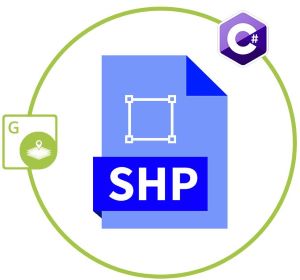
The Shapefile format is a geospatial vector data format used to display geographical information. We can store the location, geometry, and attribution of point, line, and polygon features in Shapefile. In this article, we will learn how to create and read Shapefile in C#.
This article shall cover the following topics:
- What is a Shapefile
- How to Use a Shapefile
- C# API to Create and Read Shapefile – .NET GIS Library
- Create a Shapefile using C#
- Add Features to an Existing ShapeFile in C#
- Read a Shapefile using C#
What is a Shapefile
A shapefile represents Geospatial information in the form of vector data to be used by GIS applications. It was developed by ESRI as an open specification to facilitate interoperability between ESRI and other software products. It contains the geometric data that draws points, lines or polygons on a map and the data attributes. Shapefiles can be directly read by several GIS software programs such as ArcGIS and QGIS.
How to Use a Shapefile
A standalone shapefile (.shp) cannot be used by software applications. However, a valid shapefile that can be used in GIS software should contain the following additional mandatory files:
- Shape index file (.shx) - a positional index of the feature geometry;
- dBase attribute file (.dbf) - a dBASE file that stores all the attributes of the shapes;
- Code page file (.cpg) - the file to identify the character encoding.
C# API to Create and Read Shapefile – .NET GIS Library
To create or read shapefiles, we will be using the Aspose.GIS for .NET API. It allows to render maps, create, read, and convert geographic data without additional software. It supports working with shapefiles along with several other supported file formats.
The VectorLayer class of the API represents a vector layer. It offers various properties and methods to work with a collection of geographic features stored in a file. The Create() method of this class allows the creation of supported vector layers. The Drivers class provides drivers for all supported formats. The Feature class of the API represents a geographic feature composed of geometry and user-defined attributes.
Please either download the DLL of the API or install it using NuGet.
PM> Install-Package Aspose.GIS
Create Shapefile using C#
We can easily create a shapefile programmatically by following the steps given below:
- Firstly, create a layer using the VectorLayer.Create() method.
- Next, add FeatureAttributes to the layer’s collection of attributes.
- Then, create an instance of the Feature class using the ConstructFeature() method.
- After that, set values of different attributes.
- Finally, add the feature using the Add() method.
The following code sample shows how to create a shapefile in C#:
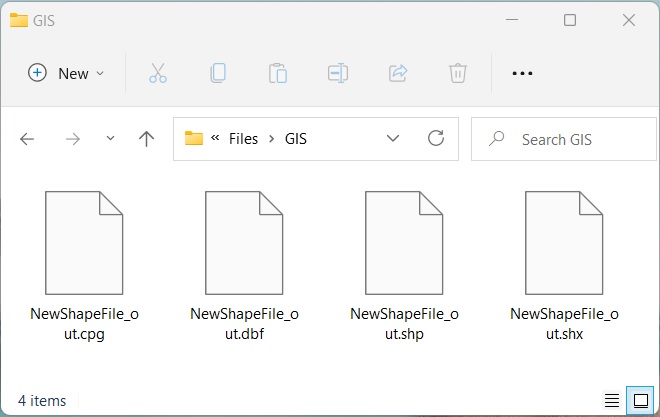
Create Shapefile using C#.
Add Features to Existing ShapeFile using C#
We can also add new features to an existing shapefile by following the steps given below:
- Firstly, load a shapefile using the Drivers.Shapefile.EditLayer() method.
- Next, create an instance of the Feature class using the ConstructFeature() method.
- After that, set values of different attributes.
- Finally, add the feature using the Add() method.
The following code sample shows how to add features to an existing shapefile using C#.
Read a Shapefile using C#
We can read attributes from a shapefile by following the steps given below:
- Firstly, load a shapefile using the Drivers.Shapefile.OpenLayer() method.
- Loop through each Feature in the layer.
- Loop through attributes and show attribute details.
- Finally, check for Point geometry and read points.
The following code sample shows how to read a shapefile using C#.
name : John
age : 23
dob : 1982-02-05T16:30:00
POINT (33.97 -118.25) X: 33.97 Y: -118.25
---------------------
name : Mary
age : 54
dob : 1984-12-15T15:30:00
POINT (35.81 -96.28) X: 35.81 Y: -96.28
---------------------
name : Alex
age : 25
dob : 04/15/1989 15:30:00
POINT (34.81 -92.28) X: 34.81 Y: -92.28
Get a Free License
You can get a free temporary license to try the library without evaluation limitations.
Conclusion
In this article, we have learned how to
- create a new shapefile programmatically;
- add new features to the shapefile;
- edit shapefile layer;
- open the shapefile layer and read the attributes using C#.
Besides, you can explore how to work with several other GIS file formats and learn more about Aspose.GIS for .NET API using the documentation. In case of any ambiguity, please feel free to contact us on our forum.