In this blog, you will learn how to create Outlook email messages (including MSG, EML, EMLX, or MHTML) in C#. Also, we will demonstrate how to send emails synchronously or asynchronously using C# in .NET or .NET Core.
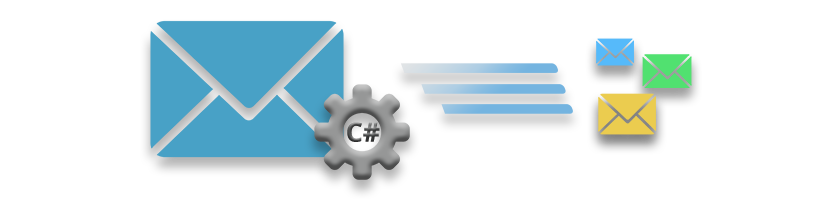
The email has become a primary source of exchanging messages and sharing content such as documents, images, or other types of files. The frequency of using emails is more within the online systems for sharing important notifications or documents with multiple entities. In such cases, preparing a template and sending emails to multiple stakeholders manually could be a time-consuming and hectic task.
Here comes the need for an Email Automation Service using which you can create and send emails seamlessly. So in this article, I am going to teach you how to create email messages of popular Outlook formats and automate the process of sending emails programmatically. After reading this article, you will be able to:
- create and save an email message in C#,
- create an email with HTML body in C#,
- create an email with a particular encoding in C#,
- send emails synchronously using SMTP in C#,
- send emails asynchronously using SMTP in C#,
- and send bulk emails in C#.
C# API to Create and Send Outlook Emails
In order to automate the process of creating and sending email messages, we will use Aspose.Email for .NET which is a powerful email manipulation and processing API. It supports popular email formats including MS Outlook messages such as MSG, EML/EMLX, etc. You can directly download the API’s DLL or install it via NuGet Package Manager or Package Manager Console in Visual Studio.
PM> Install-Package Aspose.Email
After you have created a C# (Console, ASP.NET, etc.) application and installed Aspose.Email for .NET, you can begin creating and sending the emails in C#.
Create an Outlook Email in C#
In order to create or manipulate Outlook email messages, Aspose.Email provides the MailMessage class. This class lets you set all the properties of an email message (Subject, To, From and Body), add attachments, and save the message in different email formats such as EML, MSG, MHTML, etc. The following are the steps to create an email message:
- Create an instance of MailMessage class.
- Set the message’s properties.
- Save the email message using MailMessage.Save() method.
The following code sample shows how to create and save an email message in C#.
Create an Outlook Email with HTML Body in C#
The HTML tags are used to make the body of the email more structured, presentable and enriched with different elements such as tables, images, lists, and so on. In case you want to create an email with having HTML body, you can use MailMessage.HtmlBody property. The following code sample shows how to create an Outlook email with HTML body in C#.
C# Create an Outlook Email with a Particular Encoding
You can also specify your desired encoding standard to tell the browser or email application about how to interpret the characters in the email’s body. The MailMessage.BodyEncoding property is used for this purpose. The following code sample shows how to set body encoding when creating an Outlook email using C#.
Send Outlook Emails Synchronously or Asynchronously in C#
Now, once you have created the email message, you can send it to its recipients synchronously or asynchronously. The SmtpClient class lets you send the Outlook email messages using SMTP (Simple Mail Transfer Protocol). The following are the steps to send an email in C# using Aspose.Email for .NET.
- Create or load an email message using the MailMessage class.
- Create an instance of SmtpClient class and set host, username, password and port number.
- Send email synchronously or asynchronously using SmtpClient.Send or SmtpClient.SendAsync method respectively.
Send an Outlook Email Synchronously in C#
Send an Outlook Email Asynchronously in C#
Send Bulk Emails in C#
You can also create and send emails in bulk. The MailMessageCollection class is used to contain the collection of email messages you want to send. The following code sample shows how to send bulk emails in C#.
Aspose Email API for C# - Live Demos
Conclusion
In this article, you have learned how to create Outlook emails in C#. Furthermore, you have seen how to send emails synchronously or asynchronously from within .NET applications. You can explore the documentation of Aspose.Email for .NET to learn more about the API.