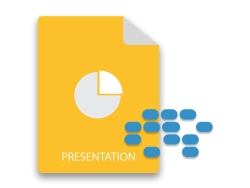
קובצי PowerPoint מכילים מידע נוסף המכונה מאפייני מסמך. מאפיינים אלו משמשים לזיהוי המצגות, הכוללות מחבר, כותרת, מילות מפתח, נושא וכו’. במאמר זה תלמדו כיצד להוסיף, לגשת או לשנות את מאפייני המסמך ב-PowerPoint PPT ב-Java.
- Java API לגישה/שינוי מאפיינים בקבצי PowerPoint
- סוגי מאפיינים במצגות PowerPoint
- גישה למאפיינים מובנים במצגות PowerPoint
- שנה מאפיינים מובנים במצגות PowerPoint
- הוסף מאפיינים מותאמים אישית במצגות PowerPoint
- גישה למאפיינים מותאמים אישית במצגות PowerPoint
- שנה מאפיינים מותאמים אישית במצגות PowerPoint
Java API עבור מאפייני מסמך ב-PowerPoint PPT
כדי לגשת או לשנות את מאפייני המסמך במצגות PowerPoint, נשתמש ב-Aspose.Slides עבור Java. ה-API מאפשר לך ליצור ולתפעל מסמכי PowerPoint ו-OpenOffice. זה זמין בתור JAR להורדה כמו גם ב-Maven. אתה יכול להתקין אותו באמצעות התצורות הבאות של Maven.
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>http://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-slides</artifactId>
<version>21.8</version>
<classifier>jdk16</classifier>
</dependency>
סוגי מאפייני מסמכים במצגות PowerPoint
ישנם שני סוגים של מאפייני מסמך בקובצי PowerPoint: מובנים ומותאמים אישית. הראשון מספק מידע כללי על המצגות כגון כותרת, מחבר, נושא וכו’. בעוד שהאחרון משמש להוספת מאפיינים המוגדרים על ידי המשתמש. בסעיפים שלהלן, תראה כיצד להוסיף, לגשת ולשנות מאפייני מסמך מובנים ומותאמים אישית במצגות PowerPoint.
גישה למאפיינים מובנים במצגות PowerPoint באמצעות Java
להלן השלבים לגישה למאפיינים המובנים במצגות PowerPoint באמצעות Java.
- ראשית, טען את מצגת PowerPoint באמצעות מחלקה מצגת.
- לאחר מכן, גש למאפיינים המובנים באובייקט IDocumentProperties באמצעות שיטת Presentation.getDocumentProperties().
- קרא כל מאפיין מובנה במצגת באמצעות אובייקט IDocumentProperties, כגון, IDocumentProperties.getAuthor().
דוגמת הקוד הבאה מראה כיצד לגשת למאפיינים מובנים במצגות PowerPoint.
// טען מצגת
Presentation pres = new Presentation("Presentation.pptx");
try {
// צור הפניה לאובייקט IDocumentProperties המשויך למצגת
IDocumentProperties dp = pres.getDocumentProperties();
// הצג את המאפיינים המובנים
System.out.println("Category : " + dp.getCategory());
System.out.println("Current Status : " + dp.getContentStatus());
System.out.println("Creation Date : " + dp.getCreatedTime());
System.out.println("Author : " + dp.getAuthor());
System.out.println("Description : " + dp.getComments());
System.out.println("KeyWords : " + dp.getKeywords());
System.out.println("Last Modified By : " + dp.getLastSavedBy());
System.out.println("Supervisor : " + dp.getManager());
System.out.println("Modified Date : " + dp.getLastSavedTime());
System.out.println("Presentation Format : " + dp.getPresentationFormat());
System.out.println("Last Print Date : " + dp.getLastPrinted());
System.out.println("Is Shared between producers : " + dp.getSharedDoc());
System.out.println("Subject : " + dp.getSubject());
System.out.println("Title : " + dp.getTitle());
} finally {
if (pres != null) pres.dispose();
}
שנה מאפיינים מובנים ב-PowerPoint PPT באמצעות Java
להלן השלבים לשינוי הערכים של המאפיינים המובנים במצגות PowerPoint באמצעות Java.
- ראשית, טען את מצגת PowerPoint באמצעות מחלקה מצגת.
- לאחר מכן, קבל הפניה למאפיינים המובנים באובייקט IDocumentProperties באמצעות שיטת Presentation.getDocumentProperties().
- שנה את המאפיין המובנה הרצוי במצגת באמצעות אובייקט IDocumentProperties, כגון, IDocumentProperties.setAuthor().
- לבסוף, שמור את המצגת בשיטת Presentation.save(String, SaveFormat).
דוגמת הקוד הבאה מראה כיצד לשנות את המאפיינים המובנים במצגות PowerPoint.
// טען מצגת
Presentation pres = new Presentation("Presentation.pptx");
try {
// צור הפניה לאובייקט IDocumentProperties המשויך למצגת
IDocumentProperties dp = pres.getDocumentProperties();
// הגדר את המאפיינים המובנים
dp.setAuthor("Aspose.Slides for Java");
dp.setTitle("Modifying Presentation Properties");
dp.setSubject("Aspose Subject");
dp.setComments("Aspose Description");
dp.setManager("Aspose Manager");
// שמור את המצגת שלך בקובץ
pres.save("DocProps.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
הוסף מאפיינים מותאמים אישית ב-PowerPoint PPT באמצעות Java
להלן השלבים להוספת מאפיינים מותאמים אישית במצגת PowerPoint באמצעות Java.
- ראשית, טען את מצגת PowerPoint באמצעות מחלקה מצגת.
- לאחר מכן, קבל הפניה למאפייני המסמך באובייקט IDocumentProperties באמצעות שיטת Presentation.getDocumentProperties().
- הוסף מאפיין מותאם אישית על ידי הגדרת המפתח והערך שלו, למשל IDocumentPropertiesd.setItem(“New Custom”, 12).
- לבסוף, שמור את המצגת בשיטת Presentation.save(String, SaveFormat).
דוגמת הקוד הבאה מראה כיצד להוסיף מאפיינים מותאמים אישית במצגת PowerPoint.
// טען מצגת
Presentation pres = new Presentation("Presentation.pptx");
try {
// קבל מאפייני מסמך
IDocumentProperties dProps = pres.getDocumentProperties();
// הוסף מאפיינים מותאמים אישית
dProps.set_Item("New Custom", 12);
dProps.set_Item("My Name", "Mudassir");
dProps.set_Item("Custom", 124);
// קבל שם נכס באינדקס מסוים
String getPropertyName = dProps.getCustomPropertyName(2);
// כדי להסיר את הנכס שנבחר
//dProps.removeCustomProperty(getPropertyName);
// שמור מצגת
pres.save("CustomDemo.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
גישה למאפיינים מותאמים אישית ב-PPT באמצעות Java
השלבים הבאים מדגימים כיצד לגשת למאפיינים המותאמים אישית במצגת PowerPoint באמצעות Java.
- ראשית, טען את מצגת PowerPoint באמצעות מחלקה מצגת.
- לאחר מכן, קבל הפניה למאפייני המסמך באובייקט IDocumentProperties באמצעות שיטת Presentation.getDocumentProperties().
- גש לכל מאפיין מותאם אישית באמצעות שיטת IDocumentProperties.getCustomPropertyName(int index) בלולאה.
דוגמת הקוד הבאה מראה כיצד לגשת למאפיינים מותאמים אישית במצגת PowerPoint.
// טען מצגת
Presentation pres = new Presentation("Presentation.pptx");
try {
// צור הפניה לאובייקט DocumentProperties המשויך למצגת
IDocumentProperties dp = pres.getDocumentProperties();
// גישה ושנה מאפיינים מותאמים אישית
for (int i = 0; i < dp.getCountOfCustomProperties(); i++) {
// הצגת שמות וערכים של נכסים מותאמים אישית
System.out.println("Custom Property Name : " + dp.getCustomPropertyName(i));
System.out.println("Custom Property Value : " + dp.get_Item(dp.getCustomPropertyName(i)));
}
// שמור את המצגת שלך בקובץ
pres.save("CustomDemoModified.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
שנה מאפיינים מותאמים אישית ב-PowerPoint באמצעות Java
להלן השלבים לשינוי המאפיינים המותאמים אישית במצגת PowerPoint.
- ראשית, טען את מצגת PowerPoint באמצעות מחלקה מצגת.
- לאחר מכן, קבל הפניה למאפייני המסמך באובייקט IDocumentProperties באמצעות שיטת Presentation.getDocumentProperties().
- גש לכל מאפיין מותאם אישית באמצעות שיטת IDocumentProperties.getCustomPropertyName(int index) בלולאה.
- הגדר את הערך של מאפיין על ידי ציון המפתח שלו.
- לבסוף, שמור את המצגת בשיטת Presentation.save(String, SaveFormat).
דוגמת הקוד הבאה מראה כיצד לשנות מאפיין מותאם אישית במצגת PowerPoint.
// טען מצגת
Presentation pres = new Presentation("Presentation.pptx");
try {
// צור הפניה לאובייקט DocumentProperties המשויך למצגת
IDocumentProperties dp = pres.getDocumentProperties();
// גישה ושנה מאפיינים מותאמים אישית
for (int i = 0; i < dp.getCountOfCustomProperties(); i++) {
// שנה ערכים של מאפיינים מותאמים אישית
dp.set_Item(dp.getCustomPropertyName(i), "New Value " + (i + 1));
}
// שמור את המצגת שלך בקובץ
pres.save("CustomDemoModified.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
מניפולציה של מאפייני PowerPoint PPT - קבל רישיון חינם
ניתן לבצע מניפולציות במאפייני מסמך במצגות PowerPoint ללא מגבלות הערכה על ידי בקשת רישיון זמני.
הדגמה מקוונת
נסה כלי מקוון מבוסס Aspose.Slides כדי להציג ולערוך מאפייני מסמך במצגות.
סיכום
במאמר זה, למדת כיצד לגשת ולשנות את מאפייני המסמך במצגות PowerPoint ב-Java. כיסינו במפורש את המניפולציה של מאפייני מסמך מובנים כמו גם בהתאמה אישית במצגות. בנוסף, אתה יכול לבקר בתיעוד כדי לחקור תכונות אחרות של Aspose.Slides עבור Java. כמו כן, אתה יכול לפרסם את השאילתות שלך בפורום שלנו.