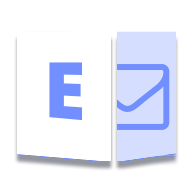
Logging is used for debugging, as well as for collecting and analyzing working information about the the application. This information is written to a file called a log. Log files contain system information about the operation of the client application, for example, user or program actions.
In this article, we will cover how to set up EWS client activity logging using C# .NET.
- C# .NET API to Work with MS Exchange Web Services
- Enable Activity Logging using App.config File
- Enable Activity Logging using appsettings.json File
- Enable Activity Logging in Programm Code
- An example of a log file info
C# .NET API to Work with MS Exchange Web Services
To manage MS Exchange Web Services, we will use Aspose.Email for .NET.
It is a powerful API that allows an access to various services of MS Exchange Server seamlessly. Also, it provides a lot of features to implement email client applications.
You can either download the API’s DLL or install it from NuGet using the following command.
PM> Install-Package Aspose.Email
Enable Activity Logging using App.config File
This option is suitable for applications where app.config
is the preferred way for keeping the app configuration.
The following are the steps to enable logging in EWSClient in C#.
- Firstly, add an application configuration file to a C# project, if it has not been added before.
- Then, add the following content to the configuration file.
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<configSections>
<sectionGroup name="applicationSettings" type="System.Configuration.ApplicationSettingsGroup, System, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" >
<section name="Aspose.Email.Properties.Settings" type="System.Configuration.ClientSettingsSection, System, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" requirePermission="false" />
</sectionGroup>
</configSections>
<applicationSettings>
<Aspose.Email.Properties.Settings>
<setting name="EWSDiagnosticLog" serializeAs="String">
<value>..\..\..\Log\Aspose.Email.EWS.log</value>
</setting>
<setting name="EWSDiagnosticLog_UseDate" serializeAs="String">
<value>False</value>
</setting>
</Aspose.Email.Properties.Settings>
</applicationSettings>
</configuration>
We can see two setting sections:
EWSDiagnosticLog
- Specifies the relative or absolute path to the log file.EWSDiagnosticLog_UseDate
- specifies whether to add a string representation of the current date to the log file name.
Enable Activity Logging using appsettings.json File
This option is preferred for .NET Core applications.
The following are the steps to enable logging in EWSClient in C#.
- Firstly, add an
appsettings.json
configuration file to a C# project, if it has not been added before. Make sure that the project file contains the following lines in the ItemGroup section:
<Content Include="appsettings.json">
<CopyToOutputDirectory>Always</CopyToOutputDirectory>
</Content>
- Then, add the following content to the appsettings.json file.
{
"EWSDiagnosticLog": "ews.log",
"EWSDiagnosticLog_UseDate": true
}
We can see two properties:
EWSDiagnosticLog
- Specifies the relative or absolute path to the log file.EWSDiagnosticLog_UseDate
- specifies whether to add a string representation of the current date to the log file name.
Enable Activity Logging in Programm Code
You can also enable logging immediately in the code. Note: even if you have already enabled logging by using configuration files, this option will be applied.
The following are the steps to enable logging in EWSClient in C#.
- First, create an EWSClient.
- Second, set the path to the log file using the LogFileName property.
- Finally, set the UseDateInLogFileName property if it is necessary.
using (var client = EWSClient.GetEWSClient("https://outlook.office365.com/EWS/Exchange.asmx", credentials))
{
client.LogFileName = @"Aspose.Email.EWS.log";
client.UseDateInLogFileName = false;
}
An example of a log file info
Below is an example of log file entries when the ListMessages method is executed. Each new entry is preceded by a timestamp.
The following entries can be distinguished in the log file:
- Log file header. Includes Aspose.Email version, name and start time.
Aspose.Email for .NET [22.10.0.0] EWS Client diagnostic log
Started: 07.11.2022 13:40:16
- SOAP request to the server.
07.11.2022 13:40:16 <soap:Envelope xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/">
<soap:Header>
<ExchangeImpersonation xmlns="http://schemas.microsoft.com/exchange/services/2006/types">
<ConnectingSID>
<SmtpAddress>someaddress@someorg.onmicrosoft.com</SmtpAddress>
</ConnectingSID>
</ExchangeImpersonation>
<RequestServerVersion xmlns="http://schemas.microsoft.com/exchange/services/2006/types" Version="Exchange2013" />
</soap:Header>
<soap:Body>
<FindItem xmlns="http://schemas.microsoft.com/exchange/services/2006/messages" Traversal="Shallow">
<ItemShape>
<BaseShape xmlns="http://schemas.microsoft.com/exchange/services/2006/types">IdOnly</BaseShape>
</ItemShape>
<IndexedPageItemView MaxEntriesReturned="2147483647" Offset="0" BasePoint="Beginning" />
<ParentFolderIds>
<DistinguishedFolderId xmlns="http://schemas.microsoft.com/exchange/services/2006/types" Id="inbox" />
</ParentFolderIds>
</FindItem>
</soap:Body>
</soap:Envelope>
- HTTP response headers
07.11.2022 13:40:18 Cache-Control: private
Transfer-Encoding: chunked
Server: Microsoft-IIS/10.0
request-id: 5c777b61-e3d9-c262-fbb0-6f071d9ff68a
Alt-Svc: h3=":443", h3-29=":443"
X-CalculatedFETarget: CH0PR03CU015.internal.outlook.com, BL0PR02CU002.internal.outlook.com
X-BackEndHttpStatus: 200, 200, 200
Set-Cookie: exchangecookie=bf07039c0ee942438170c2958ca2d330; expires=Tue, 07-Nov-2023 10:40:17 GMT; path=/; secure; HttpOnly
X-CalculatedBETarget: BLAPR10MB4915.namprd10.PROD.OUTLOOK.COM
X-RUM-Validated: 1
x-ms-appId: 3fe84e63-c57b-48eb-ab41-879415751cfd
Restrict-Access-Confirm: 1
x-EwsHandler: FindItem
X-AspNet-Version: 4.0.30319
X-BeSku: WCS6
X-DiagInfo: BLAPR10MB4915
X-BEServer: BLAPR10MB4915
X-Proxy-RoutingCorrectness: 1
X-Proxy-BackendServerStatus: 200
X-FEProxyInfo: AS9PR06CA0693.EURPRD06.PROD.OUTLOOK.COM
X-FEEFZInfo: DHR
X-FEServer: BL0PR02CA0063, CH0PR03CA0449, AS9PR06CA0693
X-FirstHopCafeEFZ: DHR
X-Powered-By: ASP.NET
Date: Mon, 07 Nov 2022 10:40:17 GMT
Content-Type: text/xml; charset=utf-8
- SOAP server response.
07.11.2022 13:40:18 <?xml version="1.0" encoding="utf-8"?>
<soap:Envelope xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/">
<soap:Header>
<ExchangeImpersonation xmlns="http://schemas.microsoft.com/exchange/services/2006/types">
<ConnectingSID>
<SmtpAddress>someaddress@someorg.onmicrosoft.com</SmtpAddress>
</ConnectingSID>
</ExchangeImpersonation>
<RequestServerVersion Version="Exchange2013" xmlns="http://schemas.microsoft.com/exchange/services/2006/types" />
</soap:Header>
<soap:Body>
<GetItem xmlns="http://schemas.microsoft.com/exchange/services/2006/messages">
<ItemShape>
<BaseShape xmlns="http://schemas.microsoft.com/exchange/services/2006/types">IdOnly</BaseShape>
<AdditionalProperties xmlns="http://schemas.microsoft.com/exchange/services/2006/types">
<FieldURI FieldURI="item:Attachments" /><FieldURI FieldURI="item:DateTimeCreated" />
<FieldURI FieldURI="item:DateTimeReceived" /><FieldURI FieldURI="item:DateTimeSent" />
<FieldURI FieldURI="item:IsDraft" /><FieldURI FieldURI="item:IsFromMe" />
<FieldURI FieldURI="item:HasAttachments" /><FieldURI FieldURI="item:IsUnmodified" />
<FieldURI FieldURI="item:ItemClass" /><FieldURI FieldURI="item:IsSubmitted" />
<FieldURI FieldURI="item:IsResend" /><FieldURI FieldURI="item:DisplayCc" /><FieldURI FieldURI="item:DisplayTo" />
<FieldURI FieldURI="item:Attachments" /><FieldURI FieldURI="item:LastModifiedTime" />
<FieldURI FieldURI="item:Size" /><FieldURI FieldURI="item:Subject" />
<FieldURI FieldURI="item:InternetMessageHeaders" /><FieldURI FieldURI="message:IsRead" />
<FieldURI FieldURI="message:InternetMessageId" /><FieldURI FieldURI="message:Sender" />
<FieldURI FieldURI="message:CcRecipients" /><FieldURI FieldURI="message:ToRecipients" />
<FieldURI FieldURI="message:BccRecipients" /><FieldURI FieldURI="message:From" />
</AdditionalProperties>
</ItemShape>
<ItemIds>
<ItemId
Id="AAMkAGJhZjYzY2I5LTdjYWMtNGFmMC05ODI1LTA5MTAzYTgwZTc4OQBGAAAAAABdN1MC60QcSpWwPYUTPhL2BwATlR+p0q0wT6WD0+d4WJhWAAAAAAEMAAATlR+p0q0wT6WD0+d4WJhWAACp2kv8AAA="
xmlns="http://schemas.microsoft.com/exchange/services/2006/types" />
</ItemIds>
</GetItem>
</soap:Body>
</soap:Envelope>
Get a Free API License
You can get a free temporary license to use Aspose.Email for .NET without evaluation limitations.
Conclusion
In this article you have learned how to set up activity logging in EWS client using C#. This feature allows you to have better monitoring of your client application. In addition, you can explore the documentation to read more about Aspose.Email for .NET. Also, you can ask your questions via our forum.