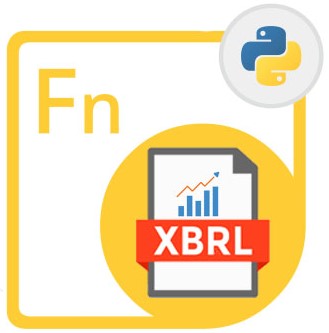
XBRLドキュメントは、ビジネスレポートを構成するファクトのコレクションです。プログラムでXBRLインスタンスドキュメントを簡単に作成し、スキーマ参照、コンテキスト、ユニット、アイテムなどのオブジェクトまたは要素を追加できます。この記事では、Pythonを使用してXBRLファイルを作成する方法を学習します。
この記事では、次のトピックについて説明します。
- XBRLとは
- XBRLファイルを作成するためのPythonファイナンスライブラリ
- XBRLファイルの作成
- XBRLにスキーマ参照を追加
- XBRLにコンテキストを追加
- XBRLでユニットを作成する
- XBRLにファクトアイテムを追加
- XBRLに脚注リンクを追加
- XBRLにロールリファレンスを挿入 10.アークロール参照をXBRLに追加
XBRLとは
XBRLは、XMLベースのマークアップ言語であるeXtensible BusinessReportingLanguageの略です。これは、企業の財務実績の標準化されたビジネスレポートに使用されます。これは、ビジネスシステム間でビジネス情報を通信および交換する方法を提供します。
XBRLファイルを作成するためのPythonファイナンスライブラリ
XBRLファイルまたはインスタンスドキュメントを作成するには、Aspose.Finance for PythonAPIを使用します。これにより、XBRLインスタンスの作成、解析、およびXBRLまたはiXBRLファイルの検証が可能になります。
APIは、1つ以上のXBRLインスタンスを含むXBRLドキュメントを表すXbrlDocumentクラスを提供します。 XBRLインスタンスはXMLフラグメントであり、ルート要素にはXBRLタグが付いています。 XbrlInstanceクラスは、XBRLインスタンスを操作するためのさまざまなメソッドとプロパティを提供します。 XbrlDocumentクラスのsave()メソッドは、XBRLファイルを作成してディスクに保存します。 APIリファレンスでXBRLオブジェクトを操作する他のクラスの詳細をお読みください。
パッケージをダウンロードするか、コンソールで次のpipコマンドを使用してPyPIからAPIをインストールしてください。
pip install aspose-finance
PythonでXBRLインスタンスドキュメントを作成する
以下の手順に従って、XBRLインスタンスドキュメントを最初から簡単に作成できます。
- まず、XbrlDocumentクラスのインスタンスを作成します。
- 次に、XbrlDocumentオブジェクトからxbrlinstancesオブジェクトにインスタンスのコレクションにアクセスします。
- その後、xbrlinstances.add()メソッドを使用して新しいXBRLインスタンスを追加します。
- 最後に、save(string)メソッドを使用してXBRLファイルを保存します。出力ファイルのパスを引数として取ります。
次のコードサンプルは、Pythonを使用してXBRLインスタンスを作成する方法を示しています。
# This code example demonstrates how to create an XBRL document
from aspose.finance.xbrl import XbrlDocument
# The path to the documents directory.
outputFile = "C:\\Files\\document.xbrl"
# Create XBRL document
xbrlDoc = XbrlDocument()
# Get document instances collection
xbrlInstances = xbrlDoc.xbrl_instances
# Add instance to the collection
xbrlInstance = xbrlInstances[xbrlInstances.add()]
# Save the file
xbrlDoc.save(outputFile)
XBRLのインスタンスを作成したら、新しく作成したXBRLインスタンスにスキーマ参照、コンテキスト、ユニット、アイテム、脚注リンク、役割参照、およびアーク役割参照を追加できます。
Pythonを使用してXBRLにスキーマ参照を追加する
以下の手順に従って、XBRLインスタンスドキュメントにスキーマ参照を追加できます。
- まず、XbrlDocumentクラスのインスタンスを作成します。
- 次に、XbrlDocumentオブジェクトのインスタンスのコレクションをxbrlinstancesオブジェクトに取得します。
- 次に、xbrlinstances.add()メソッドを使用して新しいXBRLインスタンスを追加します。
- 次に、XbrlInstanceオブジェクトのスキーマ参照コレクションをschemarefsオブジェクトに取得します。
- その後、入力スキーマファイルでschemarefs.add()メソッドを使用して新しいスキーマ参照を追加します。
- 最後に、save(string)メソッドを使用してXBRLファイルを保存します。
次のコードサンプルは、XBRLインスタンスドキュメントを作成し、Pythonでスキーマ参照を追加する方法を示しています。
# This code example demonstrates how to create an XBRL document and add schema reference object
from aspose.finance.xbrl import XbrlDocument
# The path to the documents directory.
outputFile = "C:\\Files\\document.xbrl"
inputSchemaFile = "C:\\Files\\schema.xsd"
# Create XBRL document
xbrlDoc = XbrlDocument()
# Get document instances collection
xbrlInstances = xbrlDoc.xbrl_instances
# Add instance to the collection
xbrlInstance = xbrlInstances[xbrlInstances.add()]
# Get instance schema references collection
schemaRefs = xbrlInstance.schema_refs
# Add schema
schemaRefs.add(inputSchemaFile, "example", "http://example.com/xbrl/taxonomy")
# Save the document
xbrlDoc.save(outputFile)
Pythonを使用してXBRLにコンテキストを追加する
以下の手順に従って、XBRLインスタンスドキュメントにコンテキストを追加できます。
- まず、XbrlDocumentクラスのインスタンスを作成します。
- 次に、新しいXBRLインスタンスをXbrlDocumentオブジェクトのxbrlinstancesに追加します。
- 次に、開始日と終了日を使用してContextPeriodクラスのインスタンスを作成します。
- 次に、スキーマと識別子を使用してContextEntityオブジェクトを作成します。
- 次に、定義されたContextPeriodとContextEntityを使用してContextクラスのインスタンスを作成します。
- その後、Contextオブジェクトをcontextsコレクションに追加します。
- 最後に、save(string)メソッドを使用してXBRLファイルを保存します。
次のコードサンプルは、XBRLインスタンスドキュメントを作成し、Pythonでコンテキストオブジェクトを追加する方法を示しています。
# This code example demonstrates how to create an XBRL document and add Context object
from datetime import datetime
from aspose.finance.xbrl import XbrlDocument,ContextPeriod,ContextEntity,Context
# The path to the documents directory.
outputFile = "C:\\Files\\document.xbrl"
# Create XBRL document
xbrlDoc = XbrlDocument()
# Get document instances collection
xbrlInstances = xbrlDoc.xbrl_instances
# Add instance to the collection
xbrlInstance = xbrlInstances[xbrlInstances.add()]
# Add context period
contextPeriod = ContextPeriod(datetime(2020,1,1), datetime(2020,2,10))
# Add context entity
contextEntity = ContextEntity("exampleIdentifierScheme", "exampleIdentifier")
# Define and add context
context = Context(contextPeriod, contextEntity)
xbrlInstance.contexts.append(context)
# Save the document
xbrlDoc.save(outputFile)
Pythonを使用してXBRLでユニットを作成する
XBRLの単位は数値アイテムを測定します。以下の手順に従って、XBRLインスタンスドキュメントにユニットを追加できます。
- まず、XbrlDocumentクラスのインスタンスを作成します。
- 次に、新しいXBRLインスタンスをXbrlDocumentオブジェクトのxbrlinstancesに追加します。
- 次に、UnitTypeをMEASUREとしてUnitクラスのインスタンスを作成します。
- 次に、QualifiedNameをmeasurequalifiednamesコレクションに追加します。
- その後、ユニットをユニットコレクションに追加します。
- 最後に、save(string)メソッドを使用してXBRLファイルを保存します。
次のコードサンプルは、XBRLファイルを作成してPythonでユニットオブジェクトを追加する方法を示しています。
# This code example demonstrates how to create an XBRL document and add Unit object
from aspose.finance.xbrl import XbrlDocument,Unit,QualifiedName,UnitType
# The path to the documents directory.
outputFile = "C:\\Files\\document.xbrl"
# Create XBRL document
xbrlDoc = XbrlDocument()
# Get document instances collection
xbrlInstances = xbrlDoc.xbrl_instances
# Add instance to the collection
xbrlInstance = xbrlInstances[xbrlInstances.add()]
# Add Unit
unit = Unit(UnitType.MEASURE)
unit.measure_qualified_names.append(QualifiedName("USD", "iso4217", "http://www.xbrl.org/2003/iso4217"))
xbrlInstance.units.append(unit)
# Save the document
xbrlDoc.save(outputFile)
Pythonを使用してXBRLにファクトアイテムを追加する
XBRLのファクトは、アイテム要素を使用して定義されます。 XBRLの項目には、単純なファクトの値と、そのファクトを正しく解釈するためのコンテキストへの参照が含まれています。以下の手順に従って、XBRLインスタンスドキュメントにアイテムを追加できます。
- まず、XbrlDocumentクラスのインスタンスを作成します。
- XbrlDocumentオブジェクトのxbrlinstancesに新しいXBRLインスタンスを追加します。
- XbrlInstanceオブジェクトのschemarefに新しいスキーマ参照を追加します。
- schemarefsからインデックスでSchemaRefを取得します。
- Contextインスタンスを初期化し、それをcontextsコレクションに追加します。
- Unitインスタンスを定義し、それをunitsコレクションに追加します。
- getconceptbyname()メソッドを使用してConceptクラスインスタンスを作成します。
- Conceptオブジェクトを引数としてItemクラスのインスタンスを作成します。
- contextref、unitref、precision、valueなどのアイテムプロパティを設定します。 10.その後、アイテムをファクトコレクションに追加します。 11.最後に、save(string)メソッドを使用してXBRLファイルを保存します。
次のコードサンプルは、Pythonを使用してXBRLインスタンスドキュメントにアイテム要素としてファクトを追加する方法を示しています。
# This code example demonstrates how to create an XBRL document and add Item object
from datetime import datetime
from aspose.finance.xbrl import XbrlDocument,ContextPeriod,ContextEntity,Context,Unit,UnitType,QualifiedName,Item
# The path to the documents directory.
outputFile = "C:\\Files\\document.xbrl"
inputSchemaFile = "C:\\Files\\schema.xsd"
# Create XBRL document
xbrlDoc = XbrlDocument()
# Get document instances collection
xbrlInstances = xbrlDoc.xbrl_instances
# Add instance to the collection
xbrlInstance = xbrlInstances[xbrlInstances.add()]
# Get instance schema references collection
schemaRefs = xbrlInstance.schema_refs
# Add schema
schemaRefs.add(inputSchemaFile, "example", "http://example.com/xbrl/taxonomy")
schema = schemaRefs[0]
# Add context
contextPeriod = ContextPeriod(datetime(2020,1,1), datetime(2020,2,10))
contextEntity = ContextEntity("exampleIdentifierScheme", "exampleIdentifier")
context = Context(contextPeriod, contextEntity)
xbrlInstance.contexts.append(context)
# Add unit
unit = Unit(UnitType.MEASURE)
unit.measure_qualified_names.append(QualifiedName("USD", "iso4217", "http://www.xbrl.org/2003/iso4217"))
xbrlInstance.units.append(unit)
# Add item
fixedAssetsConcept = schema.get_concept_by_name("fixedAssets")
if fixedAssetsConcept is not None:
item = Item(fixedAssetsConcept)
item.context_ref = context
item.unit_ref = unit
item.precision = 4
item.value = "1444"
xbrlInstance.facts.append(item)
# Save the document
xbrlDoc.save(outputFile)
PythonでXBRLに脚注リンクを追加する
以下の手順に従って、XBRLインスタンスドキュメントに脚注リンクを追加できます。
- まず、XbrlDocumentクラスのインスタンスを作成します。
- XbrlDocumentオブジェクトのxbrlinstancesに新しいXBRLインスタンスを追加します。
- XbrlInstanceオブジェクトのschemarefに新しいスキーマ参照を追加します。
- schemarefsからインデックスでSchemaRefを取得します。
- Contextインスタンスを初期化し、それをcontextsコレクションに追加します。
- Footnoteクラスインスタンスを定義し、そのラベルとテキストを設定します。
- Locクラスインスタンスを使用してロケータータイプを初期化します。
- 引数としてLocatorラベルとFootnoteラベルを使用してFootnoteArcオブジェクトを定義します。
- FootnoteLinkクラスのインスタンスを作成します。
- Footnote、Locator、およびFootnoteArcを関連するコレクションに追加します。 11.その後、FootnoteLinkをfootnotelinksコレクションに追加します。 12.最後に、save(string)メソッドを使用してXBRLファイルを保存します。
次のコードサンプルは、Pythonを使用してXBRLインスタンスドキュメントに脚注リンクを追加する方法を示しています。
# This code example demonstrates how to create an XBRL document and add Footnote Link object
from datetime import datetime
from aspose.finance.xbrl import XbrlDocument,ContextPeriod,ContextEntity,Context,FootnoteLink,Footnote,Loc,FootnoteArc
# The path to the documents directory.
outputFile = "C:\\Files\\document.xbrl"
inputSchemaFile = "C:\\Files\\schema.xsd"
# Create XBRL document
xbrlDoc = XbrlDocument()
# Get document instances collection
xbrlInstances = xbrlDoc.xbrl_instances
# Add instance to the collection
xbrlInstance = xbrlInstances[xbrlInstances.add()]
# Get instance schema references collection
schemaRefs = xbrlInstance.schema_refs
# Add schema
schemaRefs.add(inputSchemaFile, "example", "http://example.com/xbrl/taxonomy")
schema = schemaRefs[0]
# Add context
contextPeriod = ContextPeriod(datetime(2020,1,1), datetime(2020,2,10))
contextEntity = ContextEntity("exampleIdentifierScheme", "exampleIdentifier")
context = Context(contextPeriod, contextEntity)
xbrlInstance.contexts.append(context)
# Add Footnote
footnote = Footnote("footnote1")
footnote.text = "Including the effects of the merger."
# Add Location
loc = Loc("#cd1", "fact1")
# Define Footnote arc
footnoteArc = FootnoteArc(loc.label, footnote.label)
# Add FootnoteLink
footnoteLink = FootnoteLink()
footnoteLink.footnotes.append(footnote)
footnoteLink.locators.append(loc)
footnoteLink.footnote_arcs.append(footnoteArc)
xbrlInstance.footnote_links.append(footnoteLink)
# Save the document
xbrlDoc.save(outputFile)
Pythonを使用してXBRLにロール参照を挿入する
以下の手順に従って、XBRLインスタンスドキュメントにロール参照を追加できます。
- まず、XbrlDocumentクラスのインスタンスを作成します。
- XbrlDocumentオブジェクトのxbrlinstancesに新しいXBRLインスタンスを追加します。
- XbrlInstanceオブジェクトのschemarefに新しいスキーマ参照を追加します。
- schemarefsからインデックスでSchemaRefを取得します。
- 次に、getroletypebyuri()メソッドからRoleTypeを取得します。
- 次に、RoleTypeオブジェクトを引数としてRoleReferenceクラスのインスタンスを作成します。
- その後、RoleReferenceをrolereferencesコレクションに追加します。
- 最後に、save(string)メソッドを使用してXBRLファイルを保存します。
次のコードサンプルは、Pythonを使用してXBRLインスタンスドキュメントにロール参照を追加する方法を示しています。
# This code example demonstrates how to create an XBRL document and add Role reference object
from aspose.finance.xbrl import XbrlDocument,RoleReference
# The path to the documents directory.
outputFile = "C:\\Files\\document.xbrl"
inputSchemaFile = "C:\\Files\\schema.xsd"
# Create XBRL document
xbrlDoc = XbrlDocument()
# Get document instances collection
xbrlInstances = xbrlDoc.xbrl_instances
# Add instance to the collection
xbrlInstance = xbrlInstances[xbrlInstances.add()]
# Get instance schema references collection
schemaRefs = xbrlInstance.schema_refs
# Add schema
schemaRefs.add(inputSchemaFile, "example", "http://example.com/xbrl/taxonomy")
schema = schemaRefs[0]
# Get role type
roleType = schema.get_role_type_by_uri("http://abc.com/role/link1")
# Add role reference
if roleType is not None:
roleReference = RoleReference(roleType)
xbrlInstance.role_references.append(roleReference)
# Save the document
xbrlDoc.save(outputFile)
PythonでXBRLにアークロール参照を追加する
以下の手順に従って、XBRLインスタンスドキュメントにArcロール参照を追加できます。
- まず、XbrlDocumentクラスのインスタンスを作成します。
- XbrlDocumentオブジェクトのxbrlinstancesに新しいXBRLインスタンスを追加します。
- XbrlInstanceオブジェクトのschemarefに新しいスキーマ参照を追加します。
- schemarefsからインデックスでSchemaRefを取得します。
- 次に、getarcroletypebyuri()メソッドからRoleTypeを取得します。
- 次に、RoleTypeオブジェクトを引数としてArcroleReferenceクラスのインスタンスを作成します。
- その後、ArcroleReferenceをarcrolereferencesコレクションに追加します。
- 最後に、save(string)メソッドを使用してXBRLファイルを保存します。
次のコードサンプルは、Pythonを使用してXBRLインスタンスドキュメントにアークロール参照を追加する方法を示しています。
# This code example demonstrates how to create an XBRL document and add Arc Role reference object
from aspose.finance.xbrl import XbrlDocument,ArcroleReference
# The path to the documents directory.
outputFile = "C:\\Files\\document.xbrl"
inputSchemaFile = "C:\\Files\\schema.xsd"
# Create XBRL document
xbrlDoc = XbrlDocument()
# Get document instances collection
xbrlInstances = xbrlDoc.xbrl_instances
# Add instance to the collection
xbrlInstance = xbrlInstances[xbrlInstances.add()]
# Get instance schema references collection
schemaRefs = xbrlInstance.schema_refs
# Add schema
schemaRefs.add(inputSchemaFile, "example", "http://example.com/xbrl/taxonomy")
schema = schemaRefs[0]
# Get role type
roleType = schema.get_role_type_by_uri("http://abc.com/role/link1")
# Add role reference
if roleType is not None:
arcroleReference = ArcroleReference(roleType)
xbrlInstance.arcrole_references.append(arcroleReference)
# Save the document
xbrlDoc.save(outputFile)
無料ライセンスを取得する
無料の一時ライセンスを取得して、評価の制限なしにライブラリを試すことができます。
結論
この記事では、Pythonを使用してXBRLドキュメントを作成する方法を学びました。また、作成したXBRLインスタンスドキュメントにさまざまなXBRLオブジェクトをプログラムで追加する方法も確認しました。さらに、ドキュメントを使用して、Aspose.Finance forPythonAPIの詳細を学ぶことができます。あいまいな点がある場合は、フォーラムまでお気軽にお問い合わせください。