If you are looking for a simple to implement yet the precise method of resizing images programmatically, you have landed at the right place. This article demonstrates how to resize images in Java within a few simple steps.
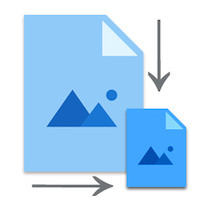
The image editors provide resizing feature that lets you alter the size of images as per your requirements. While designing an image editor or processing images from within your Java applications, you may need to resize images programmatically. To accomplish that, this article covers how to resize images in Java. Moreover, you will also learn how to resize images using different resizing techniques.
Resize Images in Java - API Installation
Aspose.Imaging for Java is a widely used image processing API that lets you manipulate images quite easily. We will use this API to resize images in this article. You can download the API from the downloads section. Also, you can install it from Maven using the following configuration in pom.xml.
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>http://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-imaging-java</artifactId>
<version>21.12</version>
<classifier>jdk16</classifier>
</dependency>
Resize Raster Images in Java
Aspose.Imaging for Java provides two ways to resize raster images. One is simple resizing in which you provide the height and width of the resultant image. Whereas, in the second method, you also specify a resize type that tells the API about the resizing technique that should be used, such as Lanczos Resampling, Nearest Neighbor Resampling, etc. In the following sections, we will discuss how to implement each of these methods to resize images.
Simple Resizing of Images in Java
In this resizing method, you only have to provide the dimensions of the resultant image. The following are the steps to resize an image in Java.
- First, use Image class to load the image you want to resize.
- Then, call Image.resize(int, int) method to resize the image.
- Finally, save the resultant image using Image.save(string) method.
The following code snippet resizes an image to the provided height and width.
Java Resize Image with a Specific Type
Aspose.Imaging for Java provides a wide range of resizing techniques, which are listed here. You can choose one that suits your scenario. For example, Lanczos Resampling is recommended for quality image resizing, however, it works slower. Whereas for fast resizing Nearest Neighbour Resampling is used, however, it compromises the quality of the image. The following are the steps to resize an image using a particular resizing technique.
- First, use Image class to load the image you want to resize.
- Then, call Image.resize(int, int, ResizeType) method to resize the image using a particular technique.
- Finally, save the resultant image using Image.save(string) method.
The following code sample shows how to resize an image using a particular resizing technique.
Proportional Image Resizing in Java
The aspect ratio is calculated while resizing the images to avoid shrunk or scaled resultant images. However, any miscalculation of the aspect ratio may cause stretched images. To get rid of such issues, you can let the API take care of the aspect ratio itself. The following are the steps to resize an image proportionally.
- First, use Image class to load the image you want to resize.
- Cache image data using Image.cacheData() method.
- Define the height and width of the resultant image.
- Call Image.resizeWidthProportionally(int) and Image.resizeHeightProportionally(int) methods to calculate proportion values.
- Finally, save the resultant image using Image.save(string) method.
You can also specify a particular resize type while resizing the image proportionally using the overload Image.resizeWidthProportionally(int, ResizeType) and Image.resizeHeightProportionally(int, ResizeType) methods.
The following Java code sample shows how to resize an image proportionally.
Resizing Vector Images in Java
You can also resize a vector image and save it to a raster image format such as PNG, JPEG, GIF, etc. The following code demonstrates how to achieve that in Java using Aspose.Imaging.
Java Image Resizing API - Get a Free License
You can use Aspose.Imaging for Java and resize images without evaluation limitations by getting a free temporary license.
Conclusion
In this article, you have learned how to resize images in Java. Furthermore, the article has covered how to resize images using different techniques such as Lanczos Resampling, Nearest Neighbor Resampling, etc. In addition, proportional image resizing and resizing vector images in Java are also discussed. You can visit documentation to read more about Java image processing API. Also, you can learn from the source code samples available on GitHub. In case of any questions, you can reach us at our forum.