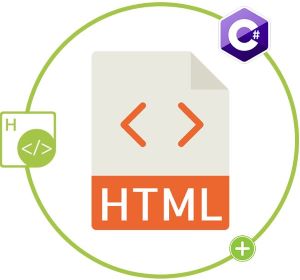
If you’re a C# programmer looking for an efficient way to work with HTML files, then this article is for you. We’ll explain the basics of how C# works with HTML files, from creating them from scratch to reading and editing existing documents. In this article, we will learn how to create, read, and edit HTML files in C#.
HTML (Hyper Text Markup Language) is a language used to create websites and web pages. C#, on the other hand, is an object-oriented programming language used to build applications. An HTML file contains markup tags used to format the structure of a webpage for display in browsers. We can easily manipulate HTML files programmatically in .NET applications. In this article, we’ll explore how to use C# to read, write, and edit HTML files. We’ll look at ways to parse HTML code for specific elements and create new elements from scratch or edit existing ones. Let’s begin!
The following topics shall be covered in this article:
- C# API to create, read, and edit HTML files
- Create an HTML file
- Read an HTML file
- Edit an HTML file
- Add Raw HTML content in C#
C# API to Create, Read, and Edit HTML Files
To create, read, and edit HTML files, we will be using the Aspose.HTML for .NET API. It is an advanced HTML processing API that allows the creation, modification, and extraction of data from HTML documents. It also allows converting and rendering HTML documents without any external software.
The HTMLDocument class of the API represents an HTML document or the HTML page that we see in the browser. The CreateElement() method of this class creates the HTML element specified by tagName. The CreateTextNode(string) method creates a Text node given the specified string. The AppendChild() method of the Node interface adds a node to the end of the list of children of a specified parent node. The Save() method save the output file. You may also read more about DOM namespace in the documentation.
Please either download the DLL of the API or install it using NuGet.
PM> Install-Package Aspose.Html
Info: Other Aspose APIs, especially Aspose.Slides for .NET, may also allow you to edit HTMLs, merge HTML files, convert HTML to JPG, PDF, XML, TIFF, and others.
Create an HTML File in C#
We can easily create an HTML file by following the steps given below:
- Firstly, create an instance of the HTMLDocument class.
- Next, call the CreateElement() method to create elements such as heading, paragraph, text, etc.
- Then, call the CreateTextNode() method to add the text node.
- Next, append the created node to the element using the AppendChild() method.
- After that, attach elements to the document body using the HTMLDocument.Body.AppendChild() method.
- Repeat the above steps to add more elements.
- Finally, save the HTML document using the Save() method. It takes the output file path as an argument.
The following code sample shows how to create an HTML file using C#.
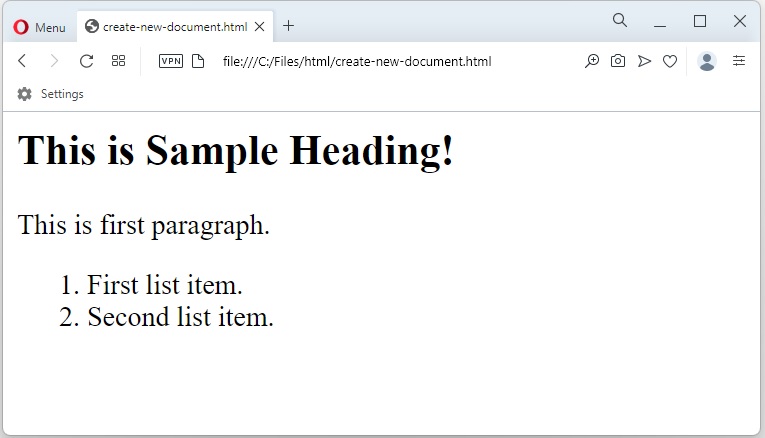
Create an HTML file in C#
Read an HTML File in C#
In the previous section, we created an HTML file. Now, we will load the file and read its content by following the steps given below:
- Load an existing HTML file using the HTMLDocument class.
- Read and show the content of the HTMLDocument.DocumentElement.OuterHTML.
The following code sample shows how to read an HTML file using C#.
<html><head></head><body><h2>This is Sample Heading!</h2><p id="first-paragraph">This is first paragraph. </p><ol><li>First list item.</li><li>Second list item.</li></ol></body></html>
Edit an HTML File in C#
We can also update the content of HTML files. We can add more nodes/elements by following the steps mentioned above. However, we can modify the existing notes/elements by following the steps given below:
- Load an existing HTML file using the HTMLDocument class.
- Next, call the CreateTextNode() method to add the text node.
- Next, get the first paragraph element using the GetElementsByTagName() method. It takes “p” as an argument.
- After that, call the AppendChild() method to append the text node.
- Repeat the above steps to modify more elements.
- Finally, save the HTML document using the Save() method. It takes the output file path as an argument.
The following code sample shows how to modify an HTML file using C#.
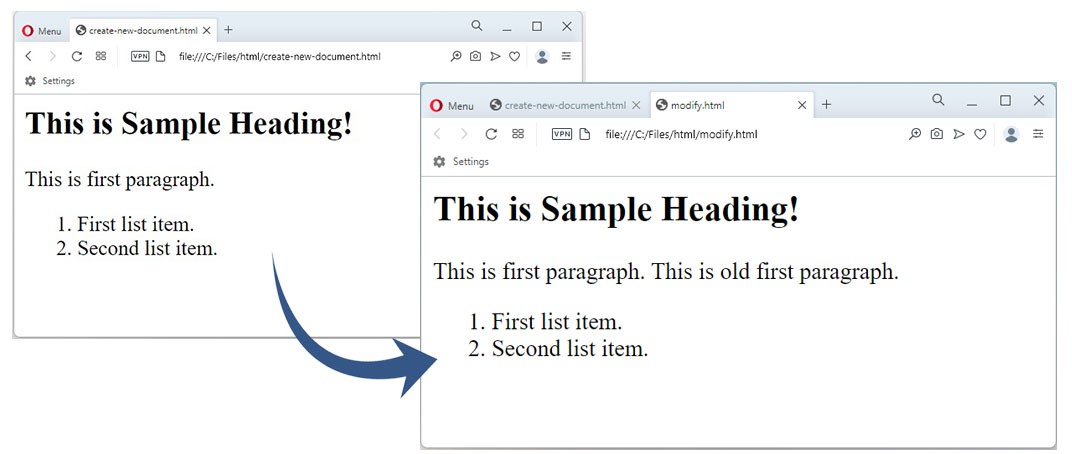
Edit an HTML file in C#
The following code sample shows a more complex example in which we are adding new elements and modifying the existing ones.
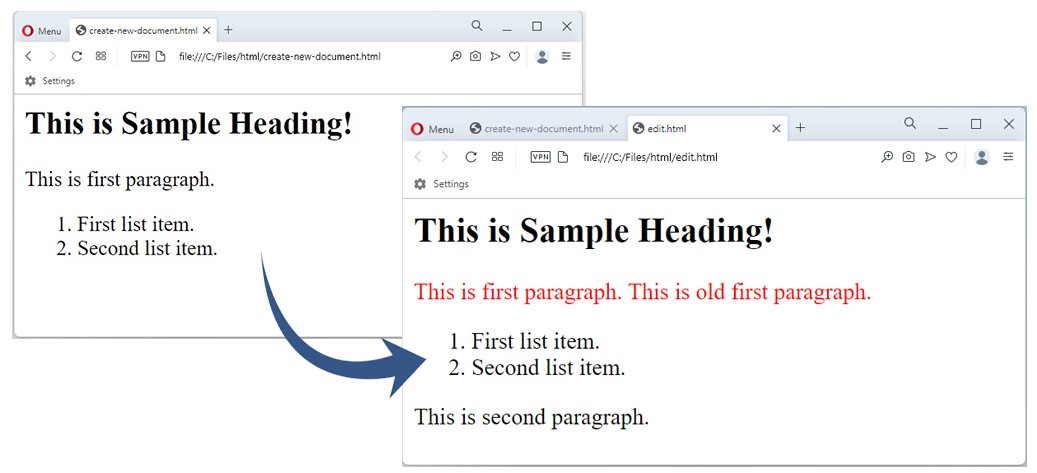
Add and edit elements in an HTML file using C#
Add Raw HTML Content in C#
We can add or edit elements by manually adding HTML code as content using the InnerHTML property of an element. We can add raw HTML by following the steps given below:
- Firstly, create an instance of the HTMLDocument class.
- Next, set the content of the Body.InnerHTML element as raw HTML string.
- Finally, save the HTML document using the Save() method. It takes the output file path as an argument.
The following code sample shows how to add raw HTML content to the file using C#.
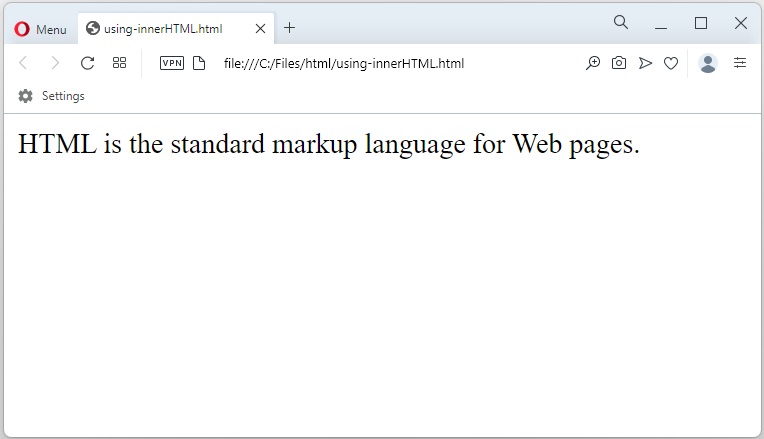
Add raw HTML in an HTML file using C#
Get Free License
You can get a free temporary license to try Aspose.HTML for .NET without evaluation limitations.
Conclusion
In this article, we have learned how to:
- create HTML documents programmatically;
- load an existing HTML document from the local disk path;
- read the HTML from the loaded file;
- add new content to existing HTML file;
- modify existing content in C#.
Besides creating, reading, and editing HTML files in C#, you can learn more about Aspose.HTML for .NET API using documentation. In case of any ambiguity, please feel free to contact us on our free support forum.