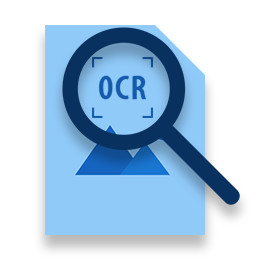
The OCR (Optical Character Recognition) technology lets you read and convert the text in the images or scanned documents to the machine-readable form. The OCR has various use cases that include reading codes from vouchers, making the text editable, self-service stores, converting printed documents into digital formats, and so on. Various OCR tools and libraries are available in the market, however, the reliability of the OCR results is a key factor. In this article, I’ll show you how to create your own OCR application and convert images to text programmatically using C++.
- C++ OCR Library to Convert Image to Text
- Convert Image Page to Text using C++
- Perform OCR for an Image with Single Line using C++
- Convert Particular Area of Image to Text in C++
C++ OCR Library to Convert Image to Text
Aspose offers a powerful C++ OCR library that uses deep learning to provide you more reliable and near to accurate OCR results. You can download the library files as well as a sample project from GitHub.
Convert Image Page to Text using C++
Lets first check out the scenario in which the image contains a multi-line text. This could be the case when you have a scanned book where each page of the book contains a bunch of text lines. The following are the steps to convert image to text in this case.
- Set the path of the image in a string variable.
- Prepare a buffer to store the OCR results.
- Perform OCR using aspose::ocr::page(const char *image_path, wchar_t *buffer, size_t buffer_size) function.
- Print the results or save them in a file.
The following code sample shows how to perform OCR and convert image to text using C++.
Perform OCR for an Image with Single Line using C++
In the previous example, we have converted an image having multiple text lines. However, there could be the case when the image contains only a single line of text such as the caption or a slogan. The following are the steps to perform OCR in such cases.
- Use a string variable to set the path of the image.
- Create a buffer to store the OCR results.
- Perform OCR using aspose::ocr::line(const char *image_path, wchar_t *buffer, size_t buffer_size) function.
- Save or print the OCR results.
The following code sample shows how to perform OCR on an image with a single line of text using C++.
Convert a Particular Area of Image to Text in C++
You can also customize the API to limit the area of the image where you want to perform the OCR. In this case, you can create a rectangle on the image to access the desired area. The following are the steps to extract text from a particular area of the image.
- Set the path of the image in a string variable.
- Prepare a buffer to store the OCR results.
- Perform OCR using aspose::ocr::page_rect(const char *image_path, wchar_t *buffer, size_t buffer_size, int x, int y, int w, int h) function.
- Print the OCR results.
The following code sample shows how to convert a particular area of the image to text using C++.
Conclusion
In this article, you have learned how to use Aspose’ OCR library to convert images to text in C++. We have seen how to perform OCR on an image with single or multiple lines of text as well as read text from a particular area of an image. You can learn more about Aspose.OCR for C++ using the documentation.