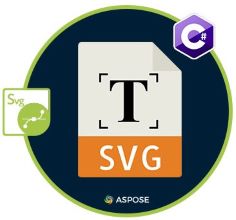
El elemento de texto SVG se utiliza para definir un texto en SVG. SVG (Gráficos vectoriales escalables) es un formato de archivo vectorial compatible con la web. Se utiliza para mostrar información visual en una página web. Podemos escribir fácilmente cualquier texto en SVG utilizando el elemento Texto SVG. En este artículo, aprenderemos cómo convertir texto a SVG en C#.
En este artículo se tratarán los siguientes temas:
- API de C# para convertir texto a SVG
- Qué es el texto SVG
- Convertir texto a SVG
- Convertir texto con TSpan a SVG
- Texto SVG con TextPath
- Aplicar estilos de texto SVG
API de C# para convertir texto a SVG
Para convertir texto a SVG, utilizaremos la API Aspose.SVG para .NET. Permite cargar, analizar, renderizar, crear y convertir archivos SVG a formatos populares sin dependencias de software.
La clase SVGDocument de la API representa la raíz de la jerarquía SVG y contiene todo el contenido. Tenemos el método Save() en esta clase que permite guardar SVG en la ruta de archivo especificada. La clase SVGTextElement representa el elemento ’texto’. La interfaz SVGTSpanElement corresponde al elemento ’tspan’. La API proporciona la clase SVGTextPathElement que representa el elemento ’textPath’ y SVGPathElement para el elemento ‘path’. Podemos establecer varias propiedades/atributos para elementos SVG usando el método SetAttribute(string, string). El método AppendChild(Node) de la API agrega el nuevo elemento secundario del nodo al final de la lista de elementos secundarios de este nodo.
Descargue la DLL de la API o instálela mediante NuGet.
PM> Install-Package Aspose.SVG
¿Qué es el texto SVG?
En SVG, el texto se representa usando el elemento . De forma predeterminada, el texto se representa con un relleno negro y sin contorno. Podemos definir los siguientes atributos:
- x: establece la posición del punto horizontalmente.
- y: establece la posición del punto verticalmente.
- relleno: define el color del texto renderizado.
- transformar: rotar, traducir, sesgar y escalar.
Convertir texto a SVG usando C#
Podemos agregar cualquier texto a SVG siguiendo los pasos que se detallan a continuación:
- En primer lugar, cree una instancia de la clase SVGDocument.
- A continuación, obtenga el elemento raíz del documento.
- Luego, cree el objeto de clase SVGTextElement.
- A continuación, establezca varios atributos utilizando el método SetAttribute().
- Después de eso, llame al método AppendChild() para agregarlo al elemento raíz.
- Finalmente, guarde la imagen SVG de salida usando el método Save().
El siguiente ejemplo de código muestra cómo convertir texto a SVG en C#.
// Este ejemplo de código demuestra cómo agregar texto a SVG.
var document = new SVGDocument();
// Obtenga el elemento svg raíz del documento
var svgElement = document.RootElement;
const string @namespace = "http://www.w3.org/2000/svg";
// Definir elemento de texto SVG
var text = (SVGTextElement)document.CreateElementNS(@namespace, "text");
// Definir texto para mostrar
text.TextContent = "The is a simple SVG text!";
// Establecer varios atributos
text.SetAttribute("fill", "blue");
text.SetAttribute("x", "10");
text.SetAttribute("y", "30");
// Agregar texto a la raíz
svgElement.AppendChild(text);
// Guardar como SVG
document.Save(@"C:\Files\simple-text.svg");
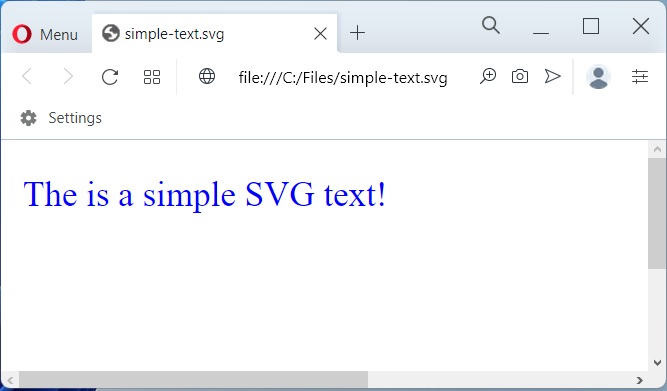
Convierte texto a SVG usando C#.
A continuación encontrará el contenido de la imagen simple-text.svg.
<svg xmlns="http://www.w3.org/2000/svg">
<text fill="blue" x="10" y="30">The is a simple SVG text!</text>
</svg>
Convierta texto con TSpan a SVG en C#
El elemento SVG define un subtexto dentro de un elemento . Podemos agregar cualquier texto con un elemento tspan a SVG siguiendo los pasos que se detallan a continuación:
- En primer lugar, cree una instancia de la clase SVGDocument.
- A continuación, obtenga el elemento raíz del documento.
- Luego, cree el objeto de clase SVGTextElement.
- Opcionalmente, establezca varios atributos usando el método SetAttribute().
- A continuación, defina el objeto SVGTSpanElement.
- Luego, establezca sus atributos usando el método SetAttribute().
- Ahora, llame al método AppendChild() para agregarlo al elemento SVGTextElement.
- Repita los pasos anteriores para agregar más objetos SVGTSpanElement.
- Después de eso, llame al método AppendChild() para agregar SVGTextElement al elemento raíz.
- Finalmente, guarde la imagen SVG de salida usando el método Save().
El siguiente ejemplo de código muestra cómo convertir texto con tspan a SVG en C#.
// Este ejemplo de código demuestra cómo agregar texto con tspan a SVG.
var document = new SVGDocument();
// Obtenga el elemento svg raíz del documento
var svgElement = document.RootElement;
const string @namespace = "http://www.w3.org/2000/svg";
// Elemento de texto SVG
var text = (SVGTextElement)document.CreateElementNS(@namespace, "text");
text.SetAttribute("style", "font-family:arial");
text.SetAttribute("x", "20");
text.SetAttribute("y", "60");
// Elemento SVG TSpan
var tspan1 = (SVGTSpanElement)document.CreateElementNS(@namespace, "tspan");
tspan1.TextContent = "ASPOSE";
tspan1.SetAttribute("style", "font-weight:bold; font-size:55px");
tspan1.SetAttribute("x", "20");
tspan1.SetAttribute("y", "60");
// Añadir a texto SVG
text.AppendChild(tspan1);
// Otro elemento TSpan
var tspan2 = (SVGTSpanElement)document.CreateElementNS(@namespace, "tspan");
tspan2.TextContent = "Your File Format APIs";
tspan2.SetAttribute("style", "font-size:20px; fill:grey");
tspan2.SetAttribute("x", "37");
tspan2.SetAttribute("y", "90");
// Agregar al texto SVG
text.AppendChild(tspan2);
// Agregar texto SVG a la raíz
svgElement.AppendChild(text);
// Guardar el SVG
document.Save(@"C:\Files\svg-tSpan.svg");
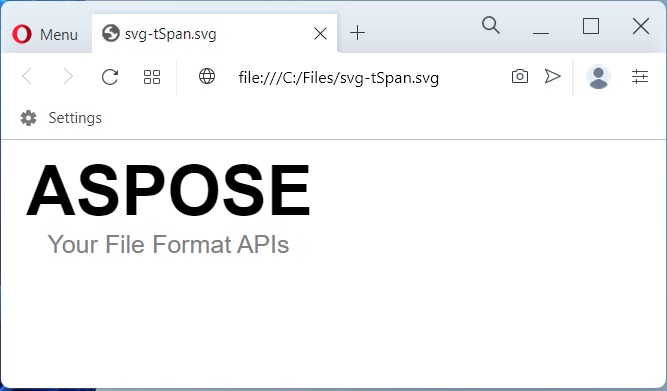
Convierta texto con tspan a SVG en C#.
A continuación encontrará el contenido de la imagen svg-tspan.svg.
<svg xmlns="http://www.w3.org/2000/svg">
<text style="font-family: arial;" x="20" y="60">
<tspan style="font-weight: bold; font-size: 55px;" x="20" y="60">ASPOSE</tspan>
<tspan style="font-size: 20px; fill: grey;" x="37" y="90">Your File Format APIs</tspan>
</text>
</svg>
Texto SVG con TextPath en C#
También podemos representar texto a lo largo de la forma de un elemento y encerrar el texto en un elemento . Permite establecer una referencia al elemento usando el atributo href. Podemos convertir texto con rutas de texto siguiendo los pasos que se detallan a continuación:
- En primer lugar, cree una instancia de la clase SVGDocument.
- A continuación, obtenga el elemento raíz del documento.
- Luego, cree el objeto de clase SVGPathElement y configure varios atributos usando el método SetAttribute().
- Después de eso, llame al método AppendChild() para agregarlo al elemento raíz.
- A continuación, cree el objeto de clase SVGTextElement.
- Luego, inicialice el objeto SVGTextPathElement y configure el contenido del texto.
- A continuación, asigne SVGPathElement a su atributo href utilizando el método SetAttribute().
- Luego, llame al método AppendChild() para agregar SVGTextPathElement al elemento SVGTextElement.
- Después de eso, agregue SVGTextElement al elemento raíz usando el método AppendChild().
- Finalmente, guarde la imagen SVG de salida usando el método Save().
El siguiente ejemplo de código muestra cómo convertir texto con textPath a SVG en C#.
// Este ejemplo de código demuestra cómo agregar texto con textPath a SVG.
var document = new SVGDocument();
// Obtenga el elemento svg raíz del documento
var svgElement = document.RootElement;
const string @namespace = "http://www.w3.org/2000/svg";
// Elemento de ruta SVG
var path1 = (SVGPathElement)document.CreateElementNS(@namespace, "path");
path1.SetAttribute("id", "path_1");
path1.SetAttribute("d", "M 50 100 Q 25 10 180 100 T 350 100 T 520 100 T 690 100");
path1.SetAttribute("fill", "transparent");
// Agregar ruta SVG al elemento raíz
svgElement.AppendChild(path1);
// Another Elemento de ruta SVG
var path2 = (SVGPathElement)document.CreateElementNS(@namespace, "path");
path2.SetAttribute("id", "path_2");
path2.SetAttribute("d", "M 50 100 Q 25 10 180 100 T 350 100");
path2.SetAttribute("transform", "translate(0,75)");
path2.SetAttribute("fill", "transparent");
// Agregar ruta SVG al elemento raíz
svgElement.AppendChild(path2);
// Elemento de texto SVG
var text = (SVGTextElement)document.CreateElementNS(@namespace, "text");
// Crear elemento de ruta de texto SVG
var textPath1 = (SVGTextPathElement)document.CreateElementNS(@namespace, "textPath");
textPath1.TextContent = "Aspose.SVG for .NET is flexible library for SVG files processing and fully compatible with its specifications.";
textPath1.SetAttribute("href", "#path_1");
// Agregar ruta de texto SVG a texto SVG
text.AppendChild(textPath1);
// Otro elemento de ruta de texto SVG
var textPath2 = (SVGTextPathElement)document.CreateElementNS(@namespace, "textPath");
textPath2.TextContent = "Aspose.SVG for .NET is flexible library for SVG files processing and fully compatible with its specifications.";
textPath2.SetAttribute("href", "#path_2");
// Agregar ruta de texto SVG a texto SVG
text.AppendChild(textPath2);
// Agregar texto SVG a la raíz
svgElement.AppendChild(text);
// Guardar el SVG
document.Save(@"C:\Files\svg-textPath.svg");
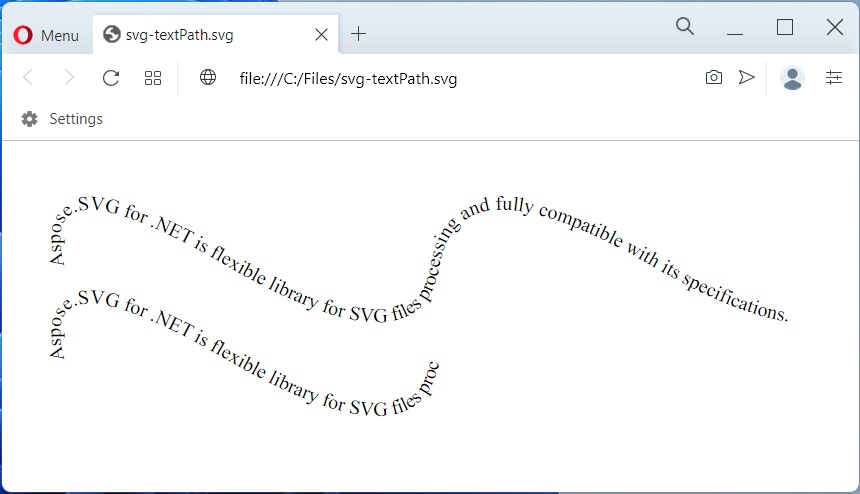
Convierta texto con textPath a SVG en C#.
A continuación encontrará el contenido de la imagen svg-textPath.svg.
<svg xmlns="http://www.w3.org/2000/svg">
<path id="path_1" d="M 50 100 Q 25 10 180 100 T 350 100 T 520 100 T 690 100" fill="transparent"/>
<path id="path_2" d="M 50 100 Q 25 10 180 100 T 350 100" transform="translate(0,75)" fill="transparent"/>
<text>
<textPath href="#path_1">Aspose.SVG for .NET is flexible library for SVG files processing and fully compatible with its specifications.</textPath>
<textPath href="#path_2">Aspose.SVG for .NET is flexible library for SVG files processing and fully compatible with its specifications.</textPath>
</text>
</svg>
Aplicar estilo de texto SVG en C#
Podemos agregar cualquier texto a SVG siguiendo los pasos que se detallan a continuación:
- En primer lugar, cree una instancia de la clase SVGDocument.
- A continuación, obtenga el elemento raíz del documento.
- Luego, cree el objeto de clase SVGTextElement.
- A continuación, establezca el atributo de estilo mediante el método SetAttribute().
- Después de eso, llame al método AppendChild() para agregarlo al elemento raíz.
- Finalmente, guarde la imagen SVG de salida usando el método Save().
El siguiente ejemplo de código muestra cómo aplicar estilos CSS a texto SVG en C#.
// Este ejemplo de código demuestra cómo agregar texto a SVG y aplicar atributos de estilo CSS.
var document = new SVGDocument();
// Obtenga el elemento svg raíz del documento
var svgElement = document.RootElement;
const string @namespace = "http://www.w3.org/2000/svg";
// Definir elemento de texto SVG
var text = (SVGTextElement)document.CreateElementNS(@namespace, "text");
// Definir texto para mostrar
text.TextContent = "The is a simple SVG text!";
// Establecer varios atributos
text.SetAttribute("fill", "blue");
text.SetAttribute("x", "10");
text.SetAttribute("y", "30");
// Aplicar estilo
text.SetAttribute("style", "font-weight:bold; font-style: italic; text-decoration: line-through; text-transform: capitalize;");
// Agregar texto a la raíz
svgElement.AppendChild(text);
// Guardar como SVG
document.Save(@"C:\Files\text-style.svg");
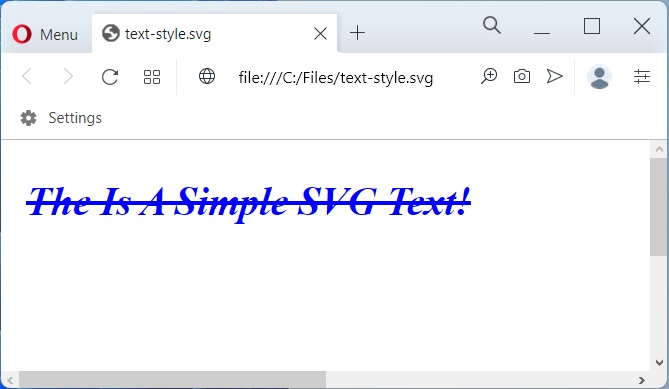
Aplicar estilo de texto SVG en C#.
A continuación encontrará el contenido de la imagen text-style.svg.
<svg xmlns="http://www.w3.org/2000/svg">
<text fill="blue" x="10" y="30" style="font-weight: bold; font-style: italic; text-decoration-line: line-through; text-transform: capitalize;">The is a simple SVG text!</text>
</svg>
Obtenga una Licencia Temporal Gratis
Puede obtener una licencia temporal gratuita para probar Aspose.SVG para .NET sin limitaciones de evaluación.
Conclusión
En este artículo, hemos aprendido a:
- crear una nueva imagen SVG;
- usar elementos de texto SVG;
- representar texto SVG en la ruta;
- establecer la posición y los atributos de relleno para el texto SVG;
- establecer atributos de estilo para el texto SVG en C#.
Además de convertir texto a SVG en C#, puede obtener más información sobre Aspose.SVG para .NET en la documentación y explorar las diferentes funciones compatibles con la API. En caso de cualquier ambigüedad, no dude en contactarnos en nuestro foro de soporte gratuito.