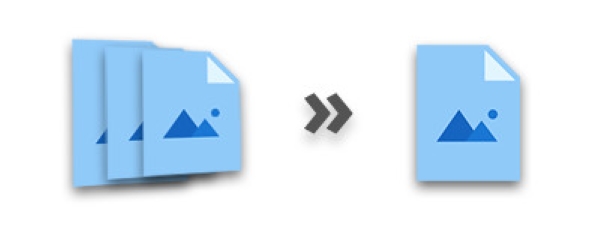
There is a range of scenarios in which image editing and manipulation is performed programmatically. In image editing, merging images is an important feature that is used to combine two or more images, for example, to make a collage. In this article, you will learn how to merge multiple images into a single image in Java. We will explicitly demonstrate how to merge images horizontally and vertically.
Java API to Merge Images - Free Download
Aspose.Imaging for Java is a powerful image processing API that lets you work with a wide range of image formats. It provides a variety of features that are required for image editing. We will use this API to merge our images in this blog post. You can either download the API or install it using the following Maven configurations.
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-imaging</artifactId>
<version>22.7</version>
</dependency>
Merge Multiple Images in Java
You can merge the images in either of the two ways: vertically and horizontally. In vertical merging, images are combined one by one in a vertical direction. Whereas, in horizontal merging, the images are appended to each other in the horizontal direction. So let’s see how to merge images in both ways.
Merge Images Horizontally in Java
The following are the steps to merge images horizontally using Java.
- First, specify the paths of the images in a string array.
- Calculate the height and width of the resultant image.
- Create an object of JpegOptions class and set the required options.
- Create an object of JpegImage class and initialize it with JpegOptions object and height and width of resultant image.
- Loop through list of images and load each image using RasterImage class.
- Create a Rectangle for each image and add it to resultant image using JpegImage.saveArgb32Pixels() method.
- Increase the stitched width in each iteration.
- Once done, save the resultant image using JpegImage.save(string) method.
The following code sample shows how to merge images horizontally in Java.
The following image shows the output of merging two similar images horizontally.
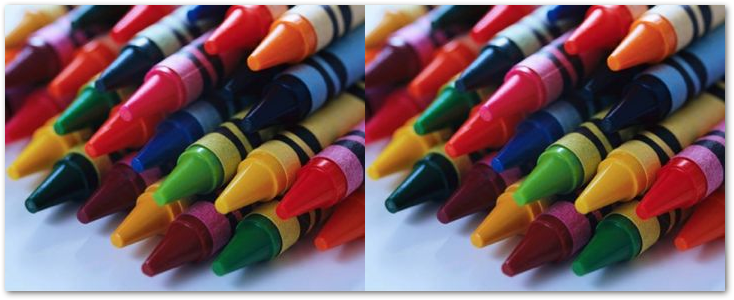
Merge Images Vertically in Java
To merge the images vertically, you only need to switch the roles of height and width properties. The rest of the code will be the same. The following code sample demonstrates how to merge multiple images vertically in Java.
The following image shows the output of merging two similar images vertically.

Merge PNG Images in Java
In the previous sections, we demonstrated how to merge images in JPG format. However, you may need to merge images in PNG format too. To merge PNG images, you only need to replace JpegImage and JpegOptions classes with PngImage and PngOptions classes respectively and rest of the code will remain the same.
The following code sample shows how to merge multiple images in PNG format in Java.
Java Image Merging API - Get a Free License
You can get a free temporary license and merge the images without evaluation limitations.
Conclusion
In this article, you have learned how to merge multiple images into a single image using Java. The Java code samples have demonstrated how to combine the images vertically and horizontally. In addition, you can explore more about the Java image processing API using documentation. Also, you can share your queries with us via our forum.
See Also
- Convert Images to Grayscale in C#
- Add Watermark to Images using C#
- Compress PNG, JPEG, and TIFF Images using C#
Info: Aspose provides a FREE Collage web app. Using this online service, you can merge JPG to JPG or PNG to PNG images, create photo grids, and so on.