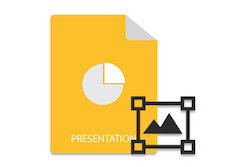
透かしは、機密、ドラフトなどのテキストでドキュメントの状態を識別し、元のドキュメントのコピーを困難にします。透かしは、会社名またはロゴを表示してドキュメントの所有権を指定するためにも使用されます。 PowerPointファイルの透かしは、画像ベースまたはテキストベースの両方にすることができます。この記事では、C++を使用してPowerPointスライドにテキストと画像の透かしを追加する方法を学習します。
PowerPointスライドに透かしを追加するためのC++API
Aspose.Slides for C++ APIを使用して、PowerPointスライドに透かしを追加します。これは、追加のソフトウェアを必要とせずにPowerPointファイルの作成、読み取り、および更新をサポートする、堅牢で機能豊富なAPIです。 APIは、NuGetからインストールするか、ダウンロードセクションから直接ダウンロードできます。
PM> Install-Package Aspose.Slides.Cpp
C++を使用してPowerPointスライドにテキスト透かしを追加する
以下の手順に従って、PowerPointスライドにテキスト透かしを追加できます。
- まず、Presentationクラスを使用してPowerPointファイルをロードします。
- マスタースライドを取得します。
- 透かしの位置を計算します。
- IMasterSlide->getShapes()->AddAutoShape(ShapeType shapeType, float x, float y, float width, float height)メソッドを使用して形状を追加します。
- IAutoShape->AddTextFrame(System::String text)メソッドを使用して、図形にテキストフレームを追加します。
- 透かしのフォントサイズ、色、回転角度を設定します。
- 透かしをロックして、移動または削除できないようにします。
- 最後に、Presentation->Save(System::String fname, Export::SaveFormat format)メソッドを使用してPowerPointファイルを保存します。
次のサンプルコードは、C++を使用してPowerPointスライドにテキスト透かしを追加する方法を示しています。
// ファイルパス
const String sourceFilePath = u"SourceDirectory\\Slides\\Presentation2.pptx";
const String outputFilePath = u"OutputDirectory\\AddTextWatermark_out.pptx";
// プレゼンテーションファイルをロードする
auto presentation = System::MakeObject<Presentation>(sourceFilePath);
// マスタースライドにアクセスする
auto master = presentation->get_Masters()->idx_get(0);
System::Drawing::PointF center(presentation->get_SlideSize()->get_Size().get_Width() / 2, presentation->get_SlideSize()->get_Size().get_Height() / 2);
float width = 300.0f;
float height = 300.0f;
float x = center.get_X() - width / 2;
float y = center.get_Y() - height / 2;
// 形を追加する
auto watermarkShape = master->get_Shapes()->AddAutoShape(ShapeType::Rectangle, x, y, width, height);
// 塗りつぶしタイプを設定
watermarkShape->get_FillFormat()->set_FillType(FillType::NoFill);
watermarkShape->get_LineFormat()->get_FillFormat()->set_FillType(FillType::NoFill);
// 回転角を設定する
watermarkShape->set_Rotation(-45);
// テキストを設定する
auto watermarkTextFrame = watermarkShape->AddTextFrame(u"Watermark");
// フォントと色を設定する
auto watermarkPortion = watermarkTextFrame->get_Paragraphs()->idx_get(0)->get_Portions()->idx_get(0);
watermarkPortion->get_PortionFormat()->set_FontHeight(52.0f);
int32_t alpha = 150, red = 200, green = 200, blue = 200;
watermarkPortion->get_PortionFormat()->get_FillFormat()->set_FillType(FillType::Solid);
watermarkPortion->get_PortionFormat()->get_FillFormat()->get_SolidFillColor()->set_Color(System::Drawing::Color::FromArgb(alpha, red, green, blue));
// 図形を変更できないようにロックする
watermarkShape->get_AutoShapeLock()->set_SelectLocked(true);
watermarkShape->get_AutoShapeLock()->set_SizeLocked(true);
watermarkShape->get_AutoShapeLock()->set_TextLocked(true);
watermarkShape->get_AutoShapeLock()->set_PositionLocked(true);
watermarkShape->get_AutoShapeLock()->set_GroupingLocked(true);
// プレゼンテーションを保存
presentation->Save(outputFilePath, Aspose::Slides::Export::SaveFormat::Pptx);
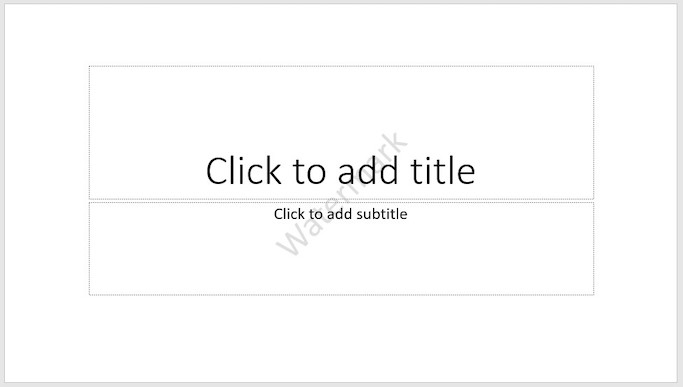
サンプルコードによって生成された出力の画像
C++を使用してPowerPointスライドに画像透かしを追加する
PowerPointスライドに画像透かしを追加するには、以下の手順に従ってください。
- まず、Presentationクラスを使用してPowerPointファイルをロードします。
- マスタースライドを取得します。
- 透かしの位置を計算します。
- IMasterSlide->getShapes()->AddAutoShape(ShapeType shapeType, float x, float y, float width, float height)メソッドを使用して形状を追加します。
- 画像を追加し、IPPImageオブジェクトでその参照を取得します。
- IAutoShape->get_FillFormat()->get_PictureFillFormat()->get_Picture()->setImage(System::SharedPtr value)メソッド。
- IAutoShapeの塗りつぶしタイプと画像塗りつぶしモードをそれぞれFillType::PictureとPictureFillMode::Stretchに設定します。
- 透かしをロックして、移動または削除できないようにします。
- 最後に、Presentation->Save(System::String fname, Export::SaveFormat format)メソッドを使用してPowerPointファイルを保存します。
次のサンプルコードは、C++を使用してPowerPointスライドに画像透かしを追加する方法を示しています。
// ファイルパス
const String sourceFilePath = u"SourceDirectory\\Slides\\Presentation2.pptx";
const String outputFilePath = u"OutputDirectory\\AddImageWatermark_out.pptx";
// プレゼンテーションファイルをロードする
auto presentation = System::MakeObject<Presentation>(sourceFilePath);
// メーザースライドにアクセスする
auto master = presentation->get_Masters()->idx_get(0);
System::Drawing::PointF center(presentation->get_SlideSize()->get_Size().get_Width() / 2, presentation->get_SlideSize()->get_Size().get_Height() / 2);
float width = 300.0f;
float height = 300.0f;
float x = center.get_X() - width / 2;
float y = center.get_Y() - height / 2;
// 形を追加する
auto watermarkShape = master->get_Shapes()->AddAutoShape(ShapeType::Rectangle, x, y, width, height);
auto image = presentation->get_Images()->AddImage(File::ReadAllBytes(u"SourceDirectory\\Images\\AsposeLogo.png"));
// 塗りつぶしタイプを設定
watermarkShape->get_FillFormat()->set_FillType(FillType::Picture);
watermarkShape->get_FillFormat()->get_PictureFillFormat()->get_Picture()->set_Image(image);
watermarkShape->get_FillFormat()->get_PictureFillFormat()->set_PictureFillMode(PictureFillMode::Stretch);
// 図形を変更できないようにロックする
watermarkShape->get_AutoShapeLock()->set_SelectLocked(true);
watermarkShape->get_AutoShapeLock()->set_SizeLocked(true);
watermarkShape->get_AutoShapeLock()->set_TextLocked(true);
watermarkShape->get_AutoShapeLock()->set_PositionLocked(true);
watermarkShape->get_AutoShapeLock()->set_GroupingLocked(true);
// プレゼンテーションを保存
presentation->Save(outputFilePath, Aspose::Slides::Export::SaveFormat::Pptx);
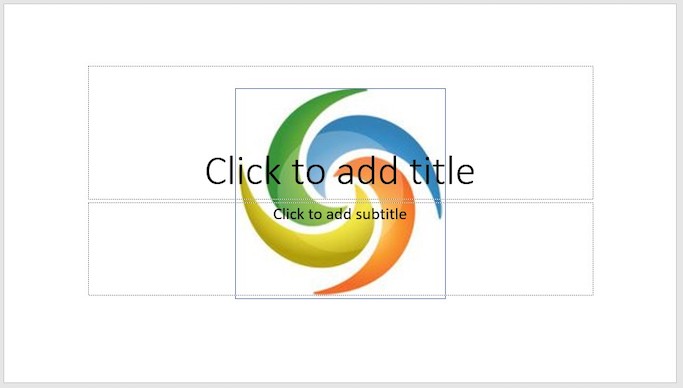
サンプルコードによって生成された出力の画像
無料ライセンスを取得する
評価制限なしでAPIを試すために、無料の一時ライセンスをリクエストできます。
結論
この記事では、C++を使用してPowerPointスライドに透かしを追加する方法を学習しました。共有コードサンプルは、Aspose.Slides to C++ APIを使用して、PowerPointスライドに画像とテキストの透かしを簡単に追加する方法を示しています。これは、PowerPoint PPTX/PPTファイルを操作するための一連の追加機能を提供する強力なAPIです。 公式ドキュメントにアクセスすると、APIの詳細を調べることができます。ご不明な点がございましたら、無料サポートフォーラムまでお気軽にお問い合わせください。
関連項目
情報:Aspose.Slidesの無料のPowerPointに透かしを追加およびPowerPointから透かしを削除オンラインツールを確認することをお勧めします。