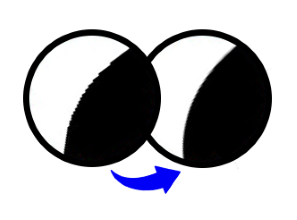
Creating realistic images is challenging because it requires the right combination of colors, textures, and line thicknesses. Aliasing is a problem that occurs when creating computer graphics-related images. To create realistic images, we need to perform antialiasing to smooth out jagged lines. Antialiasing is a technique that makes the graphics on your screen look smoother. It applies an algorithm to the edges of an object to make them more smooth and less jagged. There are different types of antialiasing techniques that can be applied, depending on the type of art you are working with. Aliasing occurs when there is a high contrast between adjacent pixels. This can cause jagged lines and stair-step curves where the lines meet or cross. This article is about antialiasing in Computer Graphics using C#. We will learn how to use Anti-aliasing with lines, curves, and text in C#.
The following topics will be covered in this article:
C# Computer Graphics Antialiasing API - Free Download
For implementing antialiasing in C#, we will be using the Aspose.Drawing for .NET API. This cross-platform 2D graphics library allows you to draw text, geometry, and images programmatically. In addition, you can load, save, and manipulate supported file formats.
Please either download the DLL of the API or install it using NuGet.
PM> Install-Package Aspose.Drawing
Antialiasing with Lines and Curves in C#
We can easily draw lines and curves with antialiasing in C# by following the steps given below:
- Firstly, create an instance of the Bitmap class.
- Next, create the Graphics class object using the Graphics.FromImage() method with the Bitmap object.
- Then, set the smoothing mode to AntiAlias.
- Meanwhile, initialize a Pen class object with the specified color and size.
- After that, draw the desired line, curve or other objects using the relevant methods.
- Finally, call the Save() method to save the output image at the specified image path.
The following code sample shows how to implement antialiasing with lines and curves in C#.
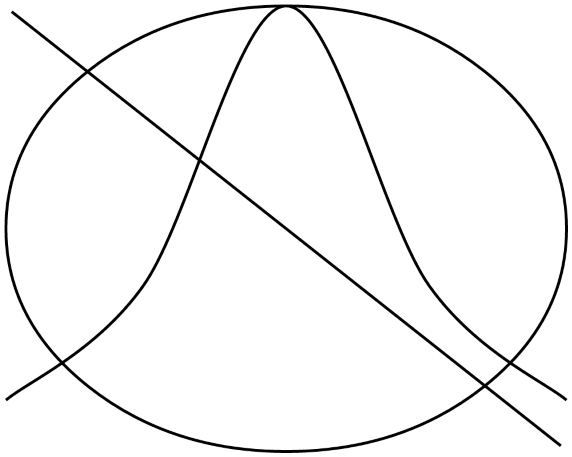
Antialiasing with Lines and Curves in C#
Antialiasing with Text in C#
Similarly, we can use antialiasing with text in C# by following the steps given below:
- Firstly, create an instance of the Bitmap class.
- Next, create the Graphics class object using the Graphics.FromImage() method with the Bitmap object.
- Then, initialize a Brush class object with the specified color.
- Meanwhile, set the TextRenderingHint to AntiAlias.
- After that, write the text using the DrawString() method.
- Finally, call the Save() method to save the output image at the specified image path.
The following code sample shows how to implement antialiasing with text in C#.
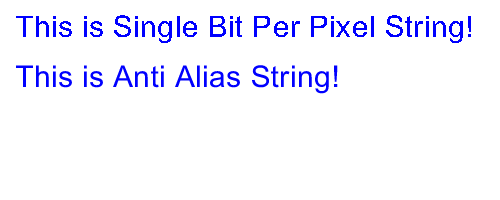
Antialiasing with Text in C#
Get Free Temporary License
You can get a free temporary license to try Aspose.Drawing for .NET without evaluation limitations.
Conclusion
In this article, we have learned how to implement antialiasing technique programmatically in C#. Besides, you can learn more about Aspose.Drawing for .NET using documentation and explore various features supported by the API. In case of any ambiguity, please feel free to contact us on our free support forum.