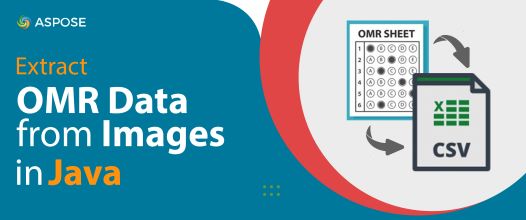
Optical mark recognition (OMR) is an electronic process that allows the reading and capturing of data marked by people on a special type of document form. This document form could be a test or a survey, consisting of bubble or square inputs filled by users. We can easily perform OMR operations on the scanned images of such survey forms, questionnaires, or test sheets and read the user inputs programmatically. In this article, we will learn how to perform OMR and extract data from an image in Java.
The following topics shall be covered in this article:
- Java OMR API to Extract Data from Image
- Extract Data from an Image
- Perform OMR and Extract Data from Multiple Images
- Extract OMR Data with Threshold
- Extract OMR Data with Recalculation
Java OMR API to Extract Data from Image
To perform OMR operations and extract data from supported image formats, we will be using the Aspose.OMR for Java API. It allows designing, creating, and recognizing answer sheets, tests, MCQ papers, quizzes, feedback forms, surveys, and ballots.
The OmrEngine class of the API handles the creation of the template and image processing. The getTemplateProcessor(String templatePath) method of this class creates the TemplateProcessor instance for processing templates and images. We can recognize an image using the recognizeImage(String imagePath) method. It returns all OMR elements as a RecognitionResult class instance. The getCsv() method of this class generates a CSV string with recognition results. The recalculate(RecognitionResult result, int recognitionThreshold) method updates the recognition result using fine-tuned parameters.
Please either download the JAR of the API or add the following pom.xml configuration in a Maven-based Java application.
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>http://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-omr</artifactId>
<version>19.12</version>
</dependency>
Extract Data from an Image in Java
We need the prepared OMR template (.omr) along with the image of user-filled forms/sheets to perform the OMR operation. We can perform an OMR operation on an image and extract data by following the steps given below:
- Firstly, create an instance of the OmrEngine class.
- Next, call the getTemplateProcessor() method and initialize TemplateProcessor class object. It takes the OMR template file path as an argument.
- Then, get the RecognitionResult object by calling the recognizeImage() method with the image path as an argument.
- After that, get recognition results as CSV strings using the getCsv() method.
- Finally, save the CSV result as a CSV file on the local disk.
The following code sample shows how to extract OMR data from an image in CSV format using Java.
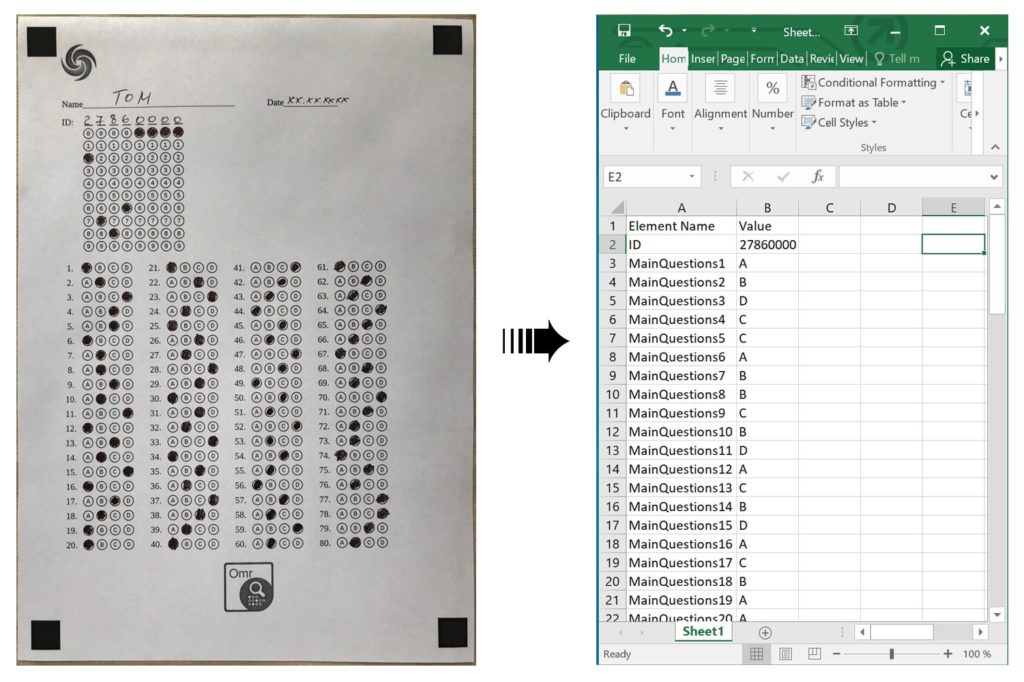
Perform OMR and Extract Data from an image in Java.
Please download the OMR template used in this blog post.
Perform OMR and Extract Data from Multiple Images
We can perform OMR operations on multiple images and extract data in a separate CSV file for each image by following the steps mentioned earlier. However, we need to repeat steps # 3, 4, and 5 for all the images one by one.
The following code sample shows how to extract OMR data from multiple images using Java.
Extract OMR Data with Threshold in Java
We can perform OMR operations with a threshold value (0 to 100) depending on the requirements. The higher the value of the threshold makes the API more strict in highlighting the answers. Please follow the steps mentioned earlier to perform OMR with the threshold. However, we just need to call the overloaded recognizeImage(string, int32) method in step # 3. It takes the image file path and the threshold value as arguments.
The following code sample shows how to perform OMR with threshold value using Java.
Extract OMR Data with Recalculation in Java
In certain cases, we may need to recalculate the OMR results with different threshold values. For this purpose, we can configure the API to automatically recalculate using TemplateProcessor.recalculate() method. It allows processing an image multiple times by changing the threshold setting to get the desired result. We can perform OMR operation with recalculation by following the steps given below:
- Firstly, create an instance of the OmrEngine class.
- Next, call the getTemplateProcessor() method and initialize TemplateProcessor class object. It takes the OMR template file path as an argument.
- Then, get the RecognitionResult object by calling the recognizeImage() method with the image path as an argument.
- Next, export recognition results as a CSV string using the getCsv() method.
- Then, save the CSV result as a CSV file on the local disk.
- Next, call the recalculate() method. It takes the RecognitionResult object and the threshold value as arguments.
- After that, export recognition results as a CSV string using the getCsv() method.
- Finally, save the CSV result as a CSV file on the local disk.
The following code sample shows how to perform OMR with the recalculation method using Java.
Get a Free License
You can get a free temporary license to try the library without evaluation limitations.
Conclusion
In this article, we have learned how to:
- perform OMR operation on images;
- extract data in CSV format programmatically;
- apply threshold setting while performing OMR on images;
- recalculate OMR results in an automotive process using Java.
Besides, extracting data from an image in Java, you can learn more about Aspose.OMR for Java API using the documentation. In case of any ambiguity, please feel free to contact us on our free support forum.