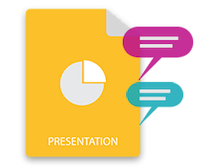
PowerPoint 프레젠테이션에서 주석은 슬라이드의 내용에 대한 피드백을 작성하는 데 사용됩니다. PowerPoint PPT/PPTX 프레젠테이션을 조작하는 동안 프로그래밍 방식으로 주석을 추가해야 할 수 있습니다. 이 기사에서는 Java에서 PowerPoint PPT 슬라이드에 주석을 추가하는 방법을 배웁니다. 또한 슬라이드 주석을 읽거나 제거하고 답글을 추가하는 방법을 다룹니다.
PowerPoint에서 주석 작업을 위한 Java API
Java용 Aspose.Slides는 PowerPoint PPT/PPTX 파일을 만들고 수정할 수 있는 널리 사용되는 프레젠테이션 조작 API입니다. 이 API를 사용하여 PowerPoint 프레젠테이션의 주석을 조작합니다. API의 JAR을 다운로드하거나 다음 Maven 구성을 사용하여 설치할 수 있습니다.
저장소:
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
의존:
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-slides</artifactId>
<version>22.2</version>
<classifier>jdk16</classifier>
</dependency>
Java에서 PowerPoint PPT 슬라이드에 주석 추가
PowerPoint 프레젠테이션에서 모든 주석은 특정 작성자에게 첨부됩니다. 반면, 각 주석에는 생성 시간, 추가된 슬라이드 및 위치와 같은 몇 가지 추가 정보가 포함되어 있습니다. 다음은 Java에서 PPT 슬라이드에 주석을 추가하는 단계입니다.
- 먼저 Presentation 클래스를 사용하여 프레젠테이션 파일을 로드하거나 새로 만듭니다.
- Presentation.getCommentAuthors().addAuthor(String, String) 메서드를 사용하여 새 작성자를 추가합니다.
- 개체에서 새로 생성된 작성자의 참조를 가져옵니다.
- 주석의 위치를 정의합니다.
- ICommentAuthor.getComments().addComment(String, ISlide, Point2D.Float, Date) 메서드를 사용하여 주석을 추가합니다.
- 마지막으로 Presentation.save(String, SaveFormat) 메서드를 사용하여 프레젠테이션을 저장합니다.
다음 코드 샘플은 Java에서 PPT 슬라이드에 주석을 추가하는 방법을 보여줍니다.
// 프레젠테이션 만들기 또는 로드
Presentation presentation = new Presentation("presentation.pptx");
try {
// 빈 슬라이드 추가 또는 기존 슬라이드의 참조 가져오기
presentation.getSlides().addEmptySlide(presentation.getLayoutSlides().get_Item(0));
// 작성자 추가
ICommentAuthor author = presentation.getCommentAuthors().addAuthor("Usman", "UA");
// 댓글 위치 설정
Point2D.Float point = new Point2D.Float(0.2f, 0.2f);
// 첫 번째 슬라이드에 슬라이드 주석 추가
author.getComments().addComment("Hello, this is slide comment", presentation.getSlides().get_Item(0), point, new Date());
// 프레젠테이션 저장
presentation.save("add-comment.pptx", SaveFormat.Pptx);
} finally {
if (presentation != null)
presentation.dispose();
}
다음은 위의 코드 샘플을 사용하여 추가한 주석의 스크린샷입니다.

Java의 PPT 슬라이드에 댓글 답글 추가
Aspose.Slides를 사용하면 댓글에 답글을 추가할 수도 있습니다. 답글 자체는 기존 댓글의 자식으로 나타나는 댓글입니다. 자바에서 PowerPoint PPT 슬라이드의 주석에 답글을 추가하는 방법을 살펴보겠습니다.
- 먼저 프레젠테이션 파일을 로드하거나 Presentation 클래스를 사용하여 새로 만듭니다.
- Presentation.getCommentAuthors().addAuthor(String, String) 메서드를 사용하여 새 작성자를 추가합니다.
- ICommentAuthor.getComments().addComment(String, ISlide, Point2D.Float, Date) 메서드를 사용하여 주석을 추가하고 반환된 개체를 가져옵니다.
- 같은 방법으로 다른 주석을 삽입하고 개체에서 참조를 가져옵니다.
- IComment.setParentComment(IComment) 메서드를 사용하여 두 번째 주석의 부모를 설정합니다.
- 마지막으로 Presentation.save(String, SaveFormat) 메서드를 사용하여 프레젠테이션을 저장합니다.
다음 코드 샘플은 Java에서 PPTX 프레젠테이션의 주석에 응답을 추가하는 방법을 보여줍니다.
// 프레젠테이션 만들기 또는 로드
Presentation presentation = new Presentation("presentation.pptx");
try {
// 빈 슬라이드 추가 또는 기존 슬라이드의 참조 가져오기
presentation.getSlides().addEmptySlide(presentation.getLayoutSlides().get_Item(0));
// 작성자 추가
ICommentAuthor author = presentation.getCommentAuthors().addAuthor("Usman", "UA");
// 댓글 위치 설정
Point2D.Float point = new Point2D.Float(0.2f, 0.2f);
// 첫 번째 슬라이드에 슬라이드 주석 추가
IComment comment = author.getComments().addComment("Hello, this is slide comment", presentation.getSlides().get_Item(0), point, new Date());
// 답글 댓글 추가
IComment subReply = author.getComments().addComment("This is the reply to the comment.", presentation.getSlides().get_Item(0), new Point2D.Float(10, 10), new Date());
subReply.setParentComment(comment);
// 답글 댓글 추가
IComment reply2 = author.getComments().addComment("This is second reply.", presentation.getSlides().get_Item(0), new Point2D.Float(10, 10), new Date());
reply2.setParentComment(comment);
// 프레젠테이션 저장
presentation.save("add-comment-reply.pptx", SaveFormat.Pptx);
} finally {
if (presentation != null)
presentation.dispose();
}
다음 스크린샷은 위 코드 샘플의 출력을 보여줍니다.

Java의 PPT 슬라이드에서 주석 읽기
Aspose.Slides를 사용하여 특정 작성자 또는 모든 작성자의 댓글을 읽을 수도 있습니다. 다음은 Java에서 PPT 슬라이드의 주석을 읽는 단계입니다.
- Presentation 클래스를 사용하여 프레젠테이션 파일을 로드합니다.
- Presentation.getCommentAuthors() 컬렉션을 사용하여 작성자 목록을 반복합니다.
- 각 작성자에 대해 ICommentAuthor.getComments() 메서드를 사용하여 주석을 반복합니다.
- 주석 세부 정보를 읽고 인쇄합니다.
다음 코드 샘플은 Java에서 PPT 슬라이드의 주석을 읽는 방법을 보여줍니다.
// 프레젠테이션 로드
Presentation presentation = new Presentation("add-comment.pptx");
try {
// 저자를 통해 루프
for (ICommentAuthor commentAuthor : presentation.getCommentAuthors())
{
// 각 작성자에 액세스
CommentAuthor author = (CommentAuthor) commentAuthor;
// 저자의 코멘트를 반복
for (IComment comment1 : author.getComments())
{
// 댓글 읽기
Comment comment = (Comment) comment1;
System.out.println("ISlide :" + comment.getSlide().getSlideNumber() + " has comment: " + comment.getText() +
" with Author: " + comment.getAuthor().getName() + " posted on time :" + comment.getCreatedTime() + "\n");
}
}
} finally {
if (presentation != null)
presentation.dispose();
}
Java의 PowerPoint PPT에서 주석 제거
이전 섹션에서 주석 컬렉션에서 주석에 액세스하여 주석을 읽는 방법을 살펴보았습니다. 마찬가지로 참조를 얻은 후 주석을 제거할 수 있습니다. 다음 코드 샘플은 Java의 PowerPoint 프레젠테이션에서 주석을 제거하는 방법을 보여줍니다.
// 프레젠테이션 로드
Presentation presentation = new Presentation("add-comment.pptx");
try {
// 첫 번째 슬라이드 가져오기
ISlide slide = presentation.getSlides().get_Item(0);
// 댓글 받기
IComment[] comments = slide.getSlideComments(null);
// 색인을 사용하여 원하는 주석 제거
comments[0].remove();
// 프레젠테이션 저장
presentation.save("remove-comments.pptx", SaveFormat.Pptx);
} finally {
if (presentation != null)
presentation.dispose();
}
무료 라이선스 받기
임시 라이선스를 요청하면 평가 제한 없이 Java용 Aspose.Slides를 사용할 수 있습니다.
결론
이 기사에서는 Java의 PowerPoint PPT 슬라이드에 주석을 추가하는 방법을 배웠습니다. 또한 프로그래밍 방식으로 댓글에 답글을 추가하는 방법을 다뤘습니다. 마지막으로 PPT 슬라이드에서 주석을 읽거나 제거하는 방법을 시연했습니다. 문서를 방문하여 Java용 Aspose.Slides에 대해 자세히 알아볼 수 있습니다. 또한 포럼에 질문을 게시할 수 있습니다.