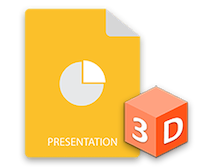
Los efectos 3D en PowerPoint se utilizan para hacer que las presentaciones sean más atractivas y captar la atención de los usuarios. Por lo tanto, es posible que se encuentre con el requisito de agregar objetos 3D a las presentaciones mediante programación. En este artículo, aprenderá cómo crear efectos 3D en PowerPoint PPT o PPTX en Java. Le mostraremos cómo crear texto y formas en 3D y aplicar efectos 3D a las imágenes.
- API de Java para crear efectos 3D en PowerPoint
- Crear un texto 3D en PowerPoint en Java
- Crear una forma 3D en PowerPoint en Java
- Establecer degradado para formas 3D
- Aplicar efectos 3D a una imagen en PowerPoint
API de Java para aplicar efectos 3D en PowerPoint PPT
Aspose.Slides for Java es una potente API que encapsula una amplia gama de funciones de manipulación de presentaciones. Con la API, puede crear presentaciones interactivas y manipular archivos PPT/PPTX existentes sin problemas. Para crear efectos 3D en las presentaciones de PowerPoint, utilizaremos esta API.
Puede descargar el JAR de la API o instalarlo usando las siguientes configuraciones de Maven.
Repositorio:
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
Dependencia:
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-slides</artifactId>
<version>22.1</version>
<classifier>jdk16</classifier>
</dependency>
Crear un texto 3D en PowerPoint en Java
Los siguientes son los pasos para crear un fragmento de texto 3D en PowerPoint PPT usando Java.
- Primero, cree un nuevo PPT o cargue uno existente usando la clase Presentation.
- Luego, agregue una nueva forma de rectángulo usando el método addAutoShape().
- Establezca las propiedades de la forma, como el tipo de relleno, el texto, etc.
- Obtenga la referencia del texto dentro de la forma en un objeto Porción.
- Aplicar formato a la porción de texto.
- Obtenga la referencia de la forma interior TextFrame.
- Aplique efectos 3D usando IThreeDFormat devuelto por el método TextFrame.getTextFrameFormat().getThreeDFormat().
- Finalmente, guarde la presentación usando el método Presentation.save(String, SaveFormat).
El siguiente ejemplo de código muestra cómo crear texto 3D en PowerPoint PPT en Java.
// Crear presentación
Presentation pres = new Presentation();
try {
// Añadir forma de rectángulo
IAutoShape shape = pres.getSlides().get_Item(0).getShapes().addAutoShape(ShapeType.Rectangle, 200, 150, 200, 200);
// Establecer texto
shape.getFillFormat().setFillType(FillType.NoFill);
shape.getLineFormat().getFillFormat().setFillType(FillType.NoFill);
shape.getTextFrame().setText("3D Text");
// Agregar porción de texto y establecer sus propiedades
Portion portion = (Portion)shape.getTextFrame().getParagraphs().get_Item(0).getPortions().get_Item(0);
portion.getPortionFormat().getFillFormat().setFillType(FillType.Pattern);
portion.getPortionFormat().getFillFormat().getPatternFormat().getForeColor().setColor(new Color(255, 140, 0));
portion.getPortionFormat().getFillFormat().getPatternFormat().getBackColor().setColor(Color.WHITE);
portion.getPortionFormat().getFillFormat().getPatternFormat().setPatternStyle(PatternStyle.LargeGrid);
// Establecer el tamaño de fuente del texto de la forma
shape.getTextFrame().getParagraphs().get_Item(0).getParagraphFormat().getDefaultPortionFormat().setFontHeight(128);
// Obtener marco de texto
ITextFrame textFrame = shape.getTextFrame();
// Configurar el efecto de transformación de WordArt "Arco hacia arriba"
textFrame.getTextFrameFormat().setTransform(TextShapeType.ArchUp);
// Aplicar efectos 3D
textFrame.getTextFrameFormat().getThreeDFormat().setExtrusionHeight(3.5f);
textFrame.getTextFrameFormat().getThreeDFormat().setDepth(3);
textFrame.getTextFrameFormat().getThreeDFormat().setMaterial(MaterialPresetType.Plastic);
textFrame.getTextFrameFormat().getThreeDFormat().getLightRig().setDirection(LightingDirection.Top);
textFrame.getTextFrameFormat().getThreeDFormat().getLightRig().setLightType(LightRigPresetType.Balanced);
textFrame.getTextFrameFormat().getThreeDFormat().getLightRig().setRotation(0, 0, 40);
textFrame.getTextFrameFormat().getThreeDFormat().getCamera().setCameraType(CameraPresetType.PerspectiveContrastingRightFacing);
// Guardar presentación
pres.save("3D-Text.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
La siguiente captura de pantalla muestra el resultado del ejemplo de código anterior.

Crear una forma 3D en PowerPoint en Java
De forma similar al texto, puede aplicar efectos 3D a las formas en las presentaciones de PowerPoint. Los siguientes son los pasos para crear una forma 3D en PowerPoint PPT usando Java.
- Primero, cree un nuevo PPT usando la clase Presentation.
- Agregue una nueva forma de rectángulo usando el método addAutoShape().
- Establezca el texto de la forma usando el método IAutoShape.getTextFrame.setText().
- Aplique efectos 3D a la forma usando IThreeDFormat devuelto por el método IAutoShape.getThreeDFormat().
- Finalmente, guarde la presentación usando el método Presentation.save(String, SaveFormat).
El siguiente ejemplo de código muestra cómo aplicar efectos 3D a formas en PowerPoint usando Java.
// Crear presentación
Presentation pres = new Presentation();
try {
// Añadir forma de rectángulo
IAutoShape shape = pres.getSlides().get_Item(0).getShapes().addAutoShape(ShapeType.Rectangle, 200, 150, 200, 200);
// Establecer texto para la forma
shape.getTextFrame().setText("3D");
shape.getTextFrame().getParagraphs().get_Item(0).getParagraphFormat().getDefaultPortionFormat().setFontHeight(64);
// Aplicar efectos 3D
shape.getThreeDFormat().getCamera().setCameraType(CameraPresetType.OrthographicFront);
shape.getThreeDFormat().getCamera().setRotation(20, 30, 40);
shape.getThreeDFormat().getLightRig().setLightType(LightRigPresetType.Flat);
shape.getThreeDFormat().getLightRig().setDirection(LightingDirection.Top);
shape.getThreeDFormat().setMaterial(MaterialPresetType.Flat);
shape.getThreeDFormat().setExtrusionHeight(100);
shape.getThreeDFormat().getExtrusionColor().setColor(Color.BLUE);
// Guardar presentación
pres.save("3D-Shape.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
La siguiente es la forma 3D que obtenemos después de ejecutar este código.
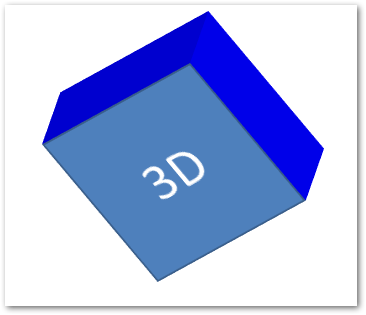
Crear degradado para formas 3D
También puede aplicar efectos de degradado a las formas siguiendo los pasos a continuación.
- Primero, cree un nuevo PPT usando la clase Presentation.
- Agregue una nueva forma de rectángulo usando el método addAutoShape().
- Establezca el texto de la forma usando la propiedad IAutoShape.getTextFrame().setText().
- Establezca el tipo de relleno de la forma en FillType.Gradient y establezca los colores del degradado.
- Aplique efectos 3D a la forma usando IThreeDFormat devuelto por el método IAutoShape.getThreeDFormat().
- Finalmente, guarde la presentación usando el método Presentation.save(String, SaveFormat).
El siguiente ejemplo de código muestra cómo aplicar efectos de degradado a formas en un PPT de PowerPoint.
// Crear presentación
Presentation pres = new Presentation();
try {
// Añadir forma de rectángulo
IAutoShape shape = pres.getSlides().get_Item(0).getShapes().addAutoShape(ShapeType.Rectangle, 200, 150, 200, 200);
// Establecer texto para la forma
shape.getTextFrame().setText("3D");
shape.getTextFrame().getParagraphs().get_Item(0).getParagraphFormat().getDefaultPortionFormat().setFontHeight(64);
shape.getFillFormat().setFillType(FillType.Gradient);
shape.getFillFormat().getGradientFormat().getGradientStops().add(0, Color.BLUE);
shape.getFillFormat().getGradientFormat().getGradientStops().add(100, Color.MAGENTA);
// Aplicar efectos 3D
shape.getThreeDFormat().getCamera().setCameraType(CameraPresetType.OrthographicFront);
shape.getThreeDFormat().getCamera().setRotation(10, 20, 30);
shape.getThreeDFormat().getLightRig().setLightType(LightRigPresetType.Flat);
shape.getThreeDFormat().getLightRig().setDirection(LightingDirection.Top);
shape.getThreeDFormat().setExtrusionHeight(150);
shape.getThreeDFormat().getExtrusionColor().setColor(new Color(255, 140, 0));
// Guardar presentación
pres.save("3D-Shape-Gradient.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
La siguiente es la forma 3D después de aplicar el efecto degradado.
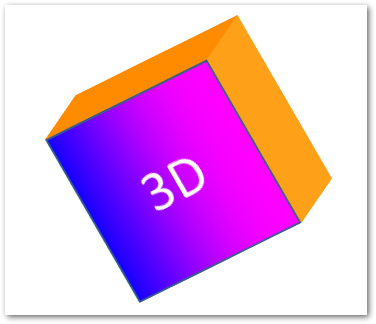
Aplicar efectos 3D a una imagen en PowerPoint en Java
Aspose.Slides for Java también le permite aplicar efectos 3D a una imagen. Los siguientes son los pasos para realizar esta operación en Java.
- Cree un nuevo PPT usando la clase Presentation.
- Agregue una nueva forma de rectángulo usando el método addAutoShape().
- Establezca el tipo de relleno de la forma en FillType.Picture y agregue la imagen.
- Aplique efectos 3D a la forma usando IThreeDFormat devuelto por el método IAutoShape.getThreeDFormat().
- Guarde la presentación usando el método Presentation.save(String, SaveFormat).
Los siguientes son los pasos para aplicar efectos 3D a una imagen en PPT usando Java.
// Crear presentación
Presentation pres = new Presentation();
try {
// Añadir forma de rectángulo
IAutoShape shape = pres.getSlides().get_Item(0).getShapes().addAutoShape(ShapeType.Rectangle, 200, 150, 200, 200);
// Establecer imagen para la forma
shape.getFillFormat().setFillType(FillType.Picture);
IPPImage picture = null;
try {
picture = pres.getImages().addImage(Files.readAllBytes(Paths.get("tiger.bmp")));
} catch (IOException e) { }
shape.getFillFormat().getPictureFillFormat().getPicture().setImage(picture);
shape.getFillFormat().getPictureFillFormat().setPictureFillMode(PictureFillMode.Stretch);
// Aplicar efectos 3D
shape.getThreeDFormat().getCamera().setCameraType(CameraPresetType.OrthographicFront);
shape.getThreeDFormat().getCamera().setRotation(10, 20, 30);
shape.getThreeDFormat().getLightRig().setLightType(LightRigPresetType.Flat);
shape.getThreeDFormat().getLightRig().setDirection(LightingDirection.Top);
shape.getThreeDFormat().setExtrusionHeight(150);
shape.getThreeDFormat().getExtrusionColor().setColor(Color.GRAY);
// Guardar presentación
pres.save("3D-Image.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
La siguiente es la imagen resultante que obtenemos después de aplicar efectos 3D.
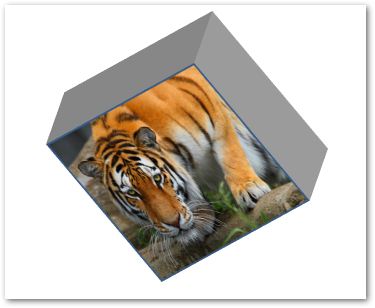
Obtenga una licencia gratis
Puede obtener una licencia temporal gratuita para usar Aspose.Slides for Java sin limitaciones de evaluación.
Conclusión
En este artículo, aprendió cómo aplicar efectos 3D en PowerPoint PPT/PPTX usando Java. Con la ayuda de ejemplos de código, hemos demostrado cómo crear texto o formas 3D y aplicar efectos 3D a imágenes en presentaciones PPT o PPTX. Puede visitar la documentación para explorar más sobre Aspose.Slides for Java. Además, puede publicar sus preguntas o consultas en nuestro foro.