention to detail. Every pixel counts, and there should be no jarring visual effects. This is where alpha blending comes into play. Almost all graphics engines today use some form of alpha blending in order to create more realistic visuals. For smooth transitions between both objects, the respective colors of the two objects are combined and partially overlapped. In this article, we will learn how to implement alpha blending in C#.
The following topics shall be covered in this article:
- What is Alpha Blending
- C# Alpha Blending API
- How to Implement Alpha Blending
- Composite Modes in Alpha Blending
What is Alpha Blending?
Alpha blending is a technique for combining two different colors, or transparency levels, by using the opacity of each color to produce a third. It allows for gradual transitions and blends the colors in your images. Alpha blending is often used for background and transparency effects.
In computer graphics, alpha blending is the process of combining two images so that they appear as one. The result is a single image where some areas appear transparent. The algorithm works by calculating the amount of transparency between two-pixel colors. It blends two images without touching any pixels.
C# Alpha Blending API - Free Download
For implementing alpha blending in C#, we will be using the Aspose.Drawing for .NET API. It is a cross-platform 2D graphics library for drawing text, geometries, and images programmatically. It allows loading, saving, and manipulating the supported file formats.
Please either download the DLL of the API or install it using NuGet.
PM> Install-Package Aspose.Drawing
Implement Alpha Blending using C#
The most common way to implement Alpha blending in C# is by using the Color.FromArgb() method. We can implement alpha blending programmatically by following the steps given below:
- Firstly, create an instance of the Bitmap class.
- Next, create the Graphics class object using the fromImage() method.
- Then, define a SolidBrush class object using the Color.FromArgb() method with the alpha channel parameter.
- After that, call the FillEllipse() method to draw a filled ellipse.
- Repeat the above steps to add more filled overlapping ellipses with different colors to generate new colors.
- Finally, save the output image using the Save() method.
The following code sample shows how to implement alpha blending in C#.
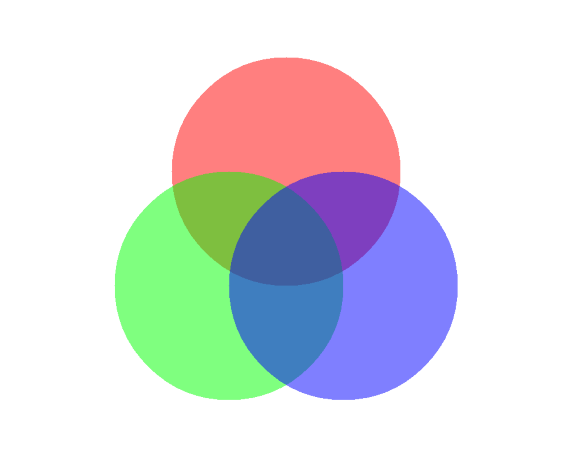
Implement Alpha Blending using C#
Composite Modes in Alpha Blending using C#
We can also use the composite modes to control the alpha blending by following the steps given below:
- Firstly, create an instance of the Bitmap class.
- Next, create the Graphics class object using the fromImage() method.
- Then, define a SolidBrush class object with the Color.FromArgb() method using the alpha channel parameter.
- Next, specify the CompositingMode and CompositingQuality for the Graphics object.
- After that, call the FillEllipse() method to draw a filled ellipse.
- Repeat the above steps to add more filled overlapping ellipses with different colors to generate new colors.
- Finally, save the output image using the Save() method.
The following code sample shows how to use composite mode to control alpha blending in C#.
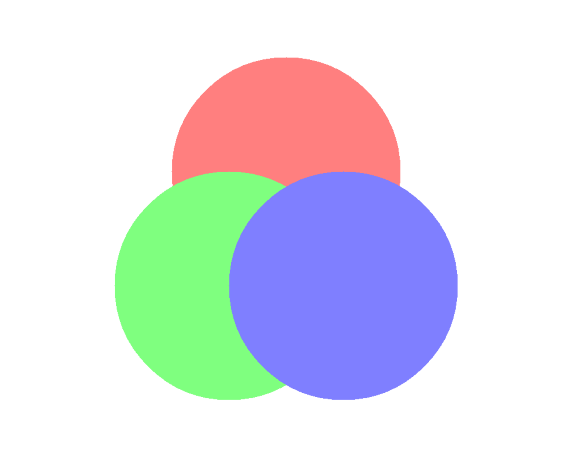
SourceCopy Composite Mode in Alpha Blending using C#
We can also use the SourceOver composite mode using the following code at the step # 4.
graphics.CompositingMode = CompositingMode.SourceOver;
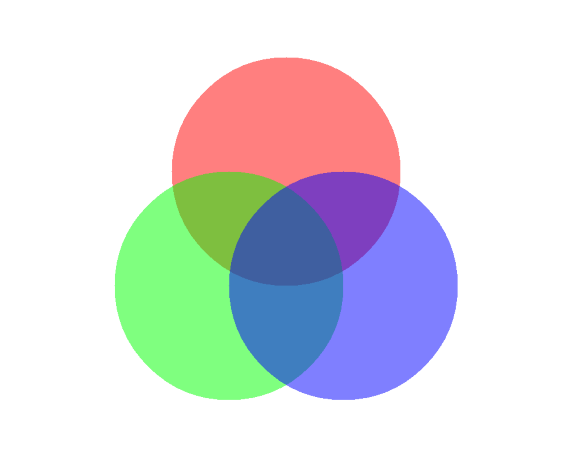
SourceOver Composite Mode in Alpha Blending using C#
Get Free Temporary License
You can get a free temporary license to try Aspose.Drawing for .NET without evaluation limitations.
Conclusion
In this article, we have learned how to implement alpha blending technique programmatically in C#. Besides, you can learn more about Aspose.Drawing for .NET using documentation and explore various features supported by the API. In case of any ambiguity, please feel free to contact us on our free support forum.