SmartArtは、PowerPointプレゼンテーションのエクスペリエンスを向上させ、データを視覚的に表示するために使用されます。テキストをより目立たせて魅力的にするために使用できます。また、フロー図、プロセス、関係図などを表示するためにも使用できます。この記事では、C++を使用してPowerPointプレゼンテーションでSmartArtを作成する方法を学習します。
- PowerPointプレゼンテーションでSmartArtを作成するためのC++API
- C++を使用してPowerPointでSmartArtシェイプを作成する
- PowerPointプレゼンテーションでSmartArtシェイプにアクセスする
- C++を使用してSmartArtシェイプのスタイルを変更する
PowerPointプレゼンテーションでSmartArtを作成するためのC++API
Aspose.Slides for C++は、PowerPointファイルを操作するためのC++APIです。これにより、追加のソフトウェアを必要とせずに、PPTおよびPPTXファイルを作成、読み取り、および変更できます。さらに、APIはPowerPointプレゼンテーションでのSmartArtの作成をサポートしています。 APIは、NuGetからインストールするか、ダウンロードセクションから直接ダウンロードできます。
PM> Install-Package Aspose.Slides.Cpp
C++を使用してPowerPointでSmartArtシェイプを作成する
以下は、PowerPointプレゼンテーションでSmartArt図形を作成する手順です。
- Presentationクラスのインスタンスを作成して、新しいPowerPointファイルを表します。
- 目的のスライドを取得します。
- ISlide->get_Shapes()->AddSmartArt(float x, float y, float width, float height, SmartArt::SmartArtLayoutType layoutType)メソッドを使用してSmartArtを追加します。
- Presentation->Save(System::String fname, Export::SaveFormat format)メソッドを使用してプレゼンテーションを保存します。
次のサンプルコードは、C++を使用してPowerPointでSmartArt図形を作成する方法を示しています。
// ファイルパス
const String outputFilePath = u"OutputDirectory\\CreateSmartArt_out.pptx";
// プレゼンテーションファイルをロードする
SharedPtr<Presentation> presentation = MakeObject<Presentation>();
// 最初のスライドを取得する
SharedPtr<ISlide> slide = presentation->get_Slides()->idx_get(0);
// SmartArtを追加する
auto smartArt = slide->get_Shapes()->AddSmartArt(0, 0, 400, 400, Aspose::Slides::SmartArt::SmartArtLayoutType::BasicBlockList);
smartArt->get_AllNodes()->idx_get(0)->get_TextFrame()->set_Text(u"First Block");
smartArt->get_AllNodes()->idx_get(1)->get_TextFrame()->set_Text(u"Second Block");
// プレゼンテーションを保存
presentation->Save(outputFilePath, Aspose::Slides::Export::SaveFormat::Pptx);
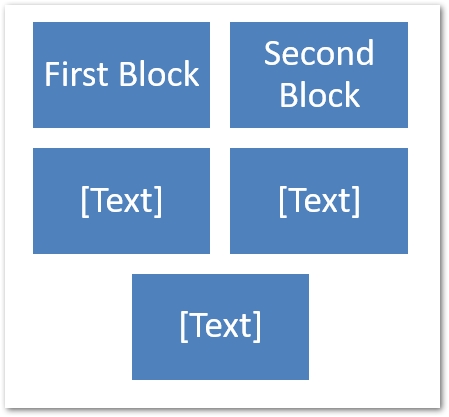
サンプルコードによって生成された出力の画像
PowerPointプレゼンテーションでSmartArtシェイプにアクセスする
以下の手順に従って、PowerPointファイルのSmartArt図形にアクセスできます。
- Presentationクラスを使用してPowerPointファイルをロードします。
- 図形をループします。
- 形状のタイプがISmartArtの場合、その参照をISmartArtオブジェクトとして取得します。
次のサンプルコードは、C++を使用してPowerPointプレゼンテーションからSmartArt図形にアクセスする方法を示しています。
// ファイルパス
const String sourceFilePath = u"OutputDirectory\\CreateSmartArt_out.pptx";
// プレゼンテーションファイルをロードする
SharedPtr<Presentation> presentation = MakeObject<Presentation>(sourceFilePath);
// 図形をループする
for (auto shape : presentation->get_Slides()->idx_get(0)->get_Shapes())
{
// 形状がSmartArtタイプかどうかを確認します
if (System::ObjectExt::Is<Aspose::Slides::SmartArt::SmartArt>(shape))
{
// SmartArtへのタイプキャストシェイプ
auto smartArt = System::DynamicCast_noexcept<ISmartArt>(shape);
Console::WriteLine(String::Format(u"Shape Name: {0}", smartArt->get_Name()));
// SmartArtレイアウトの確認
/*if (smartArt->get_Layout() == SmartArtLayoutType::BasicBlockList)
{
Console::WriteLine(u"Do some thing here....");
}*/
}
}
C++を使用してSmartArtシェイプのスタイルを変更する
SmartArt図形にアクセスした後、その図形のスタイルを簡単に変更できます。以下は、C++を使用してSmartArt図形のスタイルを変更する手順です。
- Presentationクラスを使用してPowerPointファイルをロードします。
- 図形をループします。
- 形状のタイプがISmartArtの場合、その参照をISmartArtオブジェクトとして取得します。
- ISmartArt->set_ColorStyle(SmartArtColorType value)およびISmartArt->set_QuickStyle(SmartArtQuickStyleType value)メソッドを使用してスタイルを変更します。
- Presentation->Save(System::String fname, Export::SaveFormat format)メソッドを使用してプレゼンテーションを保存します。
次のサンプルコードは、C++を使用してSmartArt図形のスタイルを変更する方法を示しています。
// ファイルパス
const String sourceFilePath = u"OutputDirectory\\CreateSmartArt_out.pptx";
const String outputFilePath = u"OutputDirectory\\ChangeSmartArt_out.pptx";
// プレゼンテーションファイルをロードする
SharedPtr<Presentation> presentation = MakeObject<Presentation>(sourceFilePath);
// 図形をループする
for (auto shape : presentation->get_Slides()->idx_get(0)->get_Shapes())
{
// 形状がSmartArtタイプかどうかを確認します
if (System::ObjectExt::Is<Aspose::Slides::SmartArt::SmartArt>(shape))
{
// SmartArtへのタイプキャストシェイプ
auto smartArt = System::DynamicCast_noexcept<ISmartArt>(shape);
// SmartArtスタイルを確認する
if (smartArt->get_QuickStyle() == SmartArtQuickStyleType::SimpleFill) {
// SmartArtスタイルを変更する
smartArt->set_QuickStyle(SmartArtQuickStyleType::Cartoon);
}
// SmartArtカラータイプを確認してください
if (smartArt->get_ColorStyle() == SmartArtColorType::ColoredFillAccent1) {
// SmartArtの色の種類を変更する
smartArt->set_ColorStyle(SmartArtColorType::ColorfulAccentColors);
}
}
}
// プレゼンテーションを保存
presentation->Save(outputFilePath, Aspose::Slides::Export::SaveFormat::Pptx);
無料ライセンスを取得する
評価制限なしでAPIを試すために、無料の一時ライセンスをリクエストできます。
結論
この記事では、C++を使用してPowerPointプレゼンテーションでSmartArt図形を作成する方法を学習しました。さらに、Aspose.Slides for C++ APIを使用して、SmartArt図形にアクセスしてスタイルを変更する方法を見てきました。これは、PowerPointファイルを操作するための多くの追加機能を提供する堅牢で機能豊富なAPIです。 公式ドキュメントにアクセスすると、APIの詳細を調べることができます。ご不明な点がございましたら、無料サポートフォーラムまでお気軽にお問い合わせください。