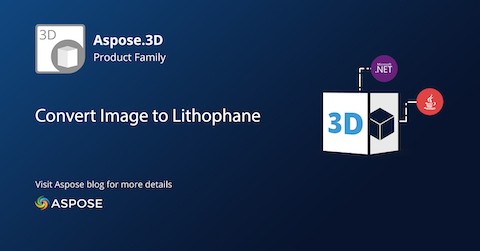
Il formato PNG è popolare in quanto può includere grafica trasparente. Considerando che, lithophane è un’opera d’arte incisa o modellata in materiale molto sottile che puoi vedere mettendo la fonte di luce dietro tale modello. Questo articolo illustra come convertire un’immagine PNG in litofane in C#.
- Convertitore da immagine PNG a litofane – Installazione dell’API C#
- Converti immagine PNG in litofane in C#
Convertitore da immagine PNG a litofane – Installazione dell’API C#
L’API Aspose.3D for .NET può essere utilizzata per lavorare con diversi modelli e scene 3D. È possibile configurare facilmente l’API scaricando i suoi file DLL di riferimento dalla pagina Nuove versioni o utilizzando il seguente comando di installazione NuGet:
PM> Install-Package Aspose.3D
Converti immagine PNG in litofane in C#
Puoi convertire un file immagine PNG in formato Lithophane seguendo i passaggi seguenti:
- Carica l’immagine PNG di input.
- Eseguire operazioni di calcolo su oggetti Mesh.
- Genera scene 3d e salva oggetti con il metodo Salva.
Il frammento di codice seguente spiega come convertire un’immagine PNG in Lithophane a livello di codice in C#:
string file = "template.png";
string output = "file.fbx";
// Crea nuovi parametri
Aspose.ThreeD.Render.TextureData td = Aspose.ThreeD.Render.TextureData.FromFile(file);
const float nozzleSize = 0.9f;
const float layerHeight = 0.2f;
var grayscale = ToGrayscale(td);
const float width = 120.0f;
float height = width / td.Width * td.Height;
float thickness = 10.0f;
float layers = thickness / layerHeight;
int widthSegs = (int)Math.Floor(width / nozzleSize);
int heightSegs = (int)Math.Floor(height / nozzleSize);
// Eseguire operazioni di calcolo su oggetti Mesh
Aspose.ThreeD.Entities.Mesh mesh = new Aspose.ThreeD.Entities.Mesh();
for (int y = 0; y < heightSegs; y++)
{
float dy = (float)y / heightSegs;
for (int x = 0; x < widthSegs; x++)
{
float dx = (float)x / widthSegs;
float gray = Sample(grayscale, td.Width, td.Height, dx, dy);
float v = (1 - gray) * thickness;
mesh.ControlPoints.Add(new Aspose.ThreeD.Utilities.Vector4(dx * width, dy * height, v));
}
}
for (int y = 0; y < heightSegs - 1; y++)
{
int row = (y * heightSegs);
int ptr = row;
for (int x = 0; x < widthSegs - 1; x++)
{
mesh.CreatePolygon(ptr, ptr + widthSegs, ptr + 1);
mesh.CreatePolygon(ptr + 1, ptr + widthSegs, ptr + widthSegs + 1);
ptr++;
}
}
// Genera scene 3d e salva oggetti
Aspose.ThreeD.Scene scene = new Aspose.ThreeD.Scene(mesh);
scene.Save(output, Aspose.ThreeD.FileFormat.FBX7400ASCII);
// Il metodo di esempio da chiamare
static float Sample(float[,] data, int w, int h, float x, float y)
{
return data[(int)(x * w), (int)(y * h)];
}
// Metodo ToGrayscale da chiamare
static float[,] ToGrayscale(Aspose.ThreeD.Render.TextureData td)
{
var ret = new float[td.Width, td.Height];
var stride = td.Stride;
var data = td.Data;
var bytesPerPixel = td.BytesPerPixel;
for (int y = 0; y < td.Height; y++)
{
int ptr = y * stride;
for (int x = 0; x < td.Width; x++)
{
var v = (data[ptr] * 0.21f + data[ptr + 1] * 0.72f + data[ptr + 2] * 0.07f) / 255.0f;
ret[x, y] = v;
ptr += bytesPerPixel;
}
}
return ret;
}
Ottieni una licenza temporanea gratuita
Puoi richiedere una licenza temporanea gratuita per valutare l’API senza alcuna limitazione.
Demo in linea
Prova l’app web PNG Image to Lithophane Converter sviluppata utilizzando questa API.
Conclusione
In questo articolo, hai esplorato la conversione dell’immagine PNG in litofane. Inoltre, puoi dare un’occhiata allo spazio documentazione per apprendere altre funzionalità dell’API. In caso di domande o domande, scrivici al forum.