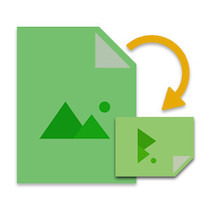
Image flipping is usually listed among the key features of the image editors. While working with images or creating your own image editor in Java, you may need to implement horizontal and vertical image flipping. To achieve that, this article shows how flip images programmatically in Java. We will also cover how to flip and rotate an image at the same time.
- Java API to Flip Images
- Steps to Flip an Image in Java
- Flip an Image in Java
- Rotate and Flip an Image in Java
Flip Images in Java - API Installation
Aspose.Imaging for Java is an image processing API that allows you to manipulate a wide range of image formats. The API makes it quite easier for you to manipulate images without writing a lot of code. You can download the API or install it into your Java applications using the following Maven configurations.
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>http://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-imaging-java</artifactId>
<version>21.12</version>
<classifier>jdk16</classifier>
</dependency>
How to Flip an Image in Java
An image can be flipped horizontally, vertically or in both directions at the same time. Aspose.Imaging for Java simplifies the image flipping in either direction. The RotateFlipType enum is used to specify the flip type (and/or rotation angle). You can perform one of the following flipping operations on an image:
- Flip an image
- Flip and rotate an image at the same time
The following are the steps to flip an image in Java.
- Load the image from the disk.
- Flip image to desired direction.
- Save the updated image on disk.
Let’s have a look at how to transform the above-mentioned steps into code and flip an image in Java.
Flip an Image in Java
To flip an image, the following RotateFlipType values are used.
- RotateNoneFlipX: No rotation with horizontal flipping
- RotateNoneFlipY: No rotation with vertical flipping
- RotateNoneFlipXY: No rotation with horizontal and vertical flipping
The following are the steps to flip an image in Java.
- First, load the image using Image class.
- Then, use Image.rotateFlip(RotateFlipType.RotateNoneFlipX) method to flip the image horizontally.
- Finally, save resultant image using Image.save(string) method.
The following code sample shows how to perform image flipping in Java.
The following are the input image and the resultant flipped image.
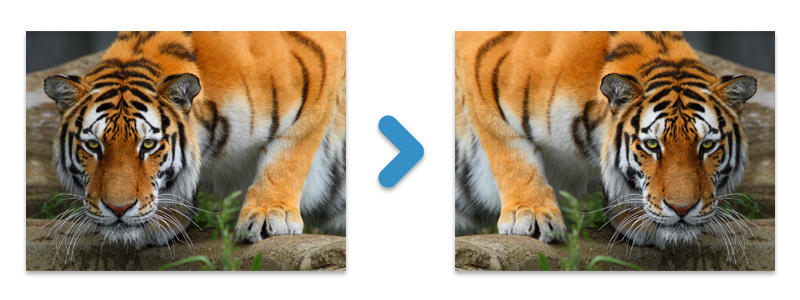
Flip an Image
Flip an Image with Rotation in Java
In the previous section, you have seen how to flip an image without rotation. However, in some cases, you have to perform both operations at the same time. To achieve this, the following RotateFlipType values are used.
- Rotate180FlipX: 180-degree rotation with horizontal flipping
- Rotate180FlipXY: 180-degree rotation with horizontal and vertical flipping
- Rotate180FlipY: 180-degree rotation with vertical flipping
- Rotate270FlipX: 270-degree rotation with horizontal flipping
- Rotate270FlipXY: 270-degree rotation with horizontal and vertical flipping
- Rotate270FlipY: 270-degree rotation with vertical flipping
- Rotate90FlipX: 90-degree rotation with horizontal flipping
- Rotate90FlipXY: 90-degree rotation with horizontal and vertical flipping
- Rotate90FlipY: 90-degree rotation with vertical flipping
The following are the steps to rotate and flip an image at the same time in Java.
- First, use Image class to load an image.
- Then, use Image.rotateFlip(RotateFlipType.Rotate180FlipX) method to rotate and flip the image.
- Finally, save resultant image using Image.save(string) method.
The following code snippet shows how to perform rotation and flipping of an image at the same time in Java.
The following is the resultant image (right) that we get after applying rotation and flipping.
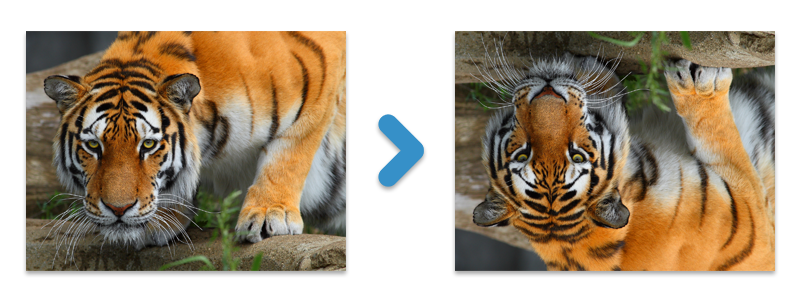
Rotate and Flip an Image
Java Flip Images with a Free License
You can get a free temporary license to flip images without evaluation limitations.
Conclusion
In this article, you have learned how to flip images in Java. Furthermore, you have seen how to rotate and flip an image at the same time programmatically. In case you want to explore more about the Java image processing API, visit documentation. Also, you can download the source code samples of the API from GitHub. In case of any questions, you can reach us at our forum.