
画像編集は、画像の品質を向上させるために非常に使用されます。モバイル カメラと画像編集アプリの登場により、すべてのモバイル ユーザーは画像の編集方法を知っています。画像編集機能の中でも、複数の画像を 1 つの画像に結合するコラージュの作成は人気があります。そこでこの記事では、Python で複数の画像を結合する方法を説明します。これは、Python アプリケーションで画像編集を扱う場合に便利です。
画像を結合するための Python ライブラリ
複数の画像を 1 つの画像に結合するには、Aspose.Imaging for Python を使用します。これは、いくつかの画像編集操作を簡単に実行するための機能が豊富な画像処理ライブラリです。ライブラリをダウンロードするか、次のコマンドを使用してインストールできます。
> pip install aspose-imaging-python-net
Python で複数の画像を結合する
画像を結合するには、垂直方向と水平方向の 2 つの方法があります。適切な方法を選択できます。次のセクションでは、両方の方法で画像を結合する方法を見てみましょう。
Python で画像を水平方向に結合する
以下は、Aspose.Imaging for Python を使用して画像を水平方向に結合する手順です。
- まず、配列内の画像のパスを指定します。
- 次に、結果の画像の高さと幅を計算します。
- JpegOptions クラスのオブジェクトを作成し、必要なオプションを設定します。
- JpegImage クラスのオブジェクトを作成し、JpegOptions オブジェクトと結果の画像の高さと幅を使用して初期化します。
- 画像のリストをループし、RasterImage クラスを使用して各画像を読み込みます。
- 画像ごとに Rectangle を作成し、JpegImage.saveargb32pixels() メソッドを使用して結果の画像に追加します。
- 反復ごとにステッチ幅を増やします。
- 最後に、JpegImage.save(string) メソッドを使用して、結果の画像を保存します。
以下は、Python で画像を水平方向に結合するコードです。
import aspose.pycore as aspycore
from aspose.imaging import Image, Rectangle, RasterImage
from aspose.imaging.imageoptions import JpegOptions
from aspose.imaging.sources import FileCreateSource
from aspose.imaging.fileformats.jpeg import JpegImage
import os
# フォルダーを定義する
if 'TEMPLATE_DIR' in os.environ:
templates_folder = os.environ['TEMPLATE_DIR']
else:
templates_folder = r"C:\Users\USER\Downloads\templates"
delete_output = 'SAVE_OUTPUT' not in os.environ
data_dir = templates_folder
image_paths = [os.path.join(data_dir, "template.jpg"),
os.path.join(data_dir, "template.jpeg")]
output_path = os.path.join(data_dir, "result.jpg")
temp_file_path = os.path.join(data_dir, "temp.jpg")
# 結果の画像サイズを取得する
image_sizes = []
for image_path in image_paths:
with Image.load(image_path) as image:
image_sizes.append(image.size)
# 新しいサイズを計算する
new_width = 0
new_height = 0
for size in image_sizes:
new_width += size.width
new_height = max(new_height, size.height)
# 画像を新しい画像に結合する
temp_file_source = FileCreateSource(temp_file_path, delete_output)
with JpegOptions() as options:
options.source = temp_file_source
options.quality = 100
with aspycore.as_of(Image.create(options, new_width, new_height), JpegImage) as new_image:
stitched_width = 0
for image_path in image_paths:
with aspycore.as_of(Image.load(image_path), RasterImage) as image:
bounds = Rectangle(stitched_width, 0, image.width, image.height)
new_image.save_argb_32_pixels(bounds, image.load_argb_32_pixels(image.bounds))
stitched_width += image.width
new_image.save(output_path)
# 一時ファイルを削除する
if delete_output:
os.remove(output_path)
if os.path.exists(temp_file_path):
os.remove(temp_file_path)
以下は、画像を水平方向に結合した後に得られた出力画像です。

Python で画像を垂直方向に結合する
複数の画像を垂直方向に結合する方法を見てみましょう。画像を垂直方向に結合する手順は、前のセクションと同じです。唯一の違いは、高さと幅のプロパティの役割を交換することです。
次のコード サンプルは、Python で垂直レイアウトで画像を結合する方法を示しています。
import aspose.pycore as aspycore
from aspose.imaging import Image, Rectangle, RasterImage
from aspose.imaging.imageoptions import JpegOptions
from aspose.imaging.sources import StreamSource
from aspose.imaging.fileformats.jpeg import JpegImage
from aspose.imaging.extensions import StreamExtensions
import os
import functools
# フォルダーを定義する
if 'TEMPLATE_DIR' in os.environ:
templates_folder = os.environ['TEMPLATE_DIR']
else:
templates_folder = r"C:\Users\USER\Downloads\templates"
delete_output = 'SAVE_OUTPUT' not in os.environ
data_dir = templates_folder
image_paths = [os.path.join(data_dir, "template.jpg"), os.path.join(data_dir, "template.jpeg")]
output_path = os.path.join(data_dir, "result.jpg")
temp_file_path = os.path.join(data_dir, "temp.jpg")
# 結果の画像サイズを取得する
image_sizes = []
for image_path in image_paths:
with Image.load(image_path) as image:
image_sizes.append(image.size)
# 新しいサイズを計算する
new_width = 0
new_height = 0
for size in image_sizes:
new_height += size.height
new_width = max(new_width, size.width)
# 画像を新しい画像に結合する
with StreamExtensions.create_memory_stream() as memory_stream:
output_stream_source = StreamSource(memory_stream)
with JpegOptions() as options:
options.source = output_stream_source
options.quality = 100
with aspycore.as_of(Image.create(options, new_width, new_height), JpegImage) as new_image:
stitched_height = 0
for image_path in image_paths:
with aspycore.as_of(Image.load(image_path), RasterImage) as image:
bounds = Rectangle(0, stitched_height, image.width, image.height)
new_image.save_argb_32_pixels(bounds, image.load_argb_32_pixels(image.bounds))
stitched_height += image.height
new_image.save(output_path)
# 一時ファイルを削除する
if delete_output:
os.remove(output_path)
次の画像は、2 つの類似した画像を垂直方向に結合した出力を示しています。

Python で PNG 画像を結合する
前のセクションで説明した手順とコード サンプルは JPG 画像を結合するためのものでしたが、PNG 画像も結合する必要がある場合があります。心配する必要はありません。同じ手順とコード サンプルは PNG 画像にも有効で、唯一の変更点は、それぞれ JpegImage クラスと JpegOptions クラスの代わりに PngImage クラスと PngOptions クラスを使用することです。
無料の Python 画像結合ライブラリを入手
無料の一時ライセンスを取得して、評価制限なしで画像を結合できます。
オンラインで画像を結合
無料の画像結合ツール を使用して、オンラインで画像を結合することもできます。このツールは Aspose.Imaging for Python に基づいており、アカウントを作成する必要はありません。
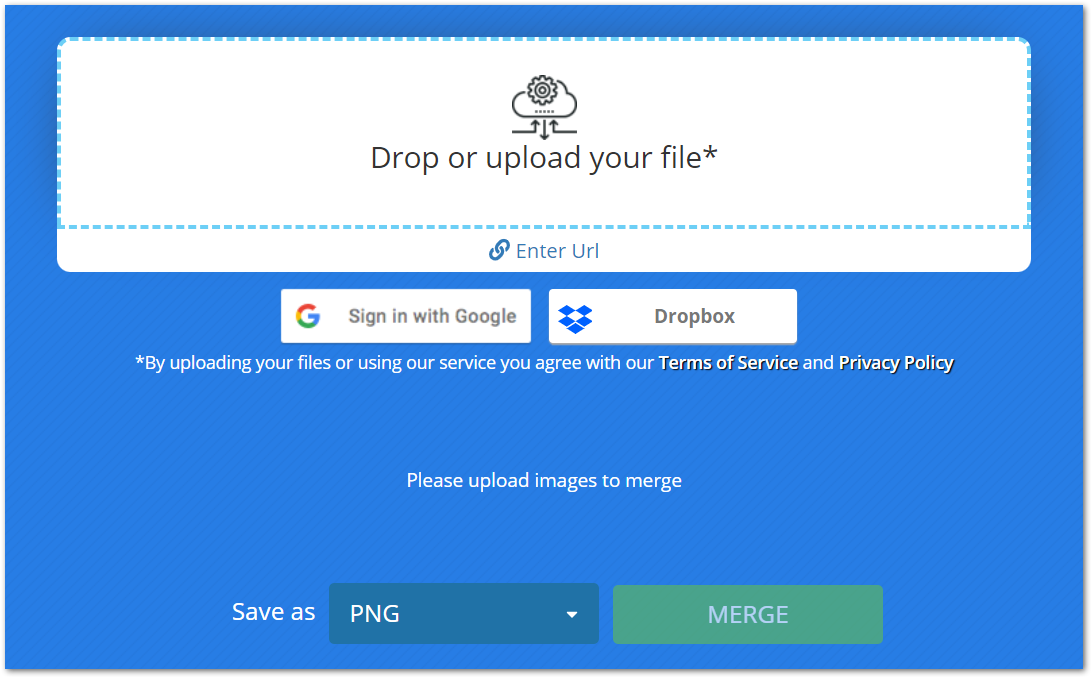
結論
この記事では、Python で複数の画像を 1 つの画像に結合するための最も簡単なソリューションの 1 つを説明しました。水平方向と垂直方向の画像の結合は、コード サンプルを使用してデモンストレーションされます。さらに、無料で画像を結合できるオンライン ツールも紹介しました。
Python 画像処理ライブラリについて詳しくは、ドキュメント を参照してください。また、フォーラム を通じて質問を共有することもできます。