
Con la creciente importancia de las aplicaciones basadas en datos, la necesidad de exportar datos desde archivos XML al formato universalmente aceptable PDF ha adquirido una importancia significativa. Esta publicación de blog proporciona una guía paso a paso para convertir XML a PDF de manera eficiente en Python. ¡Vamos a empezar!
Los siguientes temas se cubrirán en este artículo:
- Biblioteca Python para convertir XML a PDF
- Convertir XML a PDF en Python
- Generar PDF desde XML en Python
- Convertir XML a PDF en línea
- Recursos de aprendizaje gratuitos
Biblioteca Python para convertir XML a PDF
Para generar archivos PDF a partir de XML, el enfoque más sencillo es aprovechar la biblioteca Aspose.PDF for Python diseñada para la conversión de XML a PDF. Con una instalación y uso sencillos, proporciona una solución eficaz para transformar documentos XML en archivos PDF. Aspose.PDF for Python es una solución sólida para la generación, manipulación y conversión de PDF, que ofrece a los desarrolladores un control incomparable sobre los flujos de trabajo de documentos.
Por favor descargue el paquete o instale la API desde PyPI usando el siguiente comando pip en la consola:
> pip install aspose-pdf
Convertir XML a PDF en Python
Podemos transformar fácilmente XML a PDF siguiendo los pasos a continuación:
- Crea un objeto de la clase Document.
- Vincule XML utilizando el método Document.bindxml(file) proporcionando la ruta del archivo XML.
- Convierta XML a PDF utilizando el método Document.save(outputfilename).
El siguiente ejemplo de código muestra cómo convertir un archivo XML a PDF usando Python.
import aspose.pdf as ap
# Crear un nuevo documento PDF
pdfDocument = ap.Document();
# Transformar y vincular XML
pdfDocument.bind_xml( "C:\\Files\\sample.xml");
# Generar PDF desde XML
pdfDocument.save( "C:\\Files\\generated-pdf.pdf");
Archivo XML de origen
El siguiente es el archivo XML de muestra que utilizamos para convertir al documento PDF.
<?xml version="1.0" encoding="utf-8" ?>
<Document xmlns="Aspose.Pdf">
<Page>
<TextFragment>
<TextSegment>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nulla odio lorem, luctus in lorem vitae, accumsan semper lectus. Cras a auctor leo, et tincidunt lacus.</TextSegment>
</TextFragment>
</Page>
</Document>
Documento PDF generado

Convertir XML a PDF en Python
Generar PDF desde XML en Python
También podemos generar un documento PDF a partir del archivo XML que contiene los datos de la aplicación. Para ello, primero lo transformaremos al XML compatible con Aspose.PDF usando XSLT y luego lo convertiremos al formato PDF.
Los siguientes son los datos XML de muestra que necesitamos para convertir al documento PDF.
<?xml version="1.0" encoding="utf-8" ?>
<catalog>
<cd>
<Content>Hello World!</Content>
<title>Empire Burlesque</title>
<artist>Bob Dylan</artist>
<country>USA</country>
<company>Columbia</company>
<price>10.90</price>
<year>1985</year>
</cd>
<cd>
<title>Hide your heart</title>
<artist>Bonnie Tyler</artist>
<country>UK</country>
<company>CBS Records</company>
<price>9.90</price>
<year>1988</year>
</cd>
<cd>
<title>Greatest Hits</title>
<artist>Dolly Parton</artist>
<country>USA</country>
<company>RCA</company>
<price>9.90</price>
<year>1982</year>
</cd>
<cd>
<title>Still got the blues</title>
<artist>Gary Moore</artist>
<country>UK</country>
<company>Virgin records</company>
<price>10.20</price>
<year>1990</year>
</cd>
<cd>
<title>Eros</title>
<artist>Eros Ramazzotti</artist>
<country>EU</country>
<company>BMG</company>
<price>9.90</price>
<year>1997</year>
</cd>
</catalog>
Para que estos datos sean compatibles con Aspose.PDF XML, realizaremos una transformación XSLT. Para ello, definiremos una plantilla en un archivo de hoja de estilo XSLT, como se muestra a continuación.
<?xml version="1.0" encoding="utf-8"?>
<xsl:stylesheet version="1.0"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="/">
<Document xmlns="Aspose.Pdf">
<Page>
<PageInfo IsLandscape="false" Height="595" Width="420">
<Margin Top="71" Bottom="71" Left="28" Right="28" />
</PageInfo>
<Header>
<Margin Top="20" />
<Table ColumnAdjustment="AutoFitToWindow">
<Row>
<Cell Alignment="1">
<TextFragment>
<TextSegment>Date: 11/01/2024</TextSegment>
</TextFragment>
</Cell>
<Cell Alignment="3">
<TextFragment>
<TextSegment>Page $p / $P</TextSegment>
</TextFragment>
</Cell>
</Row>
</Table>
</Header>
<HtmlFragment>
<![CDATA[
<h1 style="font-family:Tahoma; font-size:16pt;">My CD Collection</h1>
]]>
</HtmlFragment>
<TextFragment>
<TextSegment>Welcome</TextSegment>
</TextFragment>
<Table ColumnAdjustment="AutoFitToWindow" ColumnWidths ="10 10 10 10">
<DefaultCellPadding Top="5" Left="0" Right="0" Bottom="5" />
<Border>
<Top Color="Black"></Top>
<Bottom Color="Black"></Bottom>
<Left Color="Black"></Left>
<Right Color="Black"></Right>
</Border>
<Margin Top="15" />
<Row BackgroundColor="LightGray" MinRowHeight="20">
<Border>
<Bottom Color="Black"></Bottom>
</Border>
<Cell Alignment="2">
<TextFragment>
<TextSegment>Title</TextSegment>
</TextFragment>
</Cell>
<Cell>
<TextFragment>
<TextSegment>Artist</TextSegment>
</TextFragment>
</Cell>
<Cell>
<TextFragment>
<TextSegment>Price</TextSegment>
</TextFragment>
</Cell>
<Cell>
<TextFragment>
<TextSegment>Year</TextSegment>
</TextFragment>
</Cell>
</Row>
<xsl:for-each select="catalog/cd">
<Row>
<Cell Alignment="2">
<TextFragment>
<TextSegment><xsl:value-of select="title"/></TextSegment>
</TextFragment>
</Cell>
<Cell>
<TextFragment>
<TextSegment><xsl:value-of select="artist"/></TextSegment>
</TextFragment>
</Cell>
<Cell>
<TextFragment>
<TextSegment><xsl:value-of select="price"/></TextSegment>
</TextFragment>
</Cell>
<Cell>
<TextFragment>
<TextSegment><xsl:value-of select="year"/></TextSegment>
</TextFragment>
</Cell>
</Row>
</xsl:for-each>
</Table>
</Page>
</Document>
</xsl:template>
</xsl:stylesheet>
Una vez que hayamos creado el archivo de plantilla, podemos generar un PDF siguiendo los pasos mencionados anteriormente. Sin embargo, solo necesitamos llamar al método Document.bindxml(xmlfile, xslfile) proporcionando rutas de archivos XML y XSLT.
El siguiente ejemplo de código muestra cómo generar un PDF a partir de un archivo XML usando Python.
import aspose.pdf as ap
# Crear un nuevo documento PDF
pdfDocument = ap.Document();
# Transformar y vincular XML
pdfDocument.bind_xml( "C:\\Files\\data.xml", "C:\\Files\\template.xslt");
# Generar PDF desde XML
pdfDocument.save( "C:\\Files\\generated-pdf-table.pdf");

Generar PDF desde XML en Python
Licencia de conversión de XML a PDF
Puede obtener una licencia temporal para utilizar la API sin limitaciones de evaluación.
Convertir XML a PDF en línea
También puede convertir archivos XML en documentos PDF en línea utilizando esta herramienta gratuita convertidor de XML a PDF.
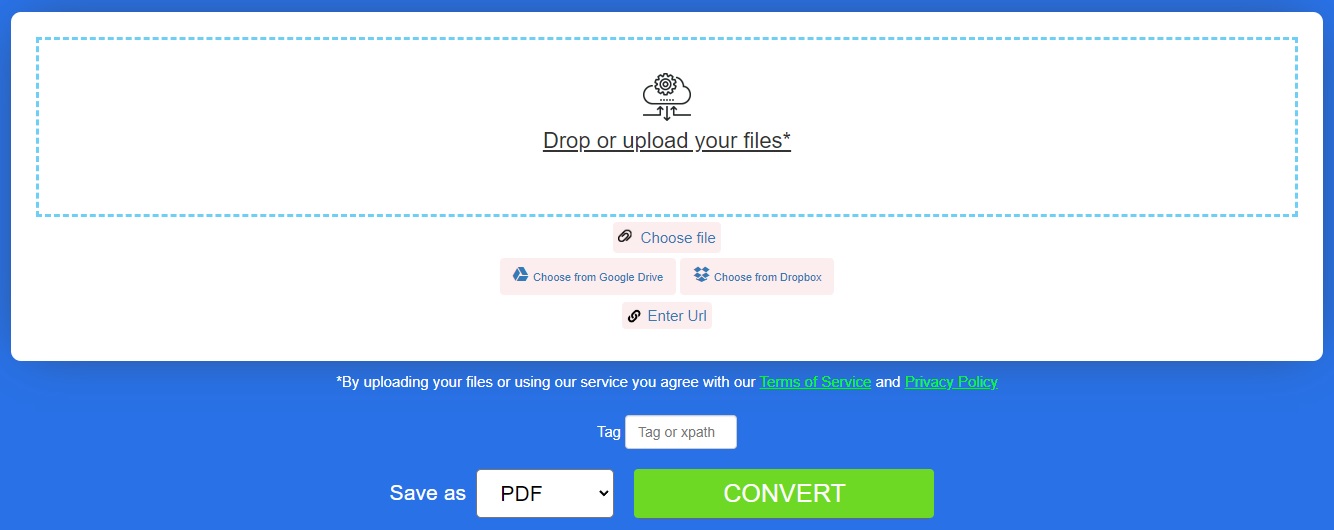
Archivo XML a PDF – Recursos de aprendizaje
Además de crear archivos XML en documentos PDF, obtenga más información sobre cómo crear, manipular y convertir documentos PDF y explore otras funciones de la biblioteca utilizando los recursos siguientes:
Conclusión
En este artículo, has aprendido cómo convertir XML a PDF en Python. Si sigue los pasos descritos en este artículo, puede integrar fácilmente esta función en sus aplicaciones Python para generar archivos PDF a partir de XML. En caso de que tenga alguna pregunta, no dude en hacérnosla saber a través de nuestro foro de soporte gratuito.