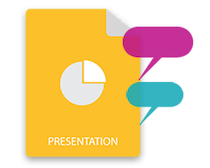
多くの場合、プレゼンテーションの内容は、フィードバックを得るために他の人がレビューする必要があります。 PowerPointでは、コメントは、スライド上の特定の単語、フレーズ、または何かに関するフィードバックを書き込むために使用されます。この記事では、PythonでプログラムによってPowerPointPPTスライドにコメントを追加する方法について説明します。さらに、既存のコメントを読み、返信を追加する方法を学びます。
PowerPointPPTにコメントを追加するPythonライブラリ
コメントとその返信を追加して読むには、Aspose.Slides for Python via .NETを使用します。ライブラリは、リッチなPowerPointプレゼンテーションを最初から作成するように設計されています。さらに、既存のプレゼンテーションをシームレスに操作できます。次のコマンドを使用して、PyPIからアプリケーションにライブラリをインストールできます。
> pip install aspose.slides
PythonでPPTスライドにコメントを追加する
PowerPointプレゼンテーションでは、コメントは作成者に関連付けられています。一方、各コメントには、作成時間、追加されたスライド、位置などの追加情報が含まれています。以下は、Pythonを使用してPPTのスライドにコメントを追加する手順です。
- まず、プレゼンテーションファイルをロードするか、Presentationクラスを使用して新しいファイルを作成します。
- 次に、新しいスライドを追加するか、Presentation.slidesコレクションから既存のスライドの参照を取得します。
- Presentation.comment \ authors.add \ author(string, string)メソッドを使用して、authorsコレクションに新しい作成者を追加します。
- オブジェクト内に新しく作成された作成者の参照を取得します。
- コメントの位置を定義します。
- Author.comments.add \ comment(string, ISlide、point, date)メソッドを使用してコメントを追加します。
- 最後に、Presentation.save(string, SaveFormat)メソッドを使用してプレゼンテーションを保存します。
次のコードサンプルは、PythonでPPTスライドにコメントを追加する方法を示しています。
import aspose.slides as slides
import aspose.pydrawing as drawing
import datetime
# Create presentation
with slides.Presentation() as presentation:
# Add empty slide
presentation.slides.add_empty_slide(presentation.layout_slides[0])
# Add author
author = presentation.comment_authors.add_author("Usman", "MF")
# Set position of comment
point = drawing.PointF(0.2, 0.2)
# Add slide comment for an author on slide 1
author.comments.add_comment("Hello, this is slide comment", presentation.slides[0], point, datetime.date.today())
# Add slide comment for an author on slide 1
author.comments.add_comment("Hello, this is second slide comment", presentation.slides[1], point, datetime.date.today())
# Save presentation
presentation.save("ppt-comments.pptx", slides.export.SaveFormat.PPTX)
以下は、コメントを追加した後に表示されるスライドのスクリーンショットです。
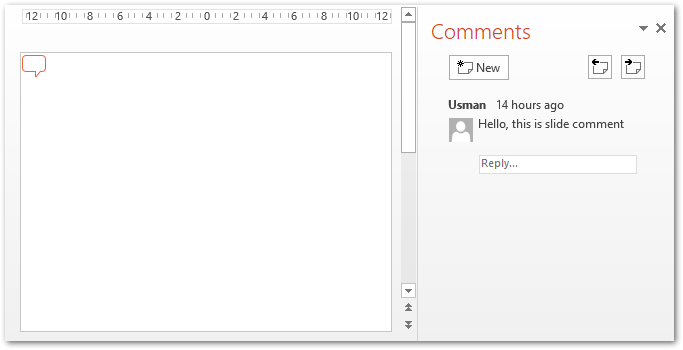
PythonのPPTスライドにコメント返信を追加する
Aspose.Slidesを使用すると、コメントに返信を追加することもできます。返信自体は、既存のコメントに関連付けられているコメントです。それでは、PythonのPowerPointPPTスライドのコメントに返信を追加する方法を見てみましょう。
- まず、プレゼンテーションファイルをロードするか、Presentationクラスを使用して新しいファイルを作成します。
- 次に、新しいスライドを追加するか、Presentation.slidesコレクションから既存のスライドの参照を取得します。
- 新しい作成者を追加し、その参照をオブジェクトで取得します。
- Author.comments.add \ comment(string, ISlide、point, date)メソッドを使用してコメントを挿入し、返されたオブジェクトを取得します。
- 同じ方法で別のコメントを挿入し、その参照をオブジェクトで取得します。
- parent \ commentプロパティを使用して、2番目のコメントの親を設定します。
- 最後に、Presentation.save(string, SaveFormat)メソッドを使用してプレゼンテーションを保存します。
次のコードサンプルは、PythonでPPTXプレゼンテーションのコメントに返信を追加する方法を示しています。
import aspose.slides as slides
import aspose.pydrawing as drawing
import datetime
# Create or load presentation
with slides.Presentation() as presentation:
# Add empty slide
presentation.slides.add_empty_slide(presentation.layout_slides[0])
# Add author and comment
author = presentation.comment_authors.add_author("Usman", "MF")
comment = author.comments.add_comment("Hello, this is slide comment.", presentation.slides[0], drawing.PointF(0.2, 0.2), datetime.date.today())
# Add reply comment
reply = author.comments.add_comment("This is the reply to the comment.", presentation.slides[0], drawing.PointF(0.2, 0.2), datetime.date.today())
reply.parent_comment = comment
# Add reply comment
reply2 = author.comments.add_comment("This is second reply.", presentation.slides[0], drawing.PointF(0.2, 0.2), datetime.date.today())
reply2.parent_comment = comment
# Save presentation
presentation.save("ppt-comments.pptx", slides.export.SaveFormat.PPTX)
次のスクリーンショットは、上記のコードサンプルの出力を示しています。
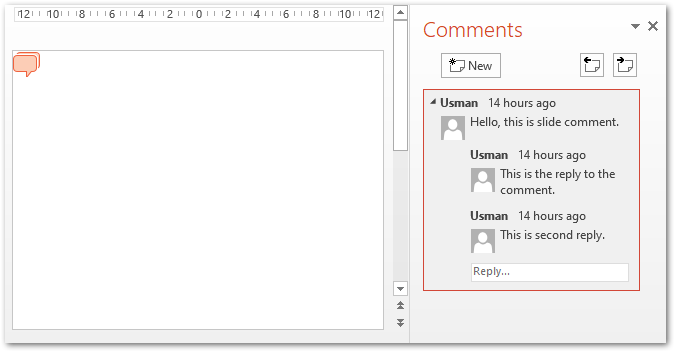
PythonのPPTスライドのコメントを読む
特定の作成者またはすべての作成者によって追加されたPPTスライドからコメントを読むこともできます。以下は、PythonのPPTスライドのコメントを読むための手順です。
- Presentationクラスを使用してプレゼンテーションファイルをロードします。
- Presentation.comment\authorsコレクションを使用して作成者のリストをループします。
- 著者ごとに、Author.commentsプロパティを使用してコメントをループします。
- そのプロパティを使用してコメントを読みます。
次のコードサンプルは、PythonのPPTスライドのコメントを読み取る方法を示しています。
import aspose.slides as slides
import aspose.pydrawing as drawing
import datetime
with slides.Presentation("ppt-comments.pptx") as presentation:
# Loop through authors
for author in presentation.comment_authors:
# Loop through comments
for comment in author.comments:
print("ISlide :" + str(comment.slide.slide_number) +
" has comment: " + comment.text +
" with Author: " + comment.author.name +
" posted on time :" + str(comment.created_time) + "\n")
無料ライセンスを取得する
一時ライセンスをリクエストすることで、評価の制限なしに.NET経由でAspose.Slides for Pythonを使用できます。
結論
この記事では、PythonのPowerPointPPTスライドにコメントを追加する方法を学びました。さらに、プログラムでPowerPointプレゼンテーションに返信を追加したりコメントを読んだりする方法についても説明しました。さらに、ドキュメントにアクセスして、.NETを介したAspose.Slides for Pythonの詳細を確認できます。また、フォーラムにクエリを投稿することもできます。