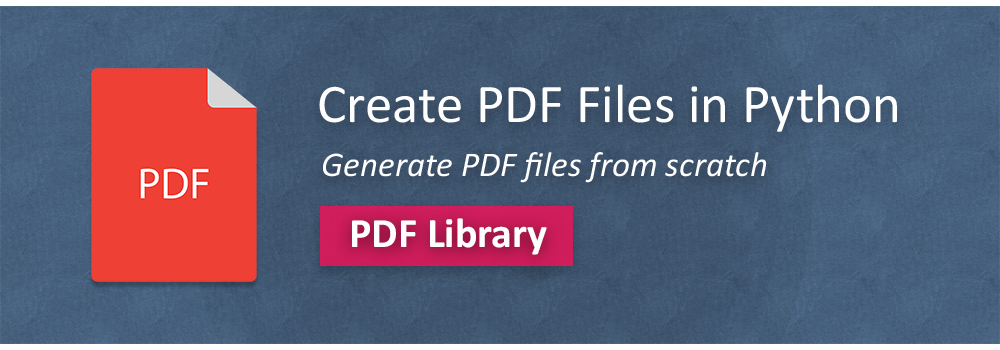
Learn how to easily create a PDF in Python from scratch with this comprehensive guide. This tutorial provides you with the best but easiest solution for creating PDF files in a few simple steps. So get rid of writing complex code and explore how to generate PDF files with our powerful and high-speed PDF library for Python.
Let’s have a look at how to create a basic PDF and insert text into it programmatically. The following sections provide you with the steps and code samples of generating, opening, and saving PDF files in Python.
- Python Library to Create PDF Files
- Create a PDF File using Python
- Open a PDF File
- Save PDF into PDF/A Format in Python
Python PDF Library
To generate PDF files in Python, we will use Aspose.PDF for Python. It is an amazing PDF manipulation library that you can use to create, edit and process PDF files with ease. Use the following pip command to install the library from PyPI.
pip install aspose-pdf
Once you have configured the library, you can proceed to create your first PDF file from scratch.
How to Create a PDF in Python
The following are the steps to create a simple PDF file from scratch and insert a piece of text in it.
- Create an instance of Document class.
- Add a new page using Document.pages.add() method and get its reference in an object.
- Create a new TextFragment object and set the text.
- Add text to the page using Page.paragraphs.add(TextFragment) method.
- Save the PDF file using Document.save(String) method.
The following code sample shows how to create a PDF file in Python.
Open a PDF in Python
In certain cases, you have to process the existing PDF files. For such cases, Aspose.PDF lets you open a PDF file using the file’s path or stream object. Also, the library allows you to open an encrypted PDF file using its password.
The following sections demonstrate how to open a PDF file using Python in different ways.
Open a PDF File using Path
The following Python code sample shows how to open a PDF using its path on disk.
Open a PDF using Stream
You can also load and open a PDF using file stream in Python, as shown in the following code sample.
Open an Encrypted PDF
Sometimes, you get the PDF file in encrypted form. To open an encrypted PDF, you need to provide the password. The following code sample shows how to open an encrypted PDF in Python.
Save PDF into PDF/A Format in Python
PDF format supports various standards, e.g. PDF/A, and each of them provides as well as prohibits certain features. In case you want to save a PDF file in PDF/A standard, you can do it in a couple of steps. This is how you can save a PDF as PDF/A in Python.
- Load the PDF file using Document class.
- Use Document.convert() method to convert PDF to desired PDF/A format.
- Save converted PDF using Document.save() method.
The following code sample shows how to save a PDF as PDF/A in Python.
Free Python PDF Generation Library
You can get a free temporary license and generate PDF files from scratch without any limitations.
Python PDF Library - Read More
You can explore more about the Python PDF library using documentation.
Conclusion
In this article, you have learned how to create PDF files from scratch in Python. Furthermore, the Python code samples have demonstrated how to insert text in a PDF and open existing PDF files in different ways. Also, we have covered how to save a PDF file in PDF/A standard. You can easily install Aspose.PDF in your application and generate your desired PDF files.