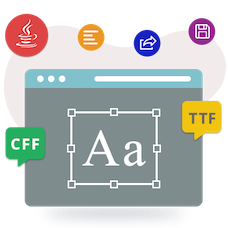
글꼴은 문서 및 웹 페이지 내에서 텍스트를 표시하는 데 중요한 역할을 합니다. 텍스트를 매력적으로 만들기 위해 멋진 문자를 사용할 수 있는 다양한 글꼴 모음을 사용할 수 있습니다. Aspose는 파일 형식 자동화를 다루기 때문에 글꼴 파일 형식으로 작업할 수 있는 전용 글꼴 조작 API를 출시했습니다. 이 기사에서는 Java 글꼴 API에 익숙해지고 Java 애플리케이션 내에서 CFF, TrueType, OpenType 및 Type1 글꼴을 사용하는 방법을 배웁니다.
자바 글꼴 조작 API
Aspose.Font for Java는 CFF, TrueType, Type1, EOT 및 OpenType. 또한 API는 글꼴 메트릭 검색과 지원되는 글꼴 유형을 사용하여 텍스트 렌더링을 지원합니다. 다음 구성을 사용하여 API를 다운로드하거나 Maven 기반 애플리케이션 내에 설치할 수 있습니다.
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-font</artifactId>
<version>20.10</version>
</dependency>
Java를 사용하여 CFF, TrueType 및 Type1 글꼴 로드
Java 프로그램 내에서 저장소에 있는 파일에서 글꼴을 쉽게 로드할 수 있습니다. 글꼴을 로드한 후에는 필요에 따라 추가 작업을 수행할 수 있습니다. 다음은 Aspose.Font for Java를 사용하여 글꼴을 로드하는 단계입니다.
- FontDefinition 클래스를 사용하여 TrueType, Type1 등과 같은 유형을 지정하여 글꼴을 로드합니다.
- CffFont, TtfFont 또는 Type1Font 클래스를 사용하여 FontDefinition 개체에서 각각 CFF, TrueType 및 Type1 글꼴에 액세스합니다.
Java를 사용하여 CFF 글꼴 로드
Java를 사용하여 파일에서 CFF 글꼴을 로드하는 방법입니다.
// 전체 예제 및 데이터 파일을 보려면 https://github.com/aspose-font/Aspose.Font-for-Java로 이동하십시오.
String fileName = Utils.getDataDir() + "OpenSans-Regular.cff"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.CFF, new FontFileDefinition("cff", new FileSystemStreamSource(fileName)));
CffFont ttfFont = (CffFont) Font.open(fd);
System.out.println("Font has been loaded");
Java를 사용하여 트루타입 글꼴 로드
다음 Java 코드 샘플은 TrueType 글꼴을 로드합니다.
// 전체 예제 및 데이터 파일을 보려면 https://github.com/aspose-font/Aspose.Font-for-Java로 이동하십시오.
String fileName = Utils.getDataDir() + "Montserrat-Regular.ttf"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new FileSystemStreamSource(fileName)));
TtfFont font = (TtfFont) Font.open(fd);
Java를 사용하여 Type1 글꼴 로드
다음 코드 샘플은 Java를 사용하여 Type1 글꼴을 로드하는 방법을 보여줍니다.
// 전체 예제 및 데이터 파일을 보려면 https://github.com/aspose-font/Aspose.Font-for-Java로 이동하십시오.
String fileName = Utils.getDataDir() + "courier.pfb"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.Type1, new FontFileDefinition("pfb", new FileSystemStreamSource(fileName)));
Type1Font font = (Type1Font) Font.open(fd);
Java를 사용하여 TrueType 또는 Type1 글꼴에서 글꼴 메트릭 추출
글꼴 파일에는 줄 간격, 아래 첨자와 위 첨자 배치, 정렬 등을 지정하는 데 사용되는 글꼴 정보도 포함되어 있습니다. Java용 Aspose.Font를 사용하면 Ascender, Descender, TypoAscender, TypoDescender를 포함한 글꼴 메트릭 정보를 추출할 수도 있습니다. 및 UnitsPerEm. 다음은 이 작업을 수행하는 단계입니다.
- FontDefinition 클래스를 사용하여 TrueType 또는 Type1 글꼴을 로드합니다.
- 글꼴 유형에 따라 TtfFont 또는 Type1Font 클래스를 사용하여 글꼴에 접근합니다.
- 글꼴의 메트릭 정보를 추출합니다.
Java를 사용하여 TrueType 글꼴에서 글꼴 메트릭 가져오기
// 전체 예제 및 데이터 파일을 보려면 https://github.com/aspose-font/Aspose.Font-for-Java로 이동하십시오.
String fileName = Utils.getDataDir() + "Montserrat-Regular.ttf"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new FileSystemStreamSource(fileName)));
TtfFont font = (TtfFont) Font.open(fd);
String name = font.getFontName();
System.out.println("Font name: " + name);
System.out.println("Glyph count: " + font.getNumGlyphs());
String metrics = MessageFormat.format(
"Font metrics: ascender - {0}, descender - {1}, typo ascender = {2}, typo descender = {3}, UnitsPerEm = {4}",
font.getMetrics().getAscender(), font.getMetrics().getDescender(),
font.getMetrics().getTypoAscender(), font.getMetrics().getTypoDescender(), font.getMetrics().getUnitsPerEM());
System.out.println(metrics);
//글꼴에서 개체 TtfCMapFormatBaseTable로 cmap 유니코드 인코딩 테이블을 가져와 기호 'A'의 글꼴 글리프에 대한 정보에 액세스합니다.
//또한 글꼴에 글리프에 액세스하기 위한 개체 TtfGlyfTable(테이블 'glyf')이 있는지 확인합니다.
TtfCMapFormatBaseTable cmapTable = null;
if (font.getTtfTables().getCMapTable() != null)
{
cmapTable = font.getTtfTables().getCMapTable().findUnicodeTable();
}
if (cmapTable != null && font.getTtfTables().getGlyfTable() != null)
{
System.out.println("Font cmap unicode table: PlatformID = " + cmapTable.getPlatformId() +
", PlatformSpecificID = " + cmapTable.getPlatformSpecificId());
//'A' 기호에 대한 코드
char unicode = (char)65;
//'A'에 대한 글리프 인덱스
long glIndex = cmapTable.getGlyphIndex(unicode);
if (glIndex != 0)
{
//'A'에 대한 글리프
Glyph glyph = font.getGlyphById(glIndex);
if (glyph != null)
{
//글리프 메트릭 인쇄
System.out.println("Glyph metrics for 'A' symbol:");
String bbox = MessageFormat.format(
"Glyph BBox: Xmin = {0}, Xmax = {1}" + ", Ymin = {2}, Ymax = {3}",
glyph.getGlyphBBox().getXMin(), glyph.getGlyphBBox().getXMax(),
glyph.getGlyphBBox().getYMin(), glyph.getGlyphBBox().getYMax());
System.out.println(bbox);
System.out.println("Width:" + font.getMetrics().getGlyphWidth(new GlyphUInt32Id(glIndex)));
}
}
}
Java를 사용하여 Type1 글꼴에서 글꼴 메트릭 추출
// 전체 예제 및 데이터 파일을 보려면 https://github.com/aspose-font/Aspose.Font-for-Java로 이동하십시오.
String fileName = Utils.getDataDir() + "courier.pfb"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.Type1, new FontFileDefinition("pfb", new FileSystemStreamSource(fileName)));
Type1Font font = (Type1Font) Font.open(fd);
String name = font.getFontName();
System.out.println("Font name: " + name);
System.out.println("Glyph count: " + font.getNumGlyphs());
String metrics = MessageFormat.format(
"Font metrics: ascender - {0}, descender - {1}, typo ascender = {2}, typo descender = {3}, UnitsPerEm = {4}",
font.getMetrics().getAscender(), font.getMetrics().getDescender(),
font.getMetrics().getTypoAscender(), font.getMetrics().getTypoDescender(), font.getMetrics().getUnitsPerEM());
System.out.println(metrics);
결론
이 기사에서는 Java를 사용하여 프로그래밍 방식으로 CFF, TrueType 및 Type1 글꼴을 사용하는 방법을 배웠습니다. 또한 특정 글꼴의 글꼴 메트릭 정보에 액세스하는 방법을 살펴보았습니다. 문서 및 소스 코드 샘플을 사용하여 Java 글꼴 조작 API에 대해 자세히 알아볼 수 있습니다.