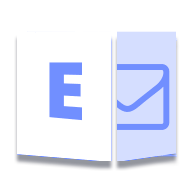
Microsoft Exchange Server adalah platform populer yang menyediakan berbagai layanan kolaborasi seperti email, kalender, kontak, dll. Di posting sebelumnya, kami telah menunjukkan cara membaca email dari Microsoft Exchange Server. Namun, Anda juga mungkin perlu bekerja dengan kontak di Exchange Server secara terprogram. Dalam artikel ini, Anda akan mempelajari cara menambah, menghapus, atau memperbarui kontak di Microsoft Exchange Server di C# .NET.
- .NET API untuk Mengakses Kontak di MS Exchange Server
- Tambahkan Kontak ke MS Exchange Server
- Hapus Kontak dari MS Exchange Server
- Perbarui Kontak di MS Exchange Server
C# .NET API untuk Mengakses Kontak di MS Exchange Server
Untuk bekerja dengan kontak di Microsoft Exchange Server, kami akan menggunakan Aspose.Email for .NET. Ini adalah API terkenal untuk bekerja dengan klien email yang berbeda dari dalam aplikasi .NET. Anda dapat mengunduh DLL API atau menginstalnya dari NuGet menggunakan perintah berikut.
PM> Install-Package Aspose.Email
Tambahkan Kontak ke MS Exchange Server di C#
Berikut adalah langkah-langkah untuk menambahkan kontak ke Microsoft Exchange Server di C#.
- Pertama, buat dan inisialisasi objek NetworkCredential dengan nama pengguna, kata sandi, dan domain.
- Kemudian, inisialisasi IEWSClient dengan URI kotak surat dan objek NetworkCredential.
- Buat objek kelas Kontak dan atur propertinya seperti nama, pekerjaan, jenis kelamin, telepon, orang terkait, dll.
- Terakhir, panggil EWSClient.CreateContact(Contact) untuk menambahkan kontak.
Contoh kode berikut menunjukkan cara menambahkan kontak ke Microsoft Exchange Server di C#.
string mailboxUri = "https://ex2010/ews/exchange.asmx";
string username = "test.exchange";
string password = "pwd";
string domain = "ex2010.local";
NetworkCredential credentials = new NetworkCredential(username, password, domain);
IEWSClient client = EWSClient.GetEWSClient(mailboxUri, credentials);
// Buat kontak baru
Contact contact = new Contact();
// Setel info umum
contact.Gender = Gender.Male;
contact.DisplayName = "Frank Lin";
contact.CompanyName = "ABC Co.";
contact.JobTitle = "Executive Manager";
// Tambahkan nomor Telepon
contact.PhoneNumbers.Add(new PhoneNumber { Number = "123456789", Category = PhoneNumberCategory.Home });
// Kontak orang terkait
contact.AssociatedPersons.Add(new AssociatedPerson { Name = "Catherine", Category = AssociatedPersonCategory.Spouse });
contact.AssociatedPersons.Add(new AssociatedPerson { Name = "Bob", Category = AssociatedPersonCategory.Child });
contact.AssociatedPersons.Add(new AssociatedPerson { Name = "Merry", Category = AssociatedPersonCategory.Sister });
// URL
contact.Urls.Add(new Url { Href = "www.blog.com", Category = UrlCategory.Blog });
contact.Urls.Add(new Url { Href = "www.homepage.com", Category = UrlCategory.HomePage });
// Tetapkan alamat email kontak
contact.EmailAddresses.Add(new EmailAddress { Address = "Frank.Lin@Abc.com", DisplayName = "Frank Lin", Category = EmailAddressCategory.Email1 });
try
{
client.CreateContact(contact);
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
Hapus Kontak dari MS Exchange Server di C#
Anda juga dapat menghapus kontak dari MS Exchange Server. Untuk memfilter kontak, Anda dapat menggunakan nama, email, atau properti lain yang sesuai. Berikut adalah langkah-langkah untuk menghapus kontak dari Microsoft Exchange Server di C#.
- Pertama, inisialisasi objek IEWSClient.
- Kemudian, dapatkan kontak dari MS Exchange Server menggunakan metode IEWSClient.GetContacts(EWSClient.MailboxInfo.ContactsUri).
- Ulangi kontak dan filter yang diperlukan.
- Terakhir, hapus kontak yang difilter menggunakan metode IEWSClient.DeleteItem(Contact.Id.EWSId, DeletionOptions.DeletePermanently).
Contoh kode berikut menunjukkan cara menghapus kontak dari Microsoft Exchange Server di C#.
// Inisialisasi EWSClient
IEWSClient client = EWSClient.GetEWSClient("https://outlook.office365.com/ews/exchange.asmx", "testUser", "pwd", "domain");
string strContactToDelete = "John Teddy";
// Dapatkan kontak
Contact[] contacts = client.GetContacts(client.MailboxInfo.ContactsUri);
// Saring kontak
foreach (Contact contact in contacts)
{
// Hapus kontak
if (contact.DisplayName.Equals(strContactToDelete))
client.DeleteItem(contact.Id.EWSId, DeletionOptions.DeletePermanently);
}
client.Dispose();
Perbarui Kontak di Exchange Server di C#
Anda juga dapat memperbarui kontak di MS Exchange Server menggunakan Aspose.Email for .NET. Berikut ini adalah langkah-langkah untuk melakukan operasi ini.
- Pertama, buat dan inisialisasi objek NetworkCredential dengan nama pengguna, kata sandi, dan domain.
- Kemudian, inisialisasi IEWSClient dengan URI kotak surat dan objek NetworkCredential.
- Dapatkan kontak dari Exchange Server menggunakan metode IEWSClient.GetContacts(EWSClient.MailboxInfo.ContactsUri).
- Setelah itu, lewati kontak dan filter kontak yang diinginkan.
- Terakhir, perbarui properti kontak dan panggil IEWSClient.UpdateContact(Contact) untuk menyimpannya.
Contoh kode berikut menunjukkan cara memperbarui kontak di MS Exchange Server di C#.
string mailboxUri = "https://ex2010/ews/exchange.asmx";
string username = "test.exchange";
string password = "pwd";
string domain = "ex2010.local";
NetworkCredential credentials = new NetworkCredential(username, password, domain);
// Inisialisasi EWSClient
IEWSClient client = EWSClient.GetEWSClient(mailboxUri, credentials);
// Buat daftar semua kontak dan Ulangi semua kontak
Contact[] contacts = client.GetContacts(client.MailboxInfo.ContactsUri);
// Pilih kontak yang diinginkan
Contact contact = contacts[0];
Console.WriteLine("Name: " + contact.DisplayName);
contact.DisplayName = "David Ch";
// Perbarui kontak
client.UpdateContact(contact);
Dapatkan Lisensi API Gratis
Anda bisa mendapatkan lisensi sementara gratis untuk menggunakan Aspose.Email for .NET tanpa batasan evaluasi.
Kesimpulan
Dalam artikel ini, Anda telah mempelajari cara bekerja dengan kontak di Microsoft Exchange Server di C#. Kami telah mendemonstrasikan cara menambah, menghapus, dan memperbarui kontak dari MS Exchange Server secara terprogram di C#. Selain itu, Anda dapat menjelajahi dokumentasi untuk membaca lebih lanjut tentang Aspose.Email for .NET. Selain itu, Anda dapat mengajukan pertanyaan melalui forum kami.