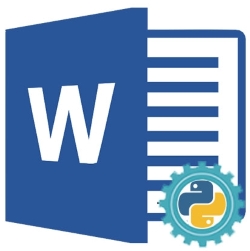
MS Wordを自動化して、新しいWord文書(DOCまたはDOCX)を作成したり、既存の文書を編集または変更したり、MicrosoftOfficeを使用せずに他の形式に変換したりできます。 Python MS Wordの自動化により、MSWordのユーザーインターフェイスを介して実行できるすべてのアクションをプログラムで実行できます。この記事では、MS Wordを自動化して、Pythonを使用してWord文書を作成、編集、または変換する方法を学習します。
この記事では、Pythonを使用してプログラムでWord文書を生成および操作するために必要なすべての基本機能について説明します。この記事には、次のトピックが含まれています。
- Word文書を作成、編集、または変換するPython MS Word Automation API
- Word文書を作成する
- Word文書を編集または変更する
- Word文書内のテキストを検索して置換する
- Word文書を変換する
- Word文書を解析する
Word文書を作成、編集、または変換するPython MS Word Automation API
Wordの自動化には、Aspose.Words for PythonAPIを使用します。これは、Word文書をプログラムで作成、編集、または分析するための、完全で機能豊富なWord自動化ソリューションです。 APIのDocumentクラスは、Wordドキュメントを表します。 APIは、テキスト、画像、およびその他のコンテンツをドキュメントに挿入するためのさまざまなメソッドを提供するDocumentBuilderクラスを提供します。このクラスでは、フォント、段落、およびセクションの書式を指定することもできます。 APIのRunクラスは、同じフォント形式の文字の実行を表します。次のpipコマンドを使用して、PyPIからPythonアプリケーションにライブラリをインストールしてください。
pip install aspose-words
Pythonを使用してWord文書を作成する
以下の手順に従って、プログラムでWord文書を作成できます。
- まず、Documentクラスのインスタンスを作成します。
- 次に、Documentオブジェクトを引数としてDocumentBuilderクラスのインスタンスを作成します。
- その後、要素を挿入/書き込みして、DocumentBuilderオブジェクトを使用してテキスト、段落、表、または画像を追加します。
- 最後に、出力ファイルのパスを引数としてsave()メソッドを呼び出し、作成されたファイルを保存します。
次のコードサンプルは、Pythonを使用してWord文書(DOCX)を作成する方法を示しています。
import aspose.words as aw
# This code example demonstrates how to create a new Word document using Python.
# Create document object
doc = aw.Document()
# Create a document builder object
builder = aw.DocumentBuilder(doc)
# Specify font formatting Font
font = builder.font
font.size = 32
font.bold = True
font.name = "Arial"
font.underline = aw.Underline.SINGLE
# Insert text
builder.writeln("Welcome")
builder.writeln()
# Set paragraph formatting
font.size = 14
font.bold = False
font.name = "Arial"
font.underline = aw.Underline.NONE
paragraphFormat = builder.paragraph_format
paragraphFormat.first_line_indent = 8
paragraphFormat.alignment = aw.ParagraphAlignment.JUSTIFY
paragraphFormat.keep_together = True
# Insert paragraph
builder.writeln('''Aspose.Words for Python is a class library that enables your applications to perform a great range of document processing tasks.
It supports most of the popular document formats such as DOC, DOCX, RTF, HTML, Markdown, PDF, XPS, EPUB, and others.
With the API, you can generate, modify, convert, render, and print documents without third-party applications or Office Automation.
''')
builder.writeln()
# Insert a Table
font.bold = True
builder.writeln("This is a sample table")
font.bold = False
# Start table
table = builder.start_table()
# Insert cell
builder.insert_cell()
table.auto_fit(aw.tables.AutoFitBehavior.AUTO_FIT_TO_CONTENTS)
# Set formatting and add text
builder.cell_format.vertical_alignment = aw.tables.CellVerticalAlignment.CENTER
builder.write("Row 1 cell 1")
builder.insert_cell()
builder.write("Row 1 cell 2")
builder.end_row()
builder.insert_cell()
builder.write("Row 2 cell 1")
builder.insert_cell()
builder.write("Row 2 cell 2")
builder.end_row()
# End table
builder.end_table()
builder.writeln()
# Insert image
builder.insert_image("C:\\Files\\aspose-icon.png")
# Save document
doc.save("C:\\Files\\sample_output.docx")
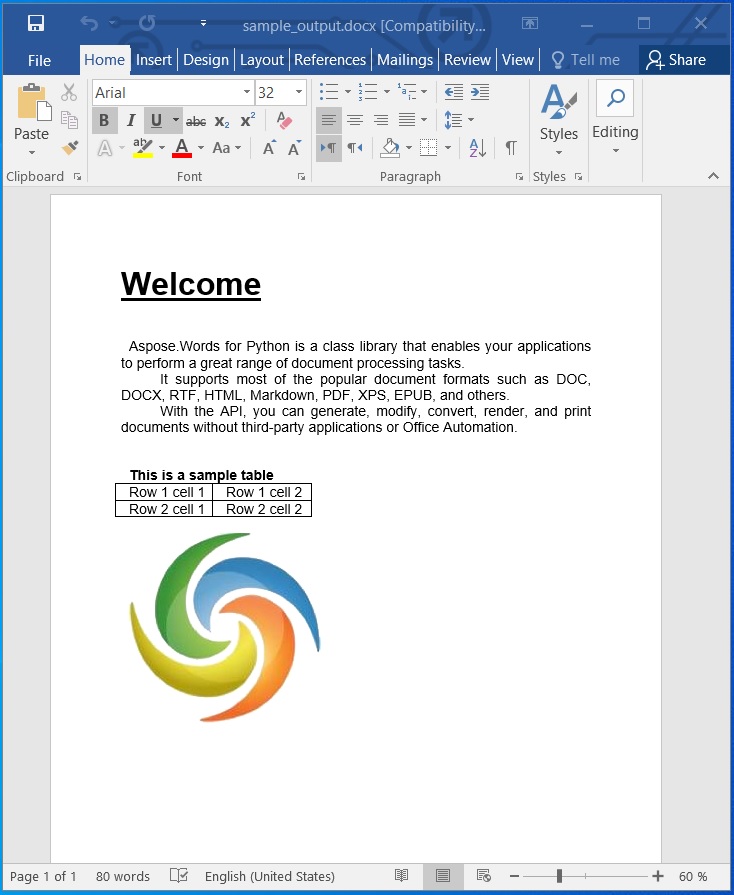
Pythonを使用してWord文書を作成します。
Pythonを使用してWord文書を編集または変更する
前のセクションでは、Word文書を作成しました。それでは、それを編集して、ドキュメントの内容を変更しましょう。以下の手順に従って、Word文書を編集できます。
- まず、Documentクラスを使用して既存のWord文書を読み込みます。
- 次に、インデックスで特定のセクションにアクセスします。
- 次に、Runクラスのオブジェクトとして最初の段落のコンテンツにアクセスします。
- その後、アクセスした段落の更新するテキストを設定します。
- 最後に、出力ファイルパスを指定してsave()メソッドを呼び出し、更新されたファイルを保存します。
次のコードサンプルは、Pythonを使用してWord文書(DOCX)を編集する方法を示しています。
import aspose.words as aw
# This code example demonstrates how to edit an existing Word document.
# Load the document
doc = aw.Document("C:\\Files\\sample_output.docx")
# Initialize document builder
builder = aw.DocumentBuilder(doc)
# Access the paragraph
paragraph = doc.sections[0].body.paragraphs[0].runs[0]
paragraph.text = "This is an updated text!"
# Save the document
doc.save("C:\\Files\\sample_updated.docx")
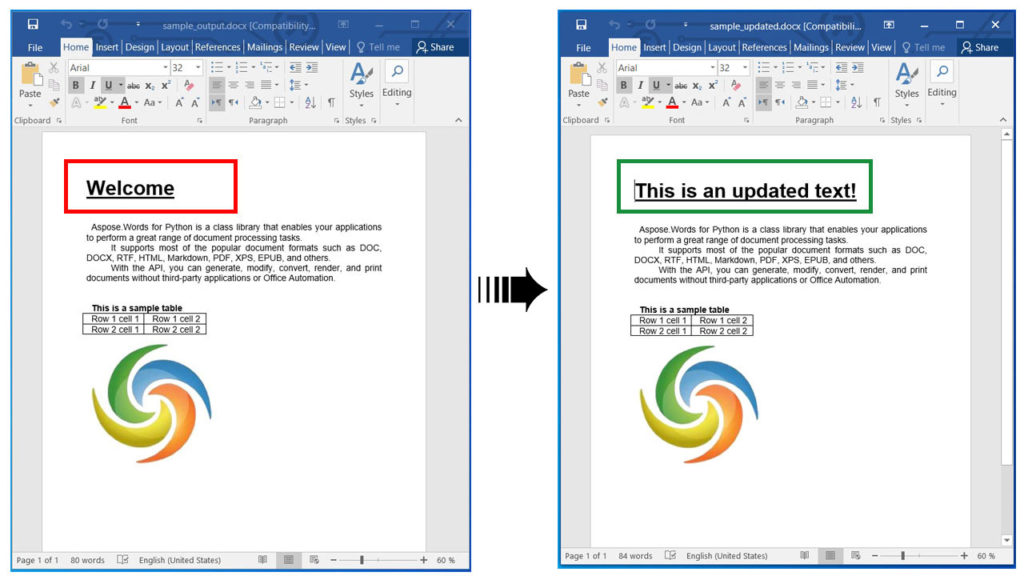
Pythonを使用してWord文書を編集または変更します。
Pythonを使用してWord文書内のテキストを検索して置換する
以下の手順に従って、任意のテキストを検索して新しいテキストに置き換えることもできます。
- まず、Documentクラスを使用してWord文書を読み込みます。
- 次に、FindReplaceOptionsクラスのインスタンスを作成します。
- その後、replace()メソッドを呼び出します。検索文字列、置換文字列、およびFindReplaceOptionsオブジェクトを引数として受け取ります。
- 最後に、出力ファイルパスを指定してsave()メソッドを呼び出し、更新されたファイルを保存します。
次のコードサンプルは、Pythonを使用してWord文書(DOCX)内の特定のテキストを検索して置換する方法を示しています。
import aspose.words as aw
# This code example demonstrates how to find and replace text in Word document.
# Load the document
doc = aw.Document("C:\\Files\\sample_output.docx")
# Update using find and replace
# Specify the search string and replace string using the Replace method.
doc.range.replace("Aspose.Words", "Hello",
aw.replacing.FindReplaceOptions(aw.replacing.FindReplaceDirection.FORWARD))
# Save the document
doc.save("C:\\Files\\find_and_replace.docx")
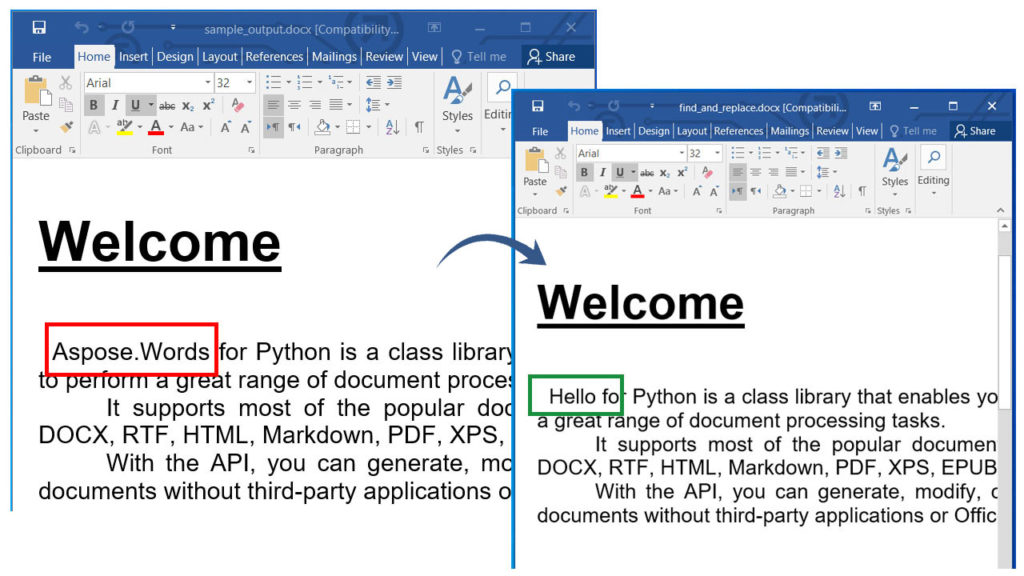
Word文書内のテキストを検索して置換します。
Pythonを使用してWord文書を変換する
Word文書をPDF、XPS、EPUB、HTML、JPG、PNGなどの他の形式に変換できます。Word文書をHTML Webページに変換するには、以下の手順に従ってください。
- まず、Documentクラスを使用してWord文書を読み込みます。
- 次に、Documentオブジェクトを引数としてHtmlSaveOptionsクラスのインスタンスを作成します。
- その後、cssstylesheettype、exportfontresources、resourcefolder、およびaliasプロパティを指定します。
- 最後に、出力ファイルパスとHtmlSaveOptionsオブジェクトを引数としてsave()メソッドを呼び出し、変換されたHTMLファイルを保存します。
次のコードサンプルは、Pythonを使用してWord文書(DOCX)をHTMLに変換する方法を示しています。
import aspose.words as aw
# This code example demonstrates how to convert a Word document to PDF.
# Load an existing Word document
doc = aw.Document("C:\\Files\\sample_output.docx")
# Specify save options
saveOptions = aw.saving.HtmlSaveOptions()
saveOptions.css_style_sheet_type = aw.saving.CssStyleSheetType.EXTERNAL
saveOptions.export_font_resources = True
saveOptions.resource_folder = "C:\\Files\\Resources"
saveOptions.resource_folder_alias = "C:/Files/resources"
# Save the converted document
doc.save("C:\\Files\\Converted.html", saveOptions)

同様に、Word文書を他のサポートされている形式に変換することもできます。ドキュメントでWordからEPUB、WordからPDF、WordドキュメントからMarkdown、WordからJPG、またはPNG画像に変換する方法の詳細をお読みください。
Pythonを使用してWord文書を解析する
以下の手順に従って、Word文書を解析し、コンテンツをプレーンテキストとして抽出できます。
- まず、Documentクラスを使用してWord文書を読み込みます。
- 次に、テキストを抽出して印刷します。
- 最後に、save()メソッドを呼び出して、Word文書をテキストファイルとして保存します。このメソッドは、出力ファイルのパスを引数として取ります。
次のコードサンプルは、Pythonを使用してWord文書(DOCX)を解析する方法を示しています。
import aspose.words as aw
# This code example demonstrates how to parse a Word document.
# Load the document
doc = aw.Document("C:\\Files\\Sample.docx")
# Extract text
print(doc.range.text)
# Save as plain text
doc.save("C:\\Files\\output.txt")
無料ライセンスを取得する
無料の一時ライセンスを取得して、評価の制限なしにライブラリを試すことができます。
結論
この記事では、次の方法を学びました。
- Pythonを使用してMSWordを自動化します。
- プログラムでWord文書を作成および編集します。
- DOCXファイルを解析または変換します。
- Pythonを使用してWord文書内のテキストを検索して置換します。
さらに、ドキュメントを使用して、Aspose.Words for PythonAPIの詳細を学ぶことができます。ご不明な点がございましたら、フォーラムまでお気軽にお問い合わせください。