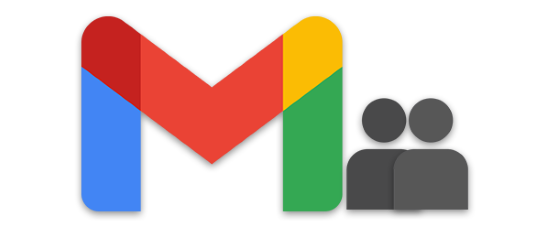
Gmail یکی از برنامههای ایمیل محبوب و پرکاربرد در سراسر جهان است. در کنار مدیریت ایمیل ها، امکان کار با تقویم، مخاطبین، چت و غیره را فراهم می کند و سایر خدمات همکاری را ارائه می دهد. در مقاله قبلی، نحوه وارد کردن مخاطبین از حساب Gmail در یک برنامه NET را مشاهده کرده اید. در این مقاله به نحوه ایجاد، به روز رسانی و حذف مخاطبین در حساب جیمیل با استفاده از C#.NET خواهیم پرداخت.
- C# .NET API برای مدیریت مخاطبین Gmail
- یک مخاطب در جیمیل ایجاد کنید
- یک مخاطب را در جیمیل به روز کنید
- مخاطب را در جیمیل حذف کنید
C# .NET API برای ایجاد، به روز رسانی و حذف مخاطبین Gmail
برای ایجاد و دستکاری مخاطبین در یک حساب Gmail، از Aspose.Email for .NET استفاده خواهیم کرد. این یک API پردازش ایمیل است که به شما امکان می دهد ایمیل ها را دستکاری کنید و با مشتریان ایمیل محبوب کار کنید. می توانید DLL API را دانلود یا با استفاده از دستور زیر از NuGet نصب کنید.
PM> Install-Package Aspose.Email
قبل از شروع کار، باید یک پروژه در کنسول برنامه نویس گوگل ایجاد کنید که به شما امکان می دهد با جیمیل ارتباط برقرار کنید. برای ایجاد یکی، می توانید [این راهنما] را دنبال کنید.
برای دسترسی و دستکاری مخاطبین در حساب جیمیل، باید کدی بنویسیم تا اطلاعات کاربر را مدیریت کرده و احراز هویت را انجام دهیم. برای کاربر جیمیل، ابتدا کلاسی به نام TestUser ایجاد می کنیم و سپس آن را از کلاس GoogleUser به ارث می بریم. در زیر اجرای کامل هر دو کلاس آورده شده است.
using System;
namespace Aspose.Email
{
internal enum GrantTypes
{
authorization_code,
refresh_token
}
public class TestUser
{
internal TestUser(string name, string eMail, string password, string domain)
{
Name = name;
EMail = eMail;
Password = password;
Domain = domain;
}
public readonly string Name;
public readonly string EMail;
public readonly string Password;
public readonly string Domain;
public static bool operator ==(TestUser x, TestUser y)
{
if ((object)x != null)
return x.Equals(y);
if ((object)y != null)
return y.Equals(x);
return true;
}
public static bool operator !=(TestUser x, TestUser y)
{
return !(x == y);
}
public static implicit operator string(TestUser user)
{
return user == null ? null : user.Name;
}
public override int GetHashCode()
{
return ToString().GetHashCode();
}
public override bool Equals(object obj)
{
return obj != null && obj is TestUser && this.ToString().Equals(obj.ToString(), StringComparison.OrdinalIgnoreCase);
}
public override string ToString()
{
return string.IsNullOrEmpty(Domain) ? Name : string.Format("{0}/{1}", Domain, Name);
}
}
public class GoogleUser : TestUser
{
public GoogleUser(string name, string eMail, string password)
: this(name, eMail, password, null, null, null)
{ }
public GoogleUser(string name, string eMail, string password, string clientId, string clientSecret)
: this(name, eMail, password, clientId, clientSecret, null)
{ }
public GoogleUser(string name, string eMail, string password, string clientId, string clientSecret, string refreshToken)
: base(name, eMail, password, "gmail.com")
{
ClientId = clientId;
ClientSecret = clientSecret;
RefreshToken = refreshToken;
}
public readonly string ClientId;
public readonly string ClientSecret;
public readonly string RefreshToken;
}
}
اکنون باید یک کلاس کمکی ایجاد کنیم که از احراز هویت یک حساب جیمیل مراقبت کند. نام این کلاس را GoogleOAuthHelper میگذاریم. در ادامه پیاده سازی کامل این کلاس ارائه شده است.
using System;
using System.Diagnostics;
using System.IO;
using System.Net;
using System.Text;
using System.Threading;
using System.Windows.Forms;
namespace Aspose.Email
{
internal class GoogleOAuthHelper
{
public const string TOKEN_REQUEST_URL = "https://accounts.google.com/o/oauth2/token";
public const string SCOPE = "https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fcalendar" + "+" + "https%3A%2F%2Fwww.google.com%2Fm8%2Ffeeds%2F" + "+" + "https%3A%2F%2Fmail.google.com%2F"; // IMAP & SMTP
public const string REDIRECT_URI = "urn:ietf:wg:oauth:2.0:oob";
public const string REDIRECT_TYPE = "code";
internal static string GetAccessToken(TestUser user)
{
return GetAccessToken((GoogleUser)user);
}
internal static string GetAccessToken(GoogleUser user)
{
string access_token;
string token_type;
int expires_in;
GetAccessToken(user, out access_token, out token_type, out expires_in);
return access_token;
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string token_type, out int expires_in)
{
access_token = null;
token_type = null;
expires_in = 0;
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(TOKEN_REQUEST_URL);
request.CookieContainer = new CookieContainer();
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
string encodedParameters = string.Format("client_id={0}&client_secret={1}&refresh_token={2}&grant_type={3}",
System.Web.HttpUtility.UrlEncode(user.ClientId), System.Web.HttpUtility.UrlEncode(user.ClientSecret), System.Web.HttpUtility.UrlEncode(user.RefreshToken), System.Web.HttpUtility.UrlEncode(GrantTypes.refresh_token.ToString()));
byte[] requestData = Encoding.UTF8.GetBytes(encodedParameters);
request.ContentLength = requestData.Length;
if (requestData.Length > 0)
using (Stream stream = request.GetRequestStream())
stream.Write(requestData, 0, requestData.Length);
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
string responseText = null;
using (TextReader reader = new StreamReader(response.GetResponseStream(), Encoding.ASCII))
responseText = reader.ReadToEnd();
foreach (string sPair in responseText.Replace("{", "").Replace("}", "").Replace("\"", "").Split(new string[] { ",\n" }, StringSplitOptions.None))
{
string[] pair = sPair.Split(':');
string name = pair[0].Trim().ToLower();
string value = System.Web.HttpUtility.UrlDecode(pair[1].Trim());
switch (name)
{
case "access_token":
access_token = value;
break;
case "token_type":
token_type = value;
break;
case "expires_in":
expires_in = Convert.ToInt32(value);
break;
}
}
Debug.WriteLine("");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("-----------OAuth 2.0 authorization information-----------");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine(string.Format("Login: '{0}'", user.EMail));
Debug.WriteLine(string.Format("Access token: '{0}'", access_token));
Debug.WriteLine(string.Format("Token type: '{0}'", token_type));
Debug.WriteLine(string.Format("Expires in: '{0}'", expires_in));
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("");
}
internal static void GetAccessToken(TestUser user, out string access_token, out string refresh_token)
{
GetAccessToken((GoogleUser)user, out access_token, out refresh_token);
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string refresh_token)
{
string token_type;
int expires_in;
GoogleOAuthHelper.GetAccessToken(user, out access_token, out refresh_token, out token_type, out expires_in);
}
internal static void GetAccessToken(TestUser user, out string access_token, out string refresh_token, out string token_type, out int expires_in)
{
GetAccessToken((GoogleUser)user, out access_token, out refresh_token, out token_type, out expires_in);
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string refresh_token, out string token_type, out int expires_in)
{
string authorizationCode = GoogleOAuthHelper.GetAuthorizationCode(user, GoogleOAuthHelper.SCOPE, GoogleOAuthHelper.REDIRECT_URI, GoogleOAuthHelper.REDIRECT_TYPE);
GoogleOAuthHelper.GetAccessToken(authorizationCode, user, out access_token, out token_type, out expires_in, out refresh_token);
}
internal static void GetAccessToken(string authorizationCode, TestUser user, out string access_token, out string token_type, out int expires_in, out string refresh_token)
{
GetAccessToken(authorizationCode, (GoogleUser)user, out access_token, out token_type, out expires_in, out refresh_token);
}
internal static void GetAccessToken(string authorizationCode, GoogleUser user, out string access_token, out string token_type, out int expires_in, out string refresh_token)
{
access_token = null;
token_type = null;
expires_in = 0;
refresh_token = null;
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(TOKEN_REQUEST_URL);
request.CookieContainer = new CookieContainer();
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
string encodedParameters = string.Format("client_id={0}&code={1}&client_secret={2}&redirect_uri={3}&grant_type={4}", System.Web.HttpUtility.UrlEncode(user.ClientId), System.Web.HttpUtility.UrlEncode(authorizationCode), System.Web.HttpUtility.UrlEncode(user.ClientSecret), System.Web.HttpUtility.UrlEncode(REDIRECT_URI), System.Web.HttpUtility.UrlEncode(GrantTypes.authorization_code.ToString()));
byte[] requestData = Encoding.UTF8.GetBytes(encodedParameters);
request.ContentLength = requestData.Length;
if (requestData.Length > 0)
using (Stream stream = request.GetRequestStream())
stream.Write(requestData, 0, requestData.Length);
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
string responseText = null;
using (TextReader reader = new StreamReader(response.GetResponseStream(), Encoding.ASCII))
responseText = reader.ReadToEnd();
foreach (string sPair in responseText.Replace("{", "").Replace("}", "").Replace("\"", "").Split(new string[] { ",\n" }, StringSplitOptions.None))
{
string[] pair = sPair.Split(':');
string name = pair[0].Trim().ToLower();
string value = System.Web.HttpUtility.UrlDecode(pair[1].Trim());
switch (name)
{
case "access_token":
access_token = value;
break;
case "token_type":
token_type = value;
break;
case "expires_in":
expires_in = Convert.ToInt32(value);
break;
case "refresh_token":
refresh_token = value;
break;
}
}
Debug.WriteLine(string.Format("Authorization code: '{0}'", authorizationCode));
Debug.WriteLine(string.Format("Access token: '{0}'", access_token));
Debug.WriteLine(string.Format("Refresh token: '{0}'", refresh_token));
Debug.WriteLine(string.Format("Token type: '{0}'", token_type));
Debug.WriteLine(string.Format("Expires in: '{0}'", expires_in));
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("");
}
internal static string GetAuthorizationCode(TestUser acc, string scope, string redirectUri, string responseType)
{
return GetAuthorizationCode((GoogleUser)acc, scope, redirectUri, responseType);
}
internal static string GetAuthorizationCode(GoogleUser acc, string scope, string redirectUri, string responseType)
{
Debug.WriteLine("");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("-----------OAuth 2.0 authorization information-----------");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine(string.Format("Login: '{0}'", acc.EMail));
string authorizationCode = null;
string error = null;
string approveUrl = string.Format("https://accounts.google.com/o/oauth2/auth?redirect_uri={0}&response_type={1}&client_id={2}&scope={3}", redirectUri, responseType, acc.ClientId, scope);
AutoResetEvent are0 = new AutoResetEvent(false);
Thread t = new Thread(delegate ()
{
bool doEvents = true;
WebBrowser browser = new WebBrowser();
browser.AllowNavigation = true;
browser.DocumentCompleted += delegate (object sender, WebBrowserDocumentCompletedEventArgs e) { doEvents = false; };
Form f = new Form();
f.FormBorderStyle = FormBorderStyle.FixedToolWindow;
f.ShowInTaskbar = false;
f.StartPosition = FormStartPosition.Manual;
f.Location = new System.Drawing.Point(-2000, -2000);
f.Size = new System.Drawing.Size(1, 1);
f.Controls.Add(browser);
f.Load += delegate (object sender, EventArgs e)
{
try
{
browser.Navigate("https://accounts.google.com/Logout");
doEvents = true;
while (doEvents) Application.DoEvents();
browser.Navigate("https://accounts.google.com/ServiceLogin?sacu=1");
doEvents = true;
while (doEvents) Application.DoEvents();
HtmlElement loginForm = browser.Document.Forms["gaia_loginform"];
if (loginForm != null)
{
HtmlElement userName = browser.Document.All["Email"];
userName.SetAttribute("value", acc.EMail);
loginForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
loginForm = browser.Document.Forms["gaia_loginform"];
HtmlElement passwd = browser.Document.All["Passwd"];
passwd.SetAttribute("value", acc.Password);
loginForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
}
else
{
error = "Login form is not found in \n" + browser.Document.Body.InnerHtml;
return;
}
browser.Navigate(approveUrl);
doEvents = true;
while (doEvents) Application.DoEvents();
HtmlElement approveForm = browser.Document.Forms["connect-approve"];
if (approveForm != null)
{
HtmlElement submitAccess = browser.Document.All["submit_access"];
submitAccess.SetAttribute("value", "true");
approveForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
}
else
{
error = "Approve form is not found in \n" + browser.Document.Body.InnerHtml;
return;
}
HtmlElement code = browser.Document.All["code"];
if (code != null)
authorizationCode = code.GetAttribute("value");
else
error = "Authorization code is not found in \n" + browser.Document.Body.InnerHtml;
}
catch (Exception ex)
{
error = ex.Message;
}
finally
{
f.Close();
}
};
Application.Run(f);
are0.Set();
});
t.SetApartmentState(ApartmentState.STA);
t.Start();
are0.WaitOne();
if (error != null)
throw new Exception(error);
return authorizationCode;
}
}
}
ایجاد مخاطب در جیمیل در سی شارپ
مراحل زیر برای ایجاد مخاطب در جیمیل در سی شارپ آمده است.
- ابتدا یک کاربر Google ایجاد کنید، یک نشانه دسترسی دریافت کنید و یک شی IGmailClient را مقداردهی اولیه کنید.
- سپس، یک شی از کلاس Contact ایجاد کنید.
- ویژگی های مخاطب مانند نام، پیشوند، حرفه و غیره را تنظیم کنید.
- برای تنظیم آدرس پستی، نمونه ای از PostalAddress ایجاد کنید و ویژگی های آن را تنظیم کنید.
- آدرس جدید ایجاد شده را با استفاده از روش Contact.PhysicalAddresses.Add(PostalAddress) به مجموعه اضافه کنید.
- جزئیات شماره تلفن را با استفاده از کلاس PhoneNumber تنظیم کنید.
- جزئیات شماره تلفن را با استفاده از روش Contact.PhoneNumbers.Add(PhoneNumber) به مجموعه اضافه کنید.
- یک نمونه از کلاس EmailAddress ایجاد کنید، آدرس ایمیل را تنظیم کنید و آن را به مخاطب اختصاص دهید.
- در نهایت، روش IGmailClient.CreateContact(Contact) را برای ایجاد مخاطب Gmail فراخوانی کنید.
نمونه کد زیر نحوه ایجاد مخاطب در جیمیل در سی شارپ را نشان می دهد.
// کاربر گوگل را راه اندازی کنید
GoogleUser User = new GoogleUser("user", "email address", "password", "clientId", "client secret");
string accessToken;
string refreshToken;
// رمز دسترسی را دریافت کنید
GoogleOAuthHelper.GetAccessToken(User, out accessToken, out refreshToken);
// کلاینت جیمیل را دریافت کنید
IGmailClient client = GmailClient.GetInstance(accessToken, User.EMail);
// یک مخاطب ایجاد کنید
Contact contact = new Contact();
contact.Prefix = "Prefix";
contact.GivenName = "GivenName";
contact.Surname = "Surname";
contact.MiddleName = "MiddleName";
contact.DisplayName = "Test User 1";
contact.Suffix = "Suffix";
contact.JobTitle = "JobTitle";
contact.DepartmentName = "DepartmentName";
contact.CompanyName = "CompanyName";
contact.Profession = "Profession";
contact.Notes = "Notes";
// آدرس پستی را تنظیم کنید
PostalAddress address = new PostalAddress();
address.Category = PostalAddressCategory.Work;
address.Address = "Address";
address.Street = "Street";
address.PostOfficeBox = "PostOfficeBox";
address.City = "City";
address.StateOrProvince = "StateOrProvince";
address.PostalCode = "PostalCode";
address.Country = "Country";
contact.PhysicalAddresses.Add(address);
// تنظیم شماره تلفن
PhoneNumber pnWork = new PhoneNumber();
pnWork.Number = "323423423423";
pnWork.Category = PhoneNumberCategory.Work;
contact.PhoneNumbers.Add(pnWork);
PhoneNumber pnHome = new PhoneNumber();
pnHome.Number = "323423423423";
pnHome.Category = PhoneNumberCategory.Home;
contact.PhoneNumbers.Add(pnHome);
PhoneNumber pnMobile = new PhoneNumber();
pnMobile.Number = "323423423423";
pnMobile.Category = PhoneNumberCategory.Mobile;
contact.PhoneNumbers.Add(pnMobile);
// ویژگی های دیگر را تنظیم کنید
contact.Urls.Blog = "Blog.ru";
contact.Urls.BusinessHomePage = "BusinessHomePage.ru";
contact.Urls.HomePage = "HomePage.ru";
contact.Urls.Profile = "Profile.ru";
contact.Events.Birthday = DateTime.Now.AddYears(-30);
contact.Events.Anniversary = DateTime.Now.AddYears(-10);
contact.InstantMessengers.AIM = "AIM";
contact.InstantMessengers.GoogleTalk = "GoogleTalk";
contact.InstantMessengers.ICQ = "ICQ";
contact.InstantMessengers.Jabber = "Jabber";
contact.InstantMessengers.MSN = "MSN";
contact.InstantMessengers.QQ = "QQ";
contact.InstantMessengers.Skype = "Skype";
contact.InstantMessengers.Yahoo = "Yahoo";
contact.AssociatedPersons.Spouse = "Spouse";
contact.AssociatedPersons.Sister = "Sister";
contact.AssociatedPersons.Relative = "Relative";
contact.AssociatedPersons.ReferredBy = "ReferredBy";
contact.AssociatedPersons.Partner = "Partner";
contact.AssociatedPersons.Parent = "Parent";
contact.AssociatedPersons.Mother = "Mother";
contact.AssociatedPersons.Manager = "Manager";
// آدرس ایمیل را تنظیم کنید
EmailAddress eAddress = new EmailAddress();
eAddress.Address = "email@gmail.com";
contact.EmailAddresses.Add(eAddress);
// ایجاد مخاطب در جیمیل
string contactUri = client.CreateContact(contact);
به روز رسانی مخاطب در جیمیل در سی شارپ
شما همچنین می توانید جزئیات یک مخاطب جیمیل را پس از دسترسی به آن به روز کنید. مراحل زیر برای به روز رسانی یک مخاطب در حساب جیمیل در سی شارپ است.
- ابتدا یک کاربر Google ایجاد کنید، یک نشانه دسترسی دریافت کنید و یک شی IGmailClient را مقداردهی اولیه کنید.
- با استفاده از روش IGmailClient.GetAllContacts() مخاطبین را در یک آرایه دریافت کنید.
- تماس مورد نیاز را از آرایه در یک شی Contact واکشی کنید.
- جزئیات تماس را بهروزرسانی کنید و با روش IGmailClient.UpdateContact(contact) تماس بگیرید.
نمونه کد زیر نحوه به روز رسانی یک مخاطب در جیمیل در سی شارپ را نشان می دهد.
// کاربر گوگل را راه اندازی کنید
GoogleUser User = new GoogleUser("user", "email address", "password", "clientId", "client secret");
string accessToken;
string refreshToken;
// رمز دسترسی را دریافت کنید
GoogleOAuthHelper.GetAccessToken(User, out accessToken, out refreshToken);
// IGmailClient را دریافت کنید
using (IGmailClient client = GmailClient.GetInstance(accessToken, User.EMail))
{
// همه مخاطبین را دریافت کنید
Contact[] contacts = client.GetAllContacts();
Contact contact = contacts[0];
contact.JobTitle = "Manager IT";
contact.DepartmentName = "Customer Support";
contact.CompanyName = "Aspose";
contact.Profession = "Software Developer";
// به روز رسانی مخاطب
client.UpdateContact(contact);
}
حذف مخاطب در جیمیل در سی شارپ
در نهایت، بیایید ببینیم چگونه یک مخاطب جیمیل را با استفاده از سی شارپ حذف کنیم. مراحل زیر برای انجام این عملیات آورده شده است.
- ابتدا یک کاربر Google ایجاد کنید، یک نشانه دسترسی دریافت کنید و یک شی IGmailClient را مقداردهی اولیه کنید.
- با استفاده از روش IGmailClient.GetAllContacts() مخاطبین را در یک آرایه دریافت کنید.
- مخاطب مورد نظر را از آرایه در یک شی Contact فیلتر کنید.
- در نهایت، با روش IGmailClient.DeleteContact(Contact.Id.GoogleId) تماس بگیرید تا مخاطب را حذف کنید.
نمونه کد زیر نحوه حذف یک مخاطب در جیمیل در سی شارپ را نشان می دهد.
// کاربر گوگل را راه اندازی کنید
GoogleUser User = new GoogleUser("user", "email address", "password", "clientId", "client secret");
string accessToken;
string refreshToken;
// رمز دسترسی را دریافت کنید
GoogleOAuthHelper.GetAccessToken(User, out accessToken, out refreshToken);
// IGmailClient را دریافت کنید
using (IGmailClient client = GmailClient.GetInstance(accessToken, User.EMail))
{
// همه مخاطبین را دریافت کنید
Contact[] contacts = client.GetAllContacts();
Contact contact = contacts[0];
// مخاطب را حذف کنید
client.DeleteContact(contact.Id.GoogleId);
}
مجوز API رایگان دریافت کنید
شما می توانید از Aspose.Email برای دات نت بدون محدودیت ارزیابی با استفاده از مجوز موقت رایگان استفاده کنید.
نتیجه
در این مقاله با نحوه ایجاد و به روز رسانی مخاطبین در اکانت جیمیل در سی شارپ دات نت آشنا شدید. علاوه بر این، نحوه حذف یک مخاطب جیمیل را به صورت برنامهریزی مشاهده کردهاید. علاوه بر این، برای بررسی سایر ویژگیهای Aspose.Email برای داتنت میتوانید از مستندات دیدن کنید. در صورت داشتن هر گونه سوال، می توانید به [تالار گفتمان25 ما ارسال کنید.