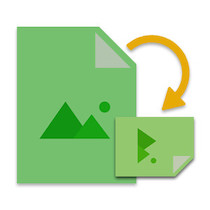
As a programmer, you may often need to manipulate the images from within your Java applications. The image manipulation and editing may also include rotation of an Image at a particular angle. To achieve that, this article shows how to rotate an image programmatically in Java. In addition, we will demonstrate how to rotate and flip an image at the same time.
- Java API to Rotate Images
- Steps to Rotate an Image in Java
- Rotate an Image in Java
- Rotate an Image on a Particular Angle
- Rotate and Flip an Image in Java
Java API to Rotate Images - Free Download
Aspose.Imaging for Java is an image processing API that allows you to manipulate a wide range of image formats. The API makes it quite easier for you to manipulate images without writing a lot of code. We will use this API to rotate the images. You can download the API or install it into your Java applications using the following Maven configurations.
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>http://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-imaging-java</artifactId>
<version>21.12</version>
<classifier>jdk16</classifier>
</dependency>
Steps to Rotate an Image in Java
You can rotate an image at either a particular angle or the standard rotation angles such as 90, 180, and 270 degrees. The RotateFlipType enum is used to specify the rotation angle (and/or flip type). You can perform one of the following rotation operations on an image:
- Rotate an image
- Rotate and flip an image at the same time
The following are the steps to rotate an image.
- Load the image from disk.
- Specify the rotation angle and rotate the image.
- Save the rotated image.
Let’s now have a look at how to rotate an image with Java.
Rotate an Image in Java
To rotate an image, you can choose one of the following values of RotateFlipType enum.
- Rotate180FlipNone: 180-degree rotation without flipping
- Rotate270FlipNone: 270-degree rotation without flipping
- Rotate90FlipNone: 90-degree rotation without flipping
Let’s see how to rotate an image at 270 degrees in Java.
- First, use Image class to load the image.
- Then, use Image.rotateFlip(RotateFlipType.Rotate270FlipNone) method to rotate image to 270 degrees.
- Finally, save resultant image using Image.save(string) method.
The following code snippet shows how to perform image rotation in Java.
The following are the input image (left) and the resultant image (right) that we get after rotation.
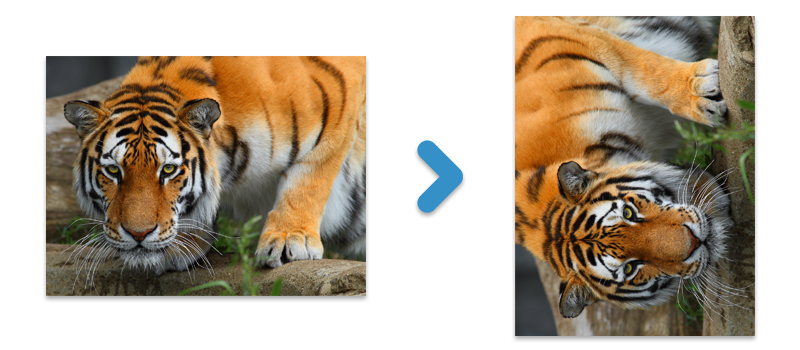
Rotate an Image
Java Image Rotation at a Particular Angle
You can also rotate an image at an angle other than 90, 180, and 270. For this, the API provides the Image.rotate(float angle) method which accepts the rotation angle as a parameter. The following code sample shows how to rotate an image at 20 degrees in Java.
The following is the output of the code sample above.
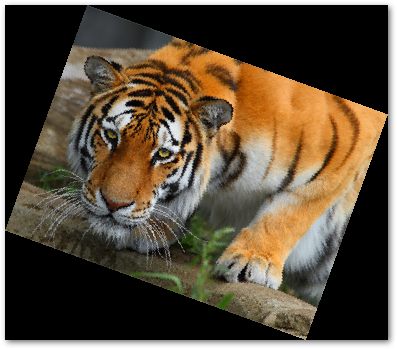
Rotate and Flip an Image in Java
In the previous sections, you have seen how to rotate an image at desired angle. However, in some cases, you have to rotate and flip images at the same time. To achieve this, the following RotateFlipType values are used.
- Rotate180FlipX: 180-degree rotation with horizontal flipping
- Rotate180FlipXY: 180-degree rotation with horizontal and vertical flipping
- Rotate180FlipY: 180-degree rotation with vertical flipping
- Rotate270FlipX: 270-degree rotation with horizontal flipping
- Rotate270FlipXY: 270-degree rotation with horizontal and vertical flipping
- Rotate270FlipY: 270-degree rotation with vertical flipping
- Rotate90FlipX: 90-degree rotation with horizontal flipping
- Rotate90FlipXY: 90-degree rotation with horizontal and vertical flipping
- Rotate90FlipY: 90-degree rotation with vertical flipping
The following are the steps to rotate and flip an image at the same time in Java.
- First, use Image class to load an image.
- Then, use Image.rotateFlip(RotateFlipType.Rotate180FlipX) method to rotate and flip the image.
- Finally, save resultant image using Image.save(string) method.
The following code snippet shows how to perform rotation and flipping of an image at the same time.
The following is the resultant image (right) that we get after applying rotation and flipping.
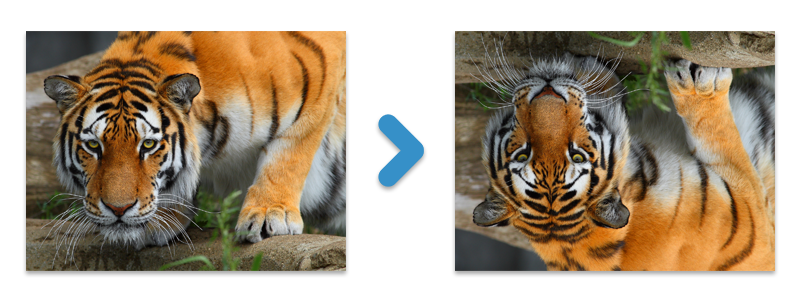
Rotate and Flip an Image
Java Image Rotation API - Get a Free License
You can get a free temporary license to rotate images without evaluation limitations.
Conclusion
In this article, you have learned how to rotate images in Java. Furthermore, you have seen how to rotate and flip an image at the same time. In addition, the article also covered how to rotate an image at a particular angle other than 90, 180, and 270 degrees.
Java Image Editing API
In case you want to explore more about Aspose’ Java image editing API, visit documentation. Also, you can download the source code samples of the API from GitHub. In case of any questions, you can reach us at our forum.