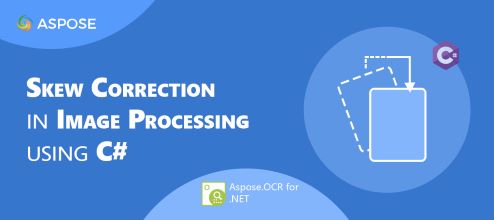
Skew correction in image processing is a task of image optimization. In computer vision, we need to correctly position our cameras and sensors, but this often leads to large distortions. Similarly, when scanning with scanners, sometimes alignment is nearly impossible. Due to this, scanned images and photographs have a slight skew (tilt). In this article, we will learn how to perform skew correction in image processing using C#.
The following topics shall be covered in this article:
- C# Skew Correction Image Processing API
- Detect Image Skew Angle
- Automatic Skew Correction
- Automatic Skew Correction with Preprocessing Filter
- Recognize Image Text with Automatic Skew Correction
- Manual Skew Correction
- Manual Skew Correction with Preprocessing Filter
C# Skew Correction Image Processing API
For skew corrections, we will be using the Aspose.OCR for .NET API. The API allows performing OCR on scanned images, smartphone photos, screenshots, areas of an image, and scanned PDFs. It enables saving the recognized text results in popular document formats.
Please either download the DLL of the API or install it using NuGet.
PM> Install-Package Aspose.OCR
Detect Image Skew Angle in C#
The API provides the CalculateSkew(string) method that calculates the skew angle of the provided source image. We can easily detect the skew angle of a text image by following the steps given below:
- Firstly, create an instance of the AsposeOCR class.
- Call the CalculateSkew() method. It takes the image path as an argument.
- Finally, show the calculated skew angle.
The following sample code shows how to calculate the skew angle of an image in C#.

The source image.
Skew angle: 5.8°
Automatic Skew Correction in C#
For automatic skew correction, we can enable the RecognitionSettings.AutoSkew property in recognition settings by following the steps given below:
- Firstly, create an instance of the AsposeOCR class.
- Next, initialize an object of the RecognitionSettings class.
- Then, set the AutoSkew property to true.
- After that, call the RecognizeImage() method with the source image and RecognitionSettings object.
- Finally, show the recognized text.
The following sample code shows how to apply auto skew correction in C#.
Automatic Skew Correction with Preprocessing Filter in C#
Alternatively, we can apply the AutoSkew preprocessing filter for automatic skew correction and save the corrected image by following the steps given below:
- Firstly, create an instance of the AsposeOCR class.
- Next, initialize an object of the PreprocessingFilter class.
- Then, add the AutoSkew() filter.
- After that, call the PreprocessImage() method to apply the filter on the source image.
- Finally, save the corrected image.
The following sample code shows how to apply auto skew correction with preprocessing filter in C#.
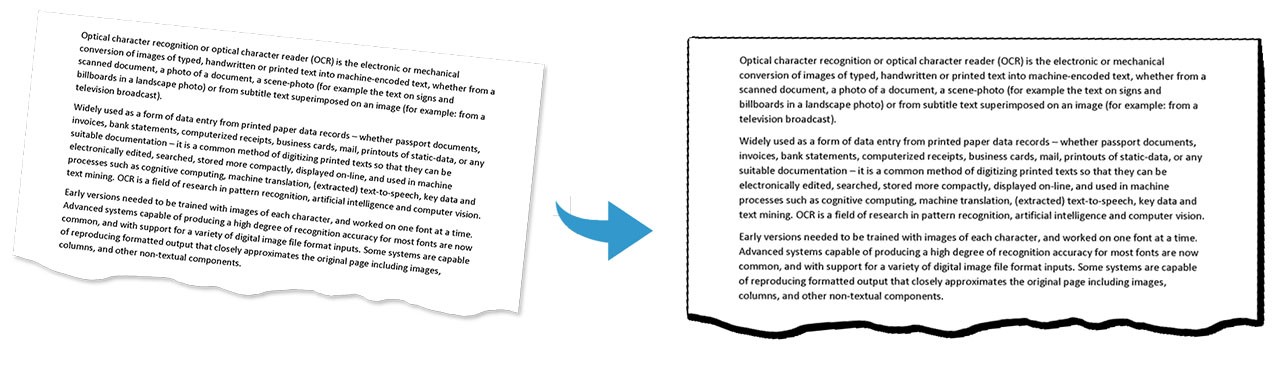
Automatic Skew Correction with Filter in C#.
Recognize Image Text with Automatic Skew Correction in C#
We can apply the AutoSkew preprocessing filter and recognize image text by following the steps given below:
- Firstly, create an instance of the AsposeOCR class.
- Next, initialize an object of the PreprocessingFilter class.
- Then, add the AutoSkew() filter.
- Meanwhile, create an instance of the RecognitionSettings class.
- Next, assign filters to the PreprocessingFilters property.
- After that, call the RecognizeImage() method with the source image and RecognitionSettings object.
- Finally, show the recognized text.
The following sample code shows how to apply auto-skew correction and recognize the text in C#.
Manual Skew Correction in C#
For manual skew correction, we can define the skew angle manually by specifying the SkewAngle property in the recognition settings by following the steps given below:
- Firstly, create an instance of the AsposeOCR class.
- Next, initialize an object of the RecognitionSettings class.
- Then, set the SkewAngle property.
- After that, call the RecognizeImage() method with the source image and RecognitionSettings object.
- Finally, show the recognized text.
The following sample code shows how to apply manual skew correction in C#.
Manual Skew Correction with Preprocessing Filter in C#
We can also define the skew angle manually by rotating the image to the specified degree by using the Rotate preprocessing filter.
Please follow the steps given below to manually apply the skew correction:
- Firstly, create an instance of the AsposeOCR class.
- Next, initialize an object of the PreprocessingFilter class.
- Then, add the Rotate() filter.
- Meanwhile, create an instance of the RecognitionSettings class.
- Next, assign filters to the PreprocessingFilters property.
- After that, call the RecognizeImage() method with the source image and RecognitionSettings object.
- Finally, show the recognized text.
The following sample code shows how to apply manual skew correction with preprocessing filter in C#.
Get a Free Evaluation License
You can get a free temporary license to try Aspose.OCR for .NET without evaluation limitations.
Conclusion
In this article, we have learned how to:
- perform OCR and recognize text on images;
- apply filters and process images;
- use recognition settings;
- save the corrected images using C#.
Besides skew correction in image processing using C#, you can learn more about Aspose.OCR for .NET API using the documentation. In case of any ambiguity, please feel free to contact us on our free support forum.