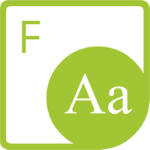
디지털 타이포그래피에서 글꼴은 문자의 모양에 사용할 특정 스타일을 정의합니다. 대부분의 경우 글꼴은 문서 및 웹 페이지에 사용되어 텍스트를 스타일화합니다. 각 글꼴은 문자의 크기, 무게, 스타일 및 인코딩에 대한 정보가 포함된 파일에 설명되어 있습니다. 글꼴은 다양한 파일 형식의 중요한 부분이므로 Aspose는 TrueType, CFF, OpenType, 및 유형1. 이 기사에서는 Aspose.Font for .NET과 함께 C#을 사용하여 글꼴에서 정보를 로드, 저장 및 추출하는 방법을 배웁니다.
- .NET 글꼴 조작 및 관리 API
- C#을 사용하여 CFF, TrueType 및 Type1 글꼴 로드 및 저장
- C#을 사용하여 글꼴 메트릭 정보 추출
- C#을 사용하여 글꼴에서 라틴 기호 감지
.NET 글꼴 조작 API - 무료 다운로드
Aspose.Font for .NET은 .NET 애플리케이션 내에서 C#을 사용하여 글꼴 관리 기능을 구현할 수 있는 온프레미스 API입니다. 글꼴에서 로드, 저장 및 정보 추출과 함께 API를 사용하면 필요한 경우 글리프 또는 텍스트를 렌더링할 수도 있습니다. API를 다운로드하거나 NuGet을 사용하여 .NET 애플리케이션 내에 설치할 수 있습니다.
PM> Install-Package Aspose.Font
C#을 사용하여 파일에서 글꼴 로드 또는 저장
글꼴 정보를 검색하기 위해 디지털 저장소에 저장된 파일에서 글꼴을 로드할 수 있습니다. .NET용 Aspose.Font는 CFF, TrueType 등과 같은 특정 유형의 글꼴을 처리하기 위해 별도의 클래스를 노출합니다. 다음은 글꼴을 로드하고 저장하는 단계입니다.
- FontDefinition 클래스를 사용하여 파일에서 글꼴을 로드합니다(파일 경로 또는 바이트 배열 사용).
- CffFont, TtfFont 또는 Type1Font 클래스를 사용하여 글꼴 정보에 액세스합니다.
- 파일을 저장합니다(필요한 경우).
CFF 글꼴 로드 및 저장
다음 코드 샘플은 C#을 사용하여 CFF 글꼴을 로드하고 저장하는 방법을 보여줍니다.
// 전체 예제 및 데이터 파일을 보려면 https://github.com/aspose-font/Aspose.Font-for-.NET으로 이동하십시오.
//글꼴을 로드할 바이트 배열
string dataDir = RunExamples.GetDataDir_Data();
byte[] fontMemoryData = File.ReadAllBytes(dataDir + "OpenSans-Regular.cff");
FontDefinition fd = new FontDefinition(FontType.CFF, new FontFileDefinition("cff", new ByteContentStreamSource(fontMemoryData)));
CffFont cffFont = Aspose.Font.Font.Open(fd) as CffFont;
//방금 로드된 CffFont 개체의 데이터 작업
//디스크에 CffFont 저장
//전체 경로가 있는 출력 글꼴 파일 이름
string outputFile = RunExamples.GetDataDir_Data() + "OpenSans-Regular_out.cff";
cffFont.Save(outputFile);
트루타입 글꼴 로드 및 저장
다음 코드 샘플은 C#을 사용하여 TrueType 글꼴을 로드하고 저장하는 방법을 보여줍니다.
// 전체 예제 및 데이터 파일을 보려면 https://github.com/aspose-font/Aspose.Font-for-.NET으로 이동하십시오.
//글꼴을 로드할 바이트 배열
string dataDir = RunExamples.GetDataDir_Data();
byte[] fontMemoryData = File.ReadAllBytes(dataDir + "Montserrat-Regular.ttf");
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new ByteContentStreamSource(fontMemoryData)));
TtfFont ttfFont = Aspose.Font.Font.Open(fd) as TtfFont;
//방금 로드된 TtfFont 개체의 데이터 작업
//디스크에 TtfFont 저장
//전체 경로가 있는 출력 글꼴 파일 이름
string outputFile = RunExamples.GetDataDir_Data() + "Montserrat-Regular_out.ttf";
ttfFont.Save(outputFile);
Type1 글꼴 로드 및 저장
다음 코드 샘플은 C#을 사용하여 Type1 글꼴을 로드하고 저장하는 방법을 보여줍니다.
// 전체 예제 및 데이터 파일을 보려면 https://github.com/aspose-font/Aspose.Font-for-.NET으로 이동하십시오.
string fileName = dataDir + "courier.pfb"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.Type1, new FontFileDefinition("pfb", new FileSystemStreamSource(fileName)));
Type1Font font = Aspose.Font.Font.Open(fd) as Type1Font;
C#을 사용하여 글꼴 메트릭 추출
.NET용 Aspose.Font를 사용하면 Ascender, Descender, TypoAscender, TypoDescender 및 UnitsPerEm과 같은 글꼴 메트릭 정보를 검색할 수도 있습니다. 다음은 그렇게 하는 단계입니다.
- FontDefinition 클래스를 사용하여 파일에서 TrueType 또는 Type1 글꼴을 로드합니다.
- Type1Font, TtfFont 등의 해당 글꼴 유형 클래스를 사용하여 글꼴을 엽니다.
- 글꼴의 메트릭 정보에 액세스합니다.
다음 코드 샘플은 C#을 사용하여 TrueType 및 Type1 글꼴에서 글꼴 메트릭을 추출하는 방법을 보여줍니다.
TrueType 글꼴에서 메트릭 추출
// 전체 예제 및 데이터 파일을 보려면 https://github.com/aspose-font/Aspose.Font-for-.NET으로 이동하십시오.
string fileName = dataDir + "Montserrat-Regular.ttf"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new FileSystemStreamSource(fileName)));
TtfFont font = Aspose.Font.Font.Open(fd) as TtfFont;
string name = font.FontName;
Console.WriteLine("Font name: " + name);
Console.WriteLine("Glyph count: " + font.NumGlyphs);
string metrics = string.Format(
"Font metrics: ascender - {0}, descender - {1}, typo ascender = {2}, typo descender = {3}, UnitsPerEm = {4}",
font.Metrics.Ascender, font.Metrics.Descender,
font.Metrics.TypoAscender, font.Metrics.TypoDescender, font.Metrics.UnitsPerEM);
Console.WriteLine(metrics);
//글꼴에서 개체 TtfCMapFormatBaseTable로 cmap 유니코드 인코딩 테이블을 가져와 기호 'A'의 글꼴 글리프에 대한 정보에 액세스합니다.
//또한 글꼴에 글리프에 액세스하기 위한 개체 TtfGlyfTable(테이블 'glyf')이 있는지 확인합니다.
Aspose.Font.TtfCMapFormats.TtfCMapFormatBaseTable cmapTable = null;
if (font.TtfTables.CMapTable != null)
{
cmapTable = font.TtfTables.CMapTable.FindUnicodeTable();
}
if (cmapTable != null && font.TtfTables.GlyfTable != null)
{
Console.WriteLine("Font cmap unicode table: PlatformID = " + cmapTable.PlatformId + ", PlatformSpecificID = " + cmapTable.PlatformSpecificId);
//'A' 기호에 대한 코드
char unicode = (char)65;
//'A'에 대한 글리프 인덱스
uint glIndex = cmapTable.GetGlyphIndex(unicode);
if (glIndex != 0)
{
//'A'에 대한 글리프
Glyph glyph = font.GetGlyphById(glIndex);
if (glyph != null)
{
//글리프 메트릭 인쇄
Console.WriteLine("Glyph metrics for 'A' symbol:");
string bbox = string.Format(
"Glyph BBox: Xmin = {0}, Xmax = {1}" + ", Ymin = {2}, Ymax = {3}",
glyph.GlyphBBox.XMin, glyph.GlyphBBox.XMax,
glyph.GlyphBBox.YMin, glyph.GlyphBBox.YMax);
Console.WriteLine(bbox);
Console.WriteLine("Width:" + font.Metrics.GetGlyphWidth(new GlyphUInt32Id(glIndex)));
}
}
}
Type1 글꼴에서 메트릭 추출
// 전체 예제 및 데이터 파일을 보려면 https://github.com/aspose-font/Aspose.Font-for-.NET으로 이동하십시오.
string fileName = dataDir + "courier.pfb"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.Type1, new FontFileDefinition("pfb", new FileSystemStreamSource(fileName)));
Type1Font font = Aspose.Font.Font.Open(fd) as Type1Font;
string name = font.FontName;
Console.WriteLine("Font name: " + name);
Console.WriteLine("Glyph count: " + font.NumGlyphs);
string metrics = string.Format(
"Font metrics: ascender - {0}, descender - {1}, typo ascender = {2}, typo descender = {3}, UnitsPerEm = {4}",
font.Metrics.Ascender, font.Metrics.Descender,
font.Metrics.TypoAscender, font.Metrics.TypoDescender, font.Metrics.UnitsPerEM);
Console.WriteLine(metrics);
C#을 사용하여 글꼴에서 라틴 기호 감지
.NET용 Aspose.Font를 사용하여 글리프 코드를 디코딩하여 글꼴에서 라틴 텍스트를 감지할 수도 있습니다. 다음은 이 작업을 수행하는 단계입니다.
- FontDefinition 클래스를 사용하여 파일에서 글꼴을 로드합니다.
- 해당 글꼴 클래스의 DecodeToGid() 메서드를 사용하여 GlyphId를 디코딩합니다.
다음 코드 샘플은 C#을 사용하여 TrueType 및 Type1 글꼴의 라틴 기호를 디코딩하는 방법을 보여줍니다.
트루타입 글꼴에서 라틴 기호 감지
// 전체 예제 및 데이터 파일을 보려면 https://github.com/aspose-font/Aspose.Font-for-.NET으로 이동하십시오.
string fileName = dataDir + "Montserrat-Regular.ttf"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new FileSystemStreamSource(fileName)));
TtfFont ttfFont = Aspose.Font.Font.Open(fd) as TtfFont;
bool latinText = true;
for (uint code = 65; code < 123; code++)
{
GlyphId gid = ttfFont.Encoding.DecodeToGid(code);
if (gid == null || gid == GlyphUInt32Id.NotDefId)
{
latinText = false;
}
}
if (latinText)
{
Console.WriteLine(string.Format("Font {0} supports latin symbols.", ttfFont.FontName));
}
else
{
Console.WriteLine(string.Format("Latin symbols are not supported by font {0}.", ttfFont.FontName));
}
Type1 글꼴에서 라틴 기호 감지
// 전체 예제 및 데이터 파일을 보려면 https://github.com/aspose-font/Aspose.Font-for-.NET으로 이동하십시오.
string fileName = dataDir + "courier.pfb"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.Type1, new FontFileDefinition("pfb", new FileSystemStreamSource(fileName)));
Type1Font font = Aspose.Font.Font.Open(fd) as Type1Font;
bool latinText = true;
for (uint code = 65; code < 123; code++)
{
GlyphId gid = font.Encoding.DecodeToGid(code);
if (gid == null || gid == GlyphUInt32Id.NotDefId)
{
latinText = false;
}
}
if (latinText)
{
Console.WriteLine(string.Format("Font {0} supports latin symbols.", font.FontName));
}
else
{
Console.WriteLine(string.Format("Latin symbols are not supported by font {0}.", font.FontName));
}
결론
이 기사에서는 C#을 사용하여 프로그래밍 방식으로 CFF, TrueType 및 Type1 글꼴을 로드하고 저장하는 방법을 살펴보았습니다. 또한 .NET 응용 프로그램 내의 TrueType 및 Type1 글꼴에서 글꼴 메트릭 정보를 추출하는 방법을 배웠습니다. 문서에서 .NET용 Aspose.Font가 제공하는 더 흥미로운 기능을 탐색할 수 있습니다. 더 많은 업데이트를 보려면 Aspose.Font 블로그를 계속 방문하십시오.