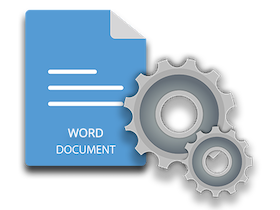
MS Wordファイルは、請求書、レポート、技術記事などのさまざまな種類のドキュメントを作成するために非常に使用されます。ドキュメントの自動化により、ユーザーはWebまたはデスクトップポータル内から動的にWordドキュメントを生成できます。したがって、この記事では、MSOfficeを使用せずにPythonでWord文書を生成する方法について説明します。さらに、DOCXまたはDOCファイルを作成し、Pythonを使用してテキストやその他の要素を動的に追加する方法を学習します。
- Wordドキュメントを作成するPythonAPI
- PythonでWordDOCX/DOCファイルを作成する
- 既存のWord文書を読み込む
- Word文書に段落を挿入する
- Word文書に表を追加する
- WordのDOCX/DOCファイルにリストを追加する
- Word文書に画像を挿入する
- Wordファイルに目次を追加する
Wordドキュメントを作成するPythonAPI
Word文書を動的に作成するために、Aspose.Words for Pythonを使用します。これは、MSWordドキュメントをシームレスに作成および操作できる強力なPythonライブラリです。次のpipコマンドを使用して、PyPIからPythonアプリケーションにライブラリをインストールできます。
pip install aspose-words
PythonでWordDOCXまたはDOCファイルを作成する
以下は、PythonでWord文書を最初から作成する手順です。
- Documentクラスのオブジェクトを作成します。
- DocumentBuilderクラスのオブジェクトを作成します。
- DocumentBuilder.write()メソッドを使用してドキュメントにテキストを挿入します。
- Document.save()メソッドを使用してWord文書を保存します。
次のコードサンプルは、WordDOCXドキュメントを作成する方法を示しています。
import aspose.words as aw
# create document object
doc = aw.Document()
# create a document builder object
builder = aw.DocumentBuilder(doc)
# add text to the document
builder.write("Hello world!")
# save document
doc.save("out.docx")
出力
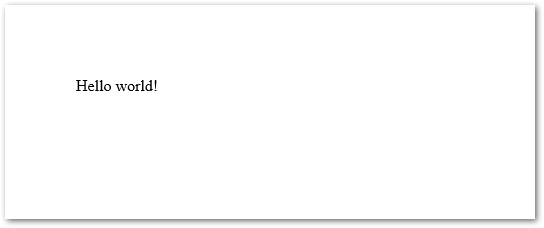
Pythonで既存のWord文書を読み込む
ドキュメントコンストラクターへのパスを指定して、既存のWordドキュメントを読み込むこともできます。次のコードサンプルは、Word文書をロードする方法を示しています。
import aspose.words as aw
# create document object
doc = aw.Document("document.docx")
# manipulate document
# save document
doc.save("out.docx")
Pythonを使用してWord文書に段落を挿入する
Word文書には、段落形式のテキストが含まれています。したがって、このセクションでは、Pythonを使用してWord文書に段落を挿入する方法を示します。
- Documentクラスのオブジェクトを作成します。
- DocumentBuilderクラスのオブジェクトを作成します。
- Documentbuilderオブジェクトからフォントの参照を取得し、フォントを設定します。
- Documentbuilderオブジェクトから段落fromatの参照を取得し、インデント、配置などを設定します。
- DocumentBuilder.write()メソッドを使用して段落にテキストを挿入します。
- Document.save()メソッドを使用してWord文書を保存します。
次のコードサンプルは、Pythonを使用してWord文書に段落を挿入する方法を示しています。
import aspose.words as aw
# create document object
doc = aw.Document()
# create a document builder object
builder = aw.DocumentBuilder(doc)
# create font
font = builder.font
font.size = 16
font.bold = True
font.name = "Arial"
font.underline = aw.Underline.DASH
# set paragraph formatting
paragraphFormat = builder.paragraph_format
paragraphFormat.first_line_indent = 8
paragraphFormat.alignment = aw.ParagraphAlignment.JUSTIFY
paragraphFormat.keep_together = True
# add text
builder.writeln("A whole paragraph.")
# save document
doc.save("out.docx")
出力
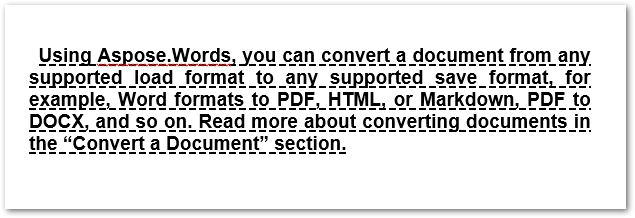
Pythonを使用したWord文書の段落の操作の詳細をご覧ください。
Pythonを使用してWord文書にテーブルを追加する
テーブルは、行と列の形式で情報を表示するためのドキュメントの不可欠な部分です。 Aspose.Words for Pythonを使用すると、テーブルの操作が非常に簡単になります。以下は、Pythonを使用してWord文書にテーブルを追加する手順です。
- Documentクラスのオブジェクトを作成します。
- DocumentBuilderクラスのオブジェクトを作成します。
- DocumentBuilder.start_table()メソッドを使用してテーブルを開始し、オブジェクト内のテーブルの参照を取得します。
- DocumentBuilder.insert_cell()メソッドを使用してセルを挿入します。
- auto_fit(aw.tables.AutoFitBehavior.FIXED_COLUMN_WIDTHS)メソッドを使用して自動フィットを設定します。
- セルの配置を設定します。
- DocumentBuilder.write()メソッドを使用してセルにテキストを挿入します。
- 必要に応じて、セルとテキストをセルに繰り返し挿入します。
- セルの挿入が完了したら、行を終了します。
- すべての行を挿入したら、テーブルを終了します。
- Document.save()メソッドを使用してWord文書を保存します。
次のコードサンプルは、Pythonを使用してWord文書にテーブルを挿入する方法を示しています。
import aspose.words as aw
# create document object
doc = aw.Document()
# create a document builder object
builder = aw.DocumentBuilder(doc)
# start table
table = builder.start_table()
# insert cell
builder.insert_cell()
table.auto_fit(aw.tables.AutoFitBehavior.AUTO_FIT_TO_CONTENTS)
# set formatting and add text
builder.cell_format.vertical_alignment = aw.tables.CellVerticalAlignment.CENTER
builder.write("This is row 1 cell 1")
# insert cell
builder.insert_cell()
builder.write("This is row 1 cell 2")
# end row
builder.end_row()
# insert another cell in the next row
builder.insert_cell()
# format row if required
builder.row_format.height = 100
builder.row_format.height_rule = aw.HeightRule.EXACTLY
# format cell and add text
builder.cell_format.orientation = aw.TextOrientation.UPWARD
builder.writeln("This is row 2 cell 1")
# insert another cell, set formatting and add text
builder.insert_cell()
builder.cell_format.orientation = aw.TextOrientation.DOWNWARD
builder.writeln("This is row 2 cell 2")
# end row
builder.end_row()
# end table
builder.end_table()
# save document
doc.save("out.docx")
出力
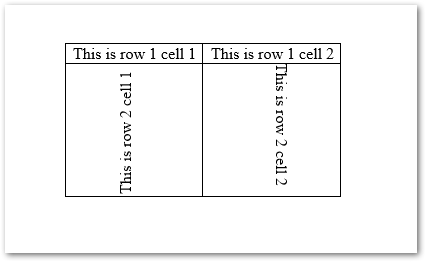
Pythonを使用してWord文書でテーブルを操作する方法の詳細をご覧ください。
Pythonを使用してWord文書にリストを作成する
以下は、Pythonを使用してWord文書にリストを作成する手順です。
- Documentクラスのオブジェクトを作成します。
- DocumentBuilderクラスのオブジェクトを作成します。
- DocumentBuilder.list_format.apply_number_default()メソッドを使用してフォーマットを設定します。
- DocumentBuilder.writeln(“Item 1”)メソッドを使用してアイテムを挿入します。
- DocumentBuilder.writeln(“Item 2”)メソッドを使用して2番目のアイテムを挿入します。
- リストの次のレベルにアイテムを挿入するには、DocumentBuilder.list_format.list_indent()メソッドを呼び出してアイテムを挿入します。
- DocumentBuilder.list_format.remove_numbers()メソッドを使用して、リストから番号を削除します。
- Document.save()メソッドを使用してWord文書を保存します。
次のコードサンプルは、Pythonを使用してWord文書にリストを作成する方法を示しています。
import aspose.words as aw
# create document object
doc = aw.Document()
# create a document builder object
builder = aw.DocumentBuilder(doc)
# set list formatting
builder.list_format.apply_number_default()
# insert item
builder.writeln("Item 1")
builder.writeln("Item 2")
# set indentation for next level of list
builder.list_format.list_indent()
builder.writeln("Item 2.1")
builder.writeln("Item 2.2")
# indent again for next level
builder.list_format.list_indent()
builder.writeln("Item 2.2.1")
builder.writeln("Item 2.2.2")
# outdent to get back to previous level
builder.list_format.list_outdent()
builder.writeln("Item 2.3")
# outdent again to get back to first level
builder.list_format.list_outdent()
builder.writeln("Item 3")
# remove numbers
builder.list_format.remove_numbers()
# save document
doc.save("out.docx")
出力
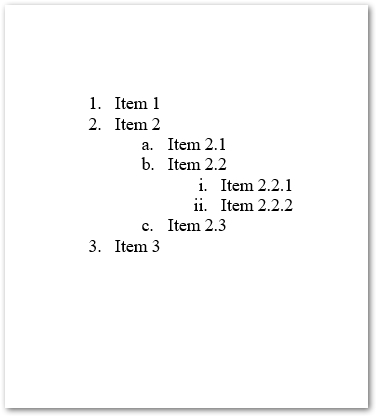
Pythonを使用したWord文書のリストの操作の詳細をお読みください。
Pythonを使用してWord文書に画像を挿入する
Word文書を操作している間は、画像などのグラフィカルオブジェクトを無視することはできません。それでは、Pythonを使用してWord文書に画像を動的に挿入する方法を見てみましょう。
- Documentクラスのオブジェクトを作成します。
- DocumentBuilderクラスのオブジェクトを作成します。
- DocumentBuilder.insert_image()メソッドを使用して画像を挿入し、画像ファイルのパスをパラメーターとして渡します。
- Document.save()メソッドを使用してWord文書を保存します。
次のコードサンプルは、Pythonを使用してWord文書に画像を挿入する方法を示しています。
import aspose.words as aw
# create document object
doc = aw.Document()
# create a document builder object
builder = aw.DocumentBuilder(doc)
# add image
builder.insert_image("Logo.jpg")
# save document
doc.save("out.docx")
出力
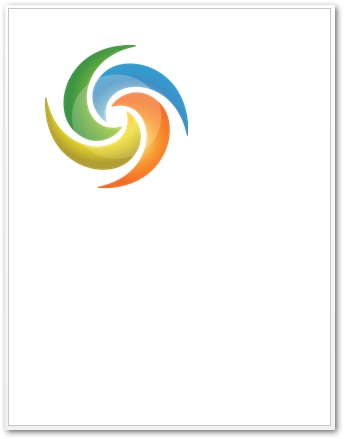
Pythonを使用してWord文書に目次を作成する
さまざまな場合、Word文書には目次(TOC)が含まれています。 TOCは、Word文書の内容の概要を示します。次の手順は、Pythonを使用してWord文書に目次を追加する方法を示しています。
- Documentクラスのオブジェクトを作成します。
- DocumentBuilderクラスのオブジェクトを作成します。
- DocumentBuilder.insert_table_of_contents()メソッドを使用して目次を挿入します。
- DocumentBuilder.insert_break(aw.BreakType.PAGE_BREAK)メソッドを使用して、目次の後にページ分割を挿入します。
- ドキュメントのコンテンツを追加/更新した後、Document.update_fields()メソッドを使用して目次を更新します。
- Document.save()メソッドを使用してWord文書を保存します。
次のコードサンプルは、Pythonを使用してWord文書に目次を挿入する方法を示しています。
import aspose.words as aw
# create document object
doc = aw.Document()
# create a document builder object
builder = aw.DocumentBuilder(doc)
# insert table of contents
builder.insert_table_of_contents("\\o \"1-3\" \\h \\z \\u")
# start the actual document content on the second page
builder.insert_break(aw.BreakType.PAGE_BREAK)
# add content
builder.paragraph_format.style_identifier = aw.StyleIdentifier.HEADING1
builder.writeln("Heading 1")
builder.paragraph_format.style_identifier = aw.StyleIdentifier.HEADING2
builder.writeln("Heading 1.1")
builder.writeln("Heading 1.2")
builder.paragraph_format.style_identifier = aw.StyleIdentifier.HEADING1
builder.writeln("Heading 2")
builder.writeln("Heading 3")
builder.paragraph_format.style_identifier = aw.StyleIdentifier.HEADING2
builder.writeln("Heading 3.1")
builder.paragraph_format.style_identifier = aw.StyleIdentifier.HEADING3
builder.writeln("Heading 3.1.1")
builder.writeln("Heading 3.1.2")
builder.writeln("Heading 3.1.3")
builder.paragraph_format.style_identifier = aw.StyleIdentifier.HEADING2
builder.writeln("Heading 3.2")
builder.writeln("Heading 3.3")
# the newly inserted table of contents will be initially empty
# it needs to be populated by updating the fields in the document
doc.update_fields()
# save document
doc.save("out.docx")
出力
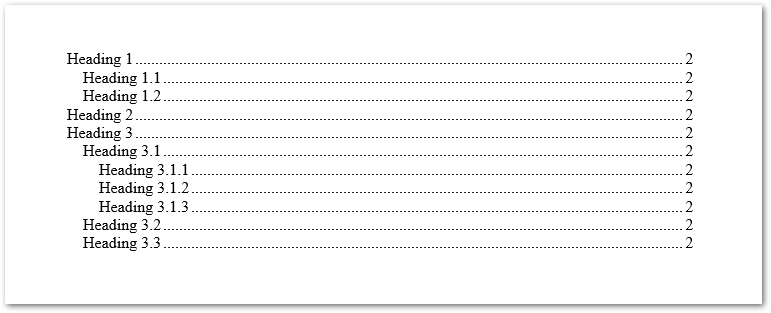
Pythonを使用した目次の操作の詳細をご覧ください。
続きを読む
この記事では、Word文書を作成し、さまざまな要素を挿入するための基本的な操作について説明しました。 Aspose.Words for Pythonには、ドキュメントを使用して探索できる他の機能も多数用意されています。
無料のAPIライセンスを取得する
評価の制限なしにAspose.Words for Pythonを使用するために、一時ライセンスを取得することができます。
結論
この記事では、PythonでWordDOCXまたはDOCファイルを作成する方法を学びました。さらに、Word文書にテキスト、画像、表、リスト、目次を動的に挿入する方法を見てきました。 APIを試して、フォーラムを介してフィードバックやクエリを共有できます。
関連項目
ヒント:Asposeは、無料のオンラインPowerPoint to Word Converterを提供しており、プレゼンテーションからWord文書をすばやく作成できます。