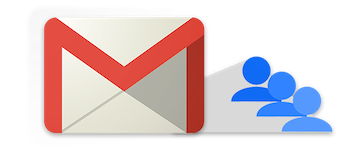
Gmail یک سرویس ایمیل محبوب و پرکاربرد است که توسط Google ارائه میشود. همراه با ارسال و دریافت ایمیل، ویژگی های اضافی مختلفی مانند پاسخ خودکار، چت و غیره را ارائه می دهد. به عنوان یک برنامه نویس، ممکن است زمانی که نیاز به وارد کردن مخاطبین از یک حساب جی میل خاص دارید، با این سناریو مواجه شوید. برای انجام این کار از داخل برنامههای NET، این مقاله نحوه وارد کردن مخاطبین Gmail در C#.NET را پوشش میدهد. علاوه بر این، نحوه واکشی مخاطبین از یک گروه ایمیل خاص را نشان خواهیم داد.
C# .NET API برای وارد کردن مخاطبین Gmail
برای وارد کردن مخاطبین از یک حساب Gmail، از Aspose.Email for .NET استفاده خواهیم کرد. این یک API غنی از ویژگی ها است که به شما امکان می دهد تا به راحتی ایمیل ایجاد و ارسال کنید. علاوه بر این، به شما امکان می دهد فرمت های ایمیل مختلف از جمله MSG و EML را دستکاری کنید. می توانید DLL API را دانلود یا با استفاده از NuGet نصب کنید.
PM> Install-Package Aspose.Email
وارد کردن مخاطبین از جیمیل در سی شارپ
برای دسترسی به مخاطبین از حساب جیمیل، باید کدی بنویسیم تا اطلاعات کاربر را مدیریت کنیم و احراز هویت جیمیل را انجام دهیم. برای کاربر جیمیل، ابتدا کلاسی به نام TestUser ایجاد می کنیم و سپس آن را از کلاس GoogleUser به ارث می بریم. در زیر اجرای کامل هر دو کلاس آورده شده است.
using System;
namespace Aspose.Email
{
internal enum GrantTypes
{
authorization_code,
refresh_token
}
public class TestUser
{
internal TestUser(string name, string eMail, string password, string domain)
{
Name = name;
EMail = eMail;
Password = password;
Domain = domain;
}
public readonly string Name;
public readonly string EMail;
public readonly string Password;
public readonly string Domain;
public static bool operator ==(TestUser x, TestUser y)
{
if ((object)x != null)
return x.Equals(y);
if ((object)y != null)
return y.Equals(x);
return true;
}
public static bool operator !=(TestUser x, TestUser y)
{
return !(x == y);
}
public static implicit operator string(TestUser user)
{
return user == null ? null : user.Name;
}
public override int GetHashCode()
{
return ToString().GetHashCode();
}
public override bool Equals(object obj)
{
return obj != null && obj is TestUser && this.ToString().Equals(obj.ToString(), StringComparison.OrdinalIgnoreCase);
}
public override string ToString()
{
return string.IsNullOrEmpty(Domain) ? Name : string.Format("{0}/{1}", Domain, Name);
}
}
public class GoogleUser : TestUser
{
public GoogleUser(string name, string eMail, string password)
: this(name, eMail, password, null, null, null)
{ }
public GoogleUser(string name, string eMail, string password, string clientId, string clientSecret)
: this(name, eMail, password, clientId, clientSecret, null)
{ }
public GoogleUser(string name, string eMail, string password, string clientId, string clientSecret, string refreshToken)
: base(name, eMail, password, "gmail.com")
{
ClientId = clientId;
ClientSecret = clientSecret;
RefreshToken = refreshToken;
}
public readonly string ClientId;
public readonly string ClientSecret;
public readonly string RefreshToken;
}
}
اکنون باید یک کلاس کمکی ایجاد کنیم که از احراز هویت یک حساب جیمیل مراقبت کند. نام این کلاس را GoogleOAuthHelper میگذاریم. در ادامه پیاده سازی کامل این کلاس ارائه شده است.
using System;
using System.Diagnostics;
using System.IO;
using System.Net;
using System.Text;
using System.Threading;
using System.Windows.Forms;
namespace Aspose.Email
{
internal class GoogleOAuthHelper
{
public const string TOKEN_REQUEST_URL = "https://accounts.google.com/o/oauth2/token";
public const string SCOPE = "https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fcalendar" + "+" + "https%3A%2F%2Fwww.google.com%2Fm8%2Ffeeds%2F" + "+" + "https%3A%2F%2Fmail.google.com%2F"; // IMAP & SMTP
public const string REDIRECT_URI = "urn:ietf:wg:oauth:2.0:oob";
public const string REDIRECT_TYPE = "code";
internal static string GetAccessToken(TestUser user)
{
return GetAccessToken((GoogleUser)user);
}
internal static string GetAccessToken(GoogleUser user)
{
string access_token;
string token_type;
int expires_in;
GetAccessToken(user, out access_token, out token_type, out expires_in);
return access_token;
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string token_type, out int expires_in)
{
access_token = null;
token_type = null;
expires_in = 0;
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(TOKEN_REQUEST_URL);
request.CookieContainer = new CookieContainer();
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
string encodedParameters = string.Format("client_id={0}&client_secret={1}&refresh_token={2}&grant_type={3}",
System.Web.HttpUtility.UrlEncode(user.ClientId), System.Web.HttpUtility.UrlEncode(user.ClientSecret), System.Web.HttpUtility.UrlEncode(user.RefreshToken), System.Web.HttpUtility.UrlEncode(GrantTypes.refresh_token.ToString()));
byte[] requestData = Encoding.UTF8.GetBytes(encodedParameters);
request.ContentLength = requestData.Length;
if (requestData.Length > 0)
using (Stream stream = request.GetRequestStream())
stream.Write(requestData, 0, requestData.Length);
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
string responseText = null;
using (TextReader reader = new StreamReader(response.GetResponseStream(), Encoding.ASCII))
responseText = reader.ReadToEnd();
foreach (string sPair in responseText.Replace("{", "").Replace("}", "").Replace("\"", "").Split(new string[] { ",\n" }, StringSplitOptions.None))
{
string[] pair = sPair.Split(':');
string name = pair[0].Trim().ToLower();
string value = System.Web.HttpUtility.UrlDecode(pair[1].Trim());
switch (name)
{
case "access_token":
access_token = value;
break;
case "token_type":
token_type = value;
break;
case "expires_in":
expires_in = Convert.ToInt32(value);
break;
}
}
Debug.WriteLine("");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("-----------OAuth 2.0 authorization information-----------");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine(string.Format("Login: '{0}'", user.EMail));
Debug.WriteLine(string.Format("Access token: '{0}'", access_token));
Debug.WriteLine(string.Format("Token type: '{0}'", token_type));
Debug.WriteLine(string.Format("Expires in: '{0}'", expires_in));
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("");
}
internal static void GetAccessToken(TestUser user, out string access_token, out string refresh_token)
{
GetAccessToken((GoogleUser)user, out access_token, out refresh_token);
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string refresh_token)
{
string token_type;
int expires_in;
GoogleOAuthHelper.GetAccessToken(user, out access_token, out refresh_token, out token_type, out expires_in);
}
internal static void GetAccessToken(TestUser user, out string access_token, out string refresh_token, out string token_type, out int expires_in)
{
GetAccessToken((GoogleUser)user, out access_token, out refresh_token, out token_type, out expires_in);
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string refresh_token, out string token_type, out int expires_in)
{
string authorizationCode = GoogleOAuthHelper.GetAuthorizationCode(user, GoogleOAuthHelper.SCOPE, GoogleOAuthHelper.REDIRECT_URI, GoogleOAuthHelper.REDIRECT_TYPE);
GoogleOAuthHelper.GetAccessToken(authorizationCode, user, out access_token, out token_type, out expires_in, out refresh_token);
}
internal static void GetAccessToken(string authorizationCode, TestUser user, out string access_token, out string token_type, out int expires_in, out string refresh_token)
{
GetAccessToken(authorizationCode, (GoogleUser)user, out access_token, out token_type, out expires_in, out refresh_token);
}
internal static void GetAccessToken(string authorizationCode, GoogleUser user, out string access_token, out string token_type, out int expires_in, out string refresh_token)
{
access_token = null;
token_type = null;
expires_in = 0;
refresh_token = null;
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(TOKEN_REQUEST_URL);
request.CookieContainer = new CookieContainer();
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
string encodedParameters = string.Format("client_id={0}&code={1}&client_secret={2}&redirect_uri={3}&grant_type={4}", System.Web.HttpUtility.UrlEncode(user.ClientId), System.Web.HttpUtility.UrlEncode(authorizationCode), System.Web.HttpUtility.UrlEncode(user.ClientSecret), System.Web.HttpUtility.UrlEncode(REDIRECT_URI), System.Web.HttpUtility.UrlEncode(GrantTypes.authorization_code.ToString()));
byte[] requestData = Encoding.UTF8.GetBytes(encodedParameters);
request.ContentLength = requestData.Length;
if (requestData.Length > 0)
using (Stream stream = request.GetRequestStream())
stream.Write(requestData, 0, requestData.Length);
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
string responseText = null;
using (TextReader reader = new StreamReader(response.GetResponseStream(), Encoding.ASCII))
responseText = reader.ReadToEnd();
foreach (string sPair in responseText.Replace("{", "").Replace("}", "").Replace("\"", "").Split(new string[] { ",\n" }, StringSplitOptions.None))
{
string[] pair = sPair.Split(':');
string name = pair[0].Trim().ToLower();
string value = System.Web.HttpUtility.UrlDecode(pair[1].Trim());
switch (name)
{
case "access_token":
access_token = value;
break;
case "token_type":
token_type = value;
break;
case "expires_in":
expires_in = Convert.ToInt32(value);
break;
case "refresh_token":
refresh_token = value;
break;
}
}
Debug.WriteLine(string.Format("Authorization code: '{0}'", authorizationCode));
Debug.WriteLine(string.Format("Access token: '{0}'", access_token));
Debug.WriteLine(string.Format("Refresh token: '{0}'", refresh_token));
Debug.WriteLine(string.Format("Token type: '{0}'", token_type));
Debug.WriteLine(string.Format("Expires in: '{0}'", expires_in));
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("");
}
internal static string GetAuthorizationCode(TestUser acc, string scope, string redirectUri, string responseType)
{
return GetAuthorizationCode((GoogleUser)acc, scope, redirectUri, responseType);
}
internal static string GetAuthorizationCode(GoogleUser acc, string scope, string redirectUri, string responseType)
{
Debug.WriteLine("");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("-----------OAuth 2.0 authorization information-----------");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine(string.Format("Login: '{0}'", acc.EMail));
string authorizationCode = null;
string error = null;
string approveUrl = string.Format("https://accounts.google.com/o/oauth2/auth?redirect_uri={0}&response_type={1}&client_id={2}&scope={3}", redirectUri, responseType, acc.ClientId, scope);
AutoResetEvent are0 = new AutoResetEvent(false);
Thread t = new Thread(delegate ()
{
bool doEvents = true;
WebBrowser browser = new WebBrowser();
browser.AllowNavigation = true;
browser.DocumentCompleted += delegate (object sender, WebBrowserDocumentCompletedEventArgs e) { doEvents = false; };
Form f = new Form();
f.FormBorderStyle = FormBorderStyle.FixedToolWindow;
f.ShowInTaskbar = false;
f.StartPosition = FormStartPosition.Manual;
f.Location = new System.Drawing.Point(-2000, -2000);
f.Size = new System.Drawing.Size(1, 1);
f.Controls.Add(browser);
f.Load += delegate (object sender, EventArgs e)
{
try
{
browser.Navigate("https://accounts.google.com/Logout");
doEvents = true;
while (doEvents) Application.DoEvents();
browser.Navigate("https://accounts.google.com/ServiceLogin?sacu=1");
doEvents = true;
while (doEvents) Application.DoEvents();
HtmlElement loginForm = browser.Document.Forms["gaia_loginform"];
if (loginForm != null)
{
HtmlElement userName = browser.Document.All["Email"];
userName.SetAttribute("value", acc.EMail);
loginForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
loginForm = browser.Document.Forms["gaia_loginform"];
HtmlElement passwd = browser.Document.All["Passwd"];
passwd.SetAttribute("value", acc.Password);
loginForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
}
else
{
error = "Login form is not found in \n" + browser.Document.Body.InnerHtml;
return;
}
browser.Navigate(approveUrl);
doEvents = true;
while (doEvents) Application.DoEvents();
HtmlElement approveForm = browser.Document.Forms["connect-approve"];
if (approveForm != null)
{
HtmlElement submitAccess = browser.Document.All["submit_access"];
submitAccess.SetAttribute("value", "true");
approveForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
}
else
{
error = "Approve form is not found in \n" + browser.Document.Body.InnerHtml;
return;
}
HtmlElement code = browser.Document.All["code"];
if (code != null)
authorizationCode = code.GetAttribute("value");
else
error = "Authorization code is not found in \n" + browser.Document.Body.InnerHtml;
}
catch (Exception ex)
{
error = ex.Message;
}
finally
{
f.Close();
}
};
Application.Run(f);
are0.Set();
});
t.SetApartmentState(ApartmentState.STA);
t.Start();
are0.WaitOne();
if (error != null)
throw new Exception(error);
return authorizationCode;
}
}
}
C# مخاطبین را از یک حساب جیمیل وارد کنید
در این مرحله ما آماده دسترسی به مخاطبین در یک حساب کاربری جیمیل هستیم. مراحل زیر برای وارد کردن مخاطبین از حساب جیمیل در سی شارپ آمده است.
- یک شی از کلاس GoogleUser ایجاد کنید و آن را با نام، ایمیل، رمز عبور، شناسه مشتری و رمز مشتری مقداردهی کنید.
- دو شی رشته برای ذخیره توکن دسترسی و رفرش توکن ایجاد کنید.
- با روش GoogleOAuthHelper.GetAccessToken (GoogleUser, out string, out string) تماس بگیرید تا به توکنها دسترسی پیدا کنید و بهروزرسانی کنید.
- نمونه ای از کلاس GmailClient را در یک شی IGmailClient دریافت کنید.
- آرایه ای از Contact ایجاد کنید و همه مخاطبین را با استفاده از روش IGmailClient.GetAllContacts() دریافت کنید.
- برای دسترسی به هر مخاطب از طریق آرایه حلقه بزنید.
نمونه کد زیر نحوه وارد کردن مخاطبین از حساب جیمیل در سی شارپ را نشان می دهد.
// یک کاربر گوگل ایجاد کنید
GoogleUser User = new GoogleUser("user", "email address", "password", "clientId", "client secret");
string accessToken;
string refreshToken;
// رمز دسترسی را دریافت کنید
GoogleOAuthHelper.GetAccessToken(User, out accessToken, out refreshToken);
// IGmailclient را دریافت کنید
using (IGmailClient client = GmailClient.GetInstance(accessToken, User.EMail))
{
// دسترسی به مخاطبین
Contact[] contacts = client.GetAllContacts();
foreach (Contact contact in contacts)
Console.WriteLine(contact.DisplayName + ", " + contact.EmailAddresses[0]);
}
وارد کردن مخاطبین Gmail از یک گروه در سی شارپ
همچنین می توانید از یک گروه ایمیل خاص در Gmail به مخاطبین دسترسی داشته باشید. مراحل زیر برای انجام این عملیات آورده شده است.
- یک شی از کلاس GoogleUser ایجاد کنید و آن را با نام، ایمیل، رمز عبور، شناسه مشتری و رمز مشتری مقداردهی کنید.
- دو شی رشته برای ذخیره توکن دسترسی و رفرش توکن ایجاد کنید.
- برای دریافت رمز دسترسی، با روش GoogleOAuthHelper.GetAccessToken (GoogleUser، out string، out string) تماس بگیرید.
- نمونه ای از کلاس GmailClient را در یک شی IGmailClient دریافت کنید.
- همه گروههای ایمیل را با استفاده از روش IGmailClient.GetAllGroups() در یک شی ContactGroupCollection دریافت کنید.
- با استفاده از نام آن به GoogleContactGroup مورد نظر دسترسی پیدا کنید.
- آرایه ای از Contact ایجاد کنید و تمام مخاطبین را با استفاده از روش IGmailClient.GetContactsFromGroup(string) از گروه دریافت کنید.
- برای دسترسی به هر مخاطب از طریق آرایه حلقه بزنید.
نمونه کد زیر نحوه وارد کردن مخاطبین را از یک گروه ایمیل خاص در C# نشان می دهد.
// یک کاربر گوگل ایجاد کنید
GoogleUser User = new GoogleUser("user", "email address", "password", "clientId", "client secret");
string accessToken;
string refreshToken;
// رمز دسترسی را دریافت کنید
GoogleOAuthHelper.GetAccessToken(User, out accessToken, out refreshToken);
// IGmailclient را دریافت کنید
using (IGmailClient client = GmailClient.GetInstance(accessToken, User.EMail))
{
// مخاطبین را از یک گروه خاص واکشی کنید
ContactGroupCollection groups = client.GetAllGroups();
GoogleContactGroup group = null;
foreach (GoogleContactGroup g in groups)
switch (g.Title)
{
case "TestGroup":
group = g;
break;
}
// مخاطبین را از گروه بازیابی کنید
if (group != null)
{
Contact[] contacts2 = client.GetContactsFromGroup(group.Id);
foreach (Contact con in contacts2)
Console.WriteLine(con.DisplayName + "," + con.EmailAddresses[0].ToString());
}
}
C# API برای وارد کردن مخاطبین Gmail - یک مجوز رایگان دریافت کنید
می توانید برای استفاده از Aspose.Email برای دات نت بدون محدودیت ارزیابی، مجوز موقت رایگان دریافت کنید.
نتیجه
در این مقاله با نحوه وارد کردن مخاطبین جیمیل از حساب ها به صورت برنامه نویسی در سی شارپ آشنا شدید. علاوه بر این، نحوه دسترسی به مخاطبین از یک گروه ایمیل خاص در Gmail را دیده اید. علاوه بر این، میتوانید سایر ویژگیهای Aspose.Email را برای داتنت با استفاده از مستندات کاوش کنید. همچنین، میتوانید سؤالات خود را از طریق [تالار گفتمان18 ما بپرسید.