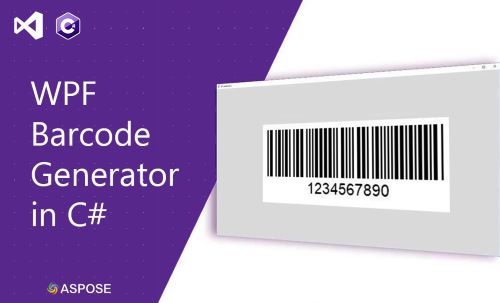
バーコードには、製品または会社に関する情報が含まれており、機械可読形式で視覚的に表示されます。バーコードは、出荷と在庫管理を追跡するために広く使用されています。 WPFアプリケーションでは、さまざまな種類のバーコードを簡単に生成できます。この記事では、WPFアプリケーションでバーコード画像を生成して表示する方法を学習します。上記の手順を実行すると、C#で独自のWPFバーコードジェネレーターが作成されます。それでは始めましょう。
この記事は、次のトピックをカバーするものとします。
- WPFバーコードジェネレーターの機能
- C#バーコードジェネレータAPI
- WPFバーコードジェネレーターを作成する手順
- 追加オプションでバーコードを生成
- デモWPFバーコードジェネレーター
- ソースコードをダウンロード
WPFバーコードジェネレーターの機能
WPFバーコードジェネレーターには次の機能があります。
- 次のタイプのバーコードシンボルを生成します。
- Code128
- Code11
- Code39
- QR
- Datamatrix
- EAN13
- EAN8
- ITF14
- PDF417
- 生成されたバーコード画像を次の形式で保存します。
- 生成されたバーコード画像をプレビューします。
C#バーコードジェネレーターAPI
Aspose.BarCode for .NET APIを使用してバーコード画像を生成し、WPFアプリケーションでプレビューします。これは、さまざまなバーコードタイプを生成、スキャン、および読み取ることができる機能豊富なAPIです。さらに、背景色、バーの色、回転角度、x寸法、画質、解像度、キャプション、サイズなど、生成されたバーコードの外観を操作できます。
WPFバーコードジェネレーターを作成する手順
以下の手順に従って、WPFアプリケーションでバーコード画像を生成して表示できます。
- まず、新しいプロジェクトを作成し、WPFアプリケーションプロジェクトテンプレートを選択します。
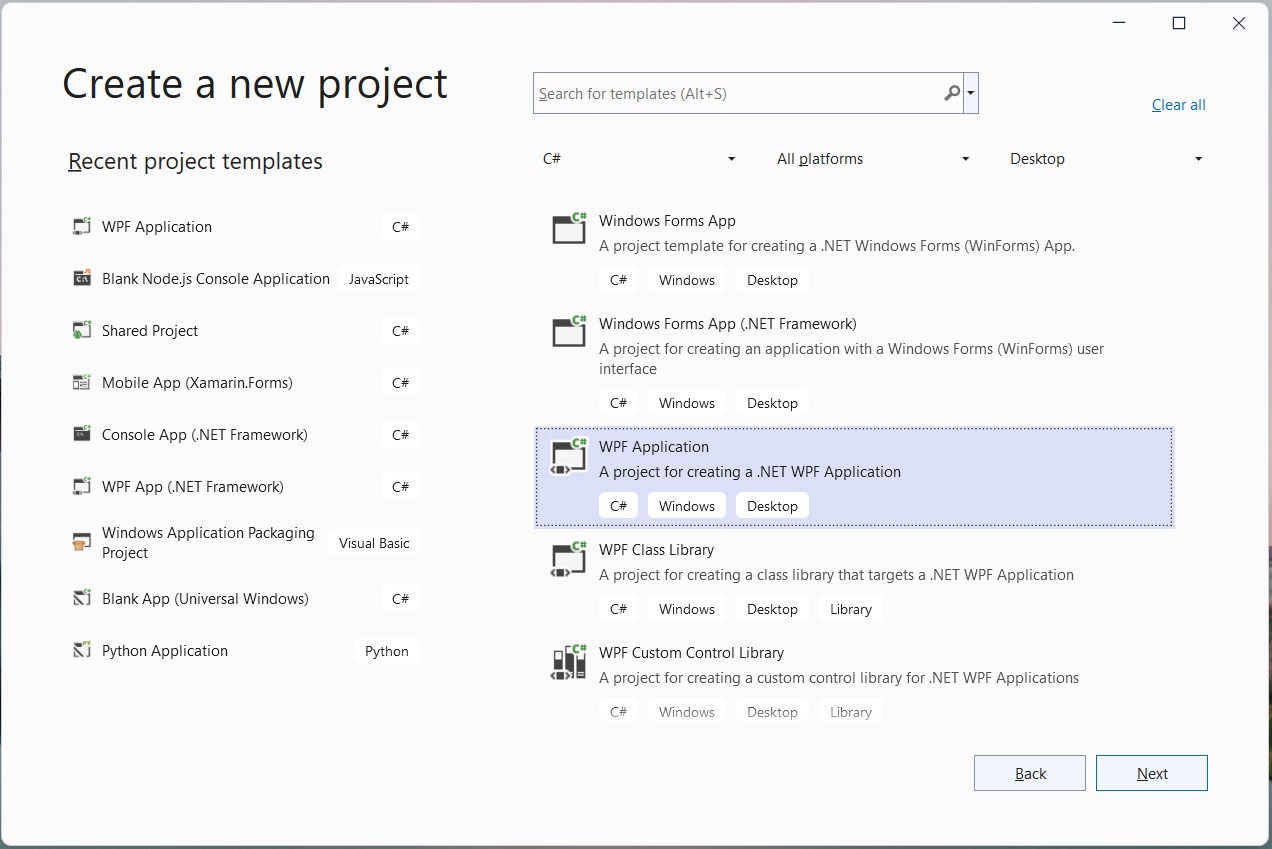
Select the project template.
次に、プロジェクトの名前(「BarcodeGen」など)を入力します。
次に、.NET Frameworkを選択してから、作成を選択します。
次に、NuGetパッケージマネージャーを開き、Aspose.BarCode for.NETパッケージをインストールします。

Aspose.BarCodefor.NETをインストールします
- 次に、新しいクラスBarcode.csを追加して、バーコードを定義します。
public class Barcode
{
public string? Text { get; set; }
public BaseEncodeType? BarcodeType { get; set; }
public BarCodeImageFormat ImageType { get; set; }
}
- 次に、MainWindow.xamlを開き、以下に示すように必要なコントロールを追加します。
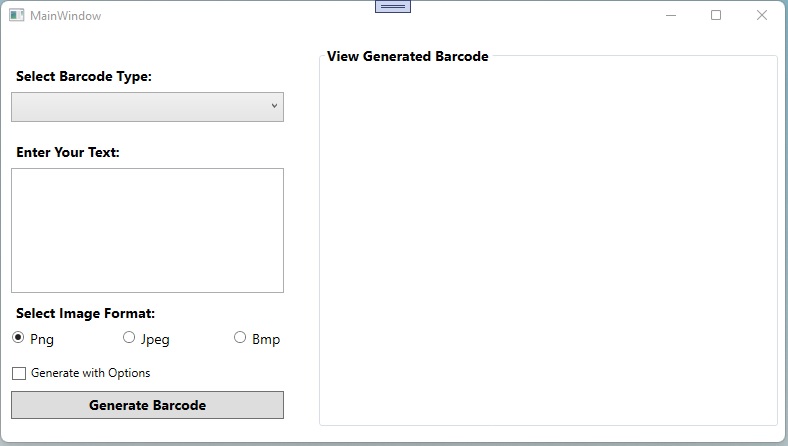
必要なコントロールを追加します
MainWindow.xamlのコンテンツを次のスクリプトに置き換えることもできます。
<Window x:Class="BarcodeGen.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:BarcodeGen"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid Width="800" Height="384">
<Grid.RowDefinitions>
<RowDefinition Height="191*"/>
<RowDefinition/>
</Grid.RowDefinitions>
<Label Content="Select Barcode Type:" HorizontalAlignment="Left" Margin="10,16,0,0" VerticalAlignment="Top" FontSize="14" FontWeight="Bold"/>
<ComboBox x:Name="comboBarcodeType" HorizontalAlignment="Left" Margin="10,47,0,305" Width="273" Text="Select Barcode Type" IsReadOnly="True" SelectedIndex="-1" FontSize="14" Height="30">
<ComboBoxItem Content="Code128"></ComboBoxItem>
<ComboBoxItem Content="Code11"></ComboBoxItem>
<ComboBoxItem Content="Code32"></ComboBoxItem>
<ComboBoxItem Content="QR"></ComboBoxItem>
<ComboBoxItem Content="DataMatrix"></ComboBoxItem>
<ComboBoxItem Content="EAN13"></ComboBoxItem>
<ComboBoxItem Content="EAN8"></ComboBoxItem>
<ComboBoxItem Content="ITF14"></ComboBoxItem>
<ComboBoxItem Content="PDF417"></ComboBoxItem>
</ComboBox>
<Button Name="btnGenerate" Click="btnGenerate_Click" Content="Generate Barcode" HorizontalAlignment="Left" Margin="10,346,0,0" VerticalAlignment="Top" Height="28" Width="273" FontSize="14" FontWeight="Bold"/>
<Label Content="Enter Your Text:" HorizontalAlignment="Left" Margin="10,92,0,0" VerticalAlignment="Top" FontSize="14" FontWeight="Bold"/>
<TextBox Name="tbCodeText" TextWrapping="Wrap" Margin="10,123,517,134" Width="273" Height="125"/>
<Label Content="Select Image Format:" HorizontalAlignment="Left" Margin="10,253,0,0" VerticalAlignment="Top" FontSize="14" FontWeight="Bold"/>
<RadioButton Name="rbPng" Content="Png" GroupName="rbImageType" Margin="10,285,739,77" Width="51" Height="20" FontSize="14" IsChecked="True"/>
<RadioButton Name="rbJpg" Content="Jpeg" GroupName="rbImageType" Margin="121,285,628,77" Width="51" Height="20" FontSize="14"/>
<RadioButton Name="rbBmp" Content="Bmp" GroupName="rbImageType" Margin="232,285,517,77" Width="51" Height="20" FontSize="14"/>
<CheckBox Name="cbGenerateWithOptions" Height="20" Margin="10,321,517,41" Content="Generate with Options" />
<GroupBox Header="View Generated Barcode" Margin="317,0,22,0" FontSize="14" FontWeight="Bold">
<Image Name="imgDynamic" Margin="6,-6,7,6" Stretch="None" />
</GroupBox>
</Grid>
</Window>
- 次に、MainWindow.xaml.csクラスを開き、btnGenerateClickイベントを追加して、[バーコードの生成]ボタンのクリックアクションを処理します。
private void btnGenerate_Click(object sender, RoutedEventArgs e)
{
// デフォルトをPngに設定
var imageType = "Png";
// ユーザーが選択した画像形式を取得する
if(rbPng.IsChecked == true)
{
imageType = rbPng.Content.ToString();
}
else if(rbBmp.IsChecked == true)
{
imageType = rbBmp.Content.ToString();
}
else if(rbJpg.IsChecked == true)
{
imageType = rbJpg.Content.ToString();
}
// 列挙型から画像形式を取得する
var imageFormat = (BarCodeImageFormat)Enum.Parse(typeof(BarCodeImageFormat), imageType.ToString());
// デフォルトをCode128に設定
var encodeType = EncodeTypes.Code128;
// ユーザーが選択したバーコードタイプを取得する
if (!string.IsNullOrEmpty(comboBarcodeType.Text))
{
switch (comboBarcodeType.Text)
{
case "Code128":
encodeType = EncodeTypes.Code128;
break;
case "ITF14":
encodeType = EncodeTypes.ITF14;
break;
case "EAN13":
encodeType = EncodeTypes.EAN13;
break;
case "Datamatrix":
encodeType = EncodeTypes.DataMatrix;
break;
case "Code32":
encodeType = EncodeTypes.Code32;
break;
case "Code11":
encodeType = EncodeTypes.Code11;
break;
case "PDF417":
encodeType = EncodeTypes.Pdf417;
break;
case "EAN8":
encodeType = EncodeTypes.EAN8;
break;
case "QR":
encodeType = EncodeTypes.QR;
break;
}
}
// バーコードオブジェクトを初期化します
Barcode barcode = new Barcode();
barcode.Text = tbCodeText.Text;
barcode.BarcodeType = encodeType;
barcode.ImageType = imageFormat;
try
{
string imagePath = "";
if (cbGenerateWithOptions.IsChecked == true)
{
// 追加オプションでバーコードを生成し、画像パスを取得します
imagePath = GenerateBarcodeWithOptions(barcode);
}
else
{
// バーコードを生成し、画像パスを取得します
imagePath = GenerateBarcode(barcode);
}
// 画像を表示する
Uri fileUri = new Uri(Path.GetFullPath(imagePath));
imgDynamic.Source = new BitmapImage(fileUri);
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
- その後、バーコードを生成する関数を追加します。
private string GenerateBarcode(Barcode barcode)
{
// 画像パス
string imagePath = comboBarcodeType.Text + "." + barcode.ImageType;
// バーコードジェネレータを初期化する
BarcodeGenerator generator = new BarcodeGenerator(barcode.BarcodeType, barcode.Text);
// 画像を保存する
generator.Save(imagePath, barcode.ImageType);
return imagePath;
}
- 最後に、アプリケーションを実行します。
追加オプションでバーコードを生成
バーコードタイプに固有の追加オプションを使用してバーコードを生成することもできます。 WPFバーコードジェネレーターに、オプション付きのバーコードを生成するためのチェックボックスを追加しました。さまざまなバーコードタイプの追加オプションを指定して、次の関数を呼び出します。
private string GenerateBarcodeWithOptions(Barcode barcode)
{
// 画像パス
string imagePath = comboBarcodeType.Text + "." + barcode.ImageType;
// バーコードジェネレータを初期化する
BarcodeGenerator generator = new BarcodeGenerator(barcode.BarcodeType, barcode.Text);
if(barcode.BarcodeType == EncodeTypes.QR)
{
generator.Parameters.Barcode.XDimension.Pixels = 4;
//自動バージョンを設定する
generator.Parameters.Barcode.QR.QrVersion = QRVersion.Auto;
//自動QRエンコードタイプを設定する
generator.Parameters.Barcode.QR.QrEncodeType = QREncodeType.Auto;
}
else if(barcode.BarcodeType == EncodeTypes.Pdf417)
{
generator.Parameters.Barcode.XDimension.Pixels = 2;
generator.Parameters.Barcode.Pdf417.Columns = 3;
}
else if(barcode.BarcodeType == EncodeTypes.DataMatrix)
{
//DataMatrixECCを140に設定します
generator.Parameters.Barcode.DataMatrix.DataMatrixEcc = DataMatrixEccType.Ecc200;
}
else if(barcode.BarcodeType == EncodeTypes.Code32)
{
generator.Parameters.Barcode.XDimension.Millimeters = 1f;
}
else
{
generator.Parameters.Barcode.XDimension.Pixels = 2;
//BarHeight40を設定します
generator.Parameters.Barcode.BarHeight.Pixels = 40;
}
// 画像を保存する
generator.Save(imagePath, barcode.ImageType);
return imagePath;
}
ドキュメントでバーコードタイプの世代の詳細の詳細を読むことができます。
デモWPFバーコードジェネレーター
以下は、作成したばかりのWPFバーコードジェネレーターアプリケーションのデモンストレーションです。
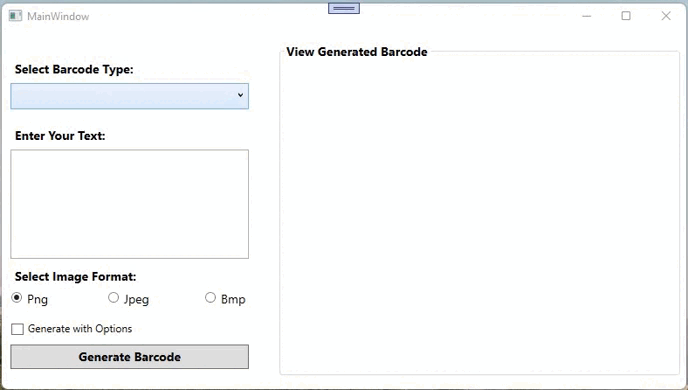
デモWPFバーコードジェネレーター
ソースコードをダウンロード
WPFバーコードジェネレータアプリケーションの完全なソースコードは、GitHubからダウンロードできます。
無料ライセンスを取得する
無料の一時ライセンスを取得して、評価の制限なしにライブラリを試すことができます。
結論
この記事では、WPFアプリケーションでさまざまな種類のバーコードを生成する方法を学びました。また、生成されたバーコード画像をプログラムでプレビューする方法も確認しました。さらに、ドキュメントを使用して、Aspose.BarCode for.NETAPIについて詳しく知ることができます。ご不明な点がございましたら、フォーラムまでお気軽にお問い合わせください。