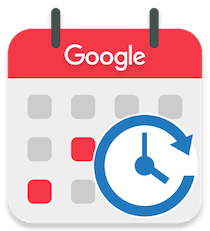
Google Calendar یک سرویس زمانبندی است که به شما امکان میدهد رویدادهایی مانند جلسات را ایجاد و پیگیری کنید. میتوانید رویدادها را در تقویم ثبت کنید و یادآوریهایی درباره رویدادهای آینده دریافت کنید. گوگل همچنین به شما اجازه می دهد تا از سرویس تقویم خود به صورت برنامه ریزی شده استفاده کنید. بنابراین، میتوانید رویدادهای خود را با استفاده از تقویمهای Google از درون برنامههای خود مدیریت کنید. در این مقاله با نحوه ایجاد، به روز رسانی و حذف تقویم گوگل در سی شارپ آشنا می شوید.
- C# API برای ایجاد و بهروزرسانی تقویم Google
- ایجاد تقویم گوگل در سی شارپ
- به روز رسانی تقویم گوگل در سی شارپ
- حذف تقویم گوگل در سی شارپ
C# API برای ایجاد و بهروزرسانی تقویم Google - دانلود رایگان
برای کار با سرویس Google Calendar، از Aspose.Email for .NET استفاده می کنیم. این یک API قدرتمند است که طیف وسیعی از ویژگیها را برای پردازش ایمیلها، کار با کلاینتهای ایمیل و استفاده از سرویسهای همکاری Google ارائه میکند. می توانید DLL API را دانلود یا با استفاده از دستور زیر از NuGet نصب کنید.
PM> Install-Package Aspose.Email
قبل از شروع کار، باید یک پروژه در کنسول برنامه نویس گوگل ایجاد کنید که به برنامه شما اجازه می دهد با سرویس های Google ارتباط برقرار کند. برای ایجاد یکی، می توانید [این راهنما] را دنبال کنید.
برای دسترسی و دستکاری Google Calendar، باید کدی بنویسیم تا اطلاعات کاربر را مدیریت کرده و احراز هویت را انجام دهیم. برای کاربر گوگل، ابتدا یک کلاس با نام TestUser ایجاد می کنیم و سپس آن را از کلاس GoogleUser به ارث می بریم. در زیر اجرای کامل هر دو کلاس آورده شده است.
using System;
namespace Aspose.Email
{
internal enum GrantTypes
{
authorization_code,
refresh_token
}
public class TestUser
{
internal TestUser(string name, string eMail, string password, string domain)
{
Name = name;
EMail = eMail;
Password = password;
Domain = domain;
}
public readonly string Name;
public readonly string EMail;
public readonly string Password;
public readonly string Domain;
public static bool operator ==(TestUser x, TestUser y)
{
if ((object)x != null)
return x.Equals(y);
if ((object)y != null)
return y.Equals(x);
return true;
}
public static bool operator !=(TestUser x, TestUser y)
{
return !(x == y);
}
public static implicit operator string(TestUser user)
{
return user == null ? null : user.Name;
}
public override int GetHashCode()
{
return ToString().GetHashCode();
}
public override bool Equals(object obj)
{
return obj != null && obj is TestUser && this.ToString().Equals(obj.ToString(), StringComparison.OrdinalIgnoreCase);
}
public override string ToString()
{
return string.IsNullOrEmpty(Domain) ? Name : string.Format("{0}/{1}", Domain, Name);
}
}
public class GoogleUser : TestUser
{
public GoogleUser(string name, string eMail, string password)
: this(name, eMail, password, null, null, null)
{ }
public GoogleUser(string name, string eMail, string password, string clientId, string clientSecret)
: this(name, eMail, password, clientId, clientSecret, null)
{ }
public GoogleUser(string name, string eMail, string password, string clientId, string clientSecret, string refreshToken)
: base(name, eMail, password, "gmail.com")
{
ClientId = clientId;
ClientSecret = clientSecret;
RefreshToken = refreshToken;
}
public readonly string ClientId;
public readonly string ClientSecret;
public readonly string RefreshToken;
}
}
اکنون، باید یک کلاس کمکی ایجاد کنیم که از احراز هویت یک حساب Google مراقبت کند. نام این کلاس را GoogleOAuthHelper میگذاریم. در ادامه پیاده سازی کامل این کلاس ارائه شده است.
using System;
using System.Diagnostics;
using System.IO;
using System.Net;
using System.Text;
using System.Threading;
using System.Windows.Forms;
namespace Aspose.Email
{
internal class GoogleOAuthHelper
{
public const string TOKEN_REQUEST_URL = "https://accounts.google.com/o/oauth2/token";
public const string SCOPE = "https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fcalendar" + "+" + "https%3A%2F%2Fwww.google.com%2Fm8%2Ffeeds%2F" + "+" + "https%3A%2F%2Fmail.google.com%2F"; // IMAP & SMTP
public const string REDIRECT_URI = "urn:ietf:wg:oauth:2.0:oob";
public const string REDIRECT_TYPE = "code";
internal static string GetAccessToken(TestUser user)
{
return GetAccessToken((GoogleUser)user);
}
internal static string GetAccessToken(GoogleUser user)
{
string access_token;
string token_type;
int expires_in;
GetAccessToken(user, out access_token, out token_type, out expires_in);
return access_token;
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string token_type, out int expires_in)
{
access_token = null;
token_type = null;
expires_in = 0;
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(TOKEN_REQUEST_URL);
request.CookieContainer = new CookieContainer();
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
string encodedParameters = string.Format("client_id={0}&client_secret={1}&refresh_token={2}&grant_type={3}",
System.Web.HttpUtility.UrlEncode(user.ClientId), System.Web.HttpUtility.UrlEncode(user.ClientSecret), System.Web.HttpUtility.UrlEncode(user.RefreshToken), System.Web.HttpUtility.UrlEncode(GrantTypes.refresh_token.ToString()));
byte[] requestData = Encoding.UTF8.GetBytes(encodedParameters);
request.ContentLength = requestData.Length;
if (requestData.Length > 0)
using (Stream stream = request.GetRequestStream())
stream.Write(requestData, 0, requestData.Length);
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
string responseText = null;
using (TextReader reader = new StreamReader(response.GetResponseStream(), Encoding.ASCII))
responseText = reader.ReadToEnd();
foreach (string sPair in responseText.Replace("{", "").Replace("}", "").Replace("\"", "").Split(new string[] { ",\n" }, StringSplitOptions.None))
{
string[] pair = sPair.Split(':');
string name = pair[0].Trim().ToLower();
string value = System.Web.HttpUtility.UrlDecode(pair[1].Trim());
switch (name)
{
case "access_token":
access_token = value;
break;
case "token_type":
token_type = value;
break;
case "expires_in":
expires_in = Convert.ToInt32(value);
break;
}
}
Debug.WriteLine("");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("-----------OAuth 2.0 authorization information-----------");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine(string.Format("Login: '{0}'", user.EMail));
Debug.WriteLine(string.Format("Access token: '{0}'", access_token));
Debug.WriteLine(string.Format("Token type: '{0}'", token_type));
Debug.WriteLine(string.Format("Expires in: '{0}'", expires_in));
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("");
}
internal static void GetAccessToken(TestUser user, out string access_token, out string refresh_token)
{
GetAccessToken((GoogleUser)user, out access_token, out refresh_token);
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string refresh_token)
{
string token_type;
int expires_in;
GoogleOAuthHelper.GetAccessToken(user, out access_token, out refresh_token, out token_type, out expires_in);
}
internal static void GetAccessToken(TestUser user, out string access_token, out string refresh_token, out string token_type, out int expires_in)
{
GetAccessToken((GoogleUser)user, out access_token, out refresh_token, out token_type, out expires_in);
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string refresh_token, out string token_type, out int expires_in)
{
string authorizationCode = GoogleOAuthHelper.GetAuthorizationCode(user, GoogleOAuthHelper.SCOPE, GoogleOAuthHelper.REDIRECT_URI, GoogleOAuthHelper.REDIRECT_TYPE);
GoogleOAuthHelper.GetAccessToken(authorizationCode, user, out access_token, out token_type, out expires_in, out refresh_token);
}
internal static void GetAccessToken(string authorizationCode, TestUser user, out string access_token, out string token_type, out int expires_in, out string refresh_token)
{
GetAccessToken(authorizationCode, (GoogleUser)user, out access_token, out token_type, out expires_in, out refresh_token);
}
internal static void GetAccessToken(string authorizationCode, GoogleUser user, out string access_token, out string token_type, out int expires_in, out string refresh_token)
{
access_token = null;
token_type = null;
expires_in = 0;
refresh_token = null;
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(TOKEN_REQUEST_URL);
request.CookieContainer = new CookieContainer();
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
string encodedParameters = string.Format("client_id={0}&code={1}&client_secret={2}&redirect_uri={3}&grant_type={4}", System.Web.HttpUtility.UrlEncode(user.ClientId), System.Web.HttpUtility.UrlEncode(authorizationCode), System.Web.HttpUtility.UrlEncode(user.ClientSecret), System.Web.HttpUtility.UrlEncode(REDIRECT_URI), System.Web.HttpUtility.UrlEncode(GrantTypes.authorization_code.ToString()));
byte[] requestData = Encoding.UTF8.GetBytes(encodedParameters);
request.ContentLength = requestData.Length;
if (requestData.Length > 0)
using (Stream stream = request.GetRequestStream())
stream.Write(requestData, 0, requestData.Length);
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
string responseText = null;
using (TextReader reader = new StreamReader(response.GetResponseStream(), Encoding.ASCII))
responseText = reader.ReadToEnd();
foreach (string sPair in responseText.Replace("{", "").Replace("}", "").Replace("\"", "").Split(new string[] { ",\n" }, StringSplitOptions.None))
{
string[] pair = sPair.Split(':');
string name = pair[0].Trim().ToLower();
string value = System.Web.HttpUtility.UrlDecode(pair[1].Trim());
switch (name)
{
case "access_token":
access_token = value;
break;
case "token_type":
token_type = value;
break;
case "expires_in":
expires_in = Convert.ToInt32(value);
break;
case "refresh_token":
refresh_token = value;
break;
}
}
Debug.WriteLine(string.Format("Authorization code: '{0}'", authorizationCode));
Debug.WriteLine(string.Format("Access token: '{0}'", access_token));
Debug.WriteLine(string.Format("Refresh token: '{0}'", refresh_token));
Debug.WriteLine(string.Format("Token type: '{0}'", token_type));
Debug.WriteLine(string.Format("Expires in: '{0}'", expires_in));
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("");
}
internal static string GetAuthorizationCode(TestUser acc, string scope, string redirectUri, string responseType)
{
return GetAuthorizationCode((GoogleUser)acc, scope, redirectUri, responseType);
}
internal static string GetAuthorizationCode(GoogleUser acc, string scope, string redirectUri, string responseType)
{
Debug.WriteLine("");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("-----------OAuth 2.0 authorization information-----------");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine(string.Format("Login: '{0}'", acc.EMail));
string authorizationCode = null;
string error = null;
string approveUrl = string.Format("https://accounts.google.com/o/oauth2/auth?redirect_uri={0}&response_type={1}&client_id={2}&scope={3}", redirectUri, responseType, acc.ClientId, scope);
AutoResetEvent are0 = new AutoResetEvent(false);
Thread t = new Thread(delegate ()
{
bool doEvents = true;
WebBrowser browser = new WebBrowser();
browser.AllowNavigation = true;
browser.DocumentCompleted += delegate (object sender, WebBrowserDocumentCompletedEventArgs e) { doEvents = false; };
Form f = new Form();
f.FormBorderStyle = FormBorderStyle.FixedToolWindow;
f.ShowInTaskbar = false;
f.StartPosition = FormStartPosition.Manual;
f.Location = new System.Drawing.Point(-2000, -2000);
f.Size = new System.Drawing.Size(1, 1);
f.Controls.Add(browser);
f.Load += delegate (object sender, EventArgs e)
{
try
{
browser.Navigate("https://accounts.google.com/Logout");
doEvents = true;
while (doEvents) Application.DoEvents();
browser.Navigate("https://accounts.google.com/ServiceLogin?sacu=1");
doEvents = true;
while (doEvents) Application.DoEvents();
HtmlElement loginForm = browser.Document.Forms["gaia_loginform"];
if (loginForm != null)
{
HtmlElement userName = browser.Document.All["Email"];
userName.SetAttribute("value", acc.EMail);
loginForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
loginForm = browser.Document.Forms["gaia_loginform"];
HtmlElement passwd = browser.Document.All["Passwd"];
passwd.SetAttribute("value", acc.Password);
loginForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
}
else
{
error = "Login form is not found in \n" + browser.Document.Body.InnerHtml;
return;
}
browser.Navigate(approveUrl);
doEvents = true;
while (doEvents) Application.DoEvents();
HtmlElement approveForm = browser.Document.Forms["connect-approve"];
if (approveForm != null)
{
HtmlElement submitAccess = browser.Document.All["submit_access"];
submitAccess.SetAttribute("value", "true");
approveForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
}
else
{
error = "Approve form is not found in \n" + browser.Document.Body.InnerHtml;
return;
}
HtmlElement code = browser.Document.All["code"];
if (code != null)
authorizationCode = code.GetAttribute("value");
else
error = "Authorization code is not found in \n" + browser.Document.Body.InnerHtml;
}
catch (Exception ex)
{
error = ex.Message;
}
finally
{
f.Close();
}
};
Application.Run(f);
are0.Set();
});
t.SetApartmentState(ApartmentState.STA);
t.Start();
are0.WaitOne();
if (error != null)
throw new Exception(error);
return authorizationCode;
}
}
}
ایجاد تقویم گوگل در سی شارپ
پس از انجام تنظیمات فوق، می توانید به کار با سرویس Google Calendar ادامه دهید. در زیر مراحل ایجاد و به روز رسانی تقویم گوگل در سی شارپ آمده است.
- نمونه ای از کلاس GmailClient را با استفاده از روش GmailClient.GetInstance(String, String) در یک شی IGmailClient دریافت کنید.
- یک نمونه از کلاس تقویم ایجاد کنید و آن را با نام، توضیحات و خصوصیات دیگر مقداردهی کنید.
- برای ایجاد تقویم Google با روش IGmailClient.CreateCalendar(Calendar) تماس بگیرید.
- شناسه برگشتی تقویم را دریافت کنید.
نمونه کد زیر نحوه ایجاد تقویم گوگل در سی شارپ را نشان می دهد.
// کاربر گوگل را راه اندازی کنید
GoogleUser User = new GoogleUser("user", "email address", "password", "clientId", "client secret");
string accessToken;
string refreshToken;
// رمز دسترسی را دریافت کنید
GoogleOAuthHelper.GetAccessToken(User, out accessToken, out refreshToken);
// نمونه ای از سرویس گیرنده Gmail را دریافت کنید
using (IGmailClient client = GmailClient.GetInstance(accessToken, User.EMail))
{
// درج و دریافت تقویم
Aspose.Email.Clients.Google.Calendar calendar = new Aspose.Email.Clients.Google.Calendar("summary - " + Guid.NewGuid().ToString(), null, null, "America/Los_Angeles");
// درج تقویم و بازیابی شناسه
string id = client.CreateCalendar(calendar);
Aspose.Email.Clients.Google.Calendar cal = client.FetchCalendar(id);
}
C# یک تقویم گوگل را به روز کنید
مراحل زیر برای به روز رسانی یک تقویم گوگل به صورت برنامه نویسی در سی شارپ است.
- نمونه ای از کلاس GmailClient را با استفاده از روش [GmailClient.GetInstance(String, String]]13 در یک شی IGmailClient دریافت کنید.
- از روش IGmailClient.FetchCalendar(String) برای واکشی نمونه تقویم با استفاده از شناسه آن استفاده کنید.
- ویژگی های تقویم را به روز کنید و با روش IGmailClient.UpdateCalendar(Calendar) تماس بگیرید تا تقویم را به روز کنید.
نمونه کد زیر نحوه به روز رسانی تقویم گوگل در سی شارپ را نشان می دهد.
// نمونه ای از سرویس گیرنده Gmail را دریافت کنید
using (IGmailClient client = GmailClient.GetInstance(accessToken, User.EMail))
{
string id = "<<calendar ID>>";
// واکشی تقویم
Aspose.Email.Clients.Google.Calendar cal = client.FetchCalendar(id);
// تغییر اطلاعات در تقویم واکشی شده و به روز رسانی تقویم
cal.Description = "Description - " + Guid.NewGuid().ToString();
cal.Location = "Location - " + Guid.NewGuid().ToString();
// به روز رسانی تقویم
client.UpdateCalendar(cal);
}
C# یک تقویم گوگل را حذف کنید
همچنین می توانید یک تقویم خاص را با استفاده از Aspose.Email برای دات نت حذف کنید. مراحل زیر برای انجام این عملیات آورده شده است.
- نمونه ای از کلاس GmailClient را با استفاده از روش GmailClient.GetInstance(String, String) در یک شی IGmailClient دریافت کنید.
- لیست تقویم ها را با استفاده از روش IGmailClient.ListCalendars() دریافت کنید.
- لیست را حلقه زده و مورد نظر را فیلتر کنید.
- با استفاده از روش IGmailClient.DeleteCalendar(Calendar.Id) تقویم را حذف کنید.
نمونه کد زیر نحوه حذف تقویم گوگل در سی شارپ را نشان می دهد.
// نمونه ای از سرویس گیرنده Gmail را دریافت کنید
using (IGmailClient client = GmailClient.GetInstance(accessToken, User.EMail))
{
// دسترسی و حذف تقویم با خلاصه از "خلاصه تقویم - "
string summary = "Calendar summary - ";
// دریافت لیست تقویم
ExtendedCalendar[] lst0 = client.ListCalendars();
foreach (ExtendedCalendar extCal in lst0)
{
// حذف تقویم های انتخاب شده
if (extCal.Summary.StartsWith(summary))
client.DeleteCalendar(extCal.Id);
}
}
C# API for Google Calendar - یک مجوز رایگان دریافت کنید
می توانید برای استفاده از Aspose.Email برای دات نت بدون محدودیت ارزیابی، مجوز موقت رایگان دریافت کنید.
نتیجه
در این مقاله با نحوه ایجاد تقویم گوگل به صورت برنامه نویسی در سی شارپ آشنا شدید. علاوه بر این، نحوه به روز رسانی و حذف یک تقویم خاص گوگل در سی شارپ را مشاهده کرده اید. علاوه بر این، میتوانید اسناد را برای مطالعه بیشتر درباره Aspose.Email برای دات نت کاوش کنید. همچنین، میتوانید سؤالات خود را از طریق [تالار گفتمان23 ما بپرسید.