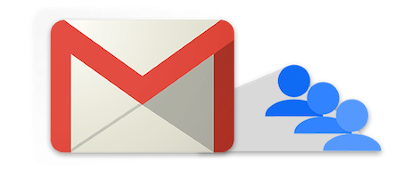
Google’s Gmail is among the most popular and commonly used email services. Gmail provides a range of features along with just sending and receiving emails, such as calendars, chats, etc. In certain cases, you may need to connect to Gmail and import contacts programmatically from within your applications. To achieve that, this article shows how to import Gmail contacts using Java. Also, we will cover how to access contacts in a specific email group.
Java API to Import Gmail Contacts
Aspose.Email for Java is a powerful API to create email client applications. Moreover, it supports working with Gmail such as accessing contacts, calendars, appointments, etc. We will use this API to access and import contacts from Gmail accounts. You can either download the API or install it using the following Maven configurations.
Repository:
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>http://repository.aspose.com/repo/</url>
</repository>
Dependency:
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-email</artifactId>
<version>22.2</version>
<classifier>jdk16</classifier>
</dependency>
Import Contacts from Gmail in Java
Before you start, you need to create a project on the Google Developer Console, which will allow you to execute the code. To create one, you can follow this guide.
Now, create a helper class named GoogleOAuthHelper to take care of the authentication of a Gmail account. Also, create a class named OAuthUser to store user information. The following is the complete implementation of both classes.
Import Contacts from a Gmail Account
The following are the steps to import contacts from a Gmail account in Java.
- Create an object of OAuthUser class and initialize it with email, client ID and client secret.
- Create two string objects to store authorization code and code verifier.
- Get refresh token and access token.
- Get an instance of GmailClient class into an IGmailClient object using GmailClient.getInstance(String, String) method.
- Read contacts into an array using IGmailClient.getAllContacts() method.
- Loop through array to access each contact.
The following code sample shows how to import Gmail contacts from an account in Java.
Import Gmail Contacts from a Group
You can also access contacts from a specific email group in Gmail following the steps below.
- Follow the steps mentioned in previous section to initialize the IGmailClient.
- Call IGmailClient.getAllGroups() to get groups into a ContactGroupCollection object.
- Filter the required group(s) based on the title.
- Access contacts from a group using IGmailClient.getContactsFromGroup(String) method.
- Loop through array to access each contact.
The following code sample shows how to import contacts from a specific Gmail group in Java.
Get a Free API License
You can get a free temporary license to use Aspose.Email for Java without evaluation limitations.
Conclusion
In this article, you have learned how to import Gmail contacts from an account programmatically in Java. Moreover, you have seen how to access contacts from a particular email group in Gmail. Apart from that, you can explore the documentation to read more about Aspose.Email for Java. Also, you can ask your questions via our forum.