
Converti MP4 in MP3
Se stai cercando uno strumento di alta qualità per convertire MP4 in MP3, usa il nostro convertitore online da MP4 a MP3. Con un’interfaccia intuitiva, il nostro convertitore MP4 online ti consente di estrarre l’audio dai file video in un paio di passaggi.
Converti i tuoi file MP4 in MP3 con questo Convertitore gratuito da MP4 a MP3 senza creare un account. Sbarazzati dei convertitori MP4 installabili.
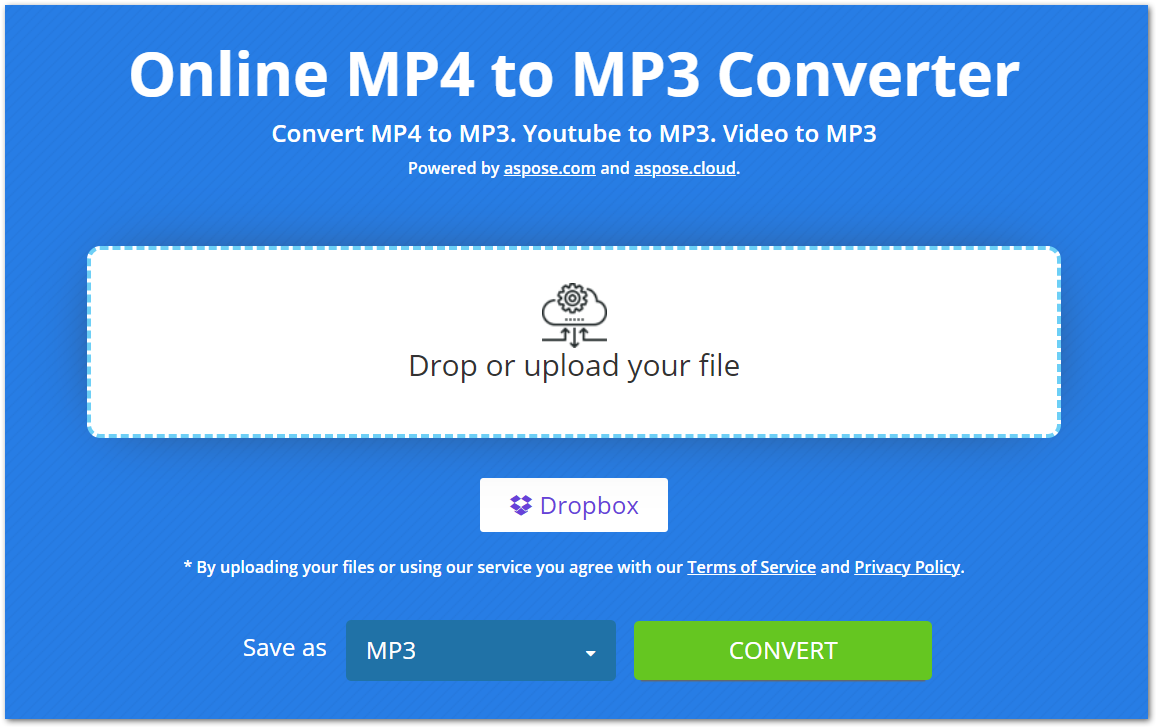
Utilizzo del convertitore online da MP4 a MP3
Con il nostro convertitore online, la conversione da MP4 a MP3 è diventata semplicissima. Devi eseguire solo un paio di passaggi per estrarre l’audio da un file MP4, come indicato di seguito.
- Carica il tuo file MP4 dal tuo computer o Dropbox.
- Una volta caricato, premi il pulsante “CONVERTI” per avviare la conversione.
- Dopo la conversione, il file MP3 sarà disponibile per il download.
Tieni presente che teniamo al sicuro i tuoi file MP4/MP3 caricati e convertiti e li cancelliamo dai nostri server dopo 24 ore.
Perché il convertitore online da MP4 a MP3?
Ci sono diversi motivi per cui questo convertitore online da MP4 a MP3 è utile, tra cui:
Facile accesso: poiché si tratta di un convertitore basato sul Web, è possibile accedervi da qualsiasi dispositivo dotato di connessione Internet.
Gratuito: è completamente gratuito convertire i tuoi file MP4 in MP3 utilizzando questo convertitore. Inoltre, non c’è limite al numero di conversioni che puoi eseguire.
Nessuna registrazione: non ti chiediamo di creare un account prima di utilizzare questo convertitore MP4 online. Basta aprirlo nel browser e iniziare a convertire i file.
Facile da usare: l’interfaccia di questo convertitore è facile da usare, quindi puoi convertire i tuoi file senza sforzo.
Guida per sviluppatori
Questo convertitore online si basa sulla nostra API, Aspose.Slides, che è disponibile per .NET, Java, C++, Python, PHP e altre piattaforme. Di seguito è riportata la dimostrazione di come utilizzare questa API e convertire una presentazione PowerPoint in un formato video.
C#
- Installa Aspose.Slides for .NET in your application.
- Scarica e installa il pacchetto ffmpeg.
- Usa il codice qui sotto per creare un video da PPT:
using System.Collections.Generic;
using Aspose.Slides;
using FFMpegCore; // Will use FFmpeg binaries we extracted to "c:\tools\ffmpeg" before
using Aspose.Slides.Animation;
using (Presentation presentation = new Presentation())
{
// Adds a smile shape and then animates it
IAutoShape smile = presentation.Slides[0].Shapes.AddAutoShape(ShapeType.SmileyFace, 110, 20, 500, 500);
IEffect effectIn = presentation.Slides[0].Timeline.MainSequence.AddEffect(smile, EffectType.Fly, EffectSubtype.TopLeft, EffectTriggerType.AfterPrevious);
IEffect effectOut = presentation.Slides[0].Timeline.MainSequence.AddEffect(smile, EffectType.Fly, EffectSubtype.BottomRight, EffectTriggerType.AfterPrevious);
effectIn.Timing.Duration = 2f;
effectOut.PresetClassType = EffectPresetClassType.Exit;
const int Fps = 33;
List<string> frames = new List<string>();
using (var animationsGenerator = new PresentationAnimationsGenerator(presentation))
using (var player = new PresentationPlayer(animationsGenerator, Fps))
{
player.FrameTick += (sender, args) =>
{
string frame = $"frame_{(sender.FrameIndex):D4}.png";
args.GetFrame().Save(frame);
frames.Add(frame);
};
animationsGenerator.Run(presentation.Slides);
}
// Configure ffmpeg binaries folder. See this page: https://github.com/rosenbjerg/FFMpegCore#installation
GlobalFFOptions.Configure(new FFOptions { BinaryFolder = @"c:\tools\ffmpeg\bin", });
// Converts frames to webm video
FFMpeg.JoinImageSequence("smile.webm", Fps, frames.Select(frame => ImageInfo.FromPath(frame)).ToArray());
}
Java
- Installa Aspose.Slides for Java in your application.
- Aggiungi la seguente dipendenza.
<dependency>
<groupId>net.bramp.ffmpeg</groupId>
<artifactId>ffmpeg</artifactId>
<version>0.7.0</version>
</dependency>
- Copia e incolla il codice qui sotto per creare un video da PPT:
Presentation presentation = new Presentation();
try {
// Adds a smile shape and then animates it
IAutoShape smile = presentation.getSlides().get_Item(0).getShapes().addAutoShape(ShapeType.SmileyFace, 110, 20, 500, 500);
ISequence mainSequence = presentation.getSlides().get_Item(0).getTimeline().getMainSequence();
IEffect effectIn = mainSequence.addEffect(smile, EffectType.Fly, EffectSubtype.TopLeft, EffectTriggerType.AfterPrevious);
IEffect effectOut = mainSequence.addEffect(smile, EffectType.Fly, EffectSubtype.BottomRight, EffectTriggerType.AfterPrevious);
effectIn.getTiming().setDuration(2f);
effectOut.setPresetClassType(EffectPresetClassType.Exit);
final int fps = 33;
ArrayList<String> frames = new ArrayList<String>();
PresentationAnimationsGenerator animationsGenerator = new PresentationAnimationsGenerator(presentation);
try
{
PresentationPlayer player = new PresentationPlayer(animationsGenerator, fps);
try {
player.setFrameTick((sender, arguments) ->
{
try {
String frame = String.format("frame_%04d.png", sender.getFrameIndex());
ImageIO.write(arguments.getFrame(), "PNG", new java.io.File(frame));
frames.add(frame);
} catch (IOException e) {
throw new RuntimeException(e);
}
});
animationsGenerator.run(presentation.getSlides());
} finally {
if (player != null) player.dispose();
}
} finally {
if (animationsGenerator != null) animationsGenerator.dispose();
}
// Configure ffmpeg binaries folder. See this page: https://github.com/rosenbjerg/FFMpegCore#installation
FFmpeg ffmpeg = new FFmpeg("path/to/ffmpeg");
FFprobe ffprobe = new FFprobe("path/to/ffprobe");
FFmpegBuilder builder = new FFmpegBuilder()
.addExtraArgs("-start_number", "1")
.setInput("frame_%04d.png")
.addOutput("output.avi")
.setVideoFrameRate(FFmpeg.FPS_24)
.setFormat("avi")
.done();
FFmpegExecutor executor = new FFmpegExecutor(ffmpeg, ffprobe);
executor.createJob(builder).run();
} catch (IOException e) {
e.printStackTrace();
}
C++
- Installa Aspose.Slides for C++ in your application.
- Scarica e installa il pacchetto ffmpeg.
- Usa il codice qui sotto per creare un video da PPT:
void OnFrameTick(System::SharedPtr<PresentationPlayer> sender, System::SharedPtr<FrameTickEventArgs> args)
{
System::String fileName = System::String::Format(u"frame_{0}.png", sender->get_FrameIndex());
args->GetFrame()->Save(fileName);
}
void Run()
{
auto presentation = System::MakeObject<Presentation>();
auto slide = presentation->get_Slide(0);
// Adds a smile shape and then animates it
System::SharedPtr<IAutoShape> smile = slide->get_Shapes()->AddAutoShape(ShapeType::SmileyFace, 110.0f, 20.0f, 500.0f, 500.0f);
auto sequence = slide->get_Timeline()->get_MainSequence();
System::SharedPtr<IEffect> effectIn = sequence->AddEffect(smile, EffectType::Fly, EffectSubtype::TopLeft, EffectTriggerType::AfterPrevious);
System::SharedPtr<IEffect> effectOut = sequence->AddEffect(smile, EffectType::Fly, EffectSubtype::BottomRight, EffectTriggerType::AfterPrevious);
effectIn->get_Timing()->set_Duration(2.0f);
effectOut->set_PresetClassType(EffectPresetClassType::Exit);
const int32_t fps = 33;
auto animationsGenerator = System::MakeObject<PresentationAnimationsGenerator>(presentation);
auto player = System::MakeObject<PresentationPlayer>(animationsGenerator, fps);
player->FrameTick += OnFrameTick;
animationsGenerator->Run(presentation->get_Slides());
const System::String ffmpegParameters = System::String::Format(
u"-loglevel {0} -framerate {1} -i {2} -y -c:v {3} -pix_fmt {4} {5}",
u"warning", m_fps, "frame_%d.png", u"libx264", u"yuv420p", "video.mp4");
auto ffmpegProcess = System::Diagnostics::Process::Start(u"ffmpeg", ffmpegParameters);
ffmpegProcess->WaitForExit();
}
Esplora le librerie di PowerPoint
Se vuoi esplorare di più sulle nostre librerie di manipolazione di PowerPoint, di seguito sono riportate alcune risorse utili.
Domande frequenti
Come convertire MP4 in MP3?
Per convertire un file MP4 in MP3, è sufficiente caricare il file e avviare la conversione premendo il pulsante CONVERTI. Una volta convertito, il file MP3 sarà pronto per il download.
È sicuro caricare i miei file MP4 qui?
Sì, manteniamo i tuoi file al sicuro e li cancelliamo dai nostri server dopo 24 ore.
Questo convertitore converte MP4 in MP3 con alta qualità?
Assolutamente! Il convertitore garantisce la conversione di alta qualità dei file MP4 in MP3.
Conclusione
In questo articolo, hai imparato come convertire i file MP4 in MP3 utilizzando il nostro convertitore online da MP4 a MP3. Pertanto, puoi convertire i tuoi file MP4 sempre e ovunque. Inoltre, ti abbiamo fornito le nostre librerie autonome che puoi utilizzare per manipolare i video nelle presentazioni PowerPoint.