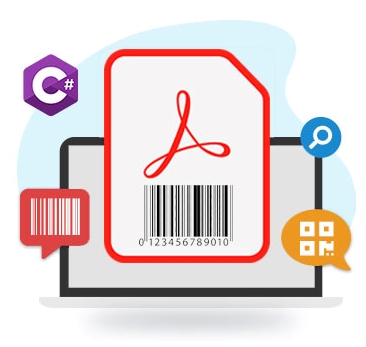
PDF is the most popular format for sharing and printing documents. Many organizations use PDF as a standard format for generating reports and sharing other official documents. In certain cases, we may need to embed specific data or information about the document, product, or company as a barcode image in PDF documents. We can add a machine-readable barcode image to a PDF document programmatically in .NET applications. In this article, we will learn how to add a barcode to PDF documents using C#.
The article shall cover the following topics:
- C# API to Add Barcode to PDF
- Create PDF Document and Add Barcode
- Add Barcode to Existing PDF Document
- Add QR Code to PDF Documents
- Read Barcode from PDF Document
C# API to Add Barcode to PDF
For adding barcodes to PDF documents, we will follow a two-step procedure. Firstly, we will be using the Aspose.PDF for .NET API to create or load a PDF document, then we will generate and add the barcode image to the PDF using the Aspose.BarCode for .NET API. Please either download the DLLs of the APIs or install them using NuGet.
PM> Install-Package Aspose.BarCode
PM> Install-Package Aspose.PDF
Create PDF Document and Add Barcode in C#
We can create a new PDF document and add a barcode image to the PDF by following the steps given below:
- Firstly, create an instance of the BarcodeGenerator class with the EncodeType and text to encode as arguments.
- Next, create an instance of the memory stream object
- Then, call the Save() method to save the barcode image to the memory stream.
- Next, create an instance of the Document class.
- Then, add a page to the newly created PDF document.
- Next, create an instance of the PdfFileMend class.
- Then, call the BindPdf() method with the Document object to bind it.
- Next, call the AddImage() method with the stream object and image position coordinates as arguments.
- After that, save the PDF using the Save() method. It takes the output PDF file path as an argument.
- Finally, call the Close() method to close the PdfFileMend object.
The following code example demonstrates how to create a new PDF document and add a barcode image using C#.
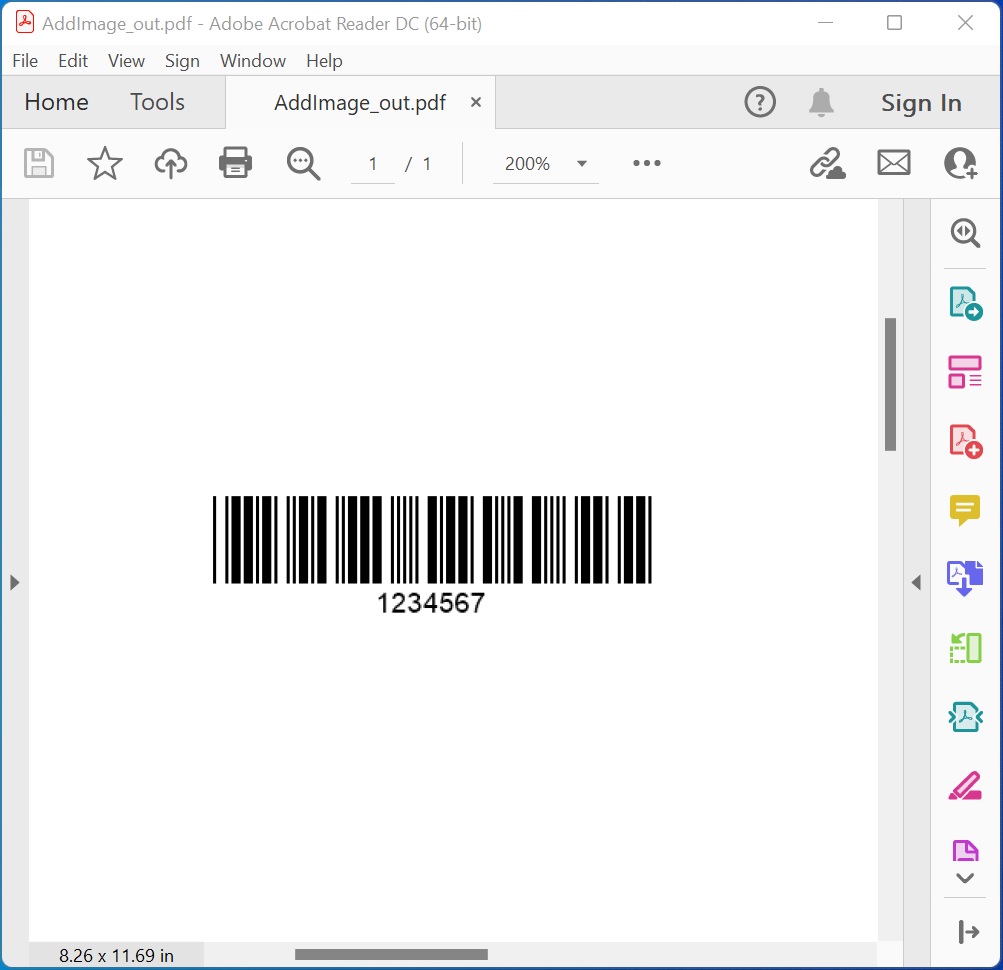
Create PDF Document and Add Barcode in C#.
Add Barcode to Existing PDF Document in C#
We can also add a barcode image to any page of existing PDF documents. The AddImage() method allows adding an image to the specified page of the PDF document at specified coordinates. We can insert an image into an existing PDF document by following the steps given below:
- Firstly, create an instance of the BarcodeGenerator class with the EncodeType and text to encode as arguments.
- Next, create an instance of the memory stream object
- Then, call the Save() method to save the barcode image to the memory stream.
- Next, load the PDF document using the Document class.
- Then, create an instance of the PdfFileMend class.
- Next, call the BindPdf() method with the Document object to bind it.
- Then, call the AddImage() method with the stream object, page number, and image position coordinates as arguments.
- After that, save the PDF using the Save() method. It takes the output PDF file path as an argument.
- Finally, call the Close() method to close the PdfFileMend object.
The following code example demonstrates how to add a barcode image to an existing PDF document using C#.
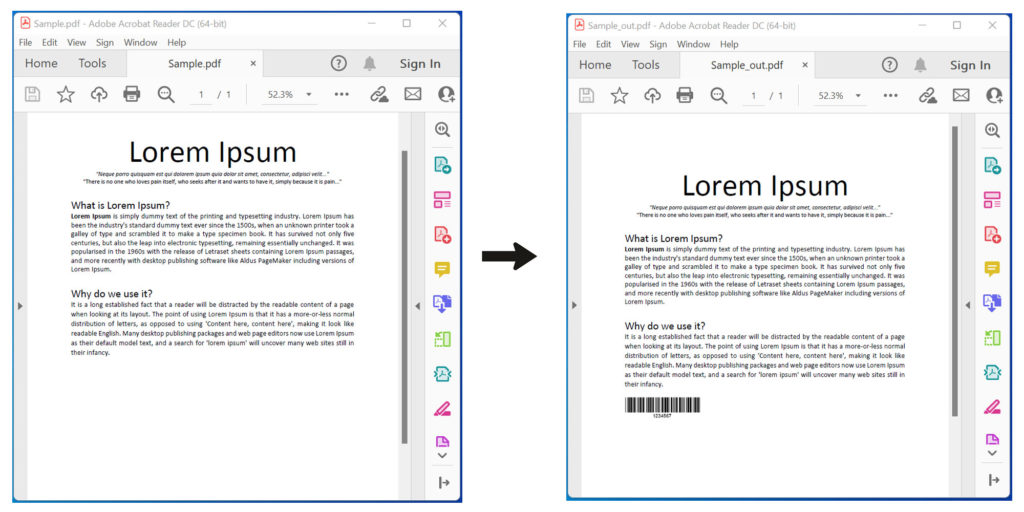
Add Barcode to Existing PDF Document in C#.
Add QR Code to PDF Documents in C#
Similarly, we can also add a QR code to the PDF documents by following the steps mentioned earlier. However, we just need to set the EncodeType as QR in the first step. We may also need to adjust the image position in step # 7.
The following code example demonstrates how to add a QR code to a PDF document using C#.

Add QR Code to PDF Documents in C#.
Read Barcode from PDF Document using C#
We can recognize any barcode image embedded on any of the pages of the PDF document using the PdfExtractor class. It allows extracting images from PDF, and then we will read the barcode information from the extracted image. We can read barcodes from the PDF documents by following the steps given below:
- Firstly, create an instance of the PdfExtractor class.
- Next, bind the input PDF document using the BindPdf() method.
- Then, set the page range for image extraction.
- Next, call the ExtractImage() method to extract the images.
- Then, save the image to stream in a loop.
- Next, create an instance of the BarCodeReader class with image stream and DecodeType as arguments.
- After that, call the ReadBarCodes() method to get the BarCodeResult object.
- Finally, show the barcode information.
The following code example demonstrates how to read a barcode image from a PDF document using C#.
Codetext found: 1234567
Symbology: Code39Standard
Get a Free License
You can get a free temporary license to try the library without evaluation limitations.
Conclusion
In this article, we have learned how to:
- create a PDF document programmatically;
- generate and add a barcode image to the PDF in C#;
- extract images from a PDF document using C#;
- read barcode image from a PDF document.
Besides, you can learn more about Aspose.BarCode for .NET API using the documentation. In case of any ambiguity, please feel free to contact us on the forum.