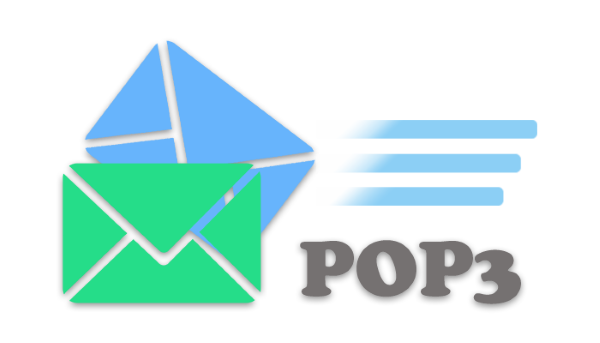
Post Office Protocol (POP3) is an email protocol that is used to fetch emails from the mailing servers. While implementing email clients and working with POP3 servers, you will first need to establish a connection to access the mailbox. To achieve this, in this article, you will learn how to connect to POP3 servers in Java.
- Java API to Connect POP3 Servers
- Connect to a POP3 Server using Java
- Connect to a POP3 Server via Proxy
Java API to Connect POP3 Servers
Aspose.Email for Java is an amazing API that allows you to create feature-rich email client applications. The API lets you create and send emails as well as retrieve messages from POP3 servers. We will use this API to connect to the POP3 servers and access the mailbox. You can either download the API or install it using the following Maven configurations.
Repository:
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>http://repository.aspose.com/repo/</url>
</repository>
Dependency:
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-email</artifactId>
<version>22.4</version>
<classifier>jdk16</classifier>
</dependency>
Connect to a POP3 Server in Java
In order to connect to a POP3 server, Aspose.Email for Java provides Pop3Client class. The following are the steps to connect to a POP3 server.
- Create an instance of the Pop3Client class.
- Specify the host, username, and password using the Pop3Client instance.
- Access the mailbox.
The following code sample shows how to connect to a POP3 server in Java.
Connect to POP3 Server via Proxy
In various cases, you have to access the email servers via a proxy. Currently, Aspose.Email for Java supports connecting to a POP3 server via SOCKS or HTTP proxy.
Connecting POP3 Server via SOCKS Proxy
The following are the steps to connect to a POP3 server via SOCKS proxy.
- Create a SocksProxy object and set the address, port, and SOCKS version.
- Create an instance of the Pop3Client class and set address, username, password, and other settings.
- Set proxy to SocksProxy object using Pop3Client.setProxy() method.
- Access mailbox.
The following code sample shows how to connect to the POP3 server via SOCKS proxy in Java.
Connecting POP3 Server via HTTP Proxy
The following are the steps to connect to a POP3 server via HTTP proxy.
- Create an HttpProxy object and set address, username, and password.
- Create an instance of the Pop3Client class and set address, username, password, and other settings.
- Set proxy to HttpProxy object using Pop3Client.setProxy() method.
- Access mailbox.
The following code sample shows how to connect to a POP3 server via HTTP proxy in Java.
Get a Free API License
You can try Aspose.Email for Java for free by getting a temporary license.
Conclusion
In this article, you have learned how to connect to the POP3 servers in Java. Furthermore, you have seen how to connect to a server via SOCKS or HTTP proxy. In addition, you can explore other features of Aspose.Email for Java using documentation. In case you would have any questions or queries, you can contact us via our forum.