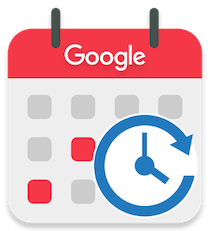
Google 캘린더는 회의와 같은 이벤트를 만들고 추적할 수 있는 일정 관리 서비스입니다. 캘린더에 이벤트를 기록하고 다가오는 이벤트에 대한 미리 알림을 받을 수 있습니다. 또한 Google에서는 프로그래밍 방식으로 캘린더 서비스를 사용할 수 있습니다. 따라서 애플리케이션 내에서 Google 캘린더를 사용하여 이벤트를 관리할 수 있습니다. 이 기사에서는 C#에서 프로그래밍 방식으로 Google 캘린더를 생성, 업데이트 및 삭제하는 방법을 배웁니다.
Google 캘린더 생성 및 조작을 위한 C# .NET API
Google 캘린더 서비스를 사용하려면 .NET용 Aspose.Email을 사용합니다. 이메일 처리, 이메일 클라이언트 작업, Google 협업 서비스 사용을 위한 다양한 기능을 제공하는 강력한 API입니다. 다음 명령을 사용하여 API의 DLL을 다운로드하거나 NuGet에서 설치할 수 있습니다.
PM> Install-Package Aspose.Email
작업을 시작하기 전에 애플리케이션이 Google 서비스와 통신할 수 있도록 Google 개발자 콘솔에서 프로젝트를 만들어야 합니다. 생성하려면 이 가이드를 따르세요.
Google 캘린더에 액세스하고 조작하려면 사용자 정보를 처리하고 인증을 수행하는 몇 가지 코드를 작성해야 합니다. Google 사용자의 경우 먼저 TestUser라는 클래스를 만든 다음 GoogleUser 클래스에서 상속합니다. 다음은 두 클래스의 완전한 구현입니다.
using System;
namespace Aspose.Email
{
internal enum GrantTypes
{
authorization_code,
refresh_token
}
public class TestUser
{
internal TestUser(string name, string eMail, string password, string domain)
{
Name = name;
EMail = eMail;
Password = password;
Domain = domain;
}
public readonly string Name;
public readonly string EMail;
public readonly string Password;
public readonly string Domain;
public static bool operator ==(TestUser x, TestUser y)
{
if ((object)x != null)
return x.Equals(y);
if ((object)y != null)
return y.Equals(x);
return true;
}
public static bool operator !=(TestUser x, TestUser y)
{
return !(x == y);
}
public static implicit operator string(TestUser user)
{
return user == null ? null : user.Name;
}
public override int GetHashCode()
{
return ToString().GetHashCode();
}
public override bool Equals(object obj)
{
return obj != null && obj is TestUser && this.ToString().Equals(obj.ToString(), StringComparison.OrdinalIgnoreCase);
}
public override string ToString()
{
return string.IsNullOrEmpty(Domain) ? Name : string.Format("{0}/{1}", Domain, Name);
}
}
public class GoogleUser : TestUser
{
public GoogleUser(string name, string eMail, string password)
: this(name, eMail, password, null, null, null)
{ }
public GoogleUser(string name, string eMail, string password, string clientId, string clientSecret)
: this(name, eMail, password, clientId, clientSecret, null)
{ }
public GoogleUser(string name, string eMail, string password, string clientId, string clientSecret, string refreshToken)
: base(name, eMail, password, "gmail.com")
{
ClientId = clientId;
ClientSecret = clientSecret;
RefreshToken = refreshToken;
}
public readonly string ClientId;
public readonly string ClientSecret;
public readonly string RefreshToken;
}
}
이제 Google 계정 인증을 처리할 도우미 클래스를 만들어야 합니다. 이 클래스의 이름을 GoogleOAuthHelper로 지정합니다. 다음은 이 클래스의 완전한 구현입니다.
using System;
using System.Diagnostics;
using System.IO;
using System.Net;
using System.Text;
using System.Threading;
using System.Windows.Forms;
namespace Aspose.Email
{
internal class GoogleOAuthHelper
{
public const string TOKEN_REQUEST_URL = "https://accounts.google.com/o/oauth2/token";
public const string SCOPE = "https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fcalendar" + "+" + "https%3A%2F%2Fwww.google.com%2Fm8%2Ffeeds%2F" + "+" + "https%3A%2F%2Fmail.google.com%2F"; // IMAP & SMTP
public const string REDIRECT_URI = "urn:ietf:wg:oauth:2.0:oob";
public const string REDIRECT_TYPE = "code";
internal static string GetAccessToken(TestUser user)
{
return GetAccessToken((GoogleUser)user);
}
internal static string GetAccessToken(GoogleUser user)
{
string access_token;
string token_type;
int expires_in;
GetAccessToken(user, out access_token, out token_type, out expires_in);
return access_token;
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string token_type, out int expires_in)
{
access_token = null;
token_type = null;
expires_in = 0;
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(TOKEN_REQUEST_URL);
request.CookieContainer = new CookieContainer();
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
string encodedParameters = string.Format("client_id={0}&client_secret={1}&refresh_token={2}&grant_type={3}",
System.Web.HttpUtility.UrlEncode(user.ClientId), System.Web.HttpUtility.UrlEncode(user.ClientSecret), System.Web.HttpUtility.UrlEncode(user.RefreshToken), System.Web.HttpUtility.UrlEncode(GrantTypes.refresh_token.ToString()));
byte[] requestData = Encoding.UTF8.GetBytes(encodedParameters);
request.ContentLength = requestData.Length;
if (requestData.Length > 0)
using (Stream stream = request.GetRequestStream())
stream.Write(requestData, 0, requestData.Length);
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
string responseText = null;
using (TextReader reader = new StreamReader(response.GetResponseStream(), Encoding.ASCII))
responseText = reader.ReadToEnd();
foreach (string sPair in responseText.Replace("{", "").Replace("}", "").Replace("\"", "").Split(new string[] { ",\n" }, StringSplitOptions.None))
{
string[] pair = sPair.Split(':');
string name = pair[0].Trim().ToLower();
string value = System.Web.HttpUtility.UrlDecode(pair[1].Trim());
switch (name)
{
case "access_token":
access_token = value;
break;
case "token_type":
token_type = value;
break;
case "expires_in":
expires_in = Convert.ToInt32(value);
break;
}
}
Debug.WriteLine("");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("-----------OAuth 2.0 authorization information-----------");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine(string.Format("Login: '{0}'", user.EMail));
Debug.WriteLine(string.Format("Access token: '{0}'", access_token));
Debug.WriteLine(string.Format("Token type: '{0}'", token_type));
Debug.WriteLine(string.Format("Expires in: '{0}'", expires_in));
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("");
}
internal static void GetAccessToken(TestUser user, out string access_token, out string refresh_token)
{
GetAccessToken((GoogleUser)user, out access_token, out refresh_token);
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string refresh_token)
{
string token_type;
int expires_in;
GoogleOAuthHelper.GetAccessToken(user, out access_token, out refresh_token, out token_type, out expires_in);
}
internal static void GetAccessToken(TestUser user, out string access_token, out string refresh_token, out string token_type, out int expires_in)
{
GetAccessToken((GoogleUser)user, out access_token, out refresh_token, out token_type, out expires_in);
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string refresh_token, out string token_type, out int expires_in)
{
string authorizationCode = GoogleOAuthHelper.GetAuthorizationCode(user, GoogleOAuthHelper.SCOPE, GoogleOAuthHelper.REDIRECT_URI, GoogleOAuthHelper.REDIRECT_TYPE);
GoogleOAuthHelper.GetAccessToken(authorizationCode, user, out access_token, out token_type, out expires_in, out refresh_token);
}
internal static void GetAccessToken(string authorizationCode, TestUser user, out string access_token, out string token_type, out int expires_in, out string refresh_token)
{
GetAccessToken(authorizationCode, (GoogleUser)user, out access_token, out token_type, out expires_in, out refresh_token);
}
internal static void GetAccessToken(string authorizationCode, GoogleUser user, out string access_token, out string token_type, out int expires_in, out string refresh_token)
{
access_token = null;
token_type = null;
expires_in = 0;
refresh_token = null;
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(TOKEN_REQUEST_URL);
request.CookieContainer = new CookieContainer();
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
string encodedParameters = string.Format("client_id={0}&code={1}&client_secret={2}&redirect_uri={3}&grant_type={4}", System.Web.HttpUtility.UrlEncode(user.ClientId), System.Web.HttpUtility.UrlEncode(authorizationCode), System.Web.HttpUtility.UrlEncode(user.ClientSecret), System.Web.HttpUtility.UrlEncode(REDIRECT_URI), System.Web.HttpUtility.UrlEncode(GrantTypes.authorization_code.ToString()));
byte[] requestData = Encoding.UTF8.GetBytes(encodedParameters);
request.ContentLength = requestData.Length;
if (requestData.Length > 0)
using (Stream stream = request.GetRequestStream())
stream.Write(requestData, 0, requestData.Length);
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
string responseText = null;
using (TextReader reader = new StreamReader(response.GetResponseStream(), Encoding.ASCII))
responseText = reader.ReadToEnd();
foreach (string sPair in responseText.Replace("{", "").Replace("}", "").Replace("\"", "").Split(new string[] { ",\n" }, StringSplitOptions.None))
{
string[] pair = sPair.Split(':');
string name = pair[0].Trim().ToLower();
string value = System.Web.HttpUtility.UrlDecode(pair[1].Trim());
switch (name)
{
case "access_token":
access_token = value;
break;
case "token_type":
token_type = value;
break;
case "expires_in":
expires_in = Convert.ToInt32(value);
break;
case "refresh_token":
refresh_token = value;
break;
}
}
Debug.WriteLine(string.Format("Authorization code: '{0}'", authorizationCode));
Debug.WriteLine(string.Format("Access token: '{0}'", access_token));
Debug.WriteLine(string.Format("Refresh token: '{0}'", refresh_token));
Debug.WriteLine(string.Format("Token type: '{0}'", token_type));
Debug.WriteLine(string.Format("Expires in: '{0}'", expires_in));
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("");
}
internal static string GetAuthorizationCode(TestUser acc, string scope, string redirectUri, string responseType)
{
return GetAuthorizationCode((GoogleUser)acc, scope, redirectUri, responseType);
}
internal static string GetAuthorizationCode(GoogleUser acc, string scope, string redirectUri, string responseType)
{
Debug.WriteLine("");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("-----------OAuth 2.0 authorization information-----------");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine(string.Format("Login: '{0}'", acc.EMail));
string authorizationCode = null;
string error = null;
string approveUrl = string.Format("https://accounts.google.com/o/oauth2/auth?redirect_uri={0}&response_type={1}&client_id={2}&scope={3}", redirectUri, responseType, acc.ClientId, scope);
AutoResetEvent are0 = new AutoResetEvent(false);
Thread t = new Thread(delegate ()
{
bool doEvents = true;
WebBrowser browser = new WebBrowser();
browser.AllowNavigation = true;
browser.DocumentCompleted += delegate (object sender, WebBrowserDocumentCompletedEventArgs e) { doEvents = false; };
Form f = new Form();
f.FormBorderStyle = FormBorderStyle.FixedToolWindow;
f.ShowInTaskbar = false;
f.StartPosition = FormStartPosition.Manual;
f.Location = new System.Drawing.Point(-2000, -2000);
f.Size = new System.Drawing.Size(1, 1);
f.Controls.Add(browser);
f.Load += delegate (object sender, EventArgs e)
{
try
{
browser.Navigate("https://accounts.google.com/Logout");
doEvents = true;
while (doEvents) Application.DoEvents();
browser.Navigate("https://accounts.google.com/ServiceLogin?sacu=1");
doEvents = true;
while (doEvents) Application.DoEvents();
HtmlElement loginForm = browser.Document.Forms["gaia_loginform"];
if (loginForm != null)
{
HtmlElement userName = browser.Document.All["Email"];
userName.SetAttribute("value", acc.EMail);
loginForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
loginForm = browser.Document.Forms["gaia_loginform"];
HtmlElement passwd = browser.Document.All["Passwd"];
passwd.SetAttribute("value", acc.Password);
loginForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
}
else
{
error = "Login form is not found in \n" + browser.Document.Body.InnerHtml;
return;
}
browser.Navigate(approveUrl);
doEvents = true;
while (doEvents) Application.DoEvents();
HtmlElement approveForm = browser.Document.Forms["connect-approve"];
if (approveForm != null)
{
HtmlElement submitAccess = browser.Document.All["submit_access"];
submitAccess.SetAttribute("value", "true");
approveForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
}
else
{
error = "Approve form is not found in \n" + browser.Document.Body.InnerHtml;
return;
}
HtmlElement code = browser.Document.All["code"];
if (code != null)
authorizationCode = code.GetAttribute("value");
else
error = "Authorization code is not found in \n" + browser.Document.Body.InnerHtml;
}
catch (Exception ex)
{
error = ex.Message;
}
finally
{
f.Close();
}
};
Application.Run(f);
are0.Set();
});
t.SetApartmentState(ApartmentState.STA);
t.Start();
are0.WaitOne();
if (error != null)
throw new Exception(error);
return authorizationCode;
}
}
}
C#에서 Google 캘린더 만들기
위의 구성을 완료하면 Google 캘린더 서비스로 작업을 진행할 수 있습니다. 다음은 C#에서 Google 캘린더를 만들고 업데이트하는 단계입니다.
- GmailClient.GetInstance(String, String) 메서드를 사용하여 GmailClient 클래스의 인스턴스를 IGmailClient 객체로 가져옵니다.
- Calendar 클래스의 인스턴스를 만들고 이름, 설명 및 기타 속성으로 초기화합니다.
- IGmailClient.CreateCalendar(Calendar) 메서드를 호출하여 Google 캘린더를 생성합니다.
- 달력의 반환된 ID를 가져옵니다.
다음 코드 샘플은 C#에서 Google 캘린더를 만드는 방법을 보여줍니다.
// Google 사용자 초기화
GoogleUser User = new GoogleUser("user", "email address", "password", "clientId", "client secret");
string accessToken;
string refreshToken;
// 액세스 토큰 가져오기
GoogleOAuthHelper.GetAccessToken(User, out accessToken, out refreshToken);
// Gmail 클라이언트 인스턴스 가져오기
using (IGmailClient client = GmailClient.GetInstance(accessToken, User.EMail))
{
// 캘린더 삽입 및 가져오기
Aspose.Email.Clients.Google.Calendar calendar = new Aspose.Email.Clients.Google.Calendar("summary - " + Guid.NewGuid().ToString(), null, null, "America/Los_Angeles");
// 캘린더 삽입 및 ID 검색
string id = client.CreateCalendar(calendar);
Aspose.Email.Clients.Google.Calendar cal = client.FetchCalendar(id);
}
C#에서 Google 캘린더 업데이트
다음은 C#에서 프로그래밍 방식으로 Google 캘린더를 업데이트하는 단계입니다.
- GmailClient.GetInstance(String, String) 메서드를 사용하여 GmailClient 클래스의 인스턴스를 IGmailClient 객체로 가져옵니다.
- IGmailClient.FetchCalendar(String) 메서드를 사용하여 해당 ID를 사용하여 캘린더 인스턴스를 가져옵니다.
- 캘린더의 속성을 업데이트하고 IGmailClient.UpdateCalendar(Calendar) 메서드를 호출하여 캘린더를 업데이트합니다.
다음 코드 샘플은 C#에서 Google 캘린더를 업데이트하는 방법을 보여줍니다.
// Gmail 클라이언트 인스턴스 가져오기
using (IGmailClient client = GmailClient.GetInstance(accessToken, User.EMail))
{
string id = "<<calendar ID>>";
// 캘린더 가져오기
Aspose.Email.Clients.Google.Calendar cal = client.FetchCalendar(id);
// 가져온 캘린더 정보 변경 및 캘린더 업데이트
cal.Description = "Description - " + Guid.NewGuid().ToString();
cal.Location = "Location - " + Guid.NewGuid().ToString();
// 캘린더 업데이트
client.UpdateCalendar(cal);
}
C#에서 Google 캘린더 삭제
.NET용 Aspose.Email을 사용하여 특정 캘린더를 삭제할 수도 있습니다. 다음은 이 작업을 수행하는 단계입니다.
- GmailClient.GetInstance(String, String) 메서드를 사용하여 GmailClient 클래스의 인스턴스를 IGmailClient 객체로 가져옵니다.
- IGmailClient.ListCalendars() 메서드를 사용하여 캘린더 목록을 가져옵니다.
- 목록을 반복하고 원하는 것을 필터링합니다.
- IGmailClient.DeleteCalendar(Calendar.Id) 메소드를 사용하여 캘린더를 삭제합니다.
다음 코드 샘플은 C#에서 Google 캘린더를 삭제하는 방법을 보여줍니다.
// Gmail 클라이언트 인스턴스 가져오기
using (IGmailClient client = GmailClient.GetInstance(accessToken, User.EMail))
{
// "캘린더 요약 - "에서 시작하는 요약이 있는 캘린더 액세스 및 삭제
string summary = "Calendar summary - ";
// 캘린더 목록 가져오기
ExtendedCalendar[] lst0 = client.ListCalendars();
foreach (ExtendedCalendar extCal in lst0)
{
// 선택한 캘린더 삭제
if (extCal.Summary.StartsWith(summary))
client.DeleteCalendar(extCal.Id);
}
}
무료 API 라이선스 받기
평가 제한 없이 Aspose.Email for .NET을 사용할 수 있는 무료 임시 라이선스를 얻을 수 있습니다.
결론
이 기사에서는 C#에서 프로그래밍 방식으로 Google 캘린더를 만드는 방법을 배웠습니다. 또한 C#에서 특정 Google 캘린더를 업데이트 및 삭제하는 방법을 살펴보았습니다. 또한 문서를 탐색하여 .NET용 Aspose.Email에 대해 자세히 알아볼 수 있습니다. 또한 포럼을 통해 질문할 수 있습니다.