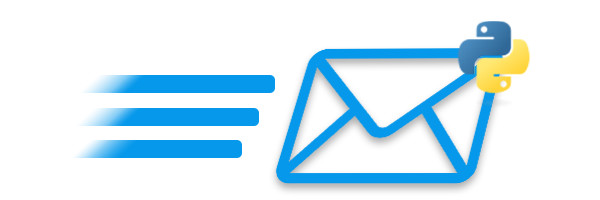
Automated emails are widely used to send notifications or other messages to subscribers. Also, various emails are triggered based on the actions or behavior of the users. In order to implement email automation, this article covers how to send emails via SMTP in Python. In addition, we will demonstrate how to create an email message with plain text or HTML body from scratch.
- Python Library to Send Emails - Free Download
- Create and Send Emails in Python
- Send Emails with HTML Body in Python
- Send Bulk Emails
- Forward an Email Message
Python Library to Send Emails via SMTP
In order to create and send emails using SMTP, we will use Aspose.Email for Python via .NET. It is a powerful Python library that lets you implement feature-rich email clients. You can install the API from PyPI using the following command.
pip install Aspose.Email-for-Python-via-NET
Or, you can follow the below steps for manual installation.
- Download the installable .whl file from the downloads section.
- From the command line, use command: pip install «FileName».whl to install the API.
- Download a complete package of source code samples from GitHub.
Send Emails via SMTP in Python
The following are the steps to create and send an email via SMTP in Python.
- Create an object of MailMessage class.
- Set subject, body, sender’s, and recipient’s addresses.
- Set Cc or Bcc (optional).
- Create a new SMTP client using SmtpClient class and set host, port, username, and password.
- Set security options.
- Send email using SmtpClient.send(MailMessage) method.
The following code sample shows how to create and send emails in Python.
Send Emails with HTML Body in Python
The following are the steps to send emails with HTML body in Python.
- Create an object of MailMessage class.
- Set body of the email using MailMessage.body_html property.
- Set MailMessage.is_body_html property to true.
- Assign subject, sender’s, and recipient’s addresses.
- Set Cc or Bcc (optional).
- Create a new SMTP client using SmtpClient class and set host, port, username, and password.
- Send email using SmtpClient.send(MailMessage) method.
The following code sample shows how to send emails with HTML body in Python.
Python Code to Send Bulk Emails
The following are the steps to send bulk emails in Python.
- Create multiple email messages using MailMessage class and set their properties, such as subject, recipients, etc.
- Create an object of MailMessageCollection class.
- Add email messages to the collection using MailMessageCollection.append(MailMessage) method.
- Create a new SMTP client using SmtpClient class and set host, port, username, and password.
- Send email using SmtpClient.send(MailMessageCollection) method.
The following code sample shows how to send bulk emails via SMTP in Python.
How to Forward an Email Message in Python
The following are the steps to forward an email message in Python.
- Load the email message into an object using MailMessage.load(String fileName) method.
- Set recipient’s address.
- Create an SMTP client using SmtpClient class.
- Forward message using SmtpClient.forward() method.
The following code sample shows how to forward an email message in Python.
Python Library for Sending Emails - Get a Free License
You may get a Free Temporary License and send emails via SMTP without evaluation limitations.
Aspose Email Library for Python - Live Demo
Conclusion
In this article, you have learned how to create and send emails via SMTP in Python. Furthermore, you have seen how to send bulk emails with plain text or HTML body programmatically. You can explore more about the Python email API using the documentation. In case you would have any questions or queries, feel free to let us know via our forum.