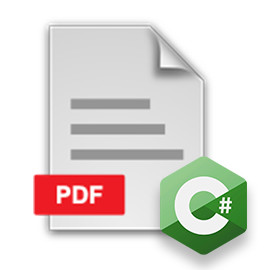
PDF is a platform-independent document format that keeps the formatting and layout of its content consistent across different operating systems or machines. This is the reason various organizations use PDF format for generating invoices, receipts, reports, and other business documents dynamically. Aspose.PDF for .NET is a PDF library that helps you implement PDF automation in .NET applications. It allows you to create, edit, parse and convert PDF files programmatically using C# or VB.NET. In this article, I’ll cover PDF generation features and show you how to create PDF files from scratch using C#.
I’ll start by creating a simple PDF document and then proceed to add other elements to the document. The following is the list of features I am going to demonstrate in this article.
- Create a PDF document using C#
- Apply text formatting in PDF using C#
- Create a multi-column PDF using C#
- Insert an image in a PDF using C#
- Create a table in a PDF using C#
- Add Hyperlinks to a PDF using C#
- Add bookmarks to a PDF using C#
C# PDF Library - Installation
You can install Aspose.PDF for .NET using NuGet Package Manager or Package Manager Console using the following command. Alternatively, it can be downloaded from the Downloads section.
Install-Package Aspose.Pdf
Create a PDF File using C#
Lets first create a PDF document containing a text fragment. The following are the steps to create a simple PDF document from scratch.
- Create an instance of Document class.
- Add a new Page to the Pages collection of the document.
- Add a new TextFragment to the paragraphs of the PDF.
- Create the PDF file using Document.Save() method.
The following code sample shows how to create a PDF file using C#.
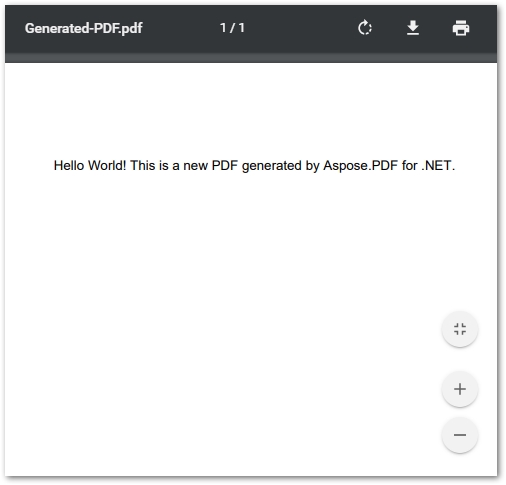
Apply Text Formatting in PDF using C#
Let’s now check out how to add text to a PDF document and use different formatting options. The following are the steps to perform this operation.
- Use Document class to create a new PDF document or load an existing one.
- Access the Page you want to place the text on.
- Create an object of TextFragment and set the text and other formatting options such as position, font, color, size, etc.
- Use the TextBuilder class to add the TextFragment object to the page.
- Use the Document.Save() method to create the PDF document.
The following code sample shows how to add formatted text in a PDF file using C#.

Create a Multi-Column PDF using C#
We often see that the text in newspapers, research articles, and other types of document is divided into two or more columns. In order to achieve this text division, Aspose.PDF for .NET allows creating a multi-column PDF. The following are the steps to create a multi-column PDF.
- Create a new PDF document using Document class.
- Add a new Page to the document using Document.Pages.Add() method.
- Set page margins using the Document.PageInfo.Margin properties.
- Create a new FloatingBox object and set ColumnCount, ColumnSpacing, and ColumnWidths properties.
- Create a new TextFragment and set its text.
- Add TextFragment to the FloatingBox using FloatingBox.Paragraphs.Add().
- Add FloatingBox to the page using Page.Paragraphs.Add().
- Save the PDF using the Document.Save() method.
The following code sample shows how to create a multi-column PDF using C#.

Insert Image in a PDF using C#
The following steps demonstrate how to insert images in PDF documents.
- Create a new PDF document or open an existing one using Document class.
- Get the page you want to insert an image in.
- Add the image to the page’s images.
- Use GSave, ConcatenateMatrix, and Do operators to place the image on the page.
- Save the PDF document using Document.Save() method.
The following code sample shows how to insert an image in PDF using C#.

Create Table in a PDF File using C#
The table is used to organize the data in the form of rows and columns and to provide a quick overview of the information. In order to create a table in the PDF document, follow the below steps.
- Create an object of Document class to create a new PDF or load an existing one.
- Access the page you want to create a table in.
- Create an instance of Table class.
- Set the table’s border and cells’ border using the BorderInfo class.
- Create and add a new Row to the Table.Rows collection.
- Add cells to the Row.Cells collection.
- Add the table to the page using Page.Paragraphs.Add() method.
- Save the PDF document using Document.Save() method.
The following code sample shows how to create a table in PDF using C#.
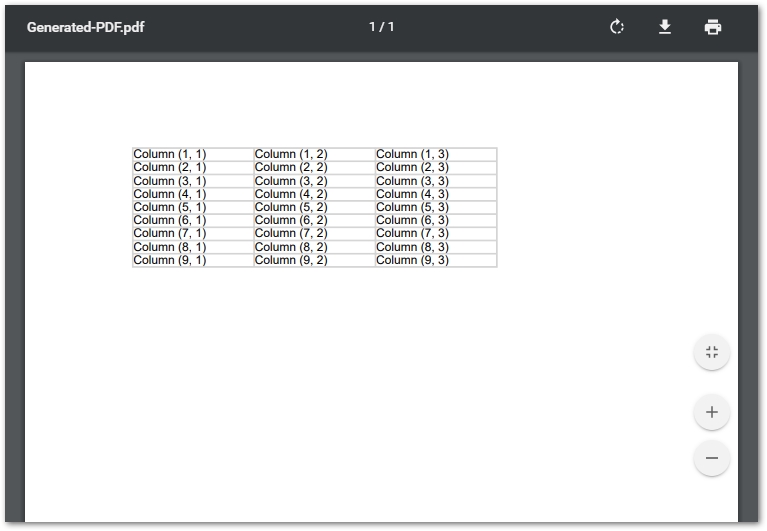
Add Annotation in a PDF using C#
Annotations are graphical objects that are used to provide additional information about the content in the PDF documents. PDF format supports various annotations including text, highlight, line, notes, and etc. The following are the steps to add an annotation in a PDF document.
- Create a new PDF document or load an existing one.
- Choose the page to which you want to add an annotation.
- Create a new annotation such as TextAnnotation, LineAnnotation, HighlightAnnotation, etc.
- Set the properties of the annotation.
- Add annotation to the Page.Annotations collection.
- Generate the PDF using the Document.Save() method.
The following code sample shows how to add an annotation to PDF using C#.

Add Hyperlinks to a PDF File using C#
You can also add hyperlinks to a PDF document using the Link Annotation. The following are the steps to perform this operation.
- Create a new Document object.
- Access the page where you want to add the hyperlink.
- Create an instance of the LinkAnnotation class.
- Set LinkAnnotation object’s properties including the action URI.
- Add the link to the Page.Annotations collection.
- Use the FreeTextAnnotation class to set the text of the hyperlink.
- Add FreeTextAnnotation object to the Page.Annotations collection.
- Create PDF document using Document.Save() method.
The following code sample shows how to add a hyperlink to a PDF document using C#.
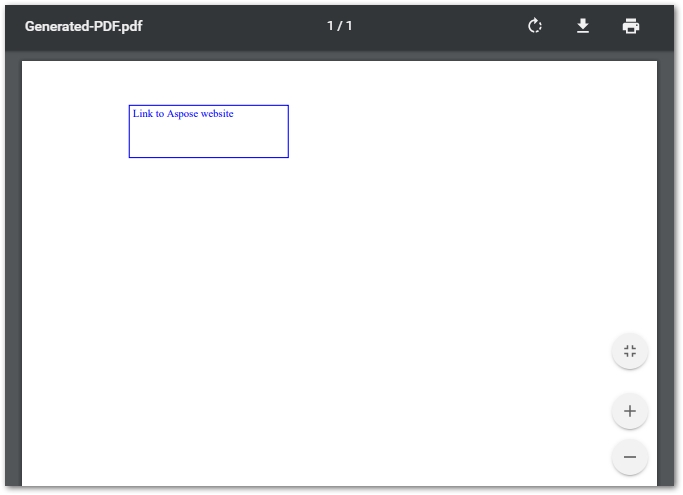
Create Bookmarks in a PDF using C#
Bookmarks are used to navigate to a particular section or page in PDF documents. Aspose.PDF for .NET allows you to add or manipulate bookmarks. The following are the steps to create and add a bookmark in a PDF document.
- Create a PDF document or open an existing one.
- Create a new bookmark object using the OutlineItemCollection class.
- Add bookmarks to Document.Outlines collection.
- Create the PDF document using Document.Save() method.
The following code sample shows how to create a PDF document with bookmarks using C#.
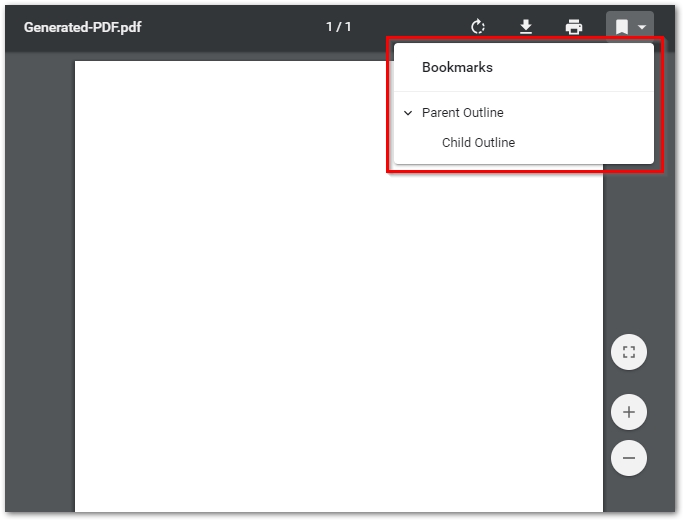
Conclusion
In this article, I have shown you how to create PDF files from scratch using C#. The step by step guide and code samples demonstrate how to add text, image, table, annotation, hyperlink, and bookmarks in the PDF files programmatically. You can explore other features of Aspose’s .NET PDF Library using the documentation.